Splitting a String by Whitespace in C#
Introduction of the lesson
Table of Contents
In the world of C#, strings are the workhorses for handling textual information. Imagine a recipe with various ingredients listed one after another. In C#, strings act like digital containers that store these “ingredients” – words, sentences, or any combination of characters. But what if you need to separate these ingredients, analyze them individually, or rearrange them? That’s where string splitting comes to the rescue!
String splitting allows you to break down a single string into an array of smaller strings, typically based on spaces (whitespace) between words. This seemingly simple technique unlocks a vast array of possibilities. From processing user input containing multiple words to analyzing text data for sentiment analysis, splitting strings is a fundamental skill for working with textual information in C#.
C# offers multiple methods for splitting strings, providing flexibility based on your needs. The most common approach utilizes the Split method available on string objects. This method allows you to specify the separator (whitespace by default) and returns an array of substrings obtained by splitting the original string at those separator points. We’ll delve deeper into the syntax and usage of this method throughout this lesson.
Objectives of the lesson
In this lesson, the objectives are centered around understanding, learning, practicing, and applying the techniques for splitting strings in C#.
- Understand
- Gain a comprehensive understanding of the concept of splitting strings in C#.
- Grasp the significance of string splitting in data processing and manipulation.
- Learn
- Learn to utilize the built-in Split method in C# for string splitting.
- Understand the syntax, parameters, and usage of the Split method.
- Acquire knowledge of implementing custom logic for specialized string splitting requirements.
- Practice
- Engage in practical exercises to reinforce learning and proficiency in string splitting techniques.
- Practice using the Split method and custom splitting logic with various examples and scenarios.
- Apply
- Apply the acquired knowledge and skills to real-world scenarios and practical applications.
- Explore the use of split strings in data analysis, text processing, and user input handling.
By accomplishing these objectives, learners will be well-equipped to understand the concept of string splitting in C#, master the relevant techniques, practice their application through hands-on exercises, and ultimately apply these skills to real-world programming tasks and challenges.
Source code example
using System; namespace SplitStringExample { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - String Splitting Example"); Console.WriteLine("Enter a sentence:"); string sentence = Console.ReadLine(); // Read user input // Split the sentence using the Split() method (default separator is whitespace) string[] words = sentence.Split(); Console.WriteLine("Original Sentence:"); Console.WriteLine(sentence); // Print the original sentence Console.WriteLine("\nWords after Splitting:"); // Access and print each word in the resulting array using a loop for (int i = 0; i < words.Length; i++) { Console.WriteLine(words[i]); } Console.ReadKey(); } } }
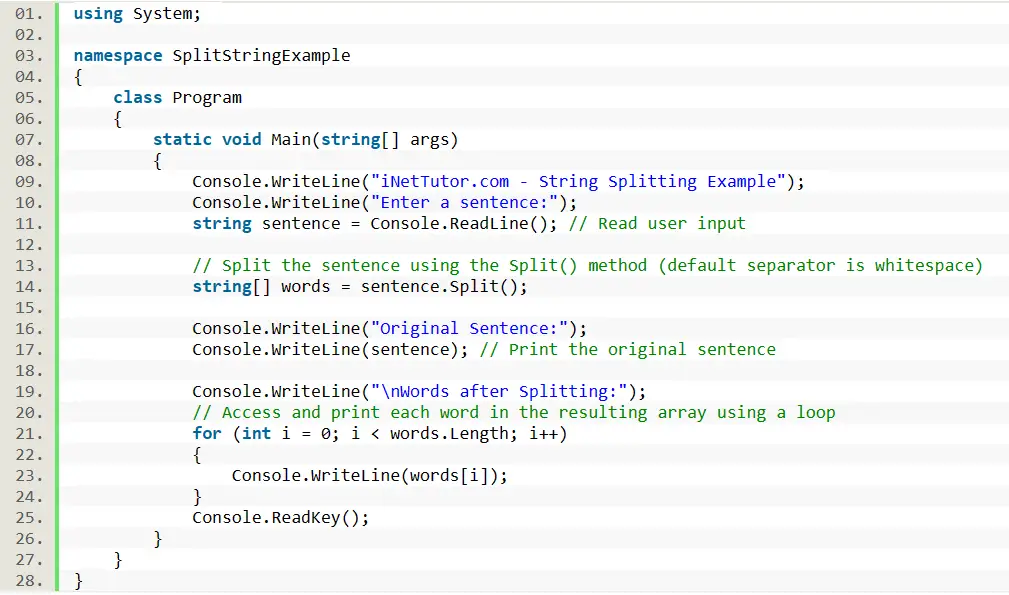
Source code explanation
Explanation of the SplitStringExample Code with User Input:
- Namespaces and Class:
- using System;: Imports the System namespace, providing essential functionalities like string manipulation and console input/output.
