Online Water Billing System in PHP
Introduction
Table of Contents
Water billing systems play a crucial role in efficiently managing and regulating water usage in both residential and commercial settings. At their core, these systems are designed to accurately measure and calculate water consumption, generate billing statements, and facilitate the collection of payments from customers. By providing a structured framework for tracking usage and managing accounts, water billing systems help utilities ensure fair distribution of resources and maintain financial sustainability.
In the field of web development, PHP (Hypertext Preprocessor) stands out as a versatile and widely-used scripting language, particularly well-suited for building dynamic web applications. When it comes to developing a water billing system, adopting a procedural approach using PHP offers numerous advantages.
First and foremost, a procedural approach simplifies the development process by breaking down complex tasks into smaller, more manageable steps. This makes it easier for developers, especially those who are new to programming or PHP, to understand and work with the codebase effectively. Unlike more advanced programming paradigms like object-oriented programming (OOP), which can involve concepts such as inheritance and polymorphism, procedural PHP relies on straightforward, linear sequences of instructions, making it accessible to beginners.
Moreover, a procedural approach in PHP lends itself well to rapid prototyping and iterative development. By focusing on defining procedures or functions to carry out specific tasks, developers can quickly prototype different features of the water billing system and refine them as needed. This iterative process allows for faster feedback and iteration, ultimately leading to a more robust and user-friendly end product.
While a procedural approach might not be as scalable for very complex systems, it’s a great choice for building a foundational water billing system in PHP, particularly when starting out or focusing on clarity.
Additionally, the procedural nature of PHP lends itself to a more straightforward debugging and troubleshooting process. Since procedural code executes in a linear fashion, it is often easier to pinpoint and resolve errors or issues that may arise during development or testing. This can save valuable time and resources, particularly in the early stages of building and refining the water billing system.
Basics of Water Billing
Water billing systems manage the process of charging customers for their water usage. Here’s a breakdown of some key components:
- Meter Readings: This is the foundation of water billing. Meters track the volume of water used by a customer over a specific period, usually measured in cubic meters or gallons. Accurate meter readings are crucial for fair and accurate billing.
- Billing Periods: Water bills are typically generated at regular intervals, such as monthly, bi-monthly, or quarterly. This billing period determines the timeframe for which meter readings are used to calculate water usage.
- Water Rates: These are the set prices charged per unit of water used (e.g., per cubic meter). Rates can be tiered, meaning the price may increase as water consumption goes up, encouraging conservation.
Importance of Accuracy
Accurate calculations and record-keeping are vital in water billing systems. Errors can lead to:
- Overbilling: Customers being charged for more water than they used.
- Underbilling: Water providers losing revenue due to undercharged customers.
- Customer dissatisfaction: Inaccurate bills can cause frustration and disputes.
How PHP Streamlines Water Billing
Procedural programming in PHP offers a valuable tool for building water billing systems:
- Automated Calculations: PHP code can automate calculations based on meter readings, water rates, and billing periods, eliminating human error.
- Data Management: Databases can be used to store customer information, meter readings, and billing history. PHP can interact with these databases to manage and retrieve data efficiently.
- Report Generation: PHP scripts can generate detailed reports on water consumption, billing details, and revenue collection.
- Improved Efficiency: Automating these tasks frees up time and resources for water providers, allowing them to focus on other areas.
By leveraging PHP’s capabilities, water providers can create efficient and reliable billing systems that ensure accurate charges and satisfied customers.
Setting up the Development Environment
Setting up a development environment is the first step towards building a water billing system in PHP. Below are step-by-step instructions on setting up the environment using XAMPP, a popular tool for PHP development, and creating a new project folder with the necessary files and directories.
- Install XAMPP:
- Download the latest version of XAMPP from the official website (https://www.apachefriends.org/index.html).
- Follow the installation instructions for your operating system (Windows, macOS, or Linux).
- During the installation process, you’ll be prompted to select components to install. Ensure that PHP, Apache, MySQL, and phpMyAdmin are selected.
- Start Apache and MySQL:
- Once XAMPP is installed, launch the XAMPP Control Panel.
- Start the Apache and MySQL services by clicking on the “Start” buttons next to their respective names. This will start the web server and database server.
