Remove Vowels in string using C#
Introduction
Table of Contents
Welcome to our new lesson on string manipulation in C#! String manipulation is a powerful technique that allows us to modify and transform text data to suit our needs. In this lesson, we will focus on the concept of removing vowels from strings and explore its potential applications in various areas, including data cleaning and text encryption.
Removing vowels from a given string is a common task that can be applied in situations where we want to filter out or manipulate text by eliminating the vowel characters. By understanding the techniques involved in removing vowels, you will gain valuable skills that can be utilized in a wide range of programming scenarios.
While seemingly straightforward, this technique holds potential applications in various scenarios:
- Data Cleaning: In the process of preparing textual data for analysis, you might want to eliminate unnecessary characters like vowels to focus on the core structure of words. This can be particularly useful in tasks like sentiment analysis or topic modeling.
- Text Encryption (Educational Purposes Only): As a basic illustration (not for real-world encryption!), removing vowels can serve as a simple encryption method to obscure the original message. However, it’s crucial to understand that this is a highly vulnerable approach and should never be used for serious encryption purposes.
Throughout this lesson, we will provide a comprehensive overview of how to write a C# program that removes all vowels from a given string. We will cover different approaches, highlight important considerations, and provide practical examples to help solidify your understanding. So let’s get started and unlock the power of string manipulation in C#!
Objectives
The objectives of this lesson are designed to provide you with a comprehensive understanding of removing vowels from a given string in C#. By the end of this lesson, you will not only grasp the concepts involved but also gain practical experience in implementing this functionality in your own C# programs. Let’s dive into the objectives of this lesson:
- Understand the Concept:
- Gain a clear understanding of why and when we might want to remove vowels from a string.
- Comprehend the potential applications of removing vowels, such as data cleaning and text encryption.
- Learn the Techniques:
- Explore different techniques and approaches to remove vowels from a string in C#.
- Understand the various methods available, including using loops, string manipulation functions, and regular expressions.
- Practice Implementing the Program:
- Get hands-on experience by practicing the implementation of a C# program that removes vowels from a given string.
- Strengthen your understanding of the techniques through practical exercises and coding challenges.
- Apply the Knowledge:
- Apply the knowledge gained in real-world scenarios where removing vowels from a string is required.
- Gain confidence in utilizing this functionality in your own C# projects and applications.
By the end of this lesson, you will have a solid foundation in removing vowels from a given string using C#. You will be equipped with the knowledge and skills to confidently implement this functionality in your programming endeavors and explore the diverse applications of string manipulation.
