Character Type Checker in C#
Introduction
Table of Contents
In this lesson, we will explore how to prompt the user to enter a character in C#. By utilizing conditional statements and logical operations, we will determine and print whether the entered character is a letter, digit, or special character. This exercise will enhance your understanding of character types and how to handle different scenarios in your C# programs. Get ready to dive into the world of character classification and expand your coding skills!
Objectives
In this lesson, our primary focus will be on understanding, learning, practicing, and applying the concept of character types in C#. We will delve into how characters can be classified as letters, digits, or special characters, and explore how to prompt the user to enter a character. Through hands-on practice, we will master the use of conditional statements and logical operations to determine the type of character entered. By the end of this lesson, you will be equipped with the skills to effectively classify and print the type of character entered in your C# programs.
Specifically, by the end of this lesson, the students should be able to:
Understand:
- Grasp the concept of character classification and its significance in C# programming.
- Understand the role of if-else statements in making decisions based on user inputs.
Learn:
- Acquire knowledge of C# methods for character classification, distinguishing between letters, digits, and special characters.
- Learn the syntax and usage of if-else statements for effective decision-making in character type identification.
Practice:
- Engage in hands-on exercises to reinforce comprehension of character classification methods.
- Practice implementing if-else statements to create a robust character type identification program.
Apply:
- Apply the acquired knowledge to develop a functional Character Type Identification program in C#.
- Implement error-handling mechanisms to ensure accurate classification even in unexpected scenarios.
Source code example
using System; namespace CharacterTypeIdentification { class Program { static void Main() { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Character Type Identification"); // Prompt user to enter a character Console.Write("Enter a character: "); char inputChar = Console.ReadKey().KeyChar; Console.WriteLine(); // Move to the next line // Determine and display the type of the entered character if (char.IsLetter(inputChar)) { Console.WriteLine("The entered character is a letter."); } else if (char.IsDigit(inputChar)) { Console.WriteLine("The entered character is a digit."); } else { Console.WriteLine("The entered character is a special character."); } Console.ReadKey(); } } }
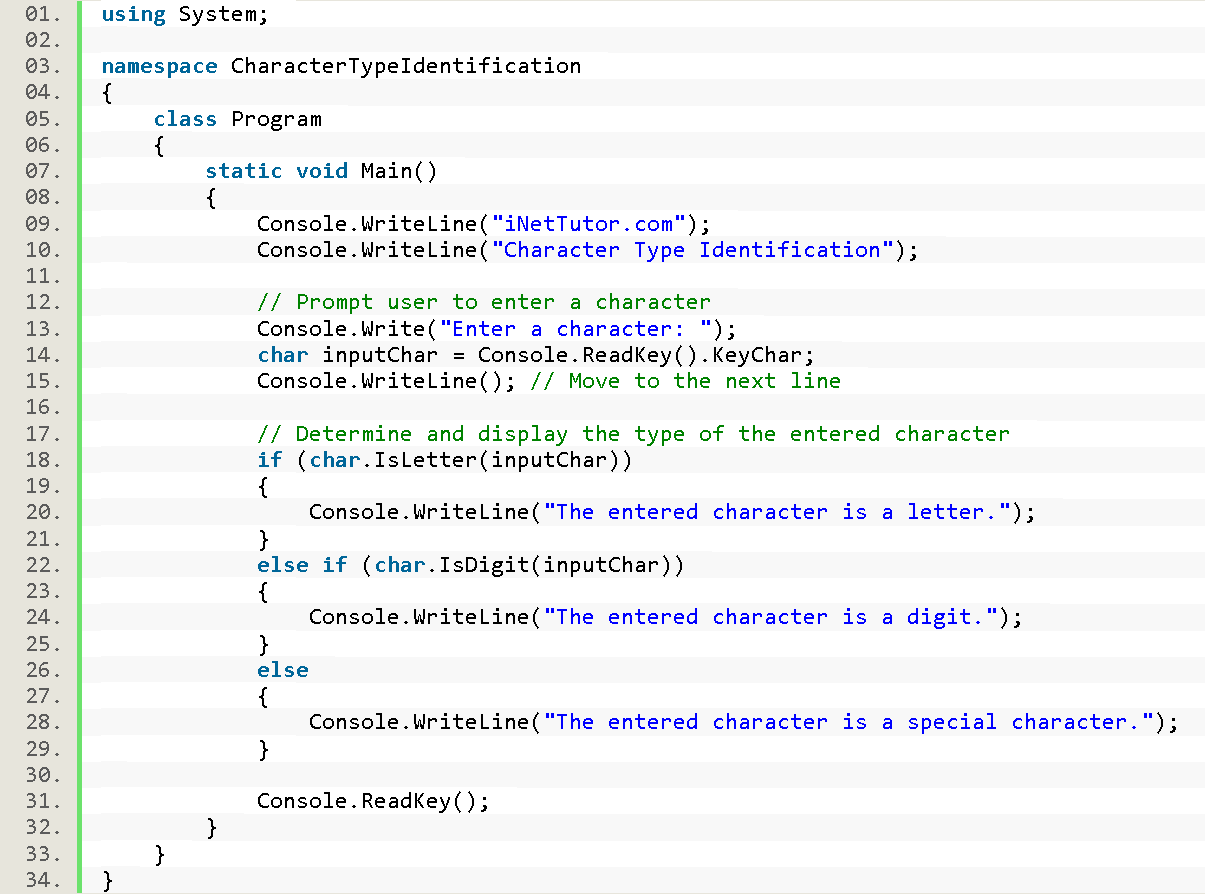
Explanation
Here’s a breakdown of the C# source code:
- Namespaces and Classes:
- using System;: Imports the System namespace, which provides essential tools like Console for input/output and char for character manipulation.
