Array in C#
Introduction
Table of Contents
This lesson is all about the array in C#. The lesson includes sample programs to better understand how array works in C#.
An array is a data structure that allows you to store a group of data items of the same data type in a single variable. The items in an array are indexed by integers, starting with 0. You can access a particular item in an array by giving its index. Arrays are an integral feature of most programming languages. In this lesson , you will learn how to create and use an array in C#. You will also learn how to access individual objects in an array, and how to manipulate arrays as a whole. Finally, you will see instances of how arrays are used in programming applications.
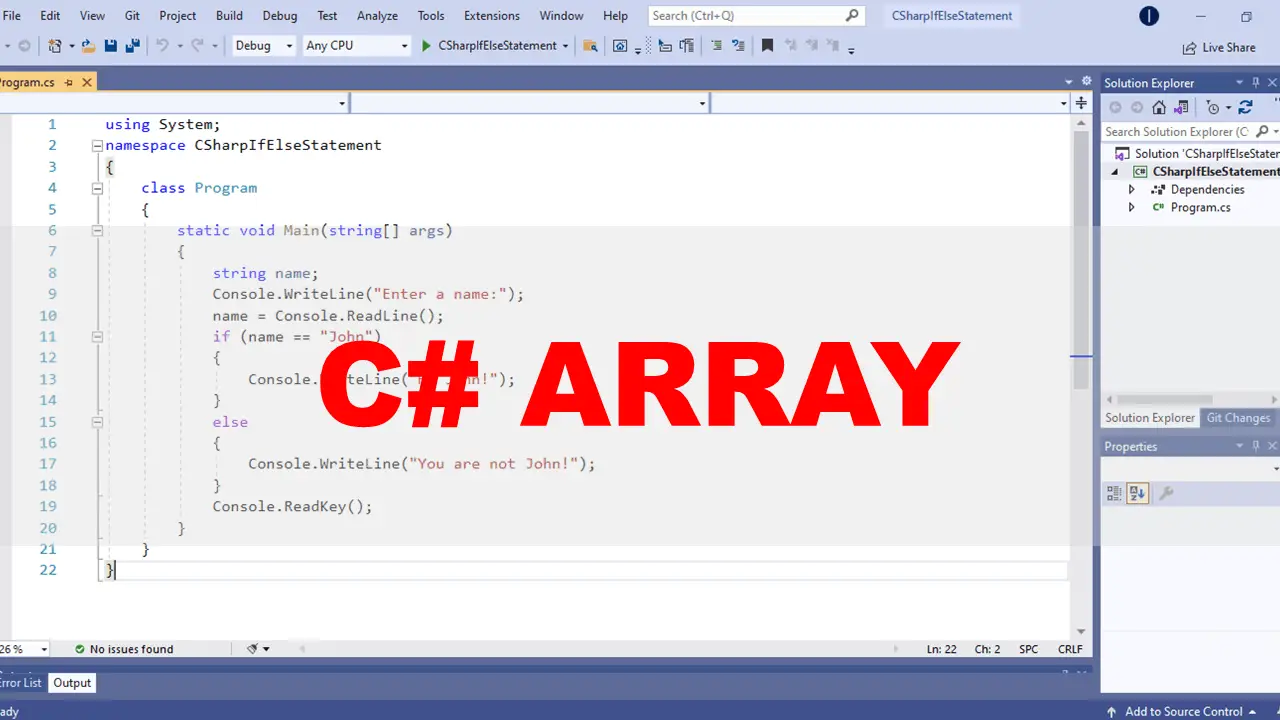
Objectives
By the end of this lesson, you should
- Research on how array works and give examples
- Explain how array works
- Design and Create a basic program that implements the use of array
Lesson Proper
Arrays are used to hold multiple values in a single variable, rather than creating distinct variables for each value as is done with regular variables.
An array is a collection of variables with the same type as the variables in the collection.
Instead of declaring individual variables such as number0, number1,…, and number99, you declare a single array variable such as numbers and use the values numbers[0], numbers[1], and…, numbers[99] to represent individual variables, as seen in the following example. The index of a specific element in an array is used to access that element.
The index begins with zero, which represents the value stored. In addition, an array can be used to store a fixed number of values. When the array size is not reached, the index of the array should be increased by one in a sequential manner.
How it works
Line 1 – To add namespaces and class files, we commonly use the using keyword. It then makes all of the classes, interfaces, and abstract classes, as well as their methods and properties, available on the current page. Console is a member function or method in the System namespace. Because we need the console function to show and read user inputs, we must include it on the first line of our program.
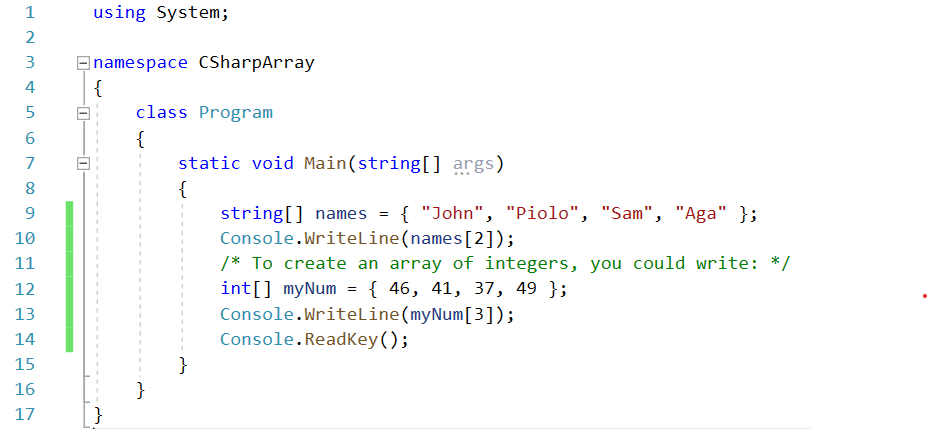
Line 3 – The line specifies the name of the program, which is CSharpArray in this case.
