Array Manipulation in C#
Introduction
Array manipulation is a fundamental concept in programming that plays a crucial role in data-driven applications. Arrays provide a structured way to store and organize data, allowing for efficient data retrieval and manipulation. In this lesson, we will explore the importance of array manipulation and dive into the specific operations of inserting, updating, and deleting elements in an array.
- Array manipulation is of paramount importance in programming as it enables us to efficiently handle and modify collections of data. Arrays provide a sequential storage structure, allowing quick access to elements based on their index. This capability is essential for various programming tasks, such as sorting, searching, and filtering data.
- The need for inserting, updating, and deleting elements in an array arises when we want to modify the existing data or add new elements to a specific position. Insertion allows us to expand the array and accommodate additional data at a desired index. Updating, on the other hand, enables us to modify the value of an element at a given index, ensuring data integrity and accuracy. Lastly, deletion provides the flexibility to remove unwanted elements, maintaining the array’s structure and optimizing memory usage.
- Array manipulation holds particular significance in data-driven applications. In these applications, arrays serve as containers for large datasets, enabling efficient processing and analysis. By mastering array manipulation techniques, programmers can effectively manipulate and transform data, facilitating tasks such as data cleansing, aggregation, and statistical analysis. The ability to insert, update, and delete elements in an array empowers developers to handle dynamic data sets, enhancing the functionality and responsiveness of data-driven applications.
In this lesson, we will delve into the intricacies of array manipulation, focusing on the operations of inserting, updating, and deleting elements. By understanding these techniques and their importance in data-driven applications, you will be equipped with essential skills to handle and manipulate arrays effectively in your programming endeavors.
Objectives
This lesson aims to provide a comprehensive understanding of array manipulation in programming. By the end of this lesson, you will have a clear grasp of the concepts, techniques, and algorithms involved in inserting, updating, and deleting elements in an array. Through a series of hands-on exercises and coding challenges, you will have ample opportunities to practice and reinforce your knowledge of array manipulation. By applying these skills to real-world programming problems and data-driven applications, you will gain the ability to effectively manipulate arrays and enhance the functionality of your programs.
- Understand the Foundations of Array Manipulation:
- Grasp the fundamental concepts underlying array manipulation in programming.
- Develop a clear comprehension of the role arrays play in efficient data organization.
- Learn Techniques for Array Manipulation:
- Acquire knowledge and skills regarding key array manipulation techniques.
- Explore methods for inserting, updating, and deleting elements within arrays.
- Practice Proficient Array Manipulation:
- Engage in hands-on exercises to reinforce understanding and enhance practical skills.
- Gain proficiency in implementing various array manipulation scenarios.
- Apply Array Manipulation in Practical Scenarios:
- Apply learned techniques to solve real-world programming challenges.
- Cultivate the ability to discern and apply the most suitable array manipulation methods in diverse programming contexts.
Source code example
using System; namespace ArrayManipulationExample { class Program { static void Main() { int[] numbers = new int[5]; int length = 0; Console.WriteLine("iNetTutor.com - Array Manipulation Example"); Console.WriteLine("This is an example of array manipulation on integers only"); while (true) { Console.WriteLine("\nChoose an operation:"); Console.WriteLine("1. Insert an element"); Console.WriteLine("2. Update an element"); Console.WriteLine("3. Delete an element"); Console.WriteLine("4. Print the array"); Console.WriteLine("5. Exit"); Console.Write("Enter your choice: "); int choice = Convert.ToInt32(Console.ReadLine()); if (choice == 1) { Console.Write("Enter the index where you want to insert the element: "); int index = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the value to insert: "); int value = Convert.ToInt32(Console.ReadLine()); if (index < 0 || index > length) { Console.WriteLine("Invalid index!"); } else { InsertElement(numbers, ref length, index, value); Console.WriteLine("Element inserted successfully!"); } } else if (choice == 2) { Console.Write("Enter the index of the element to update: "); int index = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the new value: "); int value = Convert.ToInt32(Console.ReadLine()); if (index < 0 || index >= length) { Console.WriteLine("Invalid index!"); } else { UpdateElement(numbers, index, value); Console.WriteLine("Element updated successfully!"); } } else if (choice == 3) { Console.Write("Enter the index of the element to delete: "); int index = Convert.ToInt32(Console.ReadLine()); if (index < 0 || index >= length) { Console.WriteLine("Invalid index!"); } else { DeleteElement(numbers, ref length, index); Console.WriteLine("Element deleted successfully!"); } } else if (choice == 4) { Console.WriteLine("Array elements:"); PrintArray(numbers, length); } else if (choice == 5) { Console.WriteLine("Exiting the program..."); break; } else { Console.WriteLine("Invalid choice! Please try again."); } } } static void InsertElement(int[] array, ref int length, int index, int element) { for (int i = length - 1; i >= index; i--) { array[i + 1] = array[i]; } array[index] = element; length++; } static void UpdateElement(int[] array, int index, int newValue) { array[index] = newValue; } static void DeleteElement(int[] array, ref int length, int index) { for (int i = index; i < length - 1; i++) { array[i] = array[i + 1]; } length--; } static void PrintArray(int[] array, int length) { for (int i = 0; i < length; i++) { Console.Write(array[i] + " "); } Console.WriteLine(); } } }
Explanation
This C# program demonstrates basic array manipulation operations, including inserting, updating, deleting, and printing elements in an array. Let’s go through the code step by step:
- Initialization:
- An integer array numbers is initialized with a fixed size of 5.