Source code example
using System; using System.Linq; namespace VowelRemovalExample { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - Vowel Removal Example"); Console.WriteLine("Enter a string: "); string str = Console.ReadLine(); // Get user input Console.WriteLine($"Original string: {str}"); // Method 1: Using String Replacement (Simpler approach) string withoutVowels1 = str.Replace("a", "").Replace("e", "").Replace("i", "") .Replace("o", "").Replace("u", "") .Replace("A", "").Replace("E", "").Replace("I", "") .Replace("O", "").Replace("U", ""); Console.WriteLine($"Without vowels (Method 1): {withoutVowels1}"); // Method 2: Character Iteration (More control) string withoutVowels2 = ""; foreach (char c in str) { if (!"aeiouAEIOU".Contains(c)) // Check if character is not a vowel { withoutVowels2 += c; // Add the character to the new string } } Console.WriteLine($"Without vowels (Method 2): {withoutVowels2}"); Console.ReadKey(); } } }
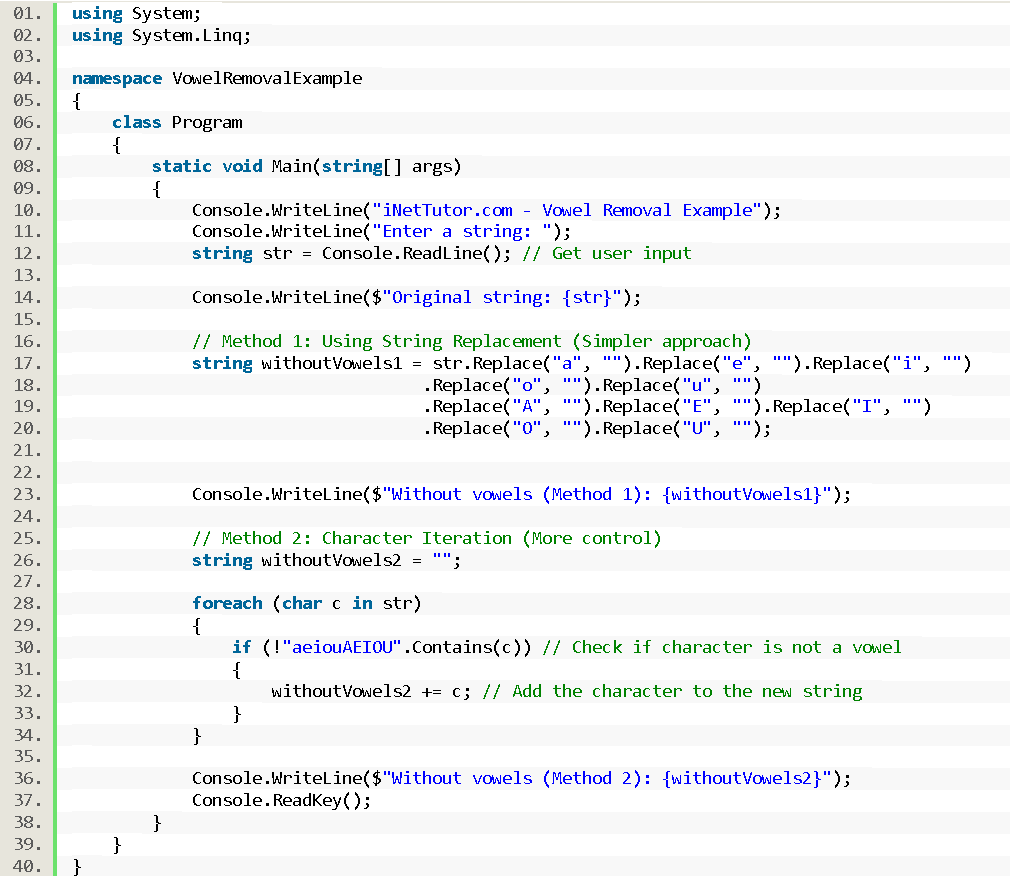
Explanation
The provided code demonstrates how to remove vowels from a user-entered string in C#. Here’s a breakdown of the code:
- Namespaces:
- using System;: This line references the core .NET libraries containing essential functionalities like console input/output.
- using System.Linq;: This line imports the Linq namespace, but it’s not directly used in the provided code for vowel removal.
- Class and Method:
- class Program: This defines a class named Program that contains the main program logic.
- static void Main(string[] args): This is the entry point of the program. The Main method is where the application execution begins.
- User Input:
- Console.WriteLine(“iNetTutor.com – Vowel Removal Example”);: Prints a message to the console.
- Console.WriteLine(“Enter a string: “);: Prompts the user to enter a string.
- string str = Console.ReadLine();: Reads the user’s input and stores it in the string variable str.
- Vowel Removal Methods:
Method 1: String Replacement (Simpler approach)
- string withoutVowels1 = str.Replace(“a”, “”).Replace(“e”, “”).Replace(“i”, “”)…:
- This line employs the Replace() method repeatedly on the string str.
- Each replacement call targets a specific vowel (“a”, “e”, “i”, etc.) and replaces it with an empty string (“”).
- This effectively removes all occurrences of the specified vowels in the string.
- Console.WriteLine($”Without vowels (Method 1): {withoutVowels1}”);: Prints the string after removing vowels using method 1.
Method 2: Character Iteration (More control)
- string withoutVowels2 = “”;: Initializes an empty string withoutVowels2 to store the vowel-less version of the input string.
- foreach (char c in str): This loop iterates through each character (c) in the original string str.
- if (!”aeiouAEIOU”.Contains(c)): This condition checks if the current character (c) is not a vowel.
- It uses the Contains() method on the string “aeiouAEIOU” which holds all the vowels.
- withoutVowels2 += c;: If the character is not a vowel, it’s appended to the withoutVowels2 string.
- Console.WriteLine($”Without vowels (Method 2): {withoutVowels2}”);: Prints the string after removing vowels using method 2.
- Pausing the Console:
- Console.ReadKey();: This line pauses the console window until the user presses any key. This is helpful to prevent the console window from closing immediately after the program execution.
