Time of Day Greeting in C#
Introduction
Table of Contents
In this lesson, we will learn how to create a C# program that greets the user based on the current time of day. The program will use an if-else statement to determine whether it is morning, afternoon, or evening, and then print the appropriate greeting. If it is morning (before 12 PM), the program will print “Good morning.” If it is afternoon (12 PM to 5 PM), it will print “Good afternoon.” Otherwise, it will print “Good evening.” This program will help us understand how to use conditional statements in C# to perform different actions based on the current time of day.
Now let’s dive into the details of implementing this program.
Determining the Time of Day
To determine the time of day in our program, we need to consider the current time and compare it to specific time ranges. The following search results provide information on how different greetings are used based on the time of day:
- According to , “Good afternoon” is used from 12 PM to 4 PM when meeting someone for the first time.
- states that “Good evening” can be used any time between 5 PM and 9 PM as a greeting.
- suggests that “Good morning” is used from 5 AM to 12 PM.
Based on this information, we can define the time ranges for each greeting in our program as follows:
- Morning: 5 AM to 12 PM
- Afternoon: 12 PM to 4 PM
- Evening: 5 PM to 9 PM
Objectives
The objectives of this lesson are to provide a comprehensive understanding of the C# program that greets the user based on the current time of day, and to guide learners in the process of learning, practicing, and applying the concepts covered in the lesson. By the end of this lesson, learners will have a clear understanding of how to use conditional statements in C# to perform different actions based on the current time of day. They will learn the necessary coding techniques and best practices to implement this program effectively. Through practice and application, learners will gain hands-on experience in writing C# code and develop the skills required to create similar programs in the future.
Objective 1: Understand The first objective of this lesson is to ensure a thorough understanding of the concept behind the C# program. Learners will learn about conditional statements and how they can be used to execute different blocks of code based on specific conditions. They will understand the logic behind determining the current time of day and associating it with the appropriate greeting. By understanding the underlying principles, learners will be able to apply these concepts to other programming scenarios.
Objective 2: Learn The second objective is to facilitate the learning process by providing step-by-step guidance on how to implement the C# program. Learners will learn about the necessary syntax and techniques required to write the code. They will learn how to retrieve the current time and extract the hour component using built-in functions. Furthermore, learners will learn how to structure the if-else statements to check the current time against predefined time ranges. Through this learning process, learners will gain a solid foundation in C# programming and improve their problem-solving skills.
Objective 3: Practice The third objective is to provide ample opportunities for learners to practice the concepts and techniques covered in the lesson. Practice exercises will be provided to reinforce the understanding of conditional statements and time-related calculations. By engaging in hands-on practice, learners will solidify their knowledge and develop proficiency in writing code. They will become more comfortable with implementing conditional logic and improve their ability to produce accurate and efficient code solutions.
Objective 4: Apply The final objective is to encourage learners to apply the knowledge and skills gained from this lesson to real-world scenarios. Learners will be encouraged to modify the program and experiment with different conditions and greetings. This will enable them to adapt the program to their specific needs and explore the possibilities of using conditional statements in other contexts. By applying their learning in practical situations, learners will strengthen their problem-solving abilities and become more confident in utilizing conditional statements in their future programming endeavors.
