Binary to Decimal in C#
Introduction
Table of Contents
In the world of computing, numbers play a crucial role, and different number systems are used to represent and manipulate data. Two fundamental number systems are binary and decimal. The binary system is a base-2 number system that uses only two digits, 0 and 1, to represent numbers. On the other hand, the decimal system, also known as the base-10 system, uses ten digits, 0 to 9, to represent numbers.
In this next lesson, we will explore the concept of converting a binary number to its decimal equivalent using C#. We will focus on using loops, specifically the for loop, to solve this problem efficiently. By understanding and implementing this conversion process, you will gain valuable insights into how numbers can be represented and manipulated in different number systems.
Using loops, such as the for loop or while loop, is a powerful technique in programming that allows us to repeat a set of instructions until a specific condition is met. We will leverage this concept to iterate through each digit of the binary number and calculate its decimal equivalent. By employing a systematic approach, we can accurately convert binary numbers to their decimal counterparts.
Mastering the conversion from binary to decimal is an essential skill for programmers, as it is widely used in various applications. From data handling and manipulation to network protocols and cryptography, understanding binary to decimal conversion is crucial for effectively working with binary data and ensuring accurate data representation.
In this lesson, we will dive into the intricacies of binary and decimal number systems, explore the use of loops to solve the conversion problem, and gain a comprehensive understanding of the topic’s relevance in the field of programming. So let’s get started and unlock the power of binary to decimal conversion using C#!
Objectives
The objective of this lesson is to provide a comprehensive understanding of converting binary numbers to their decimal equivalents using a for loop in C#. The primary objectives are to understand, learn, practice, and apply the art of converting binary numbers to their decimal counterparts. Each objective serves as a crucial milestone, fostering a comprehensive understanding of the topic and honing practical skills essential for any aspiring programmer.
- Understand Binary and Decimal Systems: Gain a profound comprehension of the binary and decimal number systems. Grasp the foundational principles of how binary, with its base-2 structure, relates to the familiar decimal system with its base-10 representation.
- Learn Binary to Decimal Conversion: Delve into the step-by-step process of converting binary numbers to decimal. Acquire the knowledge of interpreting binary digits and expressing them in the decimal system, laying the groundwork for more complex numerical manipulations.
- Practice Using Loops for Conversion: Engage in hands-on practice with loops, specifically the ‘for’ loop, as a powerful tool for converting binary to decimal. Through practical exercises, reinforce your understanding of loop structures and their application in numeric transformations.
- Apply Knowledge in Programming Contexts: Bridge theory and practice by applying your acquired knowledge to real-world programming scenarios. Explore the broader relevance of binary to decimal conversion, recognizing its importance in algorithms, data structures, and various programming challenges.
Source code example
using System; namespace BinaryToDecimal { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - Binary to Decimal"); // Prompt the user to enter a binary number Console.WriteLine("Enter a binary number: "); string binaryNumber = Console.ReadLine(); // Initialize variables for decimal calculation int decimalNumber = 0; int power = 0; // Iterate through each digit of the binary number in reverse order for (int i = binaryNumber.Length - 1; i >= 0; i--) { // Convert the current digit to an integer int digit = int.Parse(binaryNumber[i].ToString()); // Calculate the decimal value of the current digit decimalNumber += digit * (int)Math.Pow(2, power); // Increment the power for the next digit power++; } // Display the decimal equivalent of the binary number Console.WriteLine("The decimal equivalent is: " + decimalNumber); Console.ReadLine(); } } }
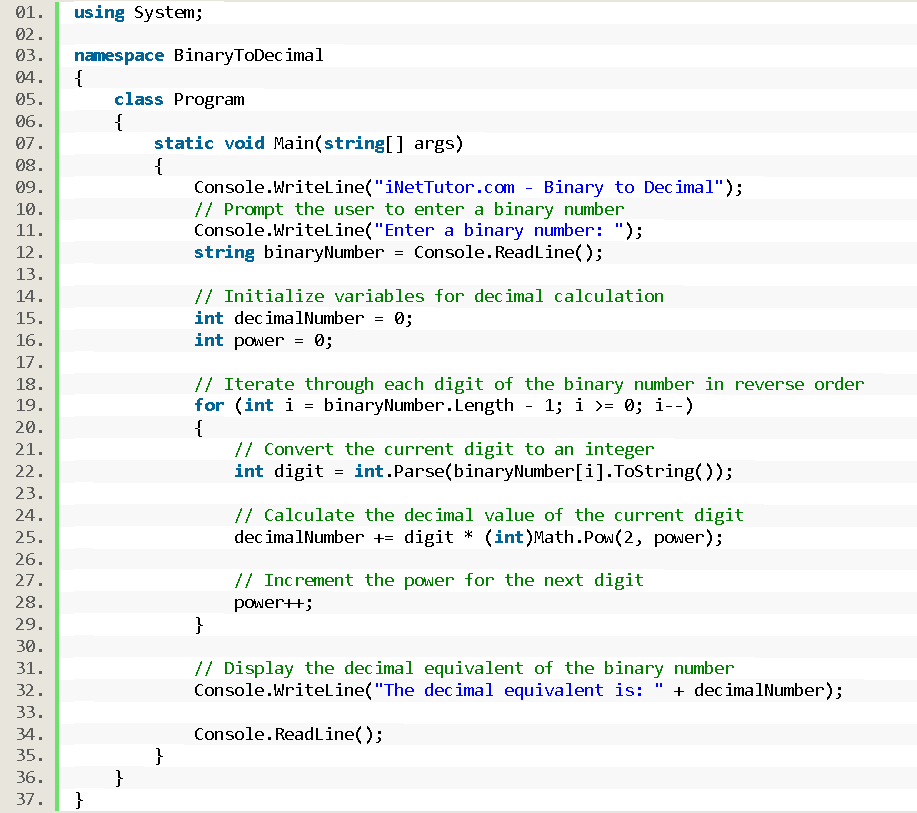
Explanation
This C# code converts a binary number entered by the user to its decimal equivalent. Here’s a breakdown of what each part does:
- Introduction:
- using System;: Includes the standard input/output library for console operations.
