String Manipulation in CSharp
String manipulation is a fundamental aspect of programming, empowering developers to transform and analyze textual data efficiently. In this tutorial, we delve into the intricacies of string manipulation using C#, exploring a practical example that demonstrates essential operations. From converting text to uppercase and lowercase to reversing strings and counting character occurrences, this tutorial serves as a guide to mastering fundamental string manipulation techniques. Join us as we unravel the power of strings in programming through hands-on exploration and application in the String Manipulation Example. Let’s dive into the world of C# string manipulation and unlock new possibilities for handling and transforming textual data.
Introduction
Table of Contents
In this lesson on string manipulation in C#, we will explore different examples and techniques for manipulating strings in the C# programming language. We will cover topics such as declaring and initializing strings, string escape sequences, string operations, and common string manipulation methods in C#.
The purpose of the String Manipulation Example in C# is to demonstrate how to manipulate strings using various techniques and methods available in the C# programming language. The example will cover different aspects of string manipulation, including creating strings, performing string operations, and using string manipulation methods.
By studying this example, learners will gain a better understanding of how to work with strings in C# and develop the skills necessary to manipulate strings effectively in their own programs.
Components of the String Manipulation Example
The String Manipulation Example in C# consists of the following components:
- Declaring and Initializing Strings: The example will show how to declare and initialize strings in C# using the string keyword and double quotes. It will demonstrate how to create strings with specific text content.
- String Operations: The example will cover various string operations, such as concatenation (combining multiple strings into one), accessing individual characters in a string, and finding the length of a string.
- String Manipulation Methods: The example will introduce learners to some of the commonly used string manipulation methods available in C#. These methods include Substring (extracting a portion of a string), ToUpper and ToLower (changing the case of characters in a string), Replace (replacing specific characters or substrings in a string), and Split (splitting a string into an array of substrings based on a delimiter).
Through these components, the String Manipulation Example will provide learners with hands-on experience and practical knowledge of manipulating strings in C#. It will equip them with the skills necessary to solve string manipulation problems and efficiently work with strings in their own C# programs.
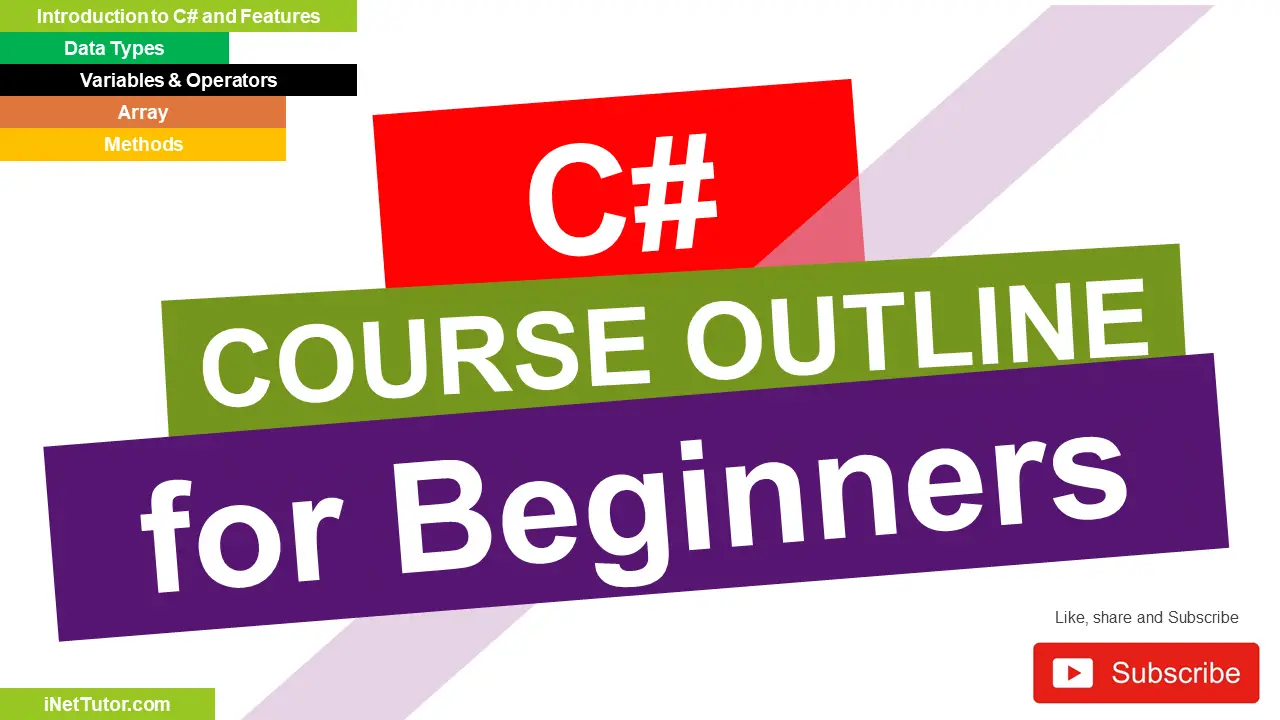
Objectives
