String Comparison in C#
Problem Statement: Develop a C# program that compares two strings and determines their relationship, checking if they are equal, one is greater than the other, or if they share a common prefix. Implement the comparison using different methods and showcase scenarios for effective string handling.
Introduction of the lesson
Table of Contents
In programming, strings are fundamental data types used to represent text. They play a pivotal role in various applications, from data processing to natural language processing. String comparison, the process of determining the equality or relative ordering of strings, is a critical operation in programming.
But just like comparing apples to oranges, comparing strings can be tricky business! Imagine a login system where usernames are case-sensitive. If a user types “JohnDoe” but the system expects “johndoe,” login fails despite them being essentially the same username. This is where understanding string comparison becomes crucial.
String comparison plays a vital role in various programming tasks:
- User Authentication: Websites and applications need to accurately compare usernames and passwords for secure logins. Case-sensitivity can make a big difference here.
- Search Functionality: When users search for specific terms on a website, efficient string comparison ensures relevant results are retrieved, regardless of minor variations in capitalization or whitespace.
- Data Validation: String comparisons ensure user input adheres to specific formats, preventing errors and maintaining data integrity. Imagine validating email addresses or phone numbers – case sensitivity shouldn’t hinder a valid entry.
- Text Processing and Analysis: String comparison forms the foundation for tasks like removing duplicates, analyzing text content, or extracting specific keywords from large datasets. Understanding how strings are compared is essential for accurate manipulation.
Objectives of the lesson
The objectives of this lesson are designed to provide a structured approach to mastering the intricacies of string comparison in C#. By focusing on understanding, learning, practicing, and applying string comparison techniques, learners will develop a solid foundation in effectively comparing and manipulating textual data within their C# programs. Through hands-on practice and practical application, participants will gain the skills and confidence to employ efficient string comparison methods, thereby enhancing the reliability and performance of their C# applications.
- Understand the Basics of String Comparison: Gain a comprehensive understanding of how string comparison works in C# and grasp the foundational concepts, including equality, ordering, and case sensitivity.
- Learn Different String Comparison Methods: Explore various string comparison methods available in C#, such as ordinal, ordinalIgnoreCase, and culture-specific comparisons, to discern when to use each method appropriately.
- Practice String Comparison Techniques: Engage in hands-on practice sessions to reinforce your knowledge of string comparison. Work with real-world examples and scenarios to enhance your proficiency in applying string comparison techniques.
- Apply String Comparison in Practical Scenarios: Apply the acquired knowledge to practical programming scenarios. Develop the ability to implement string comparison effectively in tasks like data validation, sorting, and searching within C# applications.
Source code example
using System; namespace StringComparisonDemo { class Program { static void Main() { Console.WriteLine("iNetTutor.com - String Comparison Example"); // Example 1: Basic Equality Check with String.Equals string fruit1 = "apple"; string fruit2 = "Apple"; Console.WriteLine($"Comparing '{fruit1}' and '{fruit2}' for equality:"); // Using String.Equals for ordinal comparison (case-sensitive) bool isEqualOrdinal = String.Equals(fruit1, fruit2, StringComparison.Ordinal); Console.WriteLine($"Ordinal Comparison: {isEqualOrdinal}"); // Using String.Equals for ordinal ignore case comparison bool isEqualOrdinalIgnoreCase = String.Equals(fruit1, fruit2, StringComparison.OrdinalIgnoreCase); Console.WriteLine($"Ordinal Ignore Case Comparison: {isEqualOrdinalIgnoreCase}"); // Example 2: Basic Equality Check with == bool isEqualWithEqualityOperator = (fruit1 == fruit2); Console.WriteLine($"Equality Check with == Operator: {isEqualWithEqualityOperator}"); Console.ReadLine(); } } }
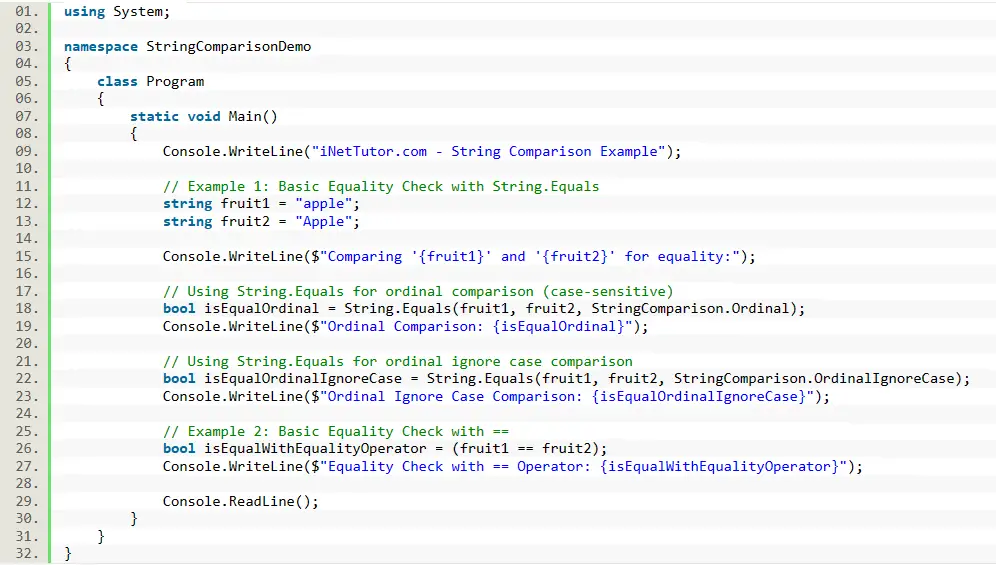
Source code explanation
Here’s a breakdown of the code, highlighting key points:
- Namespace and Class:
- The code belongs to a namespace named “StringComparisonDemo”, which organizes code elements.
