Course Outline in C#
Course Description
Table of Contents
C# is a powerful object-oriented programming language that enables developers to build a variety of applications for the Microsoft .NET platform. This course introduces programming concepts using the C# programming language. It makes no assumptions about prior programming knowledge. This course covers the basic concepts of C# which includes the data types, variables, control and repetition structures, arrays and methods.
You will receive comprehensive instructions and code in this course, which will help you gain the skills necessary to create any C# program from start. In addition, you can also watch the video presentation on our YouTube Channel for your reference.
Course Objectives and Goals
By the end of this course students should be able to:
- Explain how a computer program works
- Purpose and Usage of C#
- Learn the basic concepts in C# such as data types, variables, if statements, types of loops, arrays and methods.
- Apply the concepts to solve the exercises and assessments
- Create a simple console based project that implements the topics covered in this course
Prerequisites
As mentioned, this is intended for beginners. No prior knowledge is required but some background in computer programming would be helpful.
Students are required to install Visual Studio IDE Community Version for it will be the tool used to follow the lectures provided in this course. In case students could not afford to buy a laptop or computer, mobile applications used for coding will do.
Course Outline
- Introduction to Computer Programming
This is the first topic of the course and we will discuss about computer programming in general.
Computer programming is the process within computer science whereby a graphic representation of the steps we take to solve a problem is turned into a set of instructions a machine can understand.
- What is a Computer Program?
The second lesson of this course is a brief discussion on what is a computer program.
A computer program is a set of instructions that a computer follows to perform a task. Programs are written in a particular language which provides a structure for the programmer and uses specific instructions to control the sequence of operations that the computer carries out. The programming language used will depend on the type of task that the program is required to perform
- Programming languages
Lesson 3 will cover the discussion of the different types of computer programming languages. A programming language’s surface form is known as its syntax. A programming language is a formal language, which comprises a set of instructions that produce various kinds of output. Some of the programming languages are Java, Python, C++, C #and many more.
- Computer Programming Environment
This topic is about the tools to be used for computer programming, it will also cover how a program works and the different methods on running a program. Environment Setup is not an element of any Programming Language; it is the first step to be followed before setting on to write a program. We need to have the required software setup, i.e., installation on our PC which will be used to write computer programs, compile, and execute them.
- Introduction to C# and Features
This is the actual start of our C# discussion. This topic is intended to discuss C# and the technology behind it which is the .Net Framework, how it works and the features of C# itself.
C# is a programming language of .Net Framework. .NET Framework (pronounced as “dot net”) is a software framework developed by Microsoft that runs primarily on Microsoft Windows.
It includes a large class library named as Framework Class Library (FCL) and provides language interoperability (each language can use code written in other languages) across several programming languages.
- Visual Studio Environment
This part of the course is where students should familiarize the environment of the tool which is the Visual Studio. Microsoft Visual Studio is an integrated development environment (IDE) from Microsoft. It is used to develop computer programs, as well as websites, web apps, web services and mobile apps. This is the IDE or tool we are going to use for our coding sessions.
- C# Program Structure
This Tutorial Explains C# Program Structure And Basic Syntax. In this lesson, we will take C# programming forward and will be discussing the basics syntax and program structure that constitute a proper C# program. This is the lesson where we will create our first C# Program.
- C# Data Types
Enumerate and create a simple program that implements the concepts learned in out topic on data types.
- C# Variables and Operators
A variable is nothing more than a name given to a storage space that is accessible to our applications. Each variable in C# has a unique type that specifies its memory size and structure, the range of values that can be placed inside, and the range of actions that can be performed on the variable.
- C# Control Structures (if, if else, nested, switch statement)
This lesson is all about the decision control structures in C#, the lesson includes sample programs to better understand how to use and how the decision control structures in C# works.
Decision making structures requires the programmer to specify one or more conditions to be evaluated or tested by the program, along with a statement or statements to be executed if the condition is determined to be true, and optionally, other statements to be executed if the condition is determined to be false.
- C# Repetition Control Structures (while, do, for loop)
This lesson is all about the repetition control structures in C#, the lesson includes sample programs to better understand how to use and how the repetition control structures in C# works.
Arrays are not exclusive to C#; in fact, arrays were supported by almost every other programming and scripting language that existed prior to the advent of C#. In this lesson, we will focus on how array works and provide examples to better understand the purpose of an array.
An array is a data structure that allows you to store a group of data items of the same data type in a single variable. The items in an array are indexed by integers, starting with 0. You can access a particular item in an array by giving its index. Arrays are an integral feature of most programming languages. In this lesson, you will learn how to create and use an array in C#. You will also learn how to access individual objects in an array, and how to manipulate arrays as a whole. Finally, you will see instances of how arrays are used in programming applications.
This lesson is all about the methods in C#. The lesson includes sample program to better understand how a method works in C#.