- namespace CharacterTypeIdentification: Creates a namespace to organize the code.
- class Program: Defines the main class containing the program’s logic.
- Entry Point:
- static void Main(): This is the starting point of the program’s execution.
- Introduction:
- Console.WriteLine(“iNetTutor.com”);: Prints a welcome message.
- Console.WriteLine(“Character Type Identification”);: Displays the program’s purpose.
- User Input:
- Console.Write(“Enter a character: “);: Prompts the user to enter a character.
- char inputChar = Console.ReadKey().KeyChar;: Reads the input character and stores it in the inputChar variable.
- Console.WriteLine();: Moves the cursor to the next line for a cleaner output.
- Character Classification:
- if (char.IsLetter(inputChar)): Checks if the character is a letter using the char.IsLetter method.
- Console.WriteLine(“The entered character is a letter.”);: Prints a message if it’s a letter.
- else if (char.IsDigit(inputChar)): Checks if the character is a digit using char.IsDigit.
- Console.WriteLine(“The entered character is a digit.”);: Prints a message if it’s a digit.
- else: Handles any character that’s not a letter or digit, assuming it’s a special character.
- Console.WriteLine(“The entered character is a special character.”);: Prints a message for special characters.
- Pause:
- Console.ReadKey();: Waits for the user to press a key before closing the console window.
Output
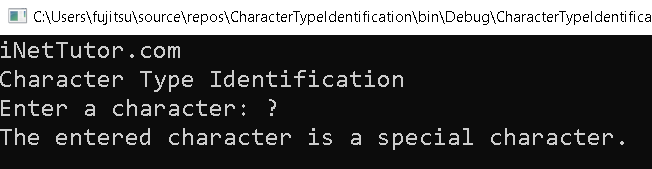
Summary
In this lesson, we focused on character type identification in C#. We learned how to prompt the user to enter a character and determine its classification as a letter, digit, or special character. Through the use of conditional statements and the char.IsLetter and char.IsDigit methods, we were able to effectively classify and display the type of character entered.
Key concepts covered in this lesson include:
- Prompting the user for input using Console.WriteLine and Console.ReadKey
- Checking character type using conditional statements (if, else if, else)
- Using the char.IsLetter and char.IsDigit methods to determine the type of character
- Printing the classification of the entered character using Console.WriteLine
By practicing and applying these concepts, you have gained the necessary skills to identify and classify characters in your C# programs. Understanding character types is crucial for handling user input and performing specific actions based on the type of character encountered. Keep exploring and applying these concepts to enhance your C# programming skills further.
Exercises and Assessment
These exercises and the lab exam will provide students with opportunities to enhance their understanding of character type identification in C# and improve their coding skills. They encourage students to think creatively, apply their knowledge, and explore different aspects of the topic.
Exercises:
- Level Up Your Classifier:
- Expand the categories: Go beyond letters, digits, and special symbols. Explore Unicode categories to identify punctuation marks, whitespace, control characters, etc. (Use char.IsPunctuation, char.IsWhiteSpace, etc.).
- Case sensitivity: Introduce checks for uppercase and lowercase letters (char.IsUpper and char.IsLower).
- Numerical analysis: For digits, explore extracting numerical values using char.Parse or converting them to different bases.
- Visualize the results: Add functionality to display the character using Console.Write(inputChar).
- Interactive Challenges:
- Guess the character: Develop a game where the program randomly chooses a character and the user has to guess its type within a limited number of tries.
- Character counting: Build a program that takes a string as input and counts the occurrences of different character types (letters, digits, special symbols).
- Password validation: Create a program that checks if a user-entered password meets specific criteria like minimum length, case sensitivity, or special character inclusion.
- Advanced Exploration:
- Unicode deep dive: Investigate different Unicode categories and properties using tools like char.GetUnicodeCategory and char.GetNumericValue.
- Regular expressions: Implement regular expressions to match patterns of specific character types or sequences.
- Character encoding and decoding: Explore encoding schemes like Base64 or ASCII and build functions to convert between them.
Lab Exam:
- Scenario: Your friend is building a program to analyze text data. They need a reliable function to classify each character in a string as a letter, digit, or special symbol.
- Task: Develop a robust and efficient C# function that takes a character as input and returns its type (letter, digit, or special symbol). Consider edge cases like Unicode characters and whitespace.
- Bonus: Extend the function to further categorize special symbols (punctuation, whitespace, etc.) and handle case sensitivity for letters.
Related Topics and Articles:
C# IF ELSE Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.