- namespace SplitStringExample { … }: This block defines a namespace named SplitStringExample. Namespaces help organize code and prevent naming conflicts between projects. Everything within this block belongs to the SplitStringExample namespace.
- Class and Method:
- class Program { … }: This defines a class named Program. Classes are blueprints for creating objects and encapsulate methods (functions) that perform specific tasks.
- Main Method:
- static void Main(string[] args): This is the program’s entry point, where execution begins. The static keyword indicates this method belongs to the class itself, not any specific object created from the class. void means the method doesn’t return any value. string[] args represents potential command-line arguments passed to the program (not used in this example).
- User Input for Sentence:
- Console.WriteLine(“Enter a sentence:”);: Prompts the user to enter a sentence using Console.WriteLine.
- string sentence = Console.ReadLine();: Reads the user’s input as a string using Console.ReadLine and stores it in the sentence variable. This allows the program to work with a sentence provided by the user rather than a pre-defined one.
- Splitting the String:
- string[] words = sentence.Split();: This line performs the core operation of string splitting.
- sentence.Split(): Calls the Split method on the sentence string object.
- By default, Split separates words based on whitespace characters (spaces, tabs, newlines) in the original string.
- The result, an array of individual words, is stored in the words variable.
- Printing Original Sentence:
- Console.WriteLine(“\nOriginal Sentence:”);: Prints a message indicating the original sentence.
- Console.WriteLine(sentence);: Prints the original un-split sentence stored in the sentence variable, which now contains the user’s input.
- Looping through Words:
- Console.WriteLine(“\nWords after Splitting:”);: Prints a message indicating the split words.
- for (int i = 0; i < words.Length; i++) { … }: This for loop iterates through the words array, which contains the individual words after splitting.
- int i = 0;: Initializes a loop counter i starting at 0 (index of the first word in the array).
- i < words.Length: The loop continues as long as i is less than the Length property of the words array. Length represents the number of elements (words) in the array.
- i++: Increments the counter for the next iteration.
- Console.WriteLine(words[i]);: Inside the loop, each word is accessed using its index i within the array and printed to the console using Console.WriteLine.
Key Points:
- This code demonstrates user interaction through Console.WriteLine for prompts and Console.ReadLine for capturing user input.
- The program takes the user’s sentence, splits it by whitespace, and prints each word individually.
- Comments are included to enhance readability for beginners.
- The code utilizes basic C# syntax like variables, strings, arrays, loops, and console input/output methods.
Output
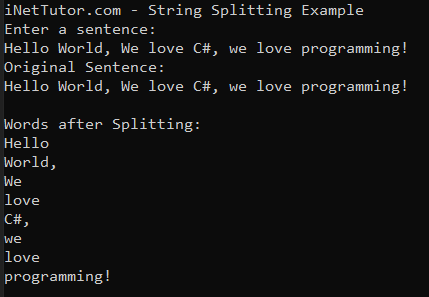
Summary and Conclusion
In this lesson, we explored the concept of splitting strings by whitespace in C#. Strings are essential for storing and manipulating text data in programming. We learned that splitting strings involves separating words or phrases based on spaces or other delimiters. We discussed various methods available in C# for splitting strings, highlighting the Split() method as a common and straightforward approach.
Key Points:
- Strings are fundamental for text processing in programming.
- Splitting strings involves breaking them into substrings based on specified delimiters.
- The Split() method in C# is used to split strings by whitespace by default.
- Other delimiters can also be specified to split strings based on different criteria.
- Splitting strings is useful for tasks such as tokenization, data processing, and text analysis.
Conclusion: Understanding how to split strings by whitespace is crucial for various text processing tasks in C#. By mastering this concept, programmers can efficiently parse and analyze textual data, enabling them to build robust and effective applications. Through this lesson, learners have gained valuable insights into string manipulation techniques, empowering them to tackle real-world programming challenges more effectively.
