Factorial Calculator in CSharp
Overall Theme: Learn how to build a powerful C# console application that calculates factorials of non-negative integers.
Target Audience: Beginner to intermediate C# programmers interested in practical applications and understanding mathematical concepts through code.
Introduction
Table of Contents
- Brief Overview of the Importance of Factorial Calculations in Mathematics and Programming:
Factorial calculations, a fundamental concept in both mathematics and programming, play a pivotal role in various fields. In mathematics, the factorial of a non-negative integer represents the product of all positive integers up to that number. This mathematical operation finds application in combinatorics, probability theory, and permutation calculations. In the realm of programming, factorial calculations offer a versatile tool for solving complex problems, facilitating iterative solutions, and forming the basis for algorithmic designs. Understanding factorials is not merely an academic exercise; it serves as a cornerstone for building computational logic and problem-solving skills.
- Introduction to the Specific Topic: Building a Factorial Calculator using C#:
In this exploration, we delve into the practical implementation of factorial calculations through the lens of C# programming. Our focus is on constructing a Factorial Calculator – a program that efficiently computes the factorial of a given non-negative integer. This hands-on journey will guide learners through the essential steps of creating a functional C# console application. By the end of this lesson, not only will readers grasp the intricacies of factorial calculations, but they will also acquire practical skills in coding and problem-solving using C#.
- Relevance of Factorial Calculations in Real-World Scenarios:
Beyond the realms of mathematics and programming, the relevance of factorial calculations extends into real-world scenarios. In fields such as statistics, where permutations and combinations play a crucial role, understanding factorials is vital for accurate data analysis. Factorial calculations also find application in optimization problems, where the arrangement of elements impacts efficiency. By exploring the Factorial Calculator in C#, learners not only gain programming prowess but also unlock a tool applicable to a spectrum of real-world challenges. This journey transcends code, offering a bridge between abstract mathematical concepts and tangible problem-solving in the world around us.
Objectives
Upon completing this lesson and reading the associated blog post, learners will achieve the following objectives:
- Understand the Core Functionalities of a Factorial Calculator:
- Grasp the fundamental concept of factorial calculations and its significance in mathematical and programming contexts.
- Comprehend the iterative logic behind calculating factorials and the importance of base cases (0! and 1!).
- Implement Mathematical Logic in C# Code:
- Translate theoretical knowledge into practical coding skills by implementing a Factorial Calculator in C#.
- Gain proficiency in structuring C# code to execute complex mathematical operations with clarity and efficiency.
- Employ Basic User Input and Output Techniques:
- Acquire hands-on experience in prompting users for input within a console application.
- Learn to display output effectively, ensuring a user-friendly experience when interacting with the Factorial Calculator.
- Handle Errors and Unexpected Situations:
- Develop the ability to implement robust error-handling mechanisms within C# code.
- Learn strategies to validate user input, providing clear and informative error messages in case of invalid inputs.
By achieving these objectives, learners will not only master the technical aspects of building a Factorial Calculator but will also cultivate foundational programming skills applicable to a broader range of scenarios. This study aims to empower learners with the ability to bridge mathematical concepts with practical coding implementations using the C# programming language.
Source code example
using System; namespace FactorialCalculator { public class Program { private const int MaxNumber = 31; // This constant defines the maximum supported number for factorial calculation public static int CalculateFactorial(int number) { if (number < 0 || number > MaxNumber) { throw new ArgumentOutOfRangeException("number", $"Number must be between 0 and {MaxNumber}."); } int factorial = 1; for (int i = 2; i <= number; i++) { factorial *= i; } return factorial; } static void Main(string[] args) { Console.WriteLine("Welcome to the Factorial Calculator!"); Console.WriteLine($"This program can calculate factorials for numbers between 0 and {MaxNumber}."); while (true) { Console.WriteLine("Enter a non-negative number: "); string input = Console.ReadLine(); int number; if (!int.TryParse(input, out number)) { Console.WriteLine("Invalid input. Please enter a number."); continue; } try { int factorial = CalculateFactorial(number); Console.WriteLine($"The factorial of {number} is: {factorial}"); } catch (ArgumentOutOfRangeException ex) { Console.WriteLine(ex.Message); } Console.WriteLine("Do you want to calculate another factorial? (y/n)"); string answer = Console.ReadLine(); if (answer.ToLower() != "y") { break; } } Console.WriteLine("Thank you for using the Factorial Calculator. Press any key to close the console"); Console.ReadKey(); } } }
Explanation
- Namespace, Class Declaration, and Constant Definition (Lines 1-7):
The code is encapsulated within the FactorialCalculator namespace, offering a logical grouping for related code elements. Within this namespace, the Program class is declared as public, serving as the main entry point for the application. A constant named MaxNumber is defined with a value of 31, representing the maximum supported number for factorial calculation.
