Find Missing Number Series in Database Using C#
In this article I will show you how to find missing number series in database using c#, let’s follow code below
Step 1:
Create Project And Add Reference To C# WinForms Project. (visit the link to the first tutorial on how to add reference to our C# project)
How to connect MySQL Database to C# Tutorial and Source code
Step 2:
Open MySQL Workbench, right click and create schema (new database), give database name as “sampledb” and create table in database and give a name as “information”, then create columns id, emp_id, name, designation, username and password.
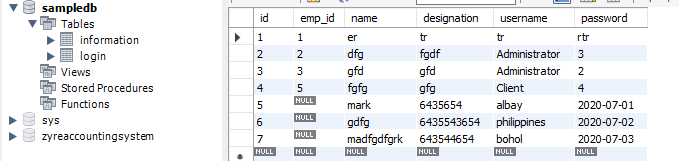
Step 3:
Back to windows form application and design form like this (see below image)
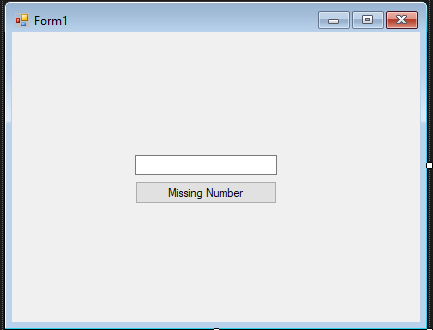
Step 4:
Code for button(Find Missing Number) – The Missing Number in Database is 4
using MySql.Data.MySqlClient; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace findmissingnumberseries { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { MySqlConnection con = new MySqlConnection("datasource= localhost; database=sampledb;port=3306; username = root; password= db1234"); con.Open(); MySqlCommand cmd = new MySqlCommand("SELECT t1.emp_id+1 AS emp_id FROM information AS t1 LEFT JOIN information AS t2 ON t1.emp_id+1 = t2.emp_id WHERE t2.emp_id IS NULL", con); MySqlDataReader myread = cmd.ExecuteReader(); if (myread.Read()) { textBox1.Text = myread.GetInt32("emp_id").ToString(); myread.Close(); cmd.Dispose(); } cmd.Dispose(); myread.Close(); con.Close(); } } }
Code Explanation:
This code explain, how to find missing number series in database, and display to textbox
Output:
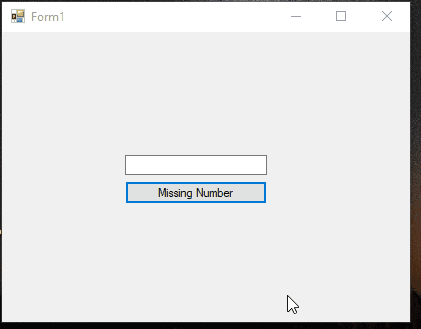
Find Missing Number Series in Database Using C# Free Download Source code
Mark Jaylo
YTC: https://www.youtube.com/c/MarkTheProgrammer
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.