Centimeter to Meter in CSharp
Introduction
Table of Contents
Welcome to the lesson on creating a Centimeter to Meter converter in C#! In this lesson, we will learn how to write a program that converts a length in centimeters to meters. Understanding how to perform conversions between different units of measurement is an important skill in programming, and this lesson will demonstrate how to implement such a conversion in C#.
By the end of this lesson, you will have a clear understanding of how to write a C# program that takes a length in centimeters as input and converts it to meters. You will also gain familiarity with handling user input and displaying the converted result.
Objectives of the Lesson
Students will not only gain proficiency in creating a centimeter to meter converter in C#, but also develop a deeper understanding of fundamental programming concepts and problem-solving skills applicable to a broader range of programming scenarios.
- Objective: Understand the concept of unit conversion in programming.
Outcome: Students will comprehend the importance of unit conversion in practical programming scenarios and recognize the need for accurate and efficient conversion algorithms.
- Objective: Implement a C# program that converts centimeters to meters.
Outcome: Students will be able to write a C# program that accepts a length in centimeters as input, performs the conversion to meters, and displays the result accurately.
- Objective: Handle user input and data validation.
Outcome: Students will gain the ability to prompt the user for input, validate the input for numeric values, and provide appropriate error messages for non-numeric inputs.
- Objective: Display the converted result using appropriate formatting.
Outcome: Students will learn how to present the converted result to the user in a clear and readable format using string interpolation or formatting techniques.
- Objective: Apply problem-solving skills to handle potential errors and edge cases.
Outcome: Students will develop problem-solving abilities by incorporating error handling mechanisms for scenarios such as invalid user input or division by zero, ensuring the program’s robustness and reliability.
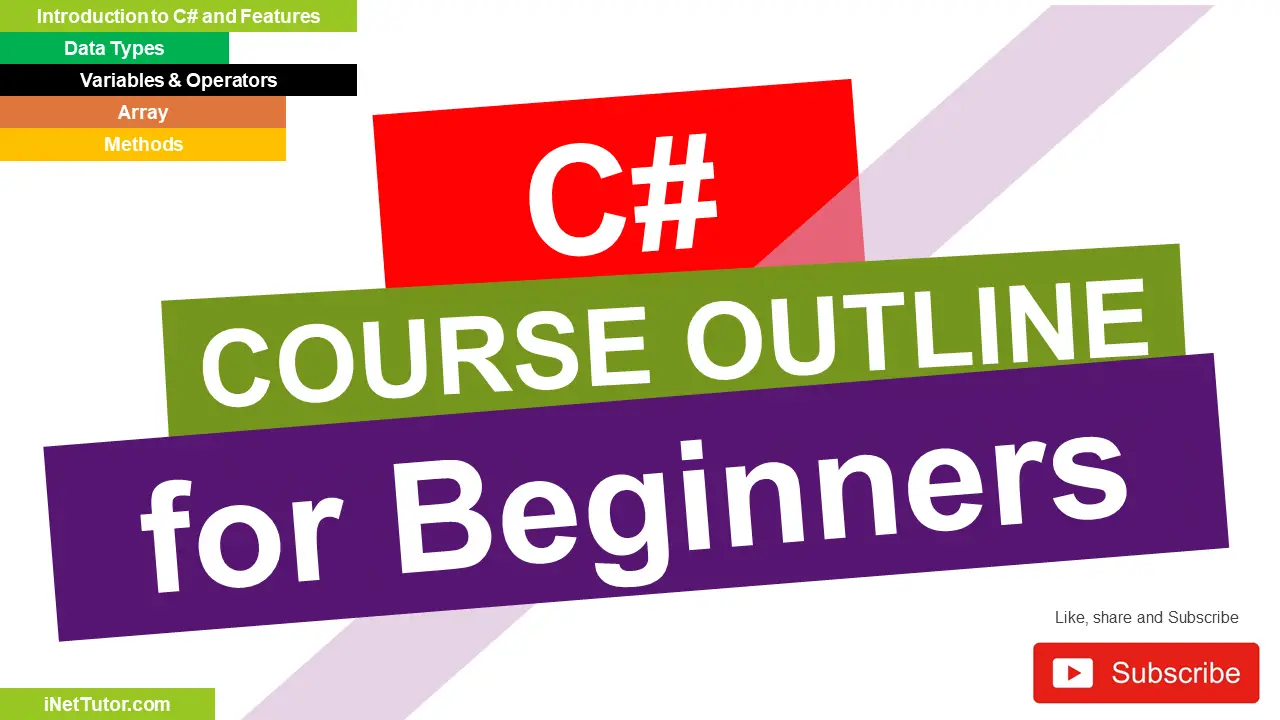
Lesson Proper
The concept of unit conversion involves converting a value from one unit of measurement to another. In programming, unit conversion is often encountered when dealing with different measurement systems or when converting data between different units for calculations or display purposes.
