C# IF ELSE Statement Video Tutorial and Source code
Introduction
Table of Contents
This lesson is all about the decision control structures in C#, specifically the IF ELSE statement. The lesson includes sample programs to better understand how if statement works in C#.
Many decision-making statements are provided in C# to aid the flow of a C# program based on certain logical circumstances. To regulate the flow based on the circumstances, you’ll learn how to use if, else, and nested if else statements.
Objectives
By the end of this lesson, students should be able to:
- demonstrate essential understanding on the if else statement
- create a program that implements the concept of if else statement
- solve problems using the concept of if else statement in C#.
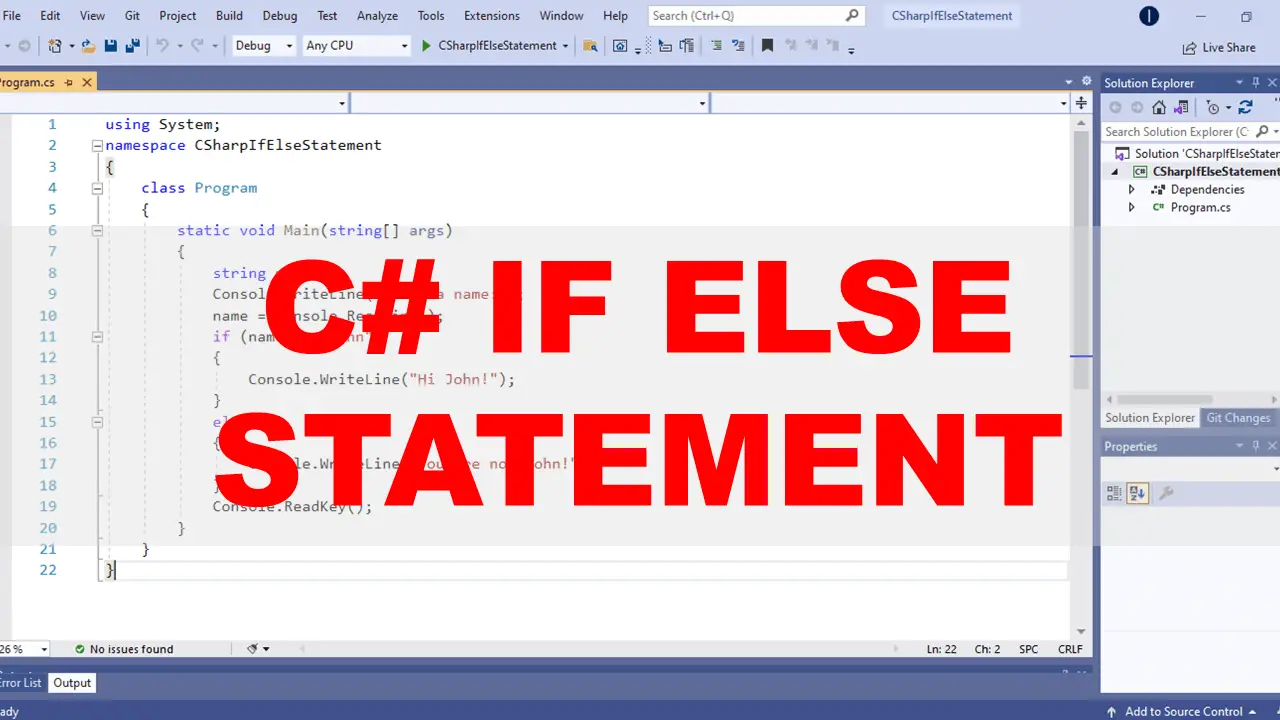
Requirements
- Visual Studio IDE
The Visual Studio IDE (Integrated Development Environment) is a software program that lets you make software, websites, and online apps. It includes a code editor, a debugger, a project system, and a number of tools to aid software development.
Lesson Proper
If one of the conditions stated in the if/else statement is true, the code in the if/else statement is executed. If the condition is not met, another block of code might be executed to complete the task. In C#, the if/else statement is part of the “Conditional” Statements, which are used to conduct different actions depending on the conditions that are encountered.
Here is the example source code of if else statement in C#. We will explain source code line by line for you to better understand how if statement works and function.
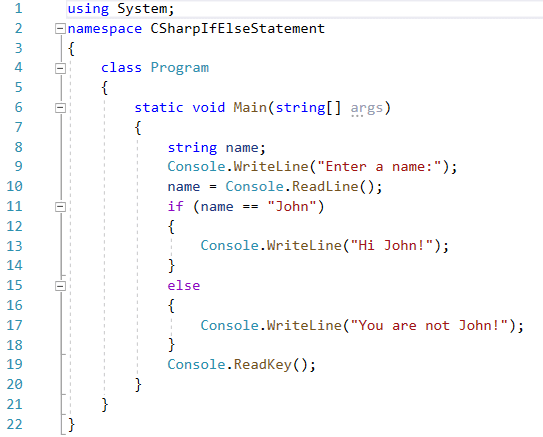
Line 1 – In code-behind and class files, we usually utilize the using keyword to add namespaces. Then, in the current page, it makes all of the classes, interfaces, and abstract classes, as well as their methods and properties, available. Console is a method or member function inside the namespace of System. We need to add it on the first line of our program since we need the console method to display and read user inputs.
Line 2 – the name of the program in CSharpIfElseStatement.
Line 6 – the entry point of C# program is the main method.
Line 8 – variable declaration. We have declared a variable name with a string type.
Line 9 – the purpose of this line is to display the text Enter a name: in the console.
Line 10 – Console.ReadLine gets user input and place that value in the name variable that we have declared earlier.
Line 11-18 – this is the scope of the if else statement, it will start at line 11 and ends at line 18.
Line 11 – this is the start of if else statement. It is where the conditional statement is being evaluated.
name == “John” – conditional statement
Line 13 – the console will display the text Hi John! If the text entered by the user is John. It means that the value of our variable is John which matches the criteria or conditional statement as presented in line 11.
Line 17 – this is the statement that will be executed if the conditional statement returns false. It simply mean that the user input or the value of the name variable is not equal to John. The console will display You are not John! since the value of the variable is not John.
Line 19 – the purpose of this line is to prevent the console from closing unless the user will press a key in the keyboard.
using System; namespace CSharpIfElseStatement { class Program { static void Main(string[] args) { string name; Console.WriteLine("Enter a name:"); name = Console.ReadLine(); if (name == "John") { Console.WriteLine("Hi John!"); } else { Console.WriteLine("You are not John!"); } Console.ReadKey(); } } }
Video Tutorial
Summary
The if else statement in C# is used to conditionally execute code. The code block within the if else statement will only execute if the condition is true. If the condition is false, the code block within the else statement will execute.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
For the step by step tutorial on how to create the if else statement console application in C#, please watch the video uploaded on our YouTube Channel.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
C# IF Statement Video Tutorial and Source code
POS System Using C#.Net Winforms and MS Access Free Source code
Select data in DataGridView Rows and Show in TextBox Using C# MySQL Database