- namespace BinaryToDecimal: Organizes the code within a specific namespace.
- class Program: Defines the main class where the code resides.
- static void Main(string[] args): Defines the entry point of the program.
- Console.WriteLine(“iNetTutor.com – Binary to Decimal”);: Prints a welcome message with the website name.
- User Input and Initialization:
- Console.WriteLine(“Enter a binary number: “);: Prompts the user to enter a binary number.
- string binaryNumber = Console.ReadLine();: Stores the user’s input as a string.
- int decimalNumber = 0;: Initializes the variable to store the calculated decimal value.
- int power = 0;: Initializes the variable to store the power of 2 for each digit.
- Conversion Loop:
- for (int i = binaryNumber.Length – 1; i >= 0; i–): This loop iterates through each digit of the binary number in reverse order.
- int digit = int.Parse(binaryNumber[i].ToString());: Converts the current character (digit) to an integer (0 or 1).
- decimalNumber += digit * (int)Math.Pow(2, power);: This calculates the decimal value of the current digit by:
- Multiplying the digit with the corresponding power of 2 calculated using Math.Pow(2, power).
- Adding the result to the decimalNumber.
- power++;: Increments the power for the next digit.
- Output and Pause:
- Console.WriteLine(“The decimal equivalent is: ” + decimalNumber);: Displays the calculated decimal equivalent.
- Console.ReadLine();: Pauses the console window before closing.
Points to Note:
- The loop iterates in reverse order because the rightmost digit has the lowest power of 2 (2^0).
- The Math.Pow(2, power) function calculates 2 raised to the power of power.
- The code includes clear comments for better understanding.
Output
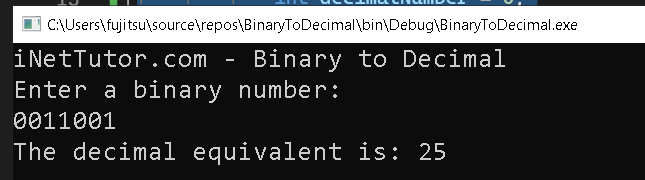
Summary
In this lesson, we explored the concept of converting a binary number to its decimal equivalent using a for loop in C#. We started by understanding the fundamental differences between the binary and decimal number systems. The binary system uses only two digits, 0 and 1, while the decimal system uses ten digits, 0 to 9.
We learned that converting a binary number to decimal involves multiplying each digit of the binary number by the corresponding power of 2 and summing up the results. To implement this conversion, we leveraged the power of loops, specifically the for loop, in C#.
By iterating through each digit of the binary number in reverse order, we converted the binary digits to decimal values using int.Parse() and Math.Pow(). We accumulated the decimal values and obtained the final decimal equivalent.
Throughout the lesson, we focused on understanding the importance of binary to decimal conversion in programming. This skill is relevant in various areas, such as data manipulation, network protocols, and cryptographic algorithms.
By practicing and applying the knowledge gained in this lesson, you can confidently convert binary numbers to decimal using a for loop in C#. This foundational knowledge will serve as a stepping stone for further exploration and understanding of number systems and their practical applications in programming.
Exercises and Assessment
To further enhance the understanding and application of the binary to decimal conversion source code, I suggest the following assessment and lab exam:
Assessment:
Objective Questions (2 points each):
- What is the base of the binary number system?
- Explain how the power of 2 is used in binary-to-decimal conversion.
- What is the purpose of iterating through the binary string in reverse order?
- Describe the role of the Math.Pow(2, power) function in the code.
- List two real-world applications of binary-to-decimal conversion.
Coding Exercise (6 points):
- Modify the code to accept only binary numbers entered as strings. Implement validation to check for invalid characters (e.g., anything other than 0 and 1).
- Add an option for the user to choose between converting from binary to decimal or vice versa (decimal to binary). Implement the necessary logic for both conversions.
- Enhance the code with comments and user-friendly messages to improve readability and provide instructions.
Total Points: 14 points
Lab Exam:
Task: Modify and enhance the existing binary-to-decimal conversion code based on the following requirements:
- Input Validation: Implement strict validation to ensure only valid binary numbers (strings containing only 0s and 1s) are accepted. Provide informative error messages for invalid inputs.
- User Interface: Create a user-friendly interface using console functions. Provide clear instructions, menus, and error handling.
- Additional Conversions: Extend the program to handle both binary-to-decimal and decimal-to-binary conversions. Allow users to choose the desired conversion type.
- Error Handling: Incorporate robust error handling for potential issues like empty input, non-numeric input, or exceeding the maximum integer value.
- Documentation: Add comments and explanations within the code to enhance clarity and understanding.
Related Topics and Articles:
C# For Loop Statement Video Tutorial and Source code
Dynamic PHP Loops using HTML Form
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.