In this tutorial, our primary objectives revolve around providing a comprehensive understanding of string manipulation in C#. First and foremost, we aim to ensure that learners comprehend the foundational concepts underlying string manipulation and recognize its importance in programming. Following this, participants will delve into the practical aspects, learning essential techniques such as converting strings to uppercase and lowercase, as well as reversing strings. The emphasis is not only on theoretical understanding but on practical skill development through hands-on practice sessions. Ultimately, the goal is to empower learners to apply their string manipulation expertise to real-world scenarios, demonstrating the versatility and significance of these techniques in solving programming challenges. Let’s embark on a journey to master C# string manipulation and unleash its potential in the realm of programming.
- Understand String Manipulation Concepts:
- Grasp the foundational concepts of string manipulation in C#.
- Explore the significance of string operations in programming.
- Learn Essential String Manipulation Techniques:
- Learn key techniques such as converting strings to uppercase and lowercase.
- Gain proficiency in reversing strings for diverse applications.
- Practice String Manipulation Skills:
- Engage in hands-on practice sessions to reinforce learning.
- Implement string manipulation methods in a controlled environment.
- Apply String Manipulation in Real-world Scenarios:
- Apply acquired knowledge to solve practical problems.
- Explore the real-world relevance of string manipulation in C# programming.
Source code example
using System; namespace StringManipulation { class Program { static void Main() { Console.WriteLine("iNetTutor.com"); Console.WriteLine("String Manipulation Example"); string text = "Hello, World!"; // Convert the string to uppercase string uppercaseText = ConvertToUppercase(text); Console.WriteLine("Uppercase: " + uppercaseText); // Convert the string to lowercase string lowercaseText = ConvertToLowercase(text); Console.WriteLine("Lowercase: " + lowercaseText); // Reverse the string string reversedText = ReverseString(text); Console.WriteLine("Reversed: " + reversedText); // Count the number of occurrences of a specific character char characterToCount = 'o'; int count = CountOccurrences(text, characterToCount); Console.WriteLine("Number of occurrences of '" + characterToCount + "': " + count); Console.ReadKey(); } static string ConvertToUppercase(string text) { return text.ToUpper(); } static string ConvertToLowercase(string text) { return text.ToLower(); } static string ReverseString(string text) { char[] charArray = text.ToCharArray(); Array.Reverse(charArray); return new string(charArray); } static int CountOccurrences(string text, char character) { int count = 0; foreach (char c in text) { if (c == character) { count++; } } return count; } } }
Explanation
Here’s a breakdown of the C# source code example:
Namespaces and Classes:
- using System;: This line imports the System namespace, which contains fundamental classes and methods used in C#, including the Console class for writing to the console.
- namespace StringManipulation: This creates a namespace called StringManipulation to organize the code within it.
- class Program: This defines a class named Program, which acts as the entry point for the application.
Main Method:
- static void Main(): This is the main method, where program execution begins.
- Console.WriteLine(…): These lines print text to the console, including a header and the initial string “Hello, World!”.
String Manipulation Functions:
- ConvertToUppercase(text): This function converts a string to uppercase using the ToUpper() method and returns the uppercase version.
