How to Print Custom Multiplication Tables in C#
Introduction
Table of Contents
Multiplication tables are fundamental mathematical tools that help us understand the relationship between numbers and perform quick calculations. They provide a structured representation of multiplication facts, allowing us to easily determine the product of two numbers.
In this lesson, we will explore the concept of multiplication tables and their significance in various mathematical operations. Our objective is to create a C# program that generates custom multiplication tables based on user input. By the end of this lesson, you will have the knowledge and skills to develop a program that can generate multiplication tables tailored to specific needs.
To achieve this, we will discuss the approach to generating multiplication tables programmatically. We will prompt the user for input, allowing them to specify the base number and the desired range of multiplication. Using this input, our program will calculate and display the custom multiplication table. We will also explore different formatting techniques to enhance the readability and presentation of the table.
By the end of this lesson, you’ll have a solid understanding of how to develop programs that manipulate and display multiplication tables in C#.
Objectives
In this lesson, our main focus is on helping you develop a comprehensive understanding of custom multiplication tables in C#. By the end of this lesson, you will not only learn the necessary concepts but also have ample opportunities to practice and apply your knowledge. Our objectives for this lesson are as follows:
- Understand:
- Gain a thorough understanding of the concept of custom multiplication tables.
- Comprehend the significance and practical applications of custom multiplication tables in programming.
- Learn:
- Learn how to prompt the user for input to determine the base number and range for the custom multiplication table.
- Acquire knowledge on how to calculate the table using loops and conditional statements.
- Explore different formatting techniques to enhance the readability and presentation of the multiplication table.
- Practice:
- Engage in hands-on exercises and coding challenges to reinforce your understanding of the concepts covered.
- Practice writing C# code to generate custom multiplication tables for various scenarios.
- Develop problem-solving skills by tackling different multiplication table requirements and scenarios.
- Apply:
- Apply the knowledge gained to create a fully functional C# program that generates custom multiplication tables.
- Extend your learning by adding additional features, such as highlighting prime numbers or customizing the table’s appearance.
- Utilize your newfound skills to solve real-world programming challenges that involve custom multiplication tables.
By focusing on these objectives, you’ll transform from a passive learner to an active programmer, capable of building a valuable tool and extending your C# skillset.
Source code example
using System; namespace CustomMultiplicationTable { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - Multiplication Table"); Console.WriteLine("Enter the number for the multiplication table:"); int number = Convert.ToInt32(Console.ReadLine()); Console.WriteLine($"Multiplication table for {number}:"); for (int i = 1; i <= 10; i++) { int result = number * i; Console.WriteLine($"{number} * {i} = {result}"); } Console.ReadLine(); } } }
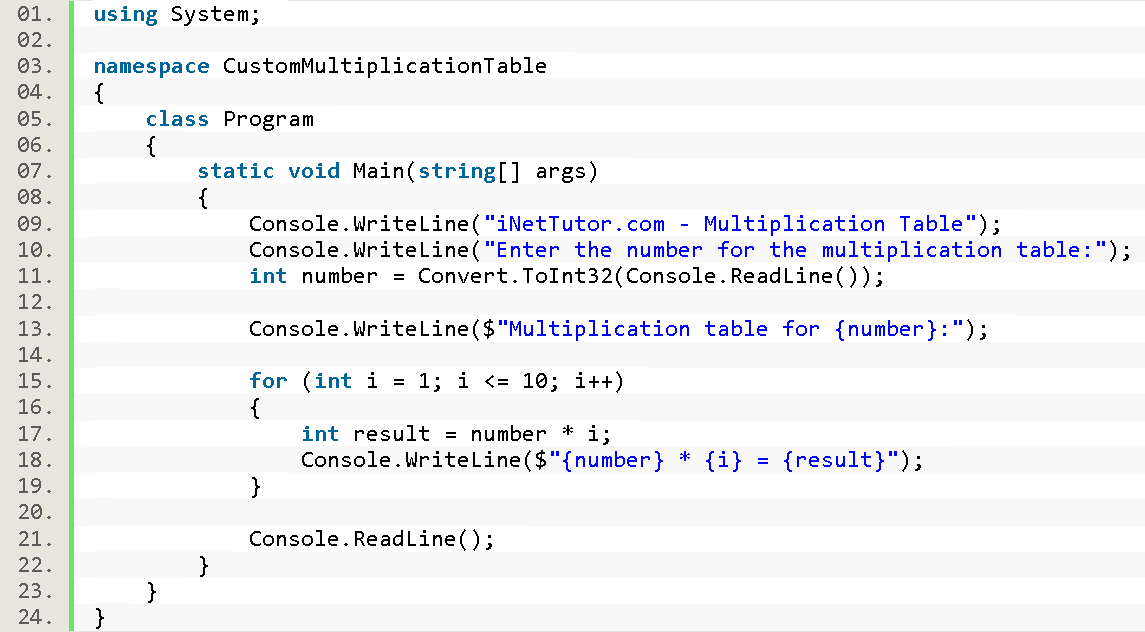
Explanation
The provided source code is a beginner-friendly program that generates a multiplication table based on user input. Here’s an explanation of the code:
- Namespaces and Class:
- using System;: Imports the System namespace, providing essential functionalities like input/output.
- namespace CustomMultiplicationTable: Defines a custom namespace to organize the code.
- class Program: Declares a class named Program that holds the program logic.
- Main Method:
- static void Main(string[] args): The program’s entry point where execution begins.
- User Interaction:
- Console.WriteLine(“iNetTutor.com – Multiplication Table”);: Prints a welcome message.
- Console.WriteLine(“Enter the number for the multiplication table:”);: Prompts the user for a number.
