C# IF ELSE IF Statement Video Tutorial and Source code
Table of Contents
This lesson is all about the decision control structures in C#, specifically the IF ELSE IF statement. The lesson includes sample programs to better understand how if statement works in C#.
The if else if statement is a conditional statement that allows for multiple conditions to be tested sequentially. If the first condition is met, the statement will execute the code block associated with that condition. If the first condition is not met, the system will test the next condition, and so on. If none of the conditions are met, the statement will execute the code block associated with the else keyword.
Objectives
By the end of this lesson, students should be able to:
- demonstrate essential understanding on the if else if statement
- create a program that implements the concept of if else if statement
- solve problems using the concept of if else if statement in C#.
Requirements
- Visual Studio IDE
The Visual Studio IDE (Integrated Development Environment) is a software program that lets you make software, websites, and online apps. It includes a code editor, a debugger, a project system, and a number of tools to aid software development.
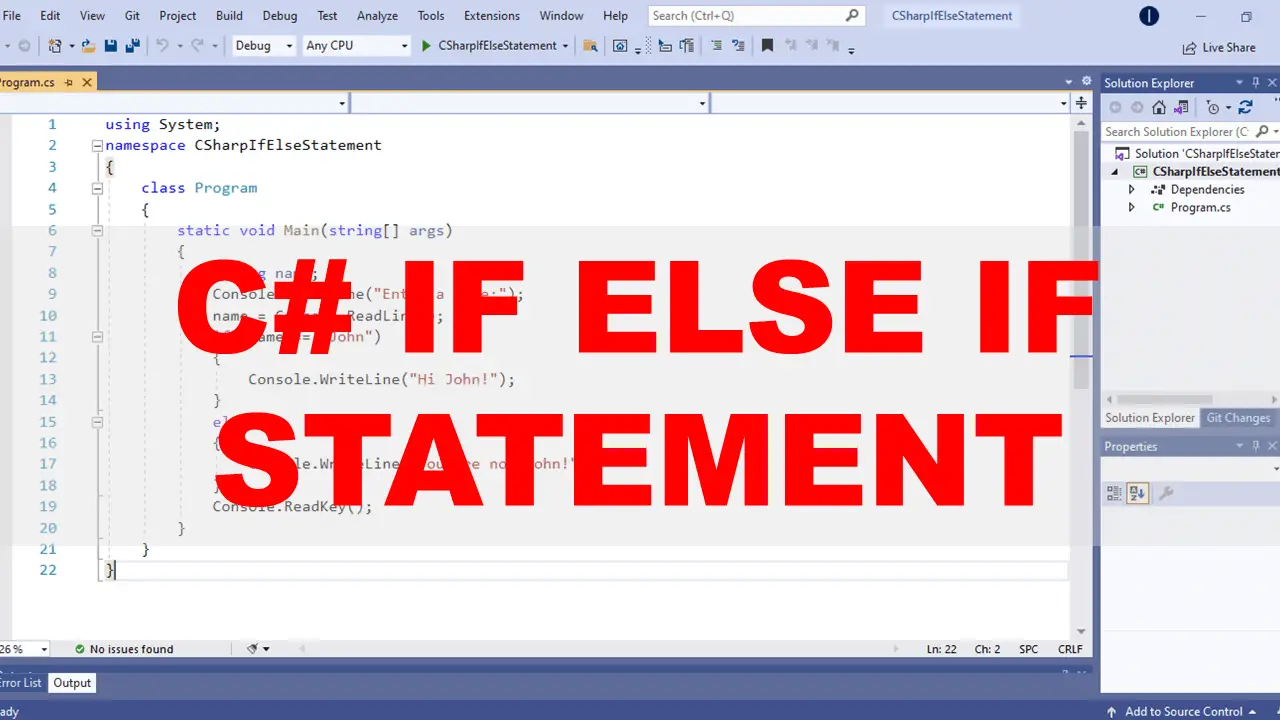
Lesson Proper
The if else if statement is a type of conditional statement that allows for multiple conditions to be checked. The statement will execute the code block associated with the first condition that is true. If no condition is true, the code block associated with the else statement will be executed. This statement is commonly used in computer programming to check for multiple conditions.
