Vending Machine in C#
Introduction
Table of Contents
Have you ever wondered how vending machines dispense refreshing drinks and delicious snacks with just a press of a button? In this C# coding adventure, you’ll unlock the secrets behind this everyday marvel by building your very own virtual vending machine!
This step-by-step guide will equip you with the tools and knowledge to:
- Craft a tempting menu: Display an array of items with their corresponding prices, inviting users to indulge their cravings.
- Harness the power of choice: Prompt users to enter a selection code, and utilize the switch statement as your trusty decision-making tool.
- Calculate the cost: Put your math skills to the test by accurately calculating the total amount based on the chosen item’s price.
- Simulate the dispense: Get ready for the satisfying part! Simulate the dispensing of the chosen item, providing a virtual taste of success.
By the end of this journey, you’ll not only boast a functional C# program but also a deeper understanding of:
- User interaction: Learn how to effectively receive and process user input to guide the program’s flow.
- Switch statement mastery: Unleash the power and efficiency of the switch statement for making informed decisions based on multiple options.
- Problem-solving: Break down the vending machine logic into smaller, manageable steps and implement them using C# code.
Objectives
Our main objectives are for you to understand the underlying concepts, learn the necessary skills, practice and apply what you’ve learned, and ultimately enhance your application. By the end of this guide, you will have a solid foundation in building vending machine programs in C#, using switch statements to handle item selection and simulate the dispensing process.
Specifically, the lesson aims to:
- Understand: The primary objective of this tutorial is to help you understand the process of building a vending machine program in C#. You will gain a clear understanding of the concepts involved, such as menu display, user input, cost calculation, and the usage of switch statements for item selection and dispensing simulation.
- Learn: By following this step-by-step guide, you will learn the necessary skills and techniques to build a vending machine program from scratch. You will explore various aspects of C# programming, including handling user input, implementing logic for item selection, and simulating the dispensing process.
- Practice and Apply: Throughout the tutorial, you will have ample opportunities to practice your programming skills. By implementing the vending machine program, you will apply the concepts and techniques learned, reinforcing your understanding of C# programming, switch statements, and problem-solving.
- Enhance Your Application: By the end of this tutorial, you will have a functional vending machine program that you can further enhance and customize. You will have the knowledge and confidence to extend the program’s capabilities, such as adding new items, implementing different pricing strategies, or integrating payment systems.
Source code example
using System; namespace VendingMachine { class Program { static void Main() { Console.WriteLine("iNetTutor.com - Vending Machine"); // Display menu Console.WriteLine("\nMenu:"); Console.WriteLine("1. Snickers - $1.50"); Console.WriteLine("2. Coke - $1.00"); Console.WriteLine("3. Chips - $0.75"); // Prompt user for selection Console.Write("\nEnter your selection code (1-3): "); int selectionCode = int.Parse(Console.ReadLine()); // Initialize variables string itemName = ""; double itemPrice = 0.0; // Use switch statement to determine chosen item switch (selectionCode) { case 1: itemName = "Snickers"; itemPrice = 1.50; break; case 2: itemName = "Coke"; itemPrice = 1.00; break; case 3: itemName = "Chips"; itemPrice = 0.75; break; default: Console.WriteLine("Invalid selection. Please try again."); break; } // Display result if (itemPrice > 0.0) { Console.WriteLine($"You selected: {itemName}"); Console.WriteLine($"Cost: ${itemPrice:F2}"); Console.WriteLine("Dispensing... Enjoy!"); } Console.ReadLine(); } } }
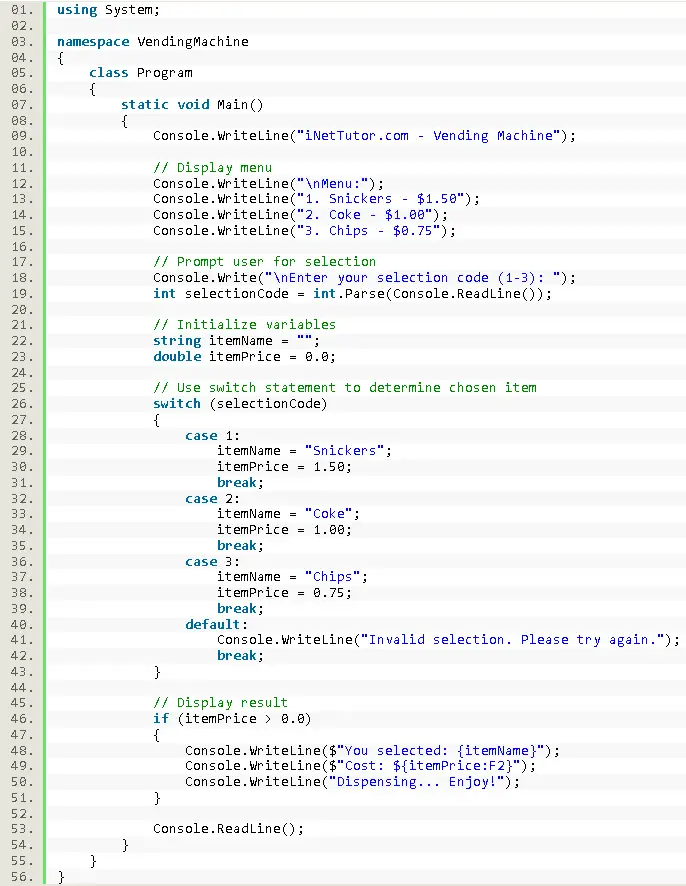
Explanation
The provided code is a C# program that simulates a simple vending machine. Here’s an explanation of the code:
- The program starts by displaying a welcome message and the menu of items with their corresponding prices.