Key Points:
- The code offers two methods for vowel removal:
- Method 1 (String Replacement): Simpler approach using multiple Replace() calls, but might be less efficient for longer strings.
- Method 2 (Character Iteration): More control over the process, iterates through each character and checks if it’s a vowel.
- The Linq namespace is not directly utilized in this specific code for vowel removal.
In summary, this code effectively removes vowels from a user-provided string using two different approaches, providing a clear understanding of string manipulation techniques in C#.
Output
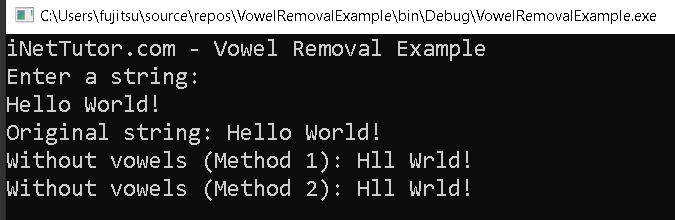
Summary
In this lesson, we explored the concept of removing vowels from a given string in C#. We discussed the potential applications of this technique, such as data cleaning and text encryption. The lesson focused on four main objectives: understanding the concept, learning the techniques, practicing implementation, and applying the knowledge.
To achieve these objectives, we provided beginner-friendly source code examples that demonstrated two different methods for removing vowels from a string. The first method used string replacement, while the second method involved character iteration. We explained each part of the code and highlighted the key points.
Throughout the lesson, we emphasized the importance of string manipulation and how it can be applied in various programming scenarios. By the end of the lesson, learners should have gained a solid understanding of removing vowels from a string in C# and be able to apply this knowledge in their own projects.
Key Points:
- String manipulation is a powerful technique in programming that allows us to modify and transform text data.
- Removing vowels from a string can be useful in scenarios such as data cleaning and text encryption.
- Two methods were demonstrated: string replacement and character iteration.
- The source code examples provided a beginner-friendly starting point for implementing vowel removal in C#.
- By the end of the lesson, learners should have a solid foundation in removing vowels from a string and be able to apply this knowledge in real-world scenarios.
Exercises and Assessment
Exercises
- Modify the code to remove only specific vowels based on user input (e.g., remove only “a” and “e”).
- Implement code to count the number of vowels present in the original string.
- Write a function that takes a string as input and returns a new string with vowels removed.
Multiple Choice
- Which of the following methods is generally more efficient for removing a large number of vowels from a long string in C#?
- a) String Replacement (Method 1)
- b) Character Iteration (Method 2)
- c) Both methods have the same efficiency.
- d) Efficiency depends solely on the specific characters being removed.
- What additional functionality can be achieved using character iteration (compared to string replacement) for vowel removal?
- a) Direct manipulation of individual characters within the loop.
- b) String replacement inherently removes all vowels.
- c) Character iteration requires more complex code for the same task.
- d) Both methods offer identical functionalities.
- How can the code be modified to remove only specific vowels (e.g., “a” and “e”) instead of all vowels?
- a) No changes are necessary, both methods handle all vowels.
- b) Modify the string used in the Replace() method (for method 1).
- c) Change the condition in the if statement (for method 2) to check for specific vowels.
- d) Vowel removal cannot be targeted to specific vowels using the provided methods.
- When using regular expressions for vowel removal in C#, which of the following statements is TRUE?
- a) Regular expressions offer a concise and efficient method for all vowel removal scenarios.
- b) Regular expressions might be less efficient for simple vowel removal compared to other methods.
- c) Regular expressions cannot be used for vowel removal in C#.
- d) Regular expressions are the only recommended approach for vowel removal.
- Which aspect is NOT crucial for effective error handling in a program that removes vowels from user input?
- a) Validating the input to ensure it’s a string.
- b) Handling situations where the user enters an empty string.
- c) Implementing mechanisms to gracefully recover from unexpected errors.
- d) Checking for the presence of special characters that might affect the vowel removal process.
Coding Exercise
Write a C# program that:
- Prompts the user to enter a string.
- Offers a menu with options to:
- Remove all vowels using either method 1 (string replacement) or method 2 (character iteration).
- Count the number of vowels present in the original string.
- Implements the chosen functionalities based on user selection.
- Displays the results (vowel-removed string or vowel count).
Related Topics and Articles:
Swap the value of two variables in C#
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.