Draw Square Pattern in C#
Introduction
Table of Contents
From Simple Loops to Square Masterpieces: Dive into C# Pattern Design!
Have you ever dreamt of controlling pixels with code, crafting intricate patterns on your screen? In this C# lesson, that dream becomes reality as you conquer nested loops to build a stunning square pattern of stars (or any character you choose!).
Whether you’re a coding beginner or a seasoned developer seeking new challenges, this journey is for you. We’ll break down the concepts step-by-step, guiding you through:
- The power of nested loops: Discover how to use loops within loops, building complex patterns by controlling rows and columns like a coding conductor.
- C# code in action: Watch your square masterpiece come to life as you write C# code, line by line, understanding each step that brings the pattern to the screen.
- From understanding to mastery: More than just following the code, you’ll gain a deep understanding of its inner workings, empowering you to create your own variations and patterns in the future.
This lesson is not just about stars; it’s about unlocking the creative potential within C# and your coding skills. By the end, you’ll be equipped with the knowledge to:
- Design and print square patterns of any character, customizing your creations.
- Apply your newfound understanding of nested loops to tackle other pattern challenges.
- Take a step forward in your C# journey, expanding your coding horizons with artistic expression.
So, are you ready to trade simple lines of code for dazzling squares of creativity? Join us on this exciting C# adventure and discover the power of nested loops!
Objectives
In this lesson, we will dive into the fascinating world of creating square patterns using nested for loops in C#. Understanding how to harness the power of nested loops is a valuable skill that allows us to generate intricate and visually appealing patterns. Our primary objective is to equip you with a solid understanding of nested for loops and their role in creating square patterns. Throughout this lesson, you will learn the step-by-step process of generating a square pattern with a given side length. By practicing and applying these techniques, you will gain confidence in using nested loops to create not only basic square patterns but also explore the endless possibilities of custom designs.
Specifically:
- Understand Nested For Loops: Gain a comprehensive understanding of nested for loops and their role in creating intricate patterns in C# programming.
- Learn Pattern Printing Techniques: Learn the techniques and principles behind printing square patterns, fostering a deep comprehension of the logic involved.
- Practice Pattern Design: Engage in hands-on practice sessions to reinforce your skills, allowing you to independently design and implement various square patterns.
- Apply Geometric Concepts: Apply geometric concepts through programming, enhancing your ability to translate abstract ideas into tangible, visually appealing patterns.
Source code example
using System; class Program { static void Main() { Console.WriteLine("iNetTutor.com - Square Pattern:"); // Define the side length int sideLength = 5; // Nested loops to print the square pattern for (int i = 0; i < sideLength; i++) { for (int j = 0; j < sideLength; j++) { Console.Write("* "); } Console.WriteLine(); // Move to the next line after printing a row } Console.ReadLine(); } }
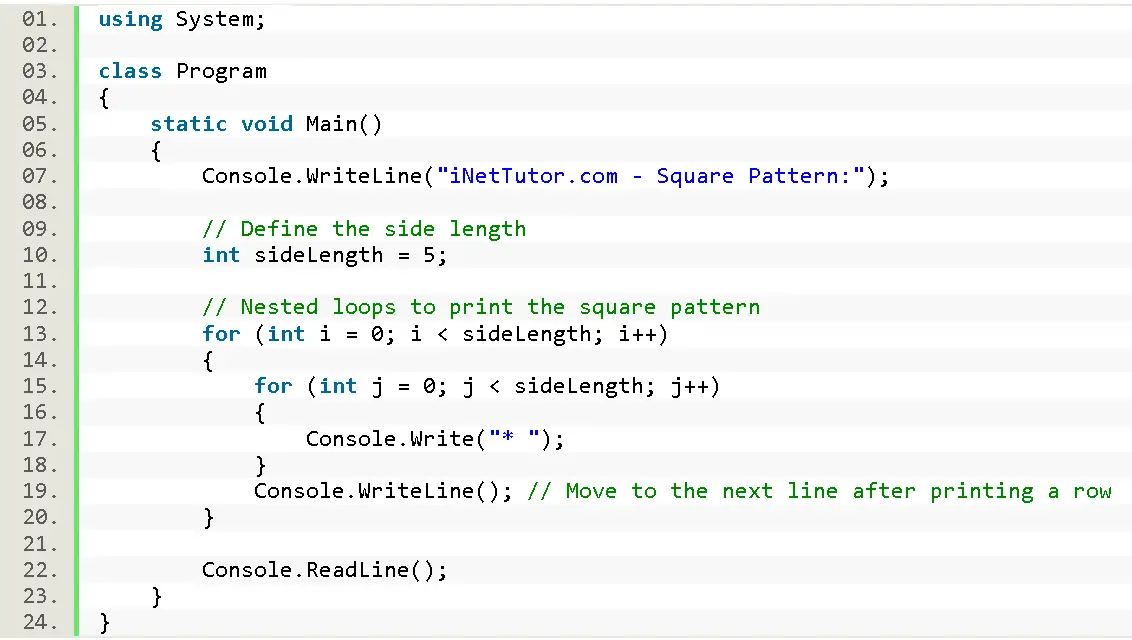
Explanation
This program uses nested loops to print a square pattern of asterisks. Adjust the sideLength variable to change the size of the square.