Here are some tips for writing if structures that we recommend:
– To avoid ambiguity, use blocks wrapped by curly brackets following if and else.
– For readability and to avoid misunderstanding, always format the code correctly by offsetting it with one tab inwards after if and else.
Here is the example source code of if else if statement in C#. We will explain source code line by line for you to better understand how if statement works and function.
How it works
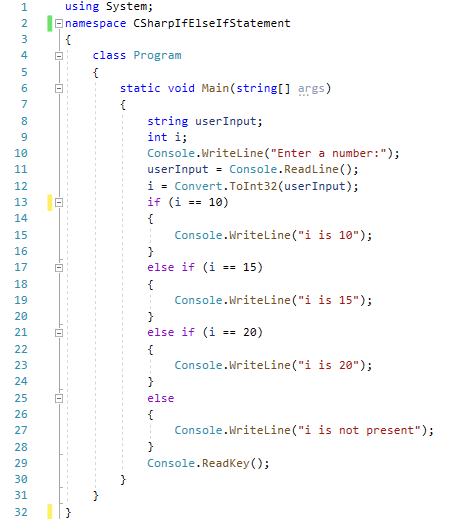
Line 1 – To add namespaces and class files, we commonly use the using keyword. It then makes all of the classes, interfaces, and abstract classes, as well as their methods and properties, available on the current page. Console is a member function or method in the System namespace. Because we need the console function to show and read user inputs, we must include it on the first line of our program.
Line 2 – this line indicates the name of the program (CSharpIfElseIfStatement).
Line 6 – the entry point of C# program is the main method.
Line 8 – this line is a declaration of variable named userInput.
Line 9 – next is to declare a variable i of integer type.
Line 10 – the purpose of this line is to display the text Enter a name: in the console.
Line 11 – Console.ReadLine takes user input and stores it in the userInput variable that we have declared in line 8.
Line 12 – By default, the Console.ReadLine() method in C# reads a string value from the console. In order to treat the user input as integer, we will use Convert.ToInt32; is a method to convert text to an integer.
Line 13-28 – this is the scope of the if else if statement, it will start at line 13 and ends at line 28.
Line 13 – this is the start of if else if statement. It is where the conditional statement is being evaluated.
i == 10
Line 15 – if the user input or the value of the I variable is equal to 10 then this line will be executed. The console will the display the text i is 10.
Line 17 – this is first else if statement, it has also a conditional statement.
i is 15
Line 19 – The line will only be executed if the value of i variable is 15. The console will the display the text i is 15.
Line 21 – this is second else if statement, it has also a conditional statement.
i is 20
Line 23 – The line will only be executed if the value of i variable is 20. The console will the display the text i is 20.
Line 25 – this is else statement, this is where the program will proceed if the user input has not met the criteria of each else if statement.
Line 27 – this is the body of the else statement. The console will display i is not present, which means that the value of i is not 10, 15 or 20.
Line 29 – the purpose of this line is to prevent the console from closing unless the user will press a key in the keyboard.
using System; namespace CSharpIfElseIfStatement { class Program { static void Main(string[] args) { string userInput; int i; Console.WriteLine("Enter a number:"); userInput = Console.ReadLine(); i = Convert.ToInt32(userInput); if (i == 10) { Console.WriteLine("i is 10"); } else if (i == 15) { Console.WriteLine("i is 15"); } else if (i == 20) { Console.WriteLine("i is 20"); } else { Console.WriteLine("i is not present"); } Console.ReadKey(); } } }
Video Tutorial
Summary
An “if else if” statement is similar to an “if”, except that it allows a programmer to test multiple statements and take different actions based on the results of the tests. The “if else if” statement is followed by multiple conditions, which are generally comparisons between values. If any one of the conditions is true, the programmer specifies a set of instructions to be executed. If all conditions are false, the “if else if” statement does not execute any instructions and the program proceeds to the else statement.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
For the step by step tutorial on how to create the if else if statement console application in C#, please watch the video uploaded on our YouTube Channel.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
C# IF ELSE Statement Video Tutorial and Source code
C# IF Statement Video Tutorial and Source code
Count All Data Registered in Database Using C#
Encrypt and Decrypt Password Using C#
Select data in DataGridView Rows and Show in TextBox Using C# MySQL Database