C# Do While Loop Statement Video Tutorial and Source code
Introduction
Table of Contents
This lesson is all about the repetition control structures in C#, specifically the DO WHILE LOOP statement. The lesson includes sample programs to better understand how if statement works in C#.
The do while loop statement is a repeating statement that will execute a given block of code while a condition is true. The code inside the do while loop will execute at least once, because the condition is verified after the code block is executed.
Objectives
By the end of this lesson, students should be able to:
- demonstrate essential understanding on the repetition control structures.
- use do while loop statement in a C# program
- solve problems using the concept of do while loop statements in C#.
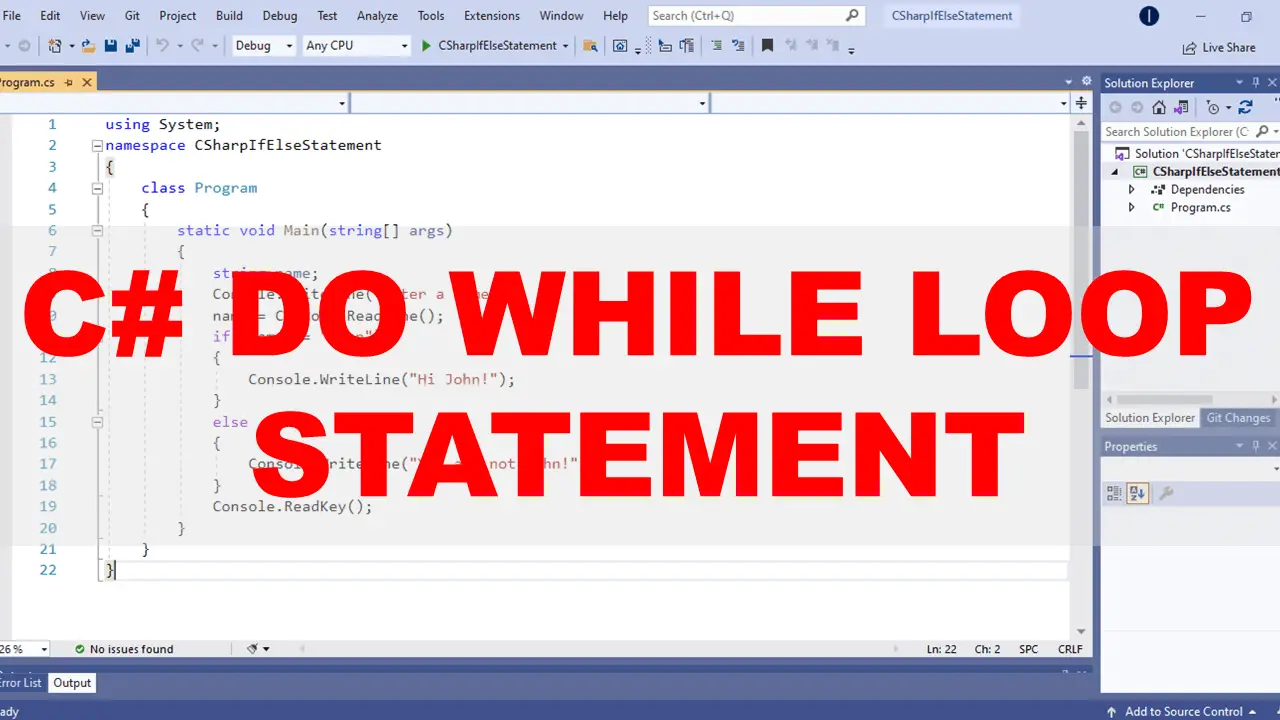
Lesson Proper
In programming, loops are used to repeatedly run a piece of code until a specific condition is satisfied.
C# programming has three types of loops.
- for loop
- while loop
- do while loop
In this tutorial, we will focus on the do while loop statement of C#.
The do-while loop is comprised of the do keyword, a code block, and a boolean expression, all of which are separated by the while keyword. When a boolean condition evaluates to false, the do while loop halts execution and terminates the loop. The while(condition) statement at the end of the block ensures that the code block will be executed at least once.
How it works
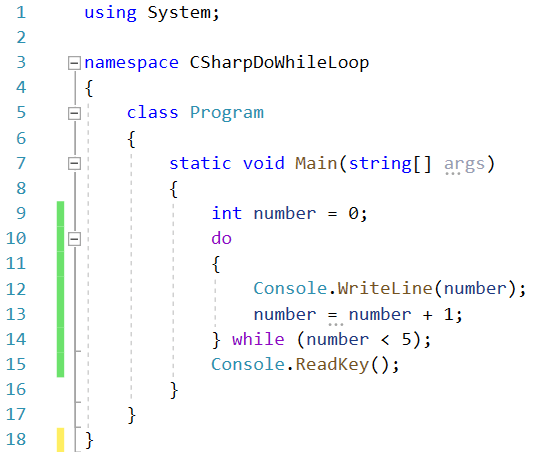
Line 1 – To add namespaces and class files, we commonly use the using keyword. It then makes all of the classes, interfaces, and abstract classes, as well as their methods and properties, available on the current page. Console is a member function or method in the System namespace. Because we need the console function to show and read user inputs, we must include it on the first line of our program.
Line 3 – this line indicates the name of the program which is CSharpDoWhileLoop.
Line 7 – The main method is where a C# program begins.
Line 9 – This line declares an integer-type variable called number. We’ve also set the variable’s initial value to zero.
Line 10 – 14 – this is the scope of the do while loop statement.
Line 10 – the do while loop starts in this line and will proceed directly to the body of the loop.
Line 12-13 – This is the do while loop’s body. The number of lines varies depending on the scope of the loop and the overall complexity of the program. The do while loop’s body only has two lines or statements in our scenario.
Line 12 – The value of the number variable will be displayed in the while loop’s body. The Console class’s WriteLine function is used to display text on the console.
Line 13 – The number variable will be increased by one with this line. Because we need to test the expression with the updated value of the variable, it is considered an update statement. This is also similar to number++.
Line 14 – this is while statement where the expression will be tested. The difference between while loop and do while loop is that the body of the loop will be executed at least once. While a while loop examines the condition before executing the statement(s), a do while loop will execute the statement(s) at least once before checking the condition. If the expression is true then it will continue to execute the loop body until the expression becomes or returns false.
Line 15 – This line’s function is to keep the console from closing unless the user presses a key on the keyboard.
using System; namespace CSharpDoWhileLoop { class Program { static void Main(string[] args) { int number = 0; do { Console.WriteLine(number); number = number + 1; } while (number < 5); Console.ReadKey(); } } }
Video Tutorial
Summary
Do while loops are used to repeat a block of code while a condition is met. The condition is tested at the while loop statement which is located at the end of the loop, and if it is true, the code within the loop is executed. This kind of loop will execute the body of the loop at least once since the evaluation of the expression is tested in the end. The condition is then tested again, and if it is still true, the code within the loop is executed again. This process continues until the condition is no longer true.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
For the step by step tutorial on how to create the do while statement in C#, please watch the video uploaded on our YouTube Channel.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
C# While Loop Statement Video Tutorial and Source code
C# NESTED IF Statement Video Tutorial and Source code
C# IF ELSE IF Statement Video Tutorial and Source code
C# IF ELSE Statement Video Tutorial and Source code
C# IF Statement Video Tutorial and Source code