Finding the Maximum Element in an Array
Introduction
Table of Contents
In programming, an array is a data structure that allows us to store a collection of elements of the same type. Arrays provide a convenient way to store and manipulate a group of related values in a single variable. By using arrays, we can efficiently organize and access data in a systematic manner.
Arrays support a wide range of operations that enable us to work with the data they contain. Some common array operations include:
- Accessing elements: We can access individual elements in an array by their index, which represents their position in the array.
- Modifying elements: We can modify the value of any element in an array by assigning a new value to its corresponding index.
- Adding elements: Depending on the programming language, we can add elements to an array dynamically or by resizing the array.
- Removing elements: Similarly, we can remove elements from an array dynamically or by resizing the array.
- Searching elements: We can search for specific elements within an array to determine their presence or obtain their index.
- Sorting elements: We can sort the elements of an array in ascending or descending order based on their values.
Finding the maximum element in an array is a fundamental operation that plays a crucial role in many programming tasks. The maximum element represents the largest value within the array, and its identification can provide valuable insights and facilitate decision-making. For example, in a financial application, finding the maximum element can help identify the highest transaction amount. In a game, it can determine the highest score achieved. Additionally, finding the maximum element is often a prerequisite for other operations, such as determining the range or calculating statistics like the average or sum of the elements. By understanding how to find the maximum element in an array, programmers can unlock powerful capabilities to process and analyze data efficiently.
In the following sections, we will explore different techniques and approaches to find the maximum element in an array, focusing on their implementation in the C# programming language. By mastering this operation, you will expand your array manipulation skills and gain the ability to work with arrays in a more comprehensive and effective manner.
Objectives
Arrays are a fundamental concept in programming, allowing us to store and manipulate collections of related data. They provide a convenient way to organize and access data in a systematic manner. In addition to basic operations like accessing and modifying elements, arrays support a variety of other operations that enable us to work with the data they contain. These operations include adding and removing elements, searching for specific values, sorting elements, and more. One important operation when working with arrays is finding the maximum element. This operation involves identifying the largest value within an array, which can be crucial in various programming tasks. In this lesson, we will explore the importance of finding the maximum element in an array and learn different techniques to accomplish this in the C# programming language. By understanding and mastering this operation, you will enhance your ability to work with arrays effectively and efficiently. Let’s dive in and explore the objectives of this lesson.
Objectives:
- Understand the significance of finding the maximum element in an array and its relevance in programming tasks.
- Learn different techniques and approaches to find the maximum element in an array using C#.
- Practice implementing the code to find the maximum element in an array using various techniques.
- Apply the knowledge gained to solve real-world programming problems that involve finding the maximum element in an array.
By the end of this lesson, you will have a solid understanding of how to find the maximum element in an array using different techniques in C#. You will be able to choose the most appropriate approach based on the requirements of your programming tasks. Let’s get started and explore the world of array operations and finding the maximum element!
Source code example
using System; namespace FindingMaximumElement { class Program { static void Main() { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Finding the Maximum Element in an Array"); // Declare and initialize an array of integers int[] numbers = { 10, 5, 8, 3, 15, 20 }; // Display the list of numbers in the array Console.WriteLine("Numbers in the array: " + string.Join(", ", numbers)); // Initialize the maximum element to the first element in the array int max = numbers[0]; // Iterate through the array to find the maximum element for (int i = 1; i < numbers.Length; i++) { if (numbers[i] > max) { max = numbers[i]; } } // Display the maximum element Console.WriteLine("The maximum element in the array is: " + max); Console.ReadKey(); } } }
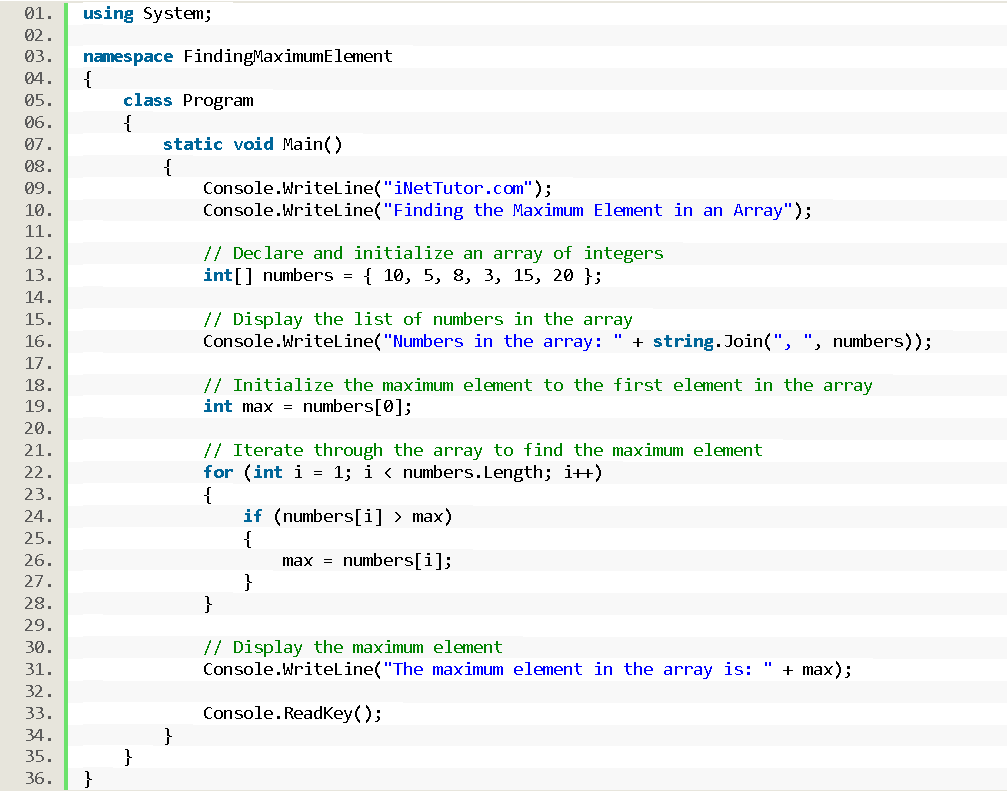
Explanation
In this program, we have an array of integers called numbers, which contains some sample values. Next is the line of code that list of numbers in the array using string.Join and Console.WriteLine. This will output the list of numbers separated by commas. The program initializes the maximum element (max) to the first element in the array.
