Sum of Even Numbers in Csharp
Introduction
Table of Contents
In the world of computer programming, the ability to calculate sums and averages is fundamental, forming the backbone of various computational tasks. Whether analyzing data, optimizing algorithms, or generating statistics, these operations are omnipresent. This lesson delves into a specific facet of this computational prowess, focusing on the nuanced process of calculating the sum and average of even numbers within a given range. Using the C# programming language, we will explore a concise yet powerful code implementation that not only reinforces the principles of iteration and mathematical computation but also equips you with practical skills applicable across a spectrum of programming scenarios. Join us on this journey as we unravel the intricacies of sum and average calculations, demonstrating their significance in the vibrant landscape of programming with C#.
Learning Objectives
- Explore Looping Concepts:
- Gain a solid understanding of loops and their significance in programming.
- Recognize how loops facilitate efficient iteration through a range of numbers.
- Master User Input and Output Techniques in C#:
- Learn to interact with users through the console, acquiring essential skills in user input and output operations.
- Understand the role of the Console.ReadLine() method in capturing user input.
- Validate User Input for Robust Programs:
- Implement robust input validation techniques to ensure users provide meaningful and valid data.
- Explore conditional statements and loops to create a responsive user input validation mechanism.
- Calculate the Sum of Even Numbers:
- Dive into the logic of summing even numbers within a specified range.
- Understand the iterative process of adding even numbers and storing the cumulative sum.
- (Optional) Calculate the Average of Even Numbers:
- Extend your understanding to calculate the average of even numbers within the given range.
- Explore the relationship between the sum and the count of even numbers to determine the average.
By the end of this lesson, you will not only grasp the core concepts of loops, user input/output, and input validation but also possess the practical skills to develop a C# program that calculates the sum and average of even numbers. These skills lay a strong foundation for tackling diverse programming challenges and form an integral part of your programming toolkit.
Source code example
using System; namespace NumberSumCalculator { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); // Initialize sum and average variables int sum = 0; double average = 0.0; // User prompt and input validation bool validInput = false; int limit = 0; while (!validInput) { Console.WriteLine("Enter the upper limit for even numbers: "); string input = Console.ReadLine(); if (int.TryParse(input, out limit) && limit > 1) { validInput = true; } else { Console.WriteLine("Invalid input. Please enter a whole number greater than 1."); } } // Loop through and calculate sum and count int count = 0; for (int i = 2; i < limit; i += 2) { sum += i; count++; } // Calculate and display average if (count > 0) { average = (double)sum / count; Console.WriteLine($"The sum of even numbers from 2 to {limit} is: {sum}"); Console.WriteLine($"The average of even numbers from 2 to {limit} is: {average:F2}"); Console.ReadKey(); } else { Console.WriteLine("No even numbers within the specified range."); Console.ReadKey(); } } } }
Explanation
Namespace, Class Declaration, and Variable Initialization (line 1-16):
- This program is encapsulated within the NumberSumCalculator namespace, with the main logic residing in the Program class. It starts by initializing variables for the sum (int) and average (double) of even numbers.
User Input and Validation (line 17-29):
- A while loop handles user input and validation. The user is prompted to enter the upper limit for even numbers, and the input is checked to ensure it’s a whole number greater than 1. The loop continues until valid input is received.
Loop for Sum and Count (line 32-37):
- A for loop iterates through even numbers from 2 to the specified limit (excluding the limit itself). Within the loop, each even number is added to the sum, and the count is incremented.
Calculate and Display Average (line 40-51):
- After the loop, the program calculates the average if there are any even numbers within the specified range. It then displays the sum and average with appropriate formatting. If no even numbers are found, a message is displayed.
This code serves as a practical example of user input validation, iterative calculation, and conditional logic in C#. It enables the user to determine the sum and average of even numbers within a given range, contributing to a foundational understanding of control flow and mathematical computation in programming.
Output
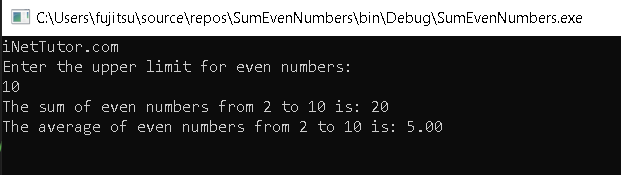
Summary
In this C# console programming lesson, we delved into the fundamental concepts of user input validation, iterative calculation, and conditional logic through the lens of calculating the sum and average of even numbers within a specified range. Beginning with the initialization of sum and average variables, the program seamlessly navigated through the intricacies of user interaction, employing a while loop for input validation to ensure the user provides meaningful and valid data.
The subsequent for loop efficiently computed the sum and count of even numbers within the user-defined range, demonstrating the elegance of iterative calculations. The program also showcased the optional calculation of the average, expanding the understanding of mathematical operations in programming.
Moreover, this lesson underscored the significance of user-friendly interfaces, employing concise error messages to guide users towards valid inputs. The interplay between user input, loops, and mathematical computations illuminated core programming principles essential for building robust and interactive applications.
By mastering these concepts, learners not only gained proficiency in developing a program that solves a specific problem but also acquired transferable skills applicable to a broader spectrum of programming challenges. This lesson serves as a stepping stone for individuals aspiring to build a strong foundation in C# programming, providing valuable insights into the realms of control flow, mathematical operations, and user-centric design.
Exercises
- Odd Numbers Calculation:
- Modify the program to calculate the sum and average of odd numbers within the specified range. Adjust the loop accordingly.
- Enhanced User Interaction:
- Improve the user interface by adding instructions, clarifying messages, or providing additional information during the input and output phases.
- Dynamic Upper Limit:
- Allow the user to decide whether to include or exclude the upper limit in the calculation. Implement the necessary adjustments in the loop logic.
- Input Validation Enhancement:
- Implement more robust input validation. Ensure that the program handles a broader range of user inputs gracefully, providing clear feedback in case of errors.
- Multiple Range Calculation:
- Extend the program to allow users to calculate the sum and average for multiple ranges in a single run. Provide a mechanism to input and process multiple range calculations.
Assessment:
- Code Review:
- Conduct a code review session, focusing on the clarity, readability, and structure of the code. Discuss potential improvements and optimizations.
- Error Handling Assessment:
- Purposefully introduce errors in user input and evaluate how well the program handles and communicates these errors to the user. Provide feedback on enhancing error-handling mechanisms.
- Efficiency Analysis:
- Evaluate the efficiency of the program, especially regarding the calculation of the sum and average. Discuss potential optimizations and improvements in terms of algorithmic efficiency.
- User Experience Evaluation:
- Assess the overall user experience by gathering feedback on the program’s interface, instructions, and clarity of output. Use this feedback to enhance user interaction.
- Scenario-Based Assessment:
- Present learners with different scenarios and ask them to adapt the program to meet specific requirements. This could include varying the data types, adjusting the range, or introducing additional calculations.
These exercises and assessments aim to challenge learners to extend and refine the existing project, reinforcing their understanding of key concepts while encouraging creativity and problem-solving skills.
Related Topics and Articles:
Factorial Calculator in CSharp
Sum of Array Elements in CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.