C# Program Structure and Basic Syntax
Introduction
Table of Contents
Welcome to the lesson on “Introduction to C# Program Structure and Basic Syntax.” In this lesson, we will dive into the fundamental aspects of C# programming, focusing on understanding the structure and syntax of C# programs. Whether you are a beginner starting your coding journey or an experienced programmer looking to expand your skills, this lesson will provide a solid foundation in C# programming.
C# is a versatile and powerful programming language widely used in various domains, including software development, web applications, game development, and more. Having a solid understanding of program structure and syntax is essential to write clean, readable, and efficient code.
Objectives
By the end of this lesson, you will be able to:
- Understand the components of a C# program and their roles: Gain a clear understanding of the essential parts of a C# program, such as the main method, namespaces, and classes. Learn how these components work together to create a functional program.
- Comprehend the purpose of statements and expressions: Learn the distinction between statements and expressions in C# and understand how they contribute to the overall program execution flow. Explore various types of statements and expressions commonly used in C# programming.
- Identify the significance of comments and documentation: Grasp the importance of adding comments and documentation within your code. Learn how to effectively use comments to provide clarity, improve code readability, and document your program’s logic and functionality.
- Gain familiarity with naming conventions and best practices: Understand the significance of consistent naming conventions in C# programming. Learn about the recommended naming conventions for variables, methods, classes, and other program elements. Explore best practices to write clean, maintainable, and professional code.
- Analyze and interpret sample C# program structures: Study and analyze sample C# program structures to develop a deeper understanding of how different components come together. Gain the ability to interpret and explain the structure and purpose of various parts of a C# program.
By achieving these objectives, you will have a comprehensive understanding of the basic structure of a C# program. This knowledge will serve as a solid foundation for your further exploration and mastery of C# programming concepts and allow you to write well-structured and efficient code.
Source Code Example
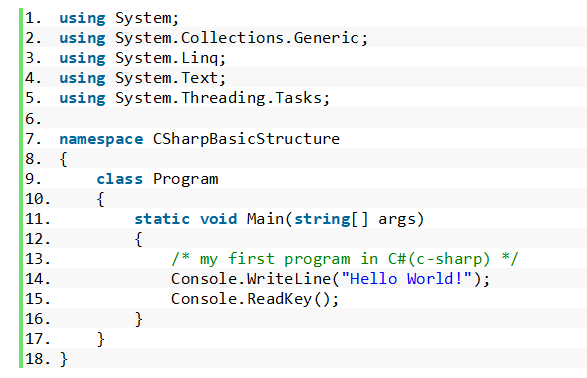
Code Explanation
- Line 1: The “using System;” statement is used to include the System namespace, which provides access to fundamental classes and functionality in C#.
- Line 2: The “using System.Collections.Generic;” statement is used to include the System.Collections.Generic namespace, which provides generic collection classes and interfaces.
- Line 3: The “using System.Linq;” statement is used to include the System.Linq namespace, which provides language-integrated query capabilities in C#.
- Line 4: The “using System.Text;” statement is used to include the System.Text namespace, which provides classes for manipulating strings and characters.
- Line 5: The “using System.Threading.Tasks;” statement is used to include the System.Threading.Tasks namespace, which provides classes and methods for asynchronous programming.
- Line 7: The “namespace CSharpBasicStructure” statement declares a namespace called “CSharpBasicStructure”. Namespaces are used to organize and group related classes and other program elements.
- Line 9: The “class Program” statement defines a class named “Program”. It serves as the entry point for the program and contains the program’s code.
- Line 11: The “static void Main(string[] args)” statement declares the main method. It is the starting point of execution for the program. Code inside this method will be executed when the program is run.
- Line 13: The comment “/* my first program in C#(c-sharp) */” is a single-line comment. It is not executed by the program but provides descriptive information or notes for the developers.
- Line 14: The “Console.WriteLine(“Hello World!”);” statement outputs the text “Hello World!” to the console. It is used to display a message or result on the console screen.
- Line 15: The “Console.ReadKey();” statement waits for the user to press a key before closing the console window. It allows the user to view the output of the program before it exits.
This code sets up the basic structure of a C# program. It includes necessary namespaces, defines the program’s entry point (the Main method), and demonstrates the use of comments, outputting messages, and pausing the program’s execution.
