How to connect MySQL Database to C# Tutorial and Source code
Connect C# to MySQL
This article show step by step manner on how to use and connect C# with MySQL using MySQL Connect/NET
Download MySQL Connector/NET
First make sure you have downloaded and installed the MySQL Connector/NET from this link https://dev.mysql.com/downloads/connector/net/ MySQL official website
Add Reference
Before you connect application to MySQL, you need to add MySQL reference in your project.
To do this, right click reference and click Add Reference and Choose “MySQL.Data.dll”
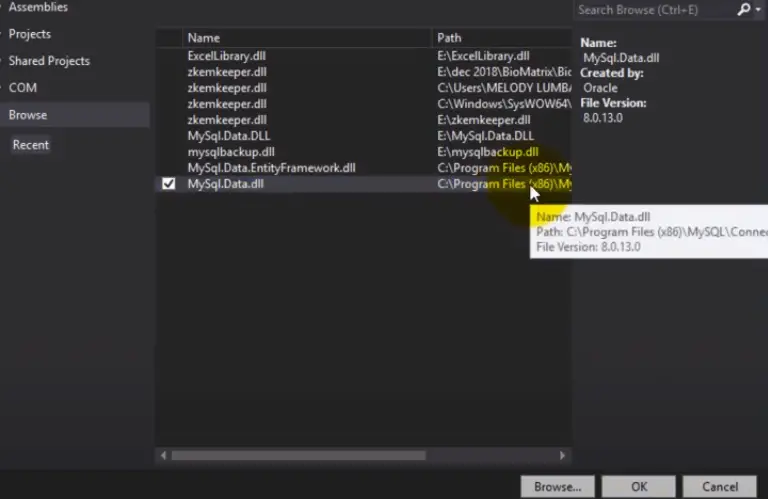
Next, add MySQL library to your project.
using Mysql.Data.MysqlClient;
C# MySQL connection string
string connectionstring = “datasource=localhost;database=sampledb;port=3306;username=root;password=db123”;
For multiple Server this is the connection string if the server in a replicated server configuration
“datasource=localhost;database=dbname1,dbname2;port=3306;username=root;password=db123”;
I created a simple form with a button in it, our connection will be established when the button is clicked
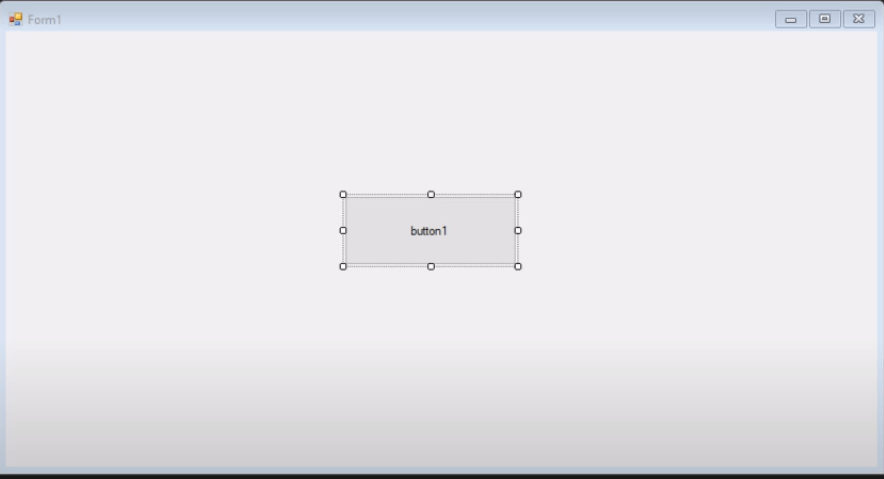
Now the code for the button clicked event:
using System; using System.Windows.Forms; using MySql.Data.MySqlClient; namespace WindowsApplication1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { string connectionstring=“datasource=localhost;database=sampledb;port=3306;username=root;password=db123”; MySqlConnection con = new MySqlConnection(connectionstring); try { con.Open(); MessageBox.Show ("Connected"); con.Close(); } catch (Exception ex) { MessageBox.Show("Connection Not Established"); } } } }
Code Explanation:
These code serve as the connection from MySQL Database to the C# Project
How to connect MySQL Database to C# Free Download Source code
Mark Jaylo
markjaylo26@gmail.com
link: https://www.youtube.com/watch?v=CFobuYUDXe0&list=PLyrZdI7gZW7qhN-RwK1b5EqIzfHz5fh9F
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.