In summary, the objectives of this lesson are to help learners understand the C# program that greets the user based on the current time of day, learn the necessary concepts and techniques, practice implementing the program, and apply their knowledge to real-world scenarios. Through achieving these objectives, learners will develop a strong foundation in C# programming and gain valuable experience in using conditional statements effectively.
Source code example
using System; namespace TimeOfDayGreeting { class Program { static void Main() { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Time of Day Greeting"); // Get the current time DateTime currentTime = DateTime.Now; // Determine the period of the day and display appropriate greeting if (currentTime.Hour < 12) { Console.WriteLine("Good morning."); } else if (currentTime.Hour >= 12 && currentTime.Hour < 17) { Console.WriteLine("Good afternoon."); } else { Console.WriteLine("Good evening."); } Console.ReadKey(); } } }
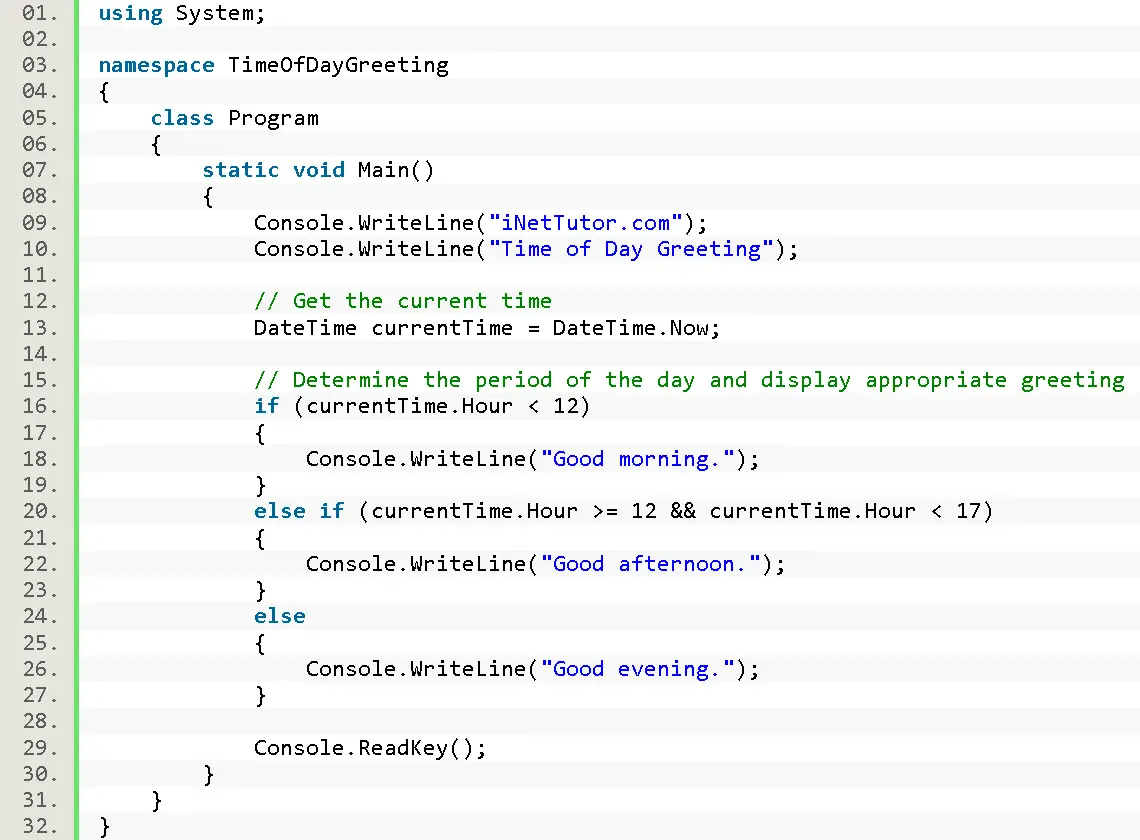
Explanation
By running this program, the user will be greeted with an appropriate message based on the current time of day.
Please note that the provided code does not include error handling or input validation. It assumes that the system time is accurate and that the user will run the program without any issues.
This is how the code works:
- The code begins by importing the System namespace using the using statement. This allows us to access the Console class and other types defined in the System namespace without fully qualifying their names.
- The program is defined within the TimeOfDayGreeting namespace.
- Inside the Program class, the Main method serves as the entry point for the program.
- The program starts by printing the text “iNetTutor.com” and “Time of Day Greeting” to the console using the Console.WriteLine method.
- The code retrieves the current date and time using the DateTime.Now property and assigns it to the currentTime variable.
- The program uses an if-else statement to determine the period of the day based on the hour component of the currentTime variable.
- If the hour is less than 12, the program prints “Good morning.”
- If the hour is between 12 (inclusive) and 17 (exclusive), the program prints “Good afternoon.”
- Otherwise, if the hour is 17 or greater, the program prints “Good evening.”
- Finally, the program waits for the user to press a key before exiting. This is achieved using the Console.ReadKey method.
Output
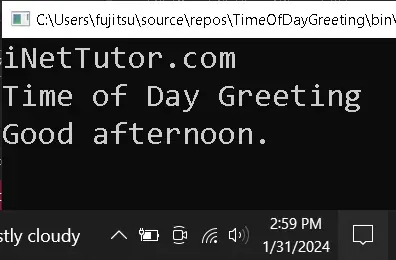
Summary
In this topic, we learned how to create a C# program that greets the user based on the current time of day. Using conditional statements, we determined the period of the day (morning, afternoon, or evening) and displayed the appropriate greeting. By understanding the concept of conditional statements, learning the necessary syntax and techniques, practicing the implementation, and applying the knowledge to real-world scenarios, we gained a solid understanding of using conditional statements in C# to perform different actions based on specific conditions.
Exercises and Assessment
To enhance and improve the source code, you can consider the following exercises and lab exam:
- Exercise 1: Add More Greetings: Modify the program to include additional greetings for specific time ranges, such as “Good night” for late evening or “Good day” for midday. Test the program with different time inputs to ensure the greetings are displayed correctly.
- Exercise 2: User Input: Modify the program to allow the user to input their name. Use the input to personalize the greeting, such as “Good morning, [Name]”. Ensure the program handles different input formats and provides appropriate error handling.
- Exercise 3: Multilingual Greetings: Extend the program to support multiple languages. Create a mechanism to detect the user’s preferred language and display the greeting accordingly. You can use language-specific resource files or implement a language detection algorithm.
- Lab Exam: Time Zone Conversion: Design a lab exam that tests the ability to convert the current time to different time zones. Provide a list of time zones and ask the students to modify the program to display the appropriate greeting based on the converted time. This will test their understanding of time zone calculations and conditional statements.
By incorporating these exercises and lab exam, learners can further enhance their coding skills, practice working with user input, handle different scenarios, and expand the functionality of the program. These activities will provide hands-on experience and reinforce the concepts covered in the initial source code.
Related Topics and Articles:
C# IF ELSE Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.