Exercises
Exercises to Enhance the SplitStringExample Code:
- Custom Separators: Modify the code to allow users to specify a custom separator (e.g., comma, hyphen) for splitting their sentence.
- Prompt the user to enter the desired separator before reading the sentence.
- Update the Split method call to include the user-provided separator as an argument (e.g., sentence.Split(separatorChar))
- Handling Empty Inputs:
- Implement code to check if the user enters an empty string (no sentence).
- Display an informative message prompting the user to enter a valid sentence if the input is empty.
- Counting Words: Extend the program to count the total number of words in the original sentence after splitting.
- Utilize a counter variable within the loop that iterates through the words array and increments for each word encountered.
- Print the total word count after the loop execution.
Lab Exam Ideas:
- Text Analyzer: Challenge students to create a program that reads a paragraph of text from the user and performs:
- Splitting the text into sentences (using a period (“.”) as a separator).
- Counting the total number of words and sentences.
- Optionally, calculating the average number of words per sentence.
- This exam assesses their ability to handle more complex string manipulation tasks involving multiple separators and calculations.
- Word Frequency Counter:
- Design a program that reads a sentence from the user and creates a dictionary to store the frequency of each word (number of times each word appears).
- Utilize string splitting to obtain individual words.
- Implement logic to check for existing words in the dictionary and update their count or add new words with a count of 1.
- This exam tests their understanding of data structures (dictionaries) and their application in conjunction with string splitting techniques.
- Custom Delimiter Challenge: For more advanced students, create a program that allows users to enter a sentence and a delimiter (e.g., “|”).
- The program should split the sentence based on the provided delimiter and print each resulting substring (section between delimiters) on a separate line.
- This exam evaluates their ability to handle user input, custom separators, and iterating through the resulting substrings for individual processing.
Quiz
Instructions: Choose the best answer for each question.
1. What is the primary purpose of using the Split method on a string in C#?
a) To reverse the order of characters in the string.
b)To separate the string into individual substrings based on a specified separator.
c) To check if a string contains a specific word.
d) To convert a string to uppercase characters.
2. The following code snippet splits a sentence into words using the Split method:
string sentence = “This is a sentence with multiple words.”;
string[] words = sentence.Split();
What will be the value stored in the words[1] element of the array after this code executes?
a) An empty string (“”)
b) The entire original sentence
c) “is” (the second word)
d) The number of words in the sentence
3. Consider the following code:
string data = “Name,Age,City;John Doe,30,New York”;
string[] parts = data.Split(new char[] { ‘,’, ‘;’ });
What does this code achieve compared to using data.Split(‘,’) alone?
a) It splits the string only once, using a comma (“,”) as the separator.
b) It splits the string into empty elements because of the additional semicolon.
c) It splits the string considering both commas (“,”) and semicolons (“;”) as separators, resulting in more elements in the parts array.
d) It removes all commas and semicolons from the original string.
4. You are building a program that reads comma-separated data from a file. The data format might have leading or trailing spaces around some values. Which of the following approaches would be most efficient for processing this data after reading each line from the file?
a) Split the line using commas (“,”) as separators and then trim leading/trailing spaces from each element in the resulting array (potentially using a loop).
b) Use a regular expression to extract comma-separated values while simultaneously removing leading/trailing spaces.
c) Split the line using a combination of commas (“,”) and spaces (” “) as separators.
d) Split the line using commas (“,”) as separators and then utilize the Trim method on each element to remove leading/trailing spaces in a single pass.
5. Imagine you want to create a program that analyzes movie reviews. How could you leverage string splitting techniques to process a review and identify frequently used words (excluding punctuation marks)?
a) Split the review by whitespace characters, remove all punctuation from each word, and then use a dictionary to store word frequency.
b) Convert the entire review to lowercase and then split by whitespace, ignoring punctuation during the splitting process.
c) Split the review by whitespace characters, iterate through the resulting words, and check if each word contains any punctuation marks. If punctuation is found, remove it before storing the word (without punctuation) in a dictionary to track frequency.
d) Split the review by individual characters and create a custom data structure to categorize characters as words or punctuation.
Related Topics and Articles:
Remove Vowels in string using C#
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.