Simple Login in C# Console
Are you interested in learning how to create a login system using C# console? If so, you’re in the right place! In today’s blog post, we will guide you through a step-by-step tutorial on how to build a simple login system in C# console.
This tutorial is perfect for beginners who want to learn the basics of C# console and user authentication. We will cover the general and specific objectives of the lesson, explain each part of the source code, and provide encouragement for you to practice and experiment with the code on your own. So, let’s dive into the world of C# console and create a login system that will help you level up your coding skills!
Introduction
Table of Contents
Welcome to this lesson on Simple Login in C# Console! In this lesson, we will be covering the basics of creating a login system in C# Console Application.
A login system is a common feature of many software applications, websites, and services. It is a security measure that requires users to provide a username and password to access a system or application. A login system is important because it allows you to control who has access to your application or system and helps to protect sensitive information from unauthorized access.
In this lesson, we will be creating a simple login system using C# Console Application. We will define the correct username and password and prompt the user to enter their credentials. We will then use if-else statements to check if the user’s input matches the correct credentials and display an appropriate message to the user based on whether their input was correct or not.
By the end of this lesson, you will have a solid understanding of how to create a basic login system in C# Console Application. So, let’s get started!
Objectives of the Lesson
General Objective:
By the end of this lesson, the learner will be able to create a simple login system using C# Console Application and understand the importance of implementing a login system in software applications.
Specific Objectives:
- Define the purpose and importance of a login system in software applications.
- Create a new C# Console Application in Visual Studio.
- Define and store the correct username and password as strings.
- Prompt the user to input their username and password using the Console.ReadLine() method.
- Use if-else statements to check if the user’s input matches the correct credentials.
- Display an appropriate message to the user based on whether their input was correct or not.
- Understand the basic structure of a login system and how it can be expanded upon to create more robust and secure systems.
- Apply the concepts learned in this lesson to create more advanced login systems in future projects.
By achieving these specific objectives, the learner will have a solid foundation in creating and understanding the importance of login systems in software applications. They will also have the necessary skills to build upon this foundation and create more advanced login systems in the future.
Lesson Proper
The purpose of this lesson on Simple Login in C# Console is to introduce learners to the concept of creating a basic login system using C# Console Application. This lesson covers the fundamental concepts of creating a login system such as defining the correct username and password, prompting the user to enter their credentials, and checking if the user’s input matches the correct credentials. Additionally, this lesson emphasizes the importance of implementing a login system in software applications as a security measure to protect sensitive information from unauthorized access. By the end of this lesson, learners will have a solid understanding of the basic structure of a login system and how it can be expanded upon to create more secure and robust systems.
Example Source code
Line 13-15

This code initializes two string variables named “username” and “password” with the values “testuser” and “testpass” respectively. These variables will be used later in the code to store the correct username and password that the user will need to enter in order to access the application.
In a real-world scenario, you would want to set these values to something more secure and complex than “testuser” and “testpass”. It is common practice to use a combination of letters, numbers, and special characters for passwords, and to store them in a secure manner such as in a database with proper encryption.
This code snippet demonstrates one of the basic building blocks of a login system – defining the correct credentials. Once these credentials are defined, the application can compare them with the user’s input and determine whether to grant or deny access to the system.
Line 17-19

This source code prompts the user to enter their username by displaying the message “Please enter your username:” on the console using the Console.WriteLine() method.
The Console.ReadLine() method is then called, which waits for the user to input their username and press the enter key. The inputted value is then stored as a string variable named “inputUsername”.
This code snippet is a crucial part of the login system, as it allows the user to input their username and provides a way for the application to receive and store the user’s input. The stored username is then later compared with the correct username to determine if the user has entered the correct credentials.
Line 21-23

This source code prompts the user to enter their password by displaying the message “Please enter your password:” on the console using the Console.WriteLine() method.
The Console.ReadLine() method is then called, which waits for the user to input their password and press the enter key. The inputted value is then stored as a string variable named “inputPassword”.
Similar to the previous code snippet, this part of the code is a crucial part of the login system as it allows the user to input their password and provides a way for the application to receive and store the user’s input. The stored password is then later compared with the correct password to determine if the user has entered the correct credentials.
Line 25-35
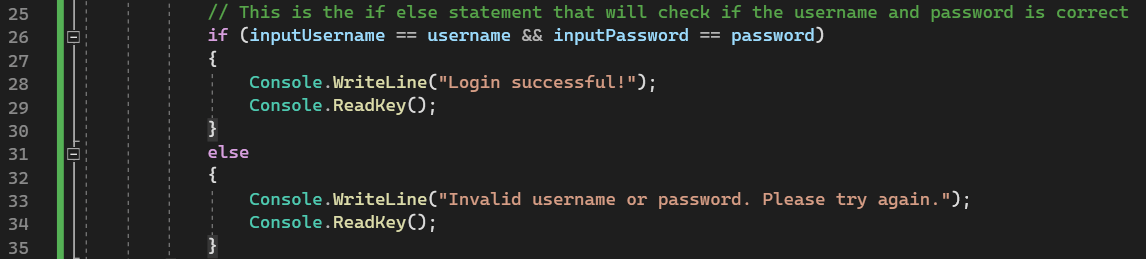
This code snippet contains an if-else statement that checks if the user’s inputted username and password match the correct username and password that were defined earlier in the code.
If the condition is true, meaning that the inputted username and password match the correct credentials, the code will execute the first block of code within the curly braces, which is to display the message “Login successful!” on the console using the Console.WriteLine() method. Then, the Console.ReadKey() method is called to wait for the user to press any key before the console window is closed.
If the condition is false, meaning that the inputted username and password do not match the correct credentials, the code will execute the second block of code within the curly braces, which is to display the message “Invalid username or password. Please try again.” on the console using the Console.WriteLine() method. Then, the Console.ReadKey() method is called to wait for the user to press any key before the console window is closed.
This if-else statement is a crucial part of the login system, as it checks whether the user’s input matches the correct credentials and determines whether to grant or deny access to the system.
Summary
In this lesson on Simple Login in C# Console, we have learned the basic building blocks of a login system, including prompting the user to input their username and password, comparing the inputted values with the correct credentials, and granting or denying access to the system based on the comparison.
We have gone through a step-by-step tutorial that demonstrates how to implement these building blocks in C# console. We have covered the general and specific objectives of the lesson, and explained each part of the source code.
As a beginner, it is essential to practice and experiment with the code on your own. By doing so, you can gain a deeper understanding of the concepts and improve your coding skills. You can modify the code to add additional features such as input validation, or create a more advanced login system using C# in a Windows Forms application.
In conclusion, we hope that this lesson has provided you with a solid foundation for creating login systems in C# console. Remember that the best way to learn is by doing, so we encourage you to continue practicing and exploring the code. Good luck!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.