A method is a function that is associated with a class. Methods are used to conduct operations on objects of the class. For example, the function print() can be used to print the contents of an object to the console. Methods can also be used to return values. For example, the method getName() can be used to return the name of an object. Methods are normally declared with the keyword method.
Assessments, Projects and Requirements
The course will divide the topics into terms. First term will cover topics from Introduction to Computer Programming to Computer Programming Environment. For this part, students should be able to write an essay about the importance of computer programming and how it can solve real world problems.
The second term is composed of topics from Introduction to C# and Features up to Variables and Operators in C#. Students should comply the requirements and source code activities for each topics covered in this term.
The third term covers the topics on C# Control Structures to C# Methods. It is again a requirement to comply of the specific activities for each and every topic on this term. Requirements also include the completion of the source code provided as part of the learning activities.
To encourage collaboration with your classmates, the final requirement is a group project.
Group project must consist of at least 3 members. The task of the group is to create a console based application wherein all of the theories and concepts discussed in this course should be applied.
It simply means that the project must consist of the application of the following:
- Application of proper data types to the variables
- Use different operators in the project
- Different decision control structures must also be visible in the program
- Use loop statements and arrays
- Use pre-defined methods and as well as to create your own user defined methods
Guidelines of the submission of requirements
- Make sure that it is your work; copy paste from your classmates is strictly not allowed.
- Double check that all exercises and activities are working
- Prepare a video presentation that discusses how did you solve the problem
- Include all the necessary file and folders of the activities
- Submission must be in a zip file with your complete name and name of the activity.
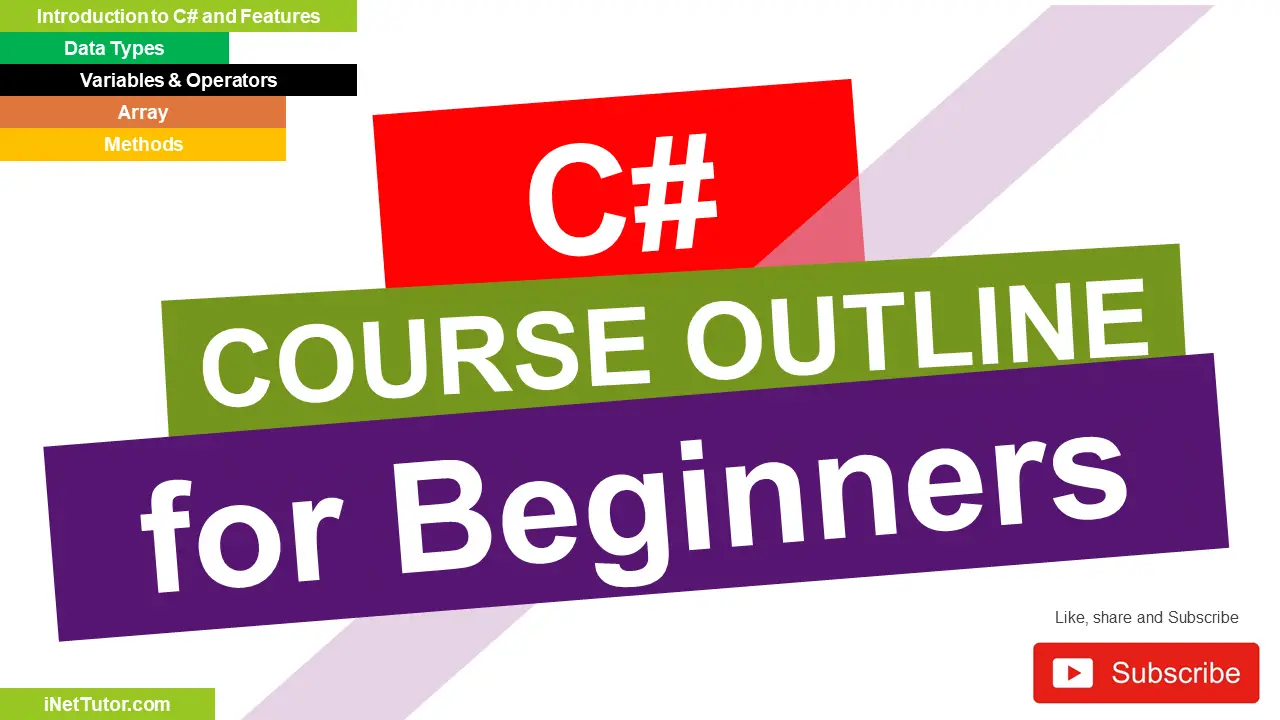
Summary
C# is a versatile language that can be used for a wide range of applications. In this course, we have covered the basics of the language and its syntax. We have also looked at how to use C# to create simple applications. Each and every topic provides lecture materials and source code for the students reference. If you would like to learn more about C#, visit the C# section of this website and as well the video contents on our YouTube channel. Happy coding everyone!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.