Unit conversion is essential in many real-world scenarios. For example, converting temperature from Celsius to Fahrenheit, converting distance from meters to feet, or converting currency from one currency to another. Being able to perform accurate and efficient unit conversions is crucial in various domains, including scientific calculations, engineering, finance, and more.
To create a simple program in C# using the console for unit conversion, you would typically follow these steps:
- Set up the project: Create a new console application project in an integrated development environment (IDE) like Visual Studio. This will provide you with a blank canvas to write your C# code.
- Define the problem statement: Identify the specific units of measurement you want to convert and determine the conversion formula or ratio between the two units.
- Prompt the user for input: Use the console to ask the user to enter the value they want to convert. Read the user’s input using Console.ReadLine() and store it in a variable.
- Perform the conversion: Apply the conversion formula or ratio to the user’s input to calculate the converted value. Use appropriate variables and arithmetic operations to perform the conversion.
- Display the result: Output the original value and the converted value to the console. You can use Console.WriteLine() or other output methods to display the results in a readable format.
- Test and handle errors: Run the program and test it with different input values to ensure it produces accurate results. Implement error handling mechanisms to handle scenarios such as invalid input or divide-by-zero errors.
A simple program in C# can be written using the Console class to create a console application. The Console class provides methods for input and output operations, allowing interaction with the user through the console window. By utilizing these methods and applying appropriate logic, we can create a program that performs unit conversions and displays the results.
Example Source code
using System; namespace LengthConverter { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Length Converter"); Console.WriteLine("1. Convert centimeters to meters"); Console.WriteLine("2. Convert meters to centimeters"); Console.WriteLine("Enter your choice (1 or 2):"); int choice; bool validChoice = int.TryParse(Console.ReadLine(), out choice); if (validChoice) { if (choice == 1) { double centimeters; bool validCentimeters = false; do { Console.WriteLine("Enter length in centimeters:"); validCentimeters = double.TryParse(Console.ReadLine(), out centimeters); if (!validCentimeters) { Console.WriteLine("Invalid input. Please enter a valid numeric value for centimeters."); } } while (!validCentimeters); double meters = centimeters / 100; Console.WriteLine($"{centimeters} centimeters = {meters} meters"); } else if (choice == 2) { double meters; bool validMeters = false; do { Console.WriteLine("Enter length in meters:"); validMeters = double.TryParse(Console.ReadLine(), out meters); if (!validMeters) { Console.WriteLine("Invalid input. Please enter a valid numeric value for meters."); } } while (!validMeters); double centimeters = meters * 100; Console.WriteLine($"{meters} meters = {centimeters} centimeters"); } else { Console.WriteLine("Invalid choice. Please enter either 1 or 2."); } } else { Console.WriteLine("Invalid choice. Please enter either 1 or 2."); } Console.ReadKey(); } } }
Output
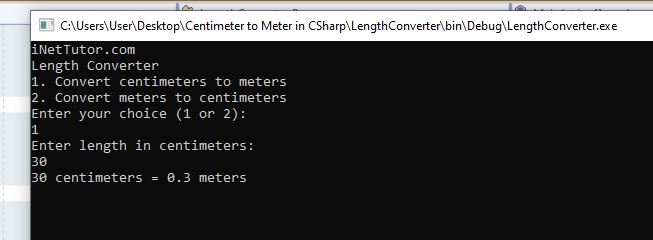
Code Explanation
The source code provided is a C# console application that allows the user to convert lengths between centimeters and meters. Let’s go through the code step by step:
- The code starts with the necessary using statements, including System, which provides access to fundamental classes and functionality.
- The code defines a namespace called LengthConverter, which helps organize related classes and program elements.
- Inside the namespace, there is a class called Program which serves as the entry point for the application.
- The Main method is the starting point of execution for the program. It accepts an array of strings args as a parameter.
- The first few lines within the Main method use Console.WriteLine to display introductory messages and instructions to the user.
- The program then prompts the user to enter their choice (either 1 or 2) for the conversion type (centimeters to meters or meters to centimeters).