It then iterates through the remaining elements of the array using a for loop. For each element, it checks if it is greater than the current maximum (max). If it is, the maximum value is updated to the new element.
After iterating through all the elements, the program displays the maximum element found.
You can modify the numbers array or add more elements to test the program with different values.
Output
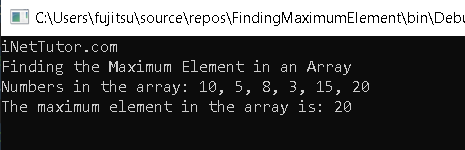
Summary
In this lesson, we explored the concept of finding the maximum element in an array using different techniques in the C# programming language. We covered the importance of this operation and its relevance in various programming tasks. Let’s summarize the key points covered:
- We discussed the significance of arrays in programming and how they provide a convenient way to store and manipulate collections of related data.
- We introduced common array operations such as accessing, modifying, adding, and removing elements, as well as searching and sorting elements.
- We emphasized the importance of finding the maximum element in an array and how it can provide valuable insights and facilitate decision-making in various programming tasks.
- We provided a sample C# source code that demonstrated these techniques and showed how to find the maximum element in an array.
Importance of Understanding Different Techniques
Understanding different techniques to find the maximum element in an array is essential for programmers. It expands their problem-solving capabilities and enables them to choose the most appropriate approach based on the requirements of their programming tasks. By mastering these techniques, programmers can efficiently process and analyze data, make informed decisions, and build robust applications.
Further Exploration and Practice
To solidify the concepts learned in this lesson, we encourage you to further explore and practice finding the maximum element in an array using different techniques. Experiment with different array sizes, data types, and scenarios. Consider implementing additional techniques or optimizing existing ones. This hands-on practice will enhance your understanding and proficiency in array operations and their applications.
Remember to consult relevant documentation and resources, as well as engage in coding exercises and challenges to reinforce your skills. By continuously exploring and practicing, you will strengthen your ability to work with arrays effectively and become a more proficient programmer.
Keep exploring the world of array operations and embrace the opportunities they offer in solving programming problems. Happy coding!
Exercises and Assessment
To enhance your understanding and practice of finding the maximum element in an array, here are some exercises and activities you can try:
- Implement Additional Techniques: Expand your knowledge by exploring and implementing additional techniques to find the maximum element in an array. Research and experiment with different algorithms, such as binary search or divide and conquer, to optimize the search process.
- Modify Array Size and Values: Create arrays with different sizes and values to test the performance of your code. Try arrays with thousands or millions of elements to see how different techniques handle larger datasets.
- Create Custom Functions: Write custom functions that perform specific operations on arrays, such as finding the minimum element, calculating the average, or sorting the array in descending order. This will deepen your understanding of array manipulation and improve your problem-solving skills.
- Combine Array Operations: Combine different array operations, such as finding the maximum element and sorting the array, into a single program. This will help you practice integrating multiple concepts and reinforce your understanding of array operations.
- Explore Real-World Applications: Research and explore real-world applications where finding the maximum element in an array is crucial. For example, you can apply this knowledge to analyze financial data, optimize resource allocation, or solve optimization problems in various domains.
- Participate in Coding Challenges: Engage in coding challenges or competitions that involve array operations. Websites like LeetCode, HackerRank, or CodeSignal offer a wide range of coding challenges that can help you improve your problem-solving skills and gain exposure to different scenarios.
- Collaborate and Discuss: Join online coding communities or forums to collaborate with other programmers and discuss different approaches to finding the maximum element in an array. Sharing ideas and learning from others can broaden your perspective and introduce you to new techniques.
Remember, practice is key to mastering any programming concept. By actively engaging in exercises and activities, you will solidify your understanding, improve your coding skills, and become more proficient in working with arrays.
Related Topics and Articles:
Counting Even Numbers in an Array
Sum of Array Elements in CSharp
Array in C# Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.