- int number = Convert.ToInt32(Console.ReadLine());: Reads the user’s input as a string, converts it to an integer, and stores it in the number variable.
- Table Information:
- Console.WriteLine($”Multiplication table for {number}:”);: Informs the user about the table for the chosen number.
- Loop for Calculation and Printing:
- for (int i = 1; i <= 10; i++): This for loop iterates 10 times, representing the multiples from 1 to 10.
- int i = 1;: Initializes a loop counter i starting from 1.
- i <= 10: The loop continues as long as i is less than or equal to 10.
- i++: Increments the counter by 1 in each iteration.
- int result = number * i;: Inside the loop, calculates the product of number and i (the multiple), storing it in the result variable.
- Console.WriteLine($”{number} * {i} = {result}”);: Prints the multiplication result in a formatted way, displaying the number, multiple, and product.
- Pausing the Console:
- Console.ReadLine();: Pauses the console window until the user presses any key.
This simple program allows beginners to understand the basic structure of a C# program, the use of loops, and the calculation of multiplication results. It provides a starting point for further exploration and enhancement of the multiplication table generation functionality.
Output
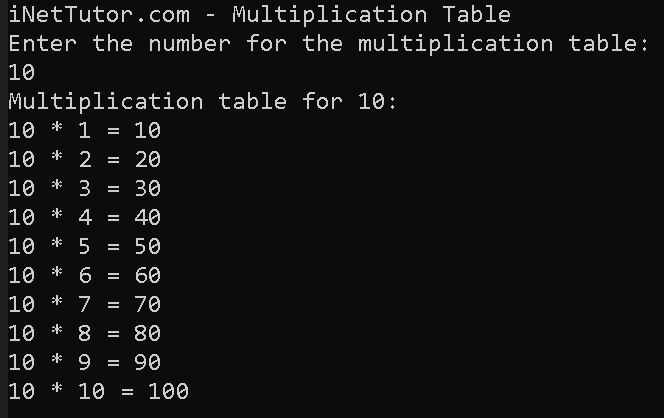
Summary
In this lesson, we explored the concept of custom multiplication tables in C#. We learned how to prompt the user for input to determine the base number and range for the table. Using loops and conditional statements, we calculated and displayed the custom multiplication table. We also discussed formatting techniques to enhance the readability and presentation of the table. Through hands-on practice and coding exercises, we gained a solid understanding of creating custom multiplication tables in C#.
Key Points:
- Custom multiplication tables are a fundamental mathematical tool that helps us understand the relationship between numbers and perform quick calculations.
- In C#, we can create a program that generates custom multiplication tables based on user input.
- Prompting the user for input allows us to determine the base number and range for the custom multiplication table.
- Using loops, such as the for loop, we can calculate the multiplication results for each number within the specified range.
- By applying formatting techniques, such as string interpolation, we can enhance the readability and presentation of the multiplication table.
- Practice and hands-on coding exercises are essential for reinforcing our understanding of custom multiplication tables in C#.
By mastering the concepts covered in this lesson, you now have the knowledge and skills to create custom multiplication tables in C#. This foundational understanding can be expanded upon to tackle more complex programming challenges and explore additional features, such as highlighting prime numbers or customizing the table’s appearance. Keep practicing and applying your knowledge to sharpen your C# programming skills!
Exercises and Assessment
This exercise provided a foundational understanding of essential C# concepts like user input, loops, calculations, and formatted output. By building this program, you’ve gained valuable practical experience and can now explore further customization options:
- Modify the loop range: Change the loop to print multiples beyond 10 or within a specific user-defined range.
- Enhance table formatting: Implement spacing or alignment techniques to create a visually appealing table.
- Error handling: Introduce input validation to ensure the user enters a valid number.
Remember, practice is key to mastering C#. Experiment and explore further to solidify your understanding and unlock the potential of C# programming!
Quiz
1. Knowledge (Remembering):
What does the following line of code achieve in the program?
int number = Convert.ToInt32(Console.ReadLine());
a) Prints a message to the user.
b) Reads the user’s input as a string.
c) Converts the user’s input from a string to an integer.
d) Calculates the product of the chosen number and its multiples.
2. Comprehension (Understanding):
Why does the for loop in the program utilize the condition i <= 10?
a) To ensure the loop iterates exactly 100 times.
b) To calculate the sum of all the multiples.
c) To repeat the loop as long as the counter i is less than or equal to 10 (representing the multiples 1 to 10).
d) To validate the user’s input for the number.
3. Application (Applying):
How can you modify the code to print only even multiples (2, 4, 6, etc.) within the existing range of 1 to 10?
a) Remove the for loop entirely.
b) Change the loop condition to i < 10.
c) Introduce an if statement inside the loop to check if the current multiple i is even.
d) Modify the loop increment to i += 3.
4. Analysis (Evaluating):
What potential issue could arise if the user enters a non-numeric value during input (e.g., “hello”)?
a) The program will automatically handle the error and continue.
b) The loop might continue indefinitely, printing unexpected results.
c) The program will display an error message, but the code might crash unexpectedly.
d) The code might throw an exception due to the failed conversion from string to integer.
5. Synthesis (Creating):
Imagine you want to enhance the program to calculate the sum of all the printed multiples. How would you achieve this?
a) Modify the existing for loop to directly calculate the sum within the loop.
b) Introduce a new variable outside the loop to store the sum and update it within the loop using addition.
c) Add an if statement outside the loop to check for even multiples and calculate their sum separately.
d) Utilize string manipulation techniques on the printed multiples to extract the numbers and calculate the sum.
Related Topics and Articles:
C# For Loop Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.