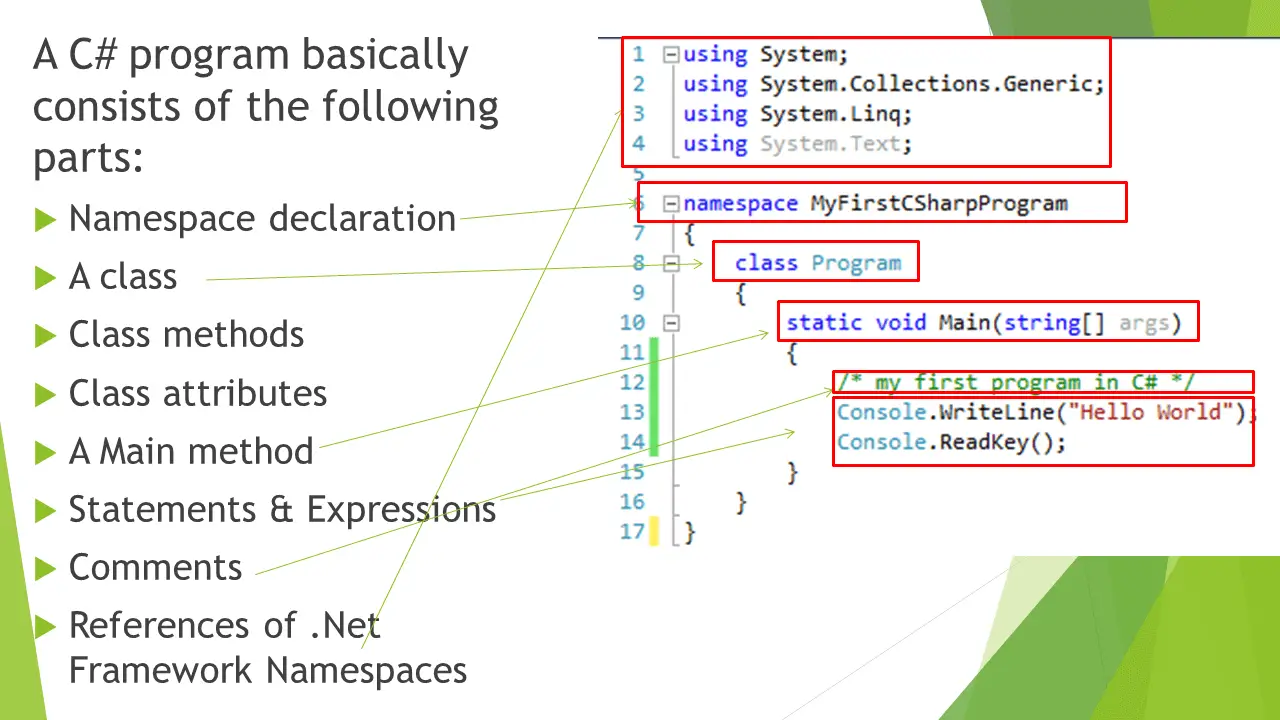
Summary
In this lesson on “Introduction to C# Program Structure and Basic Syntax,” we explored the fundamental components and syntax of a C# program. We learned about namespaces, classes, methods, statements, expressions, and the main method as the entry point of a C# program. We also discussed the importance of comments, naming conventions, and code organization.
Recap of Key Concepts:
- C# program structure: We understood the structure of a C# program, including namespaces, classes, and methods.
- Main method: We learned about the Main method, which serves as the entry point for program execution.
- Statements and expressions: We distinguished between statements and expressions and understood their role in program flow.
- Comments and documentation: We explored the significance of adding comments and documentation to our code for clarity and maintainability.
- Naming conventions and best practices: We discussed the importance of consistent naming conventions and best practices for writing clean and professional code.
Resources for Further Learning and Practice:
To further enhance your understanding and skills in C# programming, consider exploring the following resources:
- Official Microsoft C# Documentation: The official documentation provides comprehensive information on C# syntax, features, and programming concepts. Visit the Microsoft Docs website for detailed C# documentation.
- Online C# Tutorials: Various online platforms offer interactive tutorials, exercises, and projects to practice your C# programming skills. Websites like Codecademy, Pluralsight, and Udemy offer C# courses for beginners and intermediate learners.
- C# Programming Books: There are numerous books available on C# programming that cover topics ranging from basic syntax to advanced concepts.
- Community Forums and Discussion Boards: Engage with the C# programming community by participating in forums like Stack Overflow and Reddit. These platforms allow you to ask questions, seek guidance, and learn from experienced developers.
Remember, practice is key to mastering any programming language. Try solving coding challenges, work on small projects, and collaborate with fellow learners to reinforce your understanding of C# programming concepts. Happy coding!