Line 7 – The main method is where a C# program starts.
Line 9 –
Create an Array
To declare an array, define the variable type with square brackets:
string[] names;
We have now declared a variable that holds an array of strings.
Assigning Values to an Array
To insert values to it, we can use an array literal – place the values in a comma-separated list, inside curly braces:
string[] names = { “John”, “Piolo”, “Sam”, “Aga” };
Line 10 –
Access the Elements of an Array
You access an array element by referring to the index number.
This statement accesses the value of the first element in names:
string[] names = { “John”, “Piolo”, “Sam”, “Aga” };
Console.WriteLine(names[0]);
Line 12 – this is an example of an array that stores integer values
Line 13 – this line will access the array with the index value of 0, and it will print the value 46.
Console.WriteLine(myNum[0]);
Line 14 – This line’s function is to keep the console from closing unless the user presses a key on the keyboard.
using System; namespace CSharpArray { class Program { static void Main(string[] args) { string[] names = { "John", "Piolo", "Sam", "Aga" }; names[1] = "Robin"; Console.WriteLine(names[1]); Console.WriteLine(names.Length); /* To create an array of integers, you could write: */ int[] myNum = { 46, 41, 37, 49 }; myNum[1] = 53; Console.WriteLine(myNum[1]); Console.WriteLine(myNum.Length); Console.ReadKey(); } } }
Change an Array Element
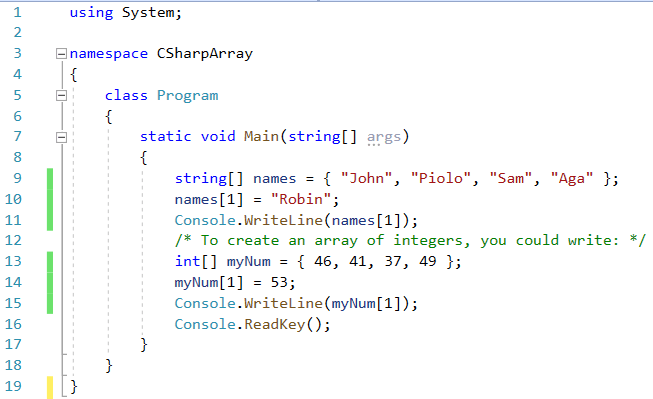
To change the value of a specific element, refer to the index number:
Line 10. it will change the value of Piolo into Robin
names[1] = “Robin“;
Line 14. it will change the value of 41 into 53
myNum[1] = 53;
Array Length
To find out how many elements an array has, use the Length property:
Console.WriteLine(names.Length);
Loop Through an Array
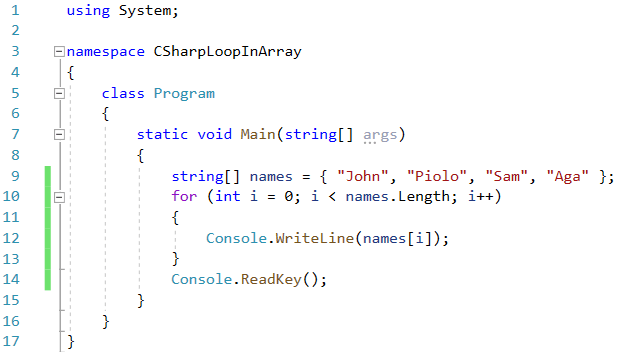
You can loop through the array elements with the for loop, and use the Length property to specify how many times the loop should run.
The following example outputs all elements in the names array:
for (int i = 0; i < names.Length; i++)
{
Console.WriteLine(names[i]);
}
using System; namespace CSharpLoopInArray { class Program { static void Main(string[] args) { string[] names = { "John", "Piolo", "Sam", "Aga" }; for (int i = 0; i < names.Length; i++) { Console.WriteLine(names[i]); } Console.ReadKey(); } } }
System.Linq Namespace
Other useful array methods, such as Min, Max, and Sum, can be found in the System.Linq namespace
Video Tutorial
Summary
Arrays are data structures that hold a fixed-size sequential collection of elements of the same type in a fixed-size sequential collection. When an array is created, the elements are stored in a numbered sequence, starting with zero. The number of elements in an array is represented by the array’s length.
Arrays are crucial in computer programming because they allow programmers to process vast amounts of data quickly and efficiently. Aside from holding temporary data, arrays are also handy for storing large amounts of data since they can be allocated quickly and released quickly. Finally, arrays can be used to store items that must be retrieved in a sequential sequence, such as the elements of a list or the elements of a set.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
For the step by step tutorial on how to create for loop statement in C#, please watch the video uploaded on our YouTube Channel.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
C# For Loop Statement Video Tutorial and Source code
C# While Loop Statement Video Tutorial and Source code
C# NESTED IF Statement Video Tutorial and Source code
C# IF ELSE IF Statement Video Tutorial and Source code