- Verify Installation:
- Open a web browser and navigate to http://localhost/. If XAMPP is installed correctly, you should see the XAMPP dashboard indicating that Apache and MySQL are running.
- Create a New Project Folder:
- Choose a location on your computer where you want to store your project files. For example, you can create a folder named “water_billing_system” in the “htdocs” directory inside the XAMPP installation directory.
- Navigate to the XAMPP installation directory (e.g., C:\xampp\htdocs) and create a new folder named “water_billing_system”.
- Set Up Necessary Files and Directories:
- Inside the “water_billing_system” folder, create the following directories:
- css: This directory will contain CSS files for styling your web application.
- js: This directory will contain JavaScript files for client-side scripting.
- img: This directory will contain images used in your web application.
- includes: This directory will contain PHP files that include reusable code, such as database connections or utility functions.
- vendor: This directory will be used if you’re planning to use external libraries or dependencies.
- Create an index.php file in the “water_billing_system” folder. This will serve as the entry point for your application.
- Optionally, create additional PHP files for different components of your water billing system, such as user authentication, meter readings, billing calculations, etc.
- Inside the “water_billing_system” folder, create the following directories:
- Start Developing:
- You’re now ready to start developing your water billing system using PHP. Open your preferred code editor and begin writing PHP, HTML, CSS, and JavaScript code to build your application.
- Remember to include PHP tags (<?php ?>) in your PHP files to execute PHP code, and save your files with a .php extension.
By following these steps, you can set up a development environment for building a water billing system using PHP with XAMPP or a similar tool. This environment provides the necessary infrastructure to develop, test, and debug your PHP application locally before deploying it to a live server.
Features of the System
Our water billing system built with PHP will offer functionalities to manage various aspects of the billing process. Here’s a breakdown of some key features:
- Customer Management:
- Add Customers: Allow users (water provider staff) to add new customer accounts to the system. This might involve capturing details like name, address, account number, and meter ID.
- View Customer Information: Provide a way to view existing customer information, enabling staff to access and update details if necessary.
- Search Customers: Implement a search function to locate specific customer accounts based on name, account number, or other relevant criteria.
- Meter Readings:
- Record Meter Readings: This feature allows authorized personnel to submit meter readings for each customer during the billing period. The system should handle recording the date, reading value, and potentially any additional notes.
- View Meter Reading History: Enable users to view historical meter reading data for a specific customer, allowing for consumption trend analysis and potential leak detection.
- Bill Generation:
- Calculate Water Usage: Based on recorded meter readings and billing period, the system should calculate the total water consumption for each customer.
- Apply Water Rates: The system should incorporate the defined water rate structure (e.g., tiered pricing) to calculate the bill amount based on the customer’s water usage.
- Generate Bill Reports: The system should generate user-friendly bill reports in a format like PDF or HTML. These reports should detail water usage, applicable rates, breakdown of charges, and the total amount due.
- Payment Management (Optional):
- Record Payments: While the focus might be on generating bills initially, you could consider adding a basic payment recording feature. This allows users to mark received payments against specific customer accounts.
Additional Considerations:
- Security: The system should enforce access control mechanisms to ensure only authorized users can create, view, and modify data.
- Data Backup: Implement a regular data backup routine to protect valuable customer information and billing history.
- Reporting & Analytics: Consider incorporating features for generating reports on overall water usage, revenue collection, and customer trends. This can provide valuable insights for water providers.
By building these functionalities in a step-by-step manner, we can create a robust water billing system that streamlines billing processes and improves efficiency for water providers.