Related Topics and Articles:
Introduction to Information Systems and Computer Programming
Odd Even Number Checker in C-Sharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Quiz
- What is the purpose of the “Main” method in a C# program?
a. It defines the namespace of the program.
b. It serves as the entry point for program execution.
c. It declares variables used in the program.
d. It prints output to the console. - What is the significance of using namespaces in C#?
a. Namespaces provide a way to comment your code.
b. Namespaces organize and group related classes and program elements.
c. Namespaces control the flow of program execution.
d. Namespaces store user input in variables. - What is the purpose of comments in C# code?
a. Comments control the execution of the program.
b. Comments are used to output messages to the console.
c. Comments provide descriptive information, notes, or explanations to developers.
d. Comments define the structure of a C# program. - Which of the following is NOT a valid C# naming convention?
a. camelCase
b. PascalCase
c. snake_case
d. kebab-case - What does the “Console.WriteLine” statement do?
a. It waits for user input.
b. It displays output to the console.
c. It declares a variable.
d. It defines a class. - What is the purpose of the “using” keyword in C#?
a. It declares a variable.
b. It initializes a loop structure.
c. It imports namespaces to provide access to classes and functionality.
d. It defines a class. - What is the role of the “static” keyword in the “Main” method?
a. It defines a variable that can be accessed from other methods.
b. It ensures the program runs in a single thread.
c. It specifies that the method belongs to the class and not an instance of the class.
d. It enables input/output operations. - What does the “Console.ReadKey” statement do?
a. It waits for user input and stores it in a variable.
b. It displays output to the console.
c. It waits for the user to press a key before closing the console window.
d. It defines a loop structure. - Which of the following is a single-line comment in C#?
a. /* This is a comment */
b. <!– This is a comment –>
c. // This is a comment
d. ”’ This is a comment - Which part of the C# program is responsible for displaying “Hello World!” on the console?
a. Namespace declaration
b. Class definition
c. Main method
d. Comment statement
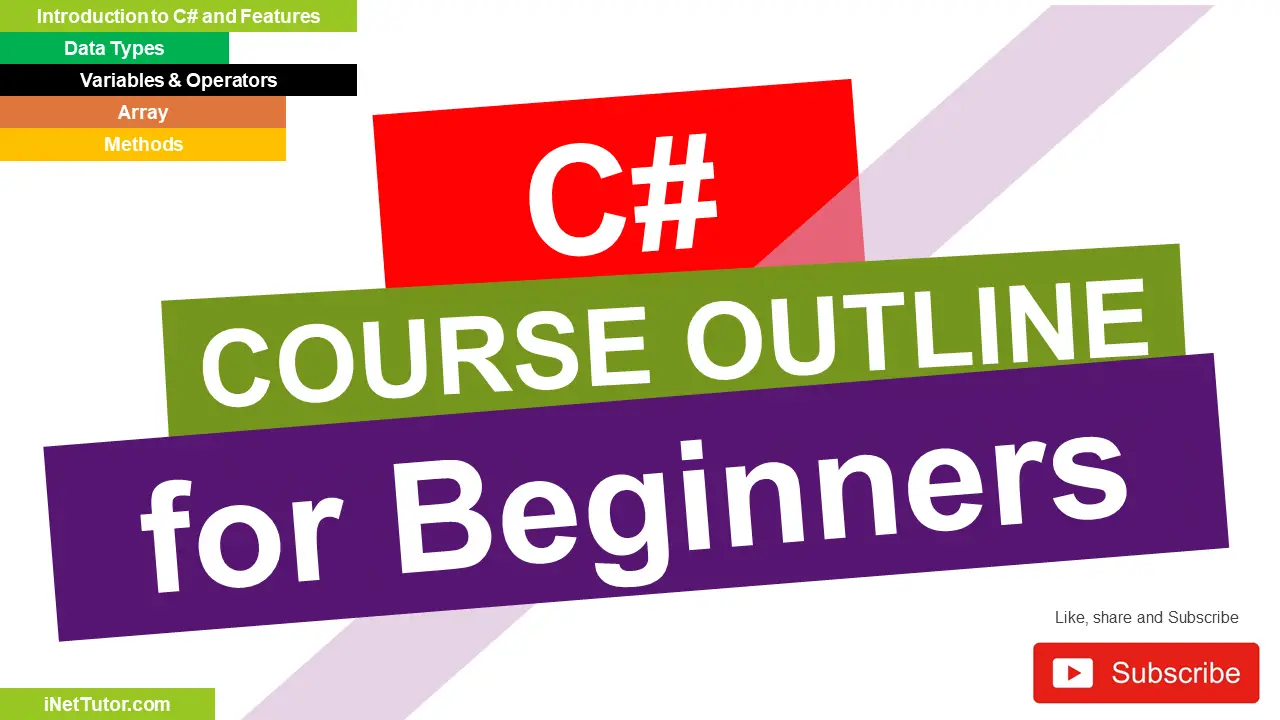