- ConvertToLowercase(text): This function converts a string to lowercase using the ToLower() method and returns the lowercase version.
- ReverseString(text): This function reverses a string by:
- Converting it to a character array using ToCharArray().
- Reversing the character array using Array.Reverse().
- Converting the reversed character array back to a string and returning it.
- CountOccurrences(text, character): This function counts the occurrences of a specific character in a string by:
- Using a foreach loop to iterate through each character in the string.
- Checking if the current character matches the target character.
- Incrementing a counter variable for each match.
- Returning the final count.
Program Flow:
- The program prints a header and the initial string.
- It calls the ConvertToUppercase and ConvertToLowercase functions to demonstrate case conversion and prints the results.
- It calls the ReverseString function to reverse the string and prints the reversed string.
- It calls the CountOccurrences function to count the occurrences of the character ‘o’ and prints the count.
- The program waits for a key press using Console.ReadKey() before exiting.
Key Points:
- The code demonstrates basic string manipulation techniques in C#, including case conversion, reversing, and character counting.
- It uses functions to modularize the code and make it more reusable.
- It utilizes built-in string methods (ToUpper(), ToLower()) and array methods (ToCharArray(), Array.Reverse()) for efficient string manipulation.
Output
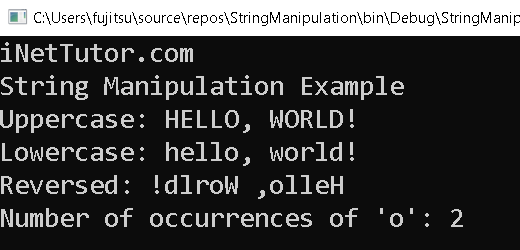
Summary
In the world of programming, string manipulation is a fundamental skill that allows developers to modify and manipulate text data to suit their needs. Whether you’re a beginner or an experienced programmer, understanding string manipulation techniques is essential for creating robust and efficient applications.
In this blog post, we dive into the fascinating realm of string manipulation in C#, providing a comprehensive guide to help you master this crucial skill. We start with a brief overview of string manipulation in programming, highlighting its significance in various domains such as web development, data analysis, and game development.
The post then introduces a practical example of string manipulation in C#, taking you through the purpose and components of the example program. Exploring the provided code snippet, we explain how to convert strings to uppercase and lowercase, reverse a string, and count the occurrences of a specific character within a string.
Throughout the blog post, we not only explain the concepts and techniques behind each string manipulation operation but also provide clear and concise code explanations. You’ll gain insight into the inner workings of the code, empowering you to apply these techniques in your own projects.
By the end of this guide, you’ll have a solid understanding of string manipulation in C#, enabling you to solve complex problems, process data efficiently, and enhance the functionality of your applications. Whether you’re a developer looking to expand your skills or a beginner eager to learn, this blog post is your roadmap to mastering string manipulation in C#.
Exercises and Assessment
This exercise aims to elevate the String Manipulation Example by encouraging participants to go beyond the initial implementation, fostering a deeper understanding of C# string manipulation and programming best practices.
- User Input Extension:
- Modify the program to accept user input for the initial string instead of a hardcoded value.
- Ensure the program gracefully handles different input scenarios.
- Multiple Character Count:
- Extend the CountOccurrences method to allow counting occurrences of multiple characters.
- Implement a mechanism to display the counts for each specified character.
- Error Handling Implementation:
- Integrate robust error handling mechanisms to manage unexpected inputs or errors during execution.
- Provide meaningful error messages for better user interaction.
- Efficiency Optimization:
- Analyze the current implementation and identify opportunities for optimizing the code for performance.
- Consider alternatives or additional methods to achieve the same results more efficiently.
- Dynamic Method Invocation:
- Implement a mechanism to dynamically invoke string manipulation methods based on user selection.
- Allow users to choose which manipulation operation to perform on the input string.
Benefits:
- Enhance user interaction by enabling dynamic input and operation choices.
- Improve code robustness through effective error handling.
- Optimize performance for a more efficient execution.
- Encourage exploration and experimentation with additional string manipulation features.
Related Topics and Articles:
Methods in C# Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.