- The int.TryParse method is used to read the user’s input and attempt to parse it as an integer. The result is stored in the choice variable.
- The program checks if the user’s choice is valid by evaluating the validChoice variable. If it is valid, the program proceeds with the chosen conversion.
- If the user chooses option 1 (centimeters to meters), the program enters a do-while loop. It prompts the user to enter the length in centimeters and attempts to parse it as a double using double.TryParse. The result is stored in the centimeters variable.
- Inside the loop, there is error handling to check if the user input for centimeters is valid. If it is not a valid numeric value, an error message is displayed.
- If the user’s input for centimeters is valid, the program calculates the equivalent length in meters by dividing centimeters by 100.
- The program then outputs the original length in centimeters and the converted length in meters using string interpolation.
- If the user chooses option 2 (meters to centimeters), a similar process is followed with the variables meters and centimeters reversed.
- If the user enters an invalid choice (neither 1 nor 2), an error message is displayed.
- Finally, Console.ReadKey is used to prevent the console window from closing immediately, allowing the user to view the output before exiting the program.
This code demonstrates how to implement a centimeter to meter converter and vice versa in C#. It incorporates user input, error handling, and output formatting, providing a simple yet functional program for length conversions.
Summary
In the lesson on “Creating Centimeter to Meter Converter in C#,” we explored the process of creating a simple program that converts lengths between centimeters and meters. By understanding the conversion formula and utilizing C# programming concepts, we developed a functional console application.
Throughout the lesson, we covered essential aspects of the program, including handling user input, performing the conversion, and displaying the results. We incorporated error handling mechanisms to validate user input, ensuring that only valid numeric values were accepted. The program utilized string interpolation to format and display the converted lengths accurately.
By following this lesson, learners gained knowledge in various areas, such as:
- Implementing console-based programs in C#.
- Using Console class methods for input/output operations.
- Validating and parsing user input.
- Applying conversion formulas for unit conversions.
- Utilizing error handling techniques to enhance program robustness.
- Formatting output messages for clear and readable results.
Creating the centimeter to meter converter in C# not only provided a practical example of unit conversion but also fostered understanding of fundamental programming concepts. Learners gained experience in data manipulation, user interaction, and program flow control.
To further enhance their skills, learners are encouraged to experiment with the code, explore additional unit conversions, and apply their knowledge to real-world scenarios. Additional topics for exploration include incorporating other measurement units, implementing a graphical user interface, or building more complex conversion programs.
By mastering the concepts covered in this lesson, learners are equipped with the foundational skills needed to tackle diverse programming challenges and expand their proficiency in C# programming.
Related Topics and Articles:
C# Program Structure and Basic Syntax
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Quiz
- What is the purpose of this lesson?
a) To create a Centimeter to Meter converter in C#
b) To explore fundamental programming concepts
c) To learn about unit conversions
d) All of the above - What is the entry point for the program?
a) Main method
b) Console.WriteLine
c) LengthConverter namespace
d) Program class - What does the line Console.WriteLine(“Enter your choice (1 or 2):”); do?
a) Prompts the user to enter their choice
b) Converts centimeters to meters
c) Converts meters to centimeters
d) None of the above - What is the purpose of the do-while loop in the code?
a) It calculates the equivalent length in meters
b) It checks if the user’s input for centimeters is valid
c) It displays the original length in centimeters and the converted length in meters
d) None of the above - What does the line Console.ReadKey(); do?
a) Ends the program
b) Waits for the user to press a key before closing the console window
c) Displays the result of the conversion
d) None of the above - What is the purpose of the int.TryParse(Console.ReadLine(), out choice); line?
a) It converts the user’s input to an integer
b) It checks if the user’s choice is valid
c) It performs the conversion from centimeters to meters
d) None of the above - What does the line double meters = centimeters / 100; do?
a) Converts centimeters to meters
b) Converts meters to centimeters
c) Checks if the user’s input for centimeters is valid
d) None of the above - What happens if the user enters an invalid choice (neither 1 nor 2)?
a) The program ends
b) An error message is displayed
c) The program continues with the chosen conversion
d) None of the above - What is the purpose of the string interpolation in the code?
a) To format the output message with variables
b) To convert strings to integers
c) To handle user input
d) None of the above - What is the overall objective of the lesson?
a) To understand unit conversions in programming
b) To handle user input and validation
c) To create a functional centimeter to meter converter in C#
d) All of the above