- The user is prompted to enter a selection code (1-3) for the desired item.
- The program uses a switch statement to determine the chosen item based on the selection code. Each case represents a different item and assigns the item name and price to variables.
- If the selection code is invalid (not 1, 2, or 3), an error message is displayed.
- If the selection code is valid, the program displays the selected item’s name and price, along with a message simulating the dispensing process.
- The program then waits for the user to press the Enter key before exiting.
This program demonstrates the usage of switch statements to handle different cases based on user input and simulate the behavior of a vending machine. You can further enhance the program by adding additional functionality, such as handling payment, maintaining inventory, or allowing multiple selections.
Please note that the code provided is a basic example and may not include error handling or additional features.
Output
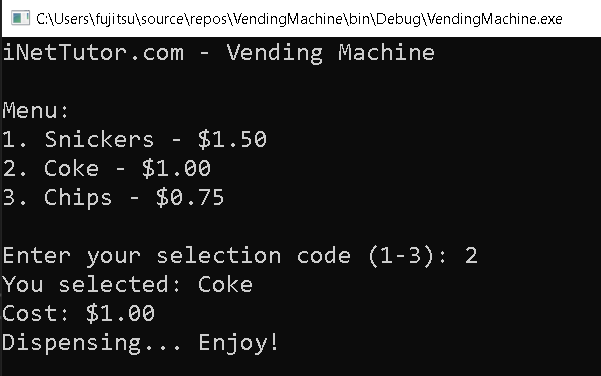
Summary
In this C# lesson, we explored the creation of a Vending Machine program using switch statements. The program simulates a vending machine experience, displaying a menu with item choices and their prices. Users enter a selection code, and the program utilizes a switch statement to determine the chosen item, calculate its cost, and simulate the dispensing process. This lesson demonstrated the effective use of switch statements for handling multiple options in a structured and readable manner, providing a practical example of their application in programming. Learners gained insights into menu creation, user input processing, and conditional execution through this hands-on exercise, enhancing their understanding of C# control flow structures.
Exercises and Assessment
Exercises and Lab Exam for Vending Machine Lesson Improvement:
Exercise 1: Vending Machine Program Enhancement
- Take the existing vending machine program and enhance it by adding the functionality to handle payment from the user.
- Prompt the user to enter the amount of money they are inserting.
- Calculate the change to be given back to the user after deducting the item price.
- Display the change to the user along with a thank you message.
Exercise 2: Multiple Item Selection
- Modify the vending machine program to allow the user to select multiple items in a single transaction.
- Prompt the user to enter the selection code of the first item.
- After dispensing the first item, ask the user if they want to continue with another selection.
- If the user chooses to continue, prompt them for the selection code of the next item.
- Repeat the process until the user indicates they are done.
- Display the total cost of all the selected items and the change to be given back to the user.
Lab Exam: Vending Machine Simulation
- Design and implement a complete vending machine simulation program in C#.
- The program should display a menu of items and their prices.
- Prompt the user to enter the selection code of the desired item.
- Use a switch statement to determine the chosen item, calculate the cost, and simulate the dispensing process.
- Implement additional features such as handling payment, maintaining inventory, and displaying a receipt.
- Test the program with different scenarios to ensure its functionality and accuracy.
Note: These exercises and lab exam are designed to enhance your understanding and practical skills in building a vending machine program in C#. Feel free to modify or expand upon them based on your learning objectives and requirements.
Related Topics and Articles:
C# SWITCH Statement Video Tutorial and Source code
Shape Area Calculator in CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.