C# IF Statement
Introduction
Table of Contents
This lesson is all about the decision control structures in C#, specifically the IF statement. The lesson includes sample programs to better understand how if statement works in C#.
Objectives
By the end of this lesson, students should be able to:
- demonstrate essential understanding on the if statement
- create a program that implements the concept of if statement
- solve problems using the concept of if statement in C#.
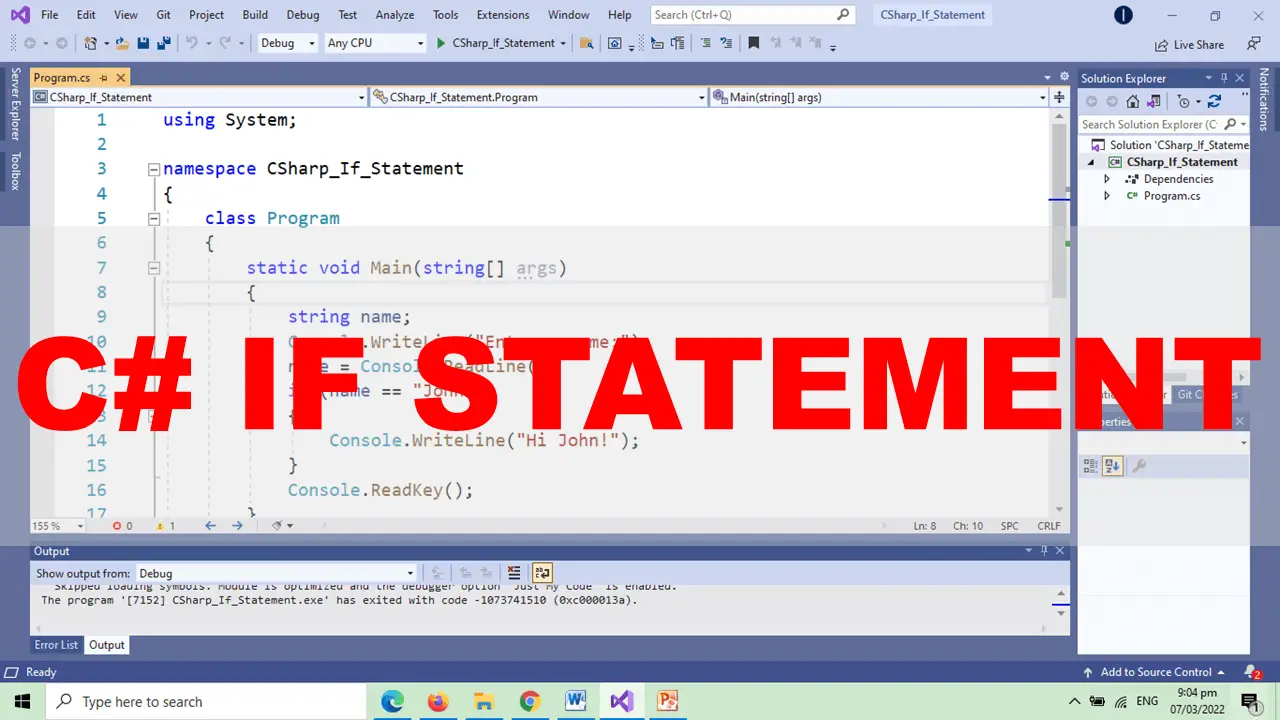
Requirements
- Visual Studio IDE
The Visual Studio IDE (Integrated Development Environment) is a software program that lets you make software, websites, and online apps. It includes a code editor, a debugger, a project system, and a number of tools to aid software development.
Lesson Proper
Conditional statements, expressions, or simply conditionals are programming language elements that instruct the computer to perform specific actions if particular criteria are met.
An if statement is a conditional statement in programming that, if true, performs a function or displays data.
Here is the example source code of if statement in C#. We will explain source code line by line for you to better understand how if statement works and function.
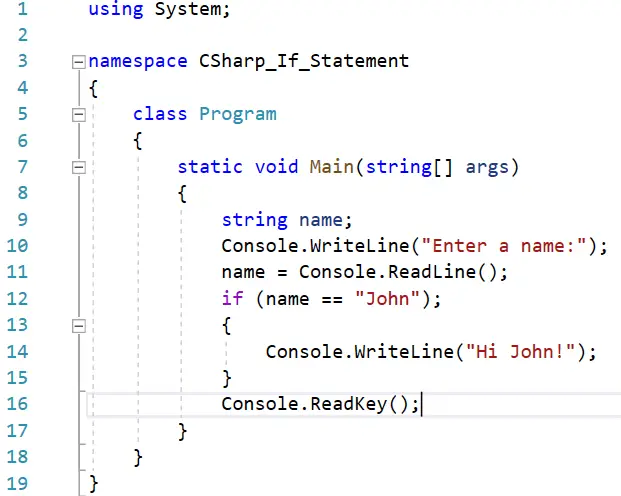
Line 1 – In code-behind and class files, we usually utilize the using keyword to add namespaces. Then, in the current page, it makes all of the classes, interfaces, and abstract classes, as well as their methods and properties, available. Console is a method or member function inside the namespace of System. We need to add it on the first line of our program since we need the console method to display and read user inputs.
Line 3 – the name of our program is CSharp_If_Statement. Please read the guidelines on how to declare literals in C#.
Line 7 – every program in C# starts in the Main Method, this is the entry point of all C# program.
Line 9 – we have declared a variable name of string type, which means that the variable can hold and handle alphanumeric values.
Line 10 – the purpose of this line is to display the text Enter a name in the console.
The Console class in C# represents the console application’s standard input, output, and error streams. WriteLine is a method that prints the values or text in the console.
Line 11 – Console.ReadLine gets user input and place that value in the name variable that we have declared earlier.
Line 12-15 – this is the scope of if statement. The condition states that if the user input or the value entered by the user is equivalent to the condition, then it will execute the body of the if statement. Example if the user enters the name John, then the value of our variable name is John which is equal to the condition (if (name == “John”);).
The == operator is the most frequent technique to compare objects in C#.
Line 16 – the purpose of this line is to prevent the console from closing unless the user will press a key in the keyboard.
Video Tutorial
using System; namespace CSharp_If_Statement { class Program { static void Main(string[] args) { string name; Console.WriteLine("Enter a name:"); name = Console.ReadLine(); if (name == "John"); { Console.WriteLine("Hi John!"); } Console.ReadKey(); } } }
Summary
The IF statement is a conditional statement that allows you to run a block of code only if one or more conditions are met. It can be used to determine whether something is equal, unequal, or has a specific value.
We have also learned the purpose and uses of WriteLine, ReadLine and ReadKey method.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
For the step by step tutorial on how to create the if statement console application in C#, please watch the video uploaded on our YouTube Channel.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
Count All Data Registered in Database Using C#
Find Missing Number Series in Database Using C#
Find Min Number Series in Database Using C#
Change DataGridView Columns Title with Database Using C#
Encrypt and Decrypt Password Using C#