Screenshots
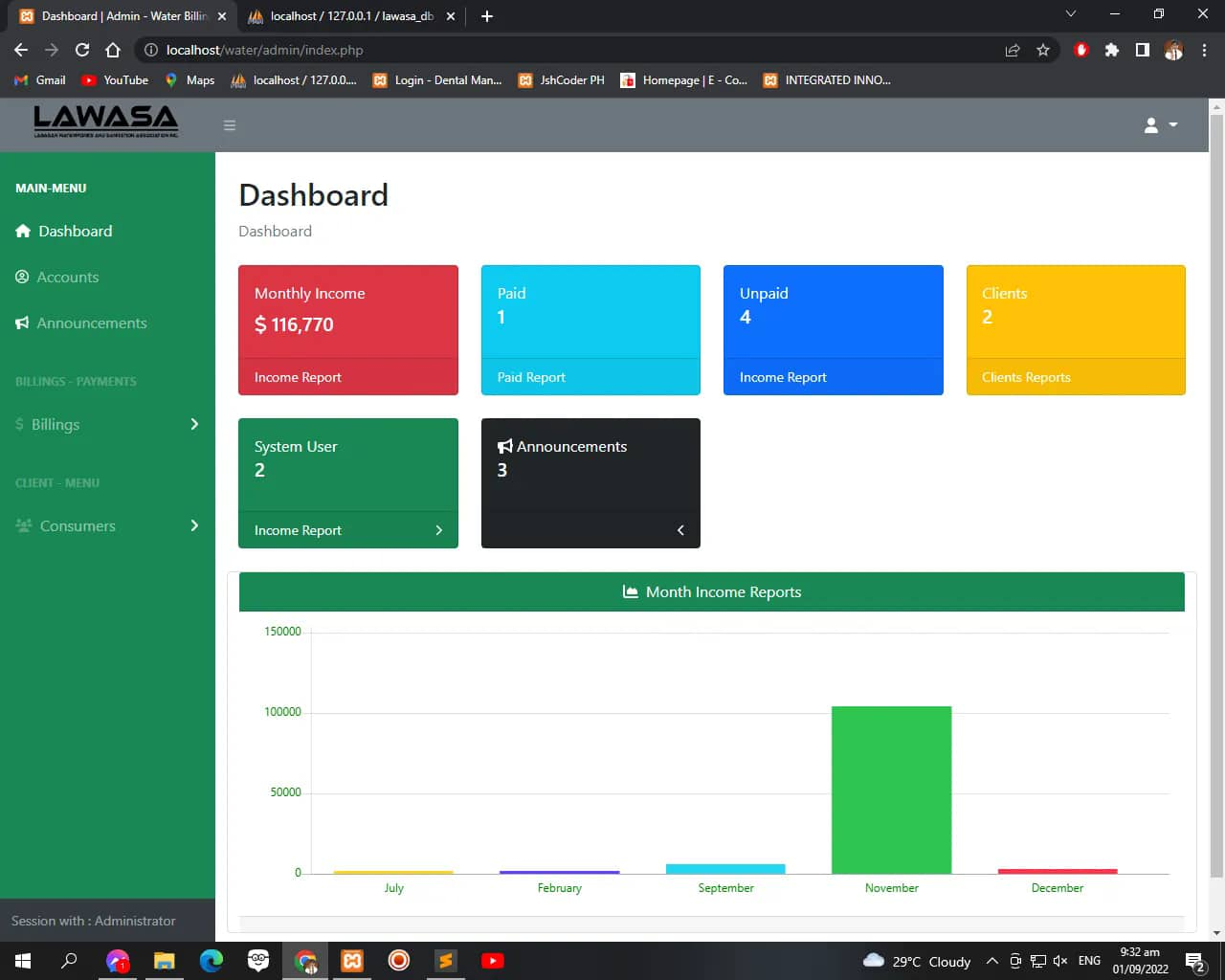
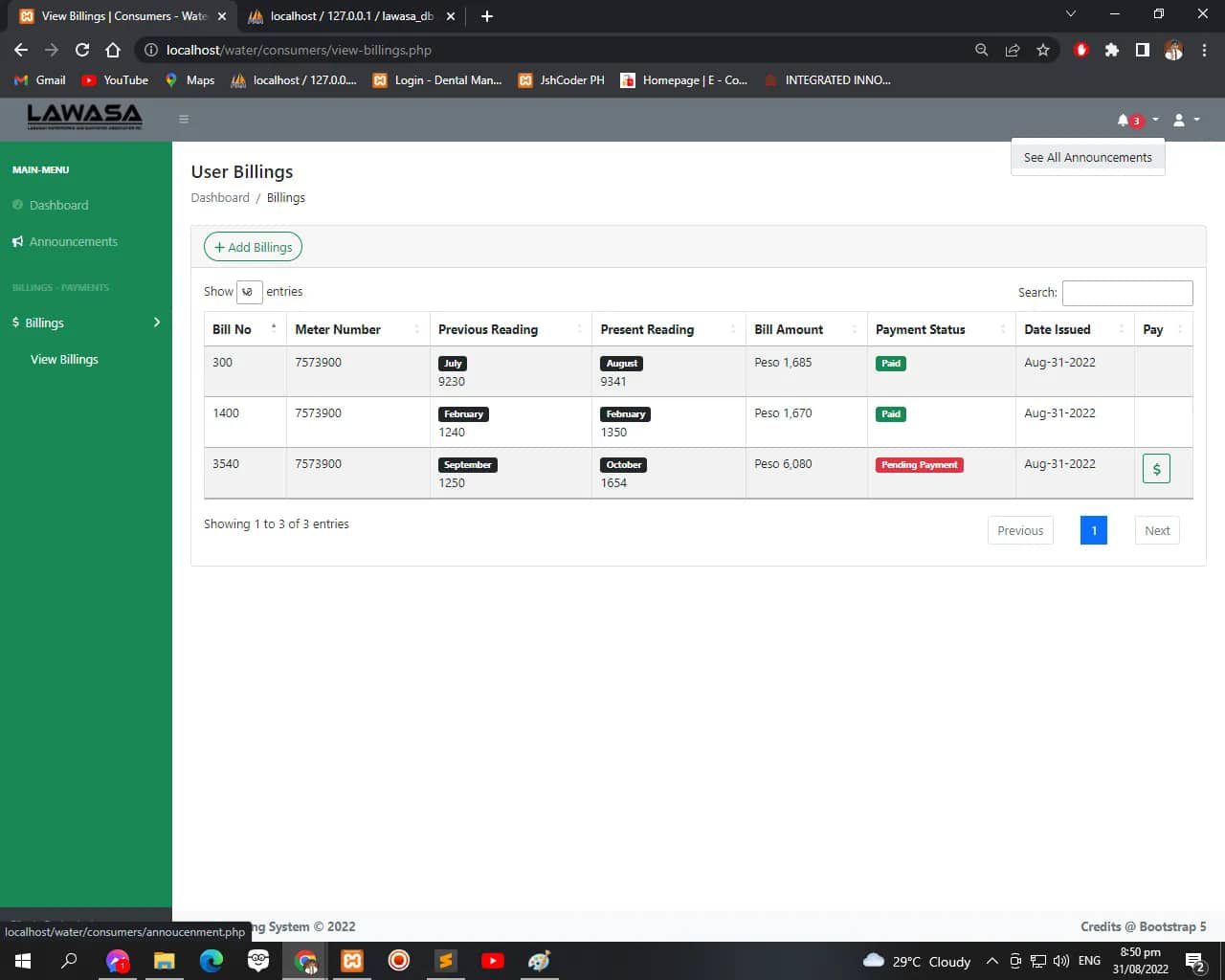
Summary
This blog post has explored the development of a water billing system using a procedural approach in PHP. Here’s a quick recap:
- Benefits of PHP: We discussed the advantages of using PHP for water billing applications. These include:
- Easier to understand code, especially for beginners.
- Modular design with reusable functions.
- Clear step-by-step logic for efficient development.
- Setting Up the Environment: We outlined the steps to set up your development environment using XAMPP, including creating project folders and ensuring the web server and database are running.
- System Functionalities: We explored the key functionalities the water billing system can offer, such as:
- Customer Management (adding, viewing, searching)
- Meter Reading Management (recording, viewing history)
- Bill Generation (calculating usage, applying rates, generating reports)
- Optional Payment Recording
- Additional Considerations: We briefly touched upon the importance of security, data backup, and potential reporting & analytics features.
Moving Forward:
By leveraging the capabilities of PHP, water providers can build efficient and user-friendly water billing systems. This can lead to improved accuracy, streamlined processes, and ultimately, happier customers.
This blog post serves as a starting point. You can extend this system by adding features like user authentication, email notifications for bills, or integration with online payment gateways. Feel free to explore further and adapt it to your specific needs.
Credits to the developer of the project:
Readers are also interested in:
Water Billing System Use Case Diagram
Water Billing System Database Project
Water Billing System Conceptual Framework
Online Water Billing System Free Source code in Bootstrap
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project