- The variable length is used to keep track of the current number of elements in the array.
- Menu and User Input:
- The program runs in a loop where the user is presented with a menu to choose from various array manipulation operations.
- The user inputs a choice (1 to insert, 2 to update, 3 to delete, 4 to print, and 5 to exit).
- Insert Element (InsertElement function):
- When the user chooses to insert an element, they are prompted to enter the index and value.
- The InsertElement function is called to insert the element at the specified index. It shifts existing elements to accommodate the new one.
- Update Element (UpdateElement function):
- For updating, the user inputs the index of the element to update and its new value.
- The UpdateElement function updates the value of the element at the specified index.
- Delete Element (DeleteElement function):
- When deleting, the user inputs the index of the element to delete.
- The DeleteElement function removes the element at the specified index and shifts the remaining elements accordingly.
- Print Array (PrintArray function):
- The program prints the array when the user chooses the “Print” option using the PrintArray function.
- Exit:
- If the user chooses the “Exit” option, the program breaks out of the loop and terminates.
- Error Handling:
- The program checks for invalid indices during insertion, updating, and deleting and provides appropriate messages.
This code allows the user to perform basic array manipulation operations interactively. It’s a beginner-friendly example that demonstrates essential concepts in handling arrays in C#.
Output
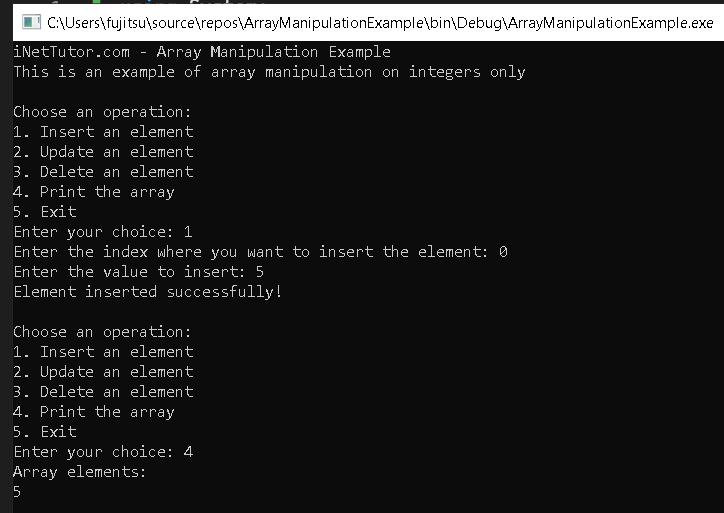
Summary
The lesson on array manipulation in the provided source code allows users to perform various operations on an array, including inserting, updating, deleting elements, and printing the array’s values. The lesson is structured as an interactive program that presents a menu of options for the user to choose from. The user can input their choices and interact with the array based on their selected operation.
The lesson begins by initializing an integer array called numbers with a size of 5 and setting the length variable to 0. The program then enters a loop where it continuously presents the user with a menu of operations, prompting the user to choose an action. The user can select from the following options:
- Insert an element: The user can input the index and value of the element they want to insert into the array. The program checks the validity of the index and performs the insertion using the InsertElement method.
- Update an element: The user can input the index of the element they want to update and the new value. The program checks the validity of the index and updates the element using the UpdateElement method.
- Delete an element: The user can input the index of the element they want to delete. The program checks the validity of the index and deletes the element using the DeleteElement method.
- Print the array: The program displays the current elements of the array up to the current length using the PrintArray method.
- Exit: The user can choose to exit the program, terminating the loop.
The lesson also includes error handling to ensure that the user’s inputs are within the valid range and provides appropriate feedback in case of invalid inputs.
The InsertElement, UpdateElement, DeleteElement, and PrintArray methods are defined to perform the respective operations on the array. These methods are called based on the user’s input to carry out the desired array manipulation.
Overall, this lesson provides a practical and interactive way for users to learn about array manipulation, allowing them to gain hands-on experience with inserting, updating, and deleting elements in an array, as well as viewing the array’s contents.
Quiz
Ready to assess your understanding of array manipulation in C#? This short quiz covers key concepts learned in the lesson, helping you solidify your knowledge of inserting, updating, deleting, and working with arrays effectively. Put your skills to the test, answer the following questions, and see how well you’ve grasped these fundamental techniques!
1. Which operation does NOT directly involve shifting elements in the array?
a) Inserting an element at the beginning
b) Updating an element’s value
c) Deleting an element from the middle
d) Printing the entire array
2. What will happen if you try to insert an element at an index beyond the array’s current size?
a) The element will be inserted automatically at the end.
b) The program will crash with an error.
c) The element will be inserted but overwrite existing data.
d) Nothing, the invalid index will be ignored.
3. What data type is used in the example code presented in the lesson?
a) Only integers
b) Any data type, chosen by the user
c) Automatically selected based on the inserted value
d) Strings and integers combined
4. What statement BEST describes the time complexity of deleting an element from the middle of the array?
a) Constant time, regardless of array size
b) Linear time, proportional to the array size
c) Logarithmic time, based on the element’s position
d) Dependent on the element’s value and other elements
5. Which function is responsible for updating the value of an element at a specific index?
a) InsertElement
b) UpdateElement
c) DeleteElement
d) None of the above, direct assignment is used.
Related Topics and Articles:
Finding the Maximum Element in an Array
Array and Array Functions in PHP
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.