- using System;: This line includes the System namespace, which provides basic functionality such as console input and output.
- class Program: This declares a class named Program, which is the entry point of the C# program.
- static void Main(): This is the main method, the starting point of the program. It’s marked as static because it belongs to the class, not an instance.
- Console.WriteLine(“iNetTutor.com – Square Pattern:”);: Prints a header indicating the purpose of the program.
- int sideLength = 5;: Declares a variable sideLength with a value of 5, representing the length of each side of the square.
- Nested Loops:
- The outer loop (for (int i = 0; i < sideLength; i++)) controls the rows.
- The inner loop (for (int j = 0; j < sideLength; j++)) controls the columns.
- Console.Write(“* “);: Prints an asterisk and a space without moving to the next line.
- Console.WriteLine();: Moves to the next line after printing each row of asterisks.
- Console.ReadLine();: Pauses the program to keep the console window open until the user presses Enter.
Key Points:
- The code effectively uses nested loops to generate a square pattern of stars.
- The outer loop controls the rows, while the inner loop controls the columns within each row.
- The sideLength variable determines the size of the square and can be easily modified.
- You can change the character used in the pattern by altering the string within Console.Write().
- Experiment with different spacing or formatting options to create unique patterns.
Output
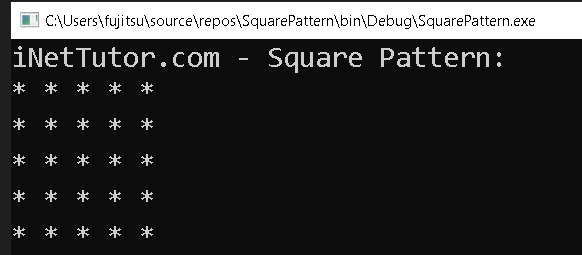
Summary
In this lesson, you explored the power of nested loops in C# by building a captivating square pattern of stars. You learned how to:
- Define the square size: Set the desired dimensions using a variable called sideLength.
- Implement nested loops: The outer loop controlled the rows, iterating for each horizontal line. The inner loop handled the columns, printing stars and spaces to create each row.
- Customize the pattern: You discovered the flexibility to modify the code easily. Change the character used, adjust the square size, or even experiment with different spacing or formatting to unleash your creative coding flair.
By understanding and applying these concepts, you unlocked a valuable skill for generating intricate patterns using C#. This foundation allows you to move forward, tackling more complex patterns and coding challenges with confidence. Remember, coding is a journey of continuous exploration and learning. Keep practicing, experimenting, and applying your newfound skills to paint your own artistic masterpieces with code!
Exercises and Assessment
Exercise: Create an exercise that requires students to modify the existing code to print a square pattern of a different character, such as ‘#’ or ‘@’. The exercise should also ask students to adjust the side length of the square pattern to 7. This exercise will test their understanding of nested loops and their ability to make modifications to the code.
Assessment: Design an assessment that evaluates students’ understanding of nested for loops and their ability to create square patterns. The assessment can include questions that require students to explain the purpose of nested loops, identify the role of each loop variable, and write code to generate a square pattern with a given side length.
Lab Exam with Rubrics: For the lab exam, provide students with a set of requirements to create a more complex square pattern using nested for loops. This can include patterns with alternating characters, patterns with increasing or decreasing characters per row, or patterns with empty spaces. Provide a rubric that assesses their code’s functionality, adherence to coding conventions, and creativity in designing the square pattern.
Rubric for Lab Exam:
- Functionality (40%): Assess the correctness and functionality of the code in generating the desired square pattern.
- Coding Conventions (30%): Evaluate the adherence to coding conventions, such as proper indentation, variable naming, and use of comments.
- Creativity (20%): Assess the creativity and complexity of the square pattern created, considering factors like alternating characters, empty spaces, or other unique designs.
- Documentation (10%): Evaluate the clarity and completeness of any documentation or comments provided in the code.
By incorporating exercises, assessments, and a lab exam with rubrics, students will have the opportunity to practice, demonstrate, and receive feedback on their understanding and application of nested for loops in creating square patterns. This comprehensive approach will help them improve their source code and enhance their programming skills.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.