- Factorial Calculation Method (Lines 8-21):
The CalculateFactorial method is created to compute the factorial of a given number. It takes an integer parameter (number) and returns its factorial. The method includes input validation to ensure the provided number is within the valid range (0 to MaxNumber). The factorial is calculated using a simple iterative loop, and the result is returned.
- Main Method and User Interaction (Lines 22-59):
The Main method is the entry point of the program, orchestrating user interactions and the overall flow. It begins with a welcome message and information about the program’s capabilities. A continuous loop (while (true)) is initiated for repeated interactions until the user decides to exit.
- User Input and Validation (Lines 29-37):
Within the loop, the program prompts the user to input a non-negative number. It validates the input, and if the input is not a valid integer, the user is prompted to re-enter.
- Factorial Calculation and Output Display (Lines 39-47):
The program attempts to calculate the factorial using the CalculateFactorial method. If successful, it displays the result. If the input is outside the valid range, it catches an ArgumentOutOfRangeException and displays an error message.
- User Interaction to Continue or Exit (Lines 49-54):
The user is asked if they want to calculate another factorial. If the user’s response is not “y,” the loop is exited, and the program concludes.
- Closing Message (Lines 57-58):
As the program wraps up, a closing message is displayed, thanking the user for using the Factorial Calculator. The program then waits for the user to press any key before closing the console window, ensuring a deliberate and controlled exit. This design enhances the user experience and provides an opportunity to review the calculated factorials.
Output
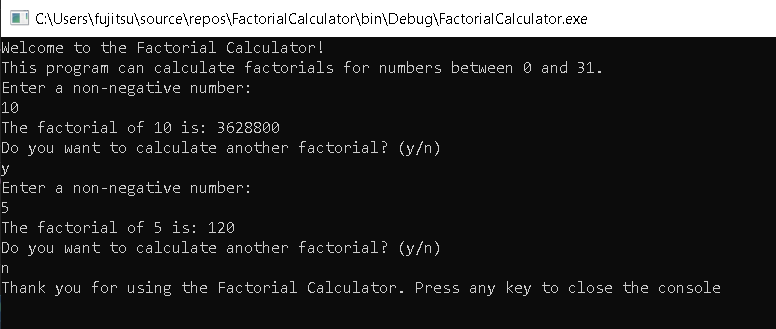
Summary
In this lesson, we explored into the development of a Factorial Calculator using C# console application. The program showcases fundamental concepts of C# programming, including user input validation, iterative calculations, and structured error handling. Through the implementation of the CalculateFactorial method, learners gained insight into the logic behind computing factorials, while the continuous loop in the Main method allowed for repeated interactions. The inclusion of a closing message and the utilization of Console.ReadKey() provided a user-friendly conclusion to the program, offering users the chance to review calculated factorials before closing the console window. This lesson serves as a practical illustration of basic C# programming principles, emphasizing user engagement, input validation, and structured program flow.
Exercises and Assessment
Objective: Build upon the existing Factorial Calculator project to deepen your understanding of C# console applications.
- Error Handling Extension:
- Enhance error handling in the CalculateFactorial method to provide more specific error messages for different scenarios, such as negative numbers or values exceeding the maximum supported limit. Consider using custom exceptions for better clarity.
- Factorial Range Extension:
- Modify the program to calculate and display factorials for a broader range of numbers. Adjust the MaxNumber constant accordingly, and ensure the program handles larger factorials efficiently.
- Input Modification:
- Modify the user input process to accept decimal numbers. Implement logic to handle non-integer inputs gracefully, allowing users to input decimal numbers and displaying an appropriate message.
Assessment: Code Review and Enhancement
Objective: Assess and enhance the Factorial Calculator project based on the exercise tasks.
- Code Review:
- Review the existing codebase to ensure adherence to coding standards, clarity, and consistency.
- Check the effectiveness of error handling and assess how well potential issues are communicated to the user.
- Exercise Implementation:
- Implement the suggested exercises, ensuring that the Factorial Calculator now handles a broader range of numbers and provides improved error messages.
- Verify that the code modifications maintain or improve the overall readability and maintainability of the program.
- Testing:
- Conduct thorough testing with various inputs, including positive, negative, and decimal numbers, to ensure the program behaves as expected.
- Verify that the extended error handling provides meaningful messages for different scenarios.
- Documentation:
- Update code comments and provide documentation for the changes made during the exercise. Clearly explain the purpose and functionality of any new code segments.
- User Experience Enhancement:
- Explore opportunities to enhance the user experience further. This could include additional prompts, clearer instructions, or any other improvements that contribute to a more user-friendly interface.
Completing this exercise and assessment will not only reinforce your understanding of the existing project but also challenge you to extend its functionality and enhance the overall quality of the Factorial Calculator.
Related Topics and Articles:
Sum of Array Elements in CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.