- Within this namespace, there’s a class named “Program” that contains the code’s logic.
- Main Method:
- The Main method acts as the program’s entry point.
- It starts by printing a title message using Console.WriteLine.
- Example 1: String.Equals for Equality Checks:
- Two string variables, fruit1 and fruit2, are declared and assigned values (“apple” and “Apple”).
- The code uses String.Equals method for different comparison types:
- Ordinal Comparison (Case-Sensitive):
- String.Equals(fruit1, fruit2, StringComparison.Ordinal) compares strings character by character, considering case. It outputs False in this case.
- Ordinal Ignore Case Comparison:
- String.Equals(fruit1, fruit2, StringComparison.OrdinalIgnoreCase) compares strings ignoring case. It outputs True.
- Ordinal Comparison (Case-Sensitive):
- Example 2: Equality Check with == Operator:
- The code demonstrates the default string comparison using == operator, which is also case-sensitive.
- (fruit1 == fruit2) outputs False due to case differences.
- Console Input:
- Console.ReadLine() pauses the program, waiting for user input to prevent the console window from closing immediately.
Discussion:
- String.Equals method is a versatile way to perform string comparisons with various options, including case-sensitive and case-insensitive comparisons.
- The == operator is a concise way to check for basic equality between strings.
- Understanding the differences between ordinal and ordinal ignore case comparisons is crucial based on the specific requirements of the application. Ordinal comparison considers the ASCII values of characters, while ordinal ignore case comparison ignores the case of characters.
- This example provides a fundamental understanding of different approaches to compare strings in
This code provides a clear and concise demonstration of string comparison in C#, showcasing both case-sensitive and case-insensitive comparison using the String.Equals method and the equality operator ==.
Output
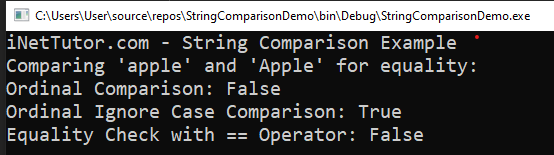
Summary and Conclusion
The “String Comparison in C#” lesson explores essential concepts and techniques for comparing strings in C#. The lesson covers both the use of String.Equals method and the == operator for basic equality checks. It highlights the importance of understanding different comparison options, such as ordinal (case-sensitive) and ordinal ignore case comparisons, and provides practical examples using strings like “apple” and “Apple.”
Important Points:
- String.Equals Method:
- The String.Equals method is used for string comparison.
- Options like StringComparison.Ordinal and StringComparison.OrdinalIgnoreCase control the comparison behavior.
- Ordinal comparison considers case, while ordinal ignore case comparison does not.
- Equality Operator (==):
- The == operator is an alternative for checking string equality.
- It provides a concise way to perform basic string equality checks.
- Understanding Ordinal Comparison:
- Ordinal comparison considers the ASCII values of characters, making it case-sensitive.
- It is suitable when case sensitivity is crucial.
- Understanding Ordinal Ignore Case Comparison:
- Ordinal ignore case comparison ignores the case of characters.
- It is useful when case sensitivity is not essential.
- Practical Examples:
- The lesson includes practical examples using strings “apple” and “Apple” to illustrate different comparison scenarios.
- Users can choose between String.Equals and the == operator based on their specific needs.
- Application in Real-world Scenarios:
- String comparison is crucial in various real-world scenarios, including data validation, sorting, and searching in C# applications.
- Versatility of String Comparison:
- Learners gain a comprehensive understanding of the versatility of string comparison techniques available in C#.
Overall, the lesson equips learners with the necessary skills to effectively compare and manipulate textual data within C# programs. It emphasizes the importance of selecting the most appropriate string comparison method for specific programming tasks, thereby enhancing the reliability and performance of C# applications.
Exercises
- Comparison Expansion:
- Modify the program to compare additional string pairs with different cases and lengths.
- Include scenarios where ordinal and ordinal ignore case comparisons yield different results.
- User Input Comparison:
- Enhance the program to accept user input for two strings and compare them.
- Provide options for users to choose between ordinal and ordinal ignore case comparisons.
Quiz
Multiple Choice Questions
- Which of the following statements is NOT true about the default string comparison operator (==) in C#?
a) It performs a character-by-character comparison.
b) It considers leading/trailing whitespace.
c) It is case-sensitive.
d) It is the simplest way to compare strings. - What does String.Equals(string a, string b, StringComparison.OrdinalIgnoreCase) achieve in C#? a) Compares strings based on current culture rules.
b) Performs a case-sensitive character-by-character comparison.
c) Compares strings, ignoring differences in case.
d) Removes leading/trailing whitespace before comparison. - What is the main advantage of using String.Equals() compared to the == operator for string comparisons in C#?
a) String.Equals() offers options for case-insensitive and whitespace handling.
b) String.Equals() is always faster than ==.
c) String.Equals() provides a more concise syntax.
d) There’s no significant difference between the two. - When might using culture-specific comparisons with CultureInfo be necessary in C#?
a) When comparing usernames for login purposes (usually not)
b) When searching for specific keywords in text data across different languages.
c) When validating product codes that only contain numbers and hyphens.
d) All of the above - What is an important consideration when comparing user input strings in C# applications?
a) User input can sometimes contain leading/trailing punctuation.
b) Users might enter mixed-case text even when expecting uppercase or lowercase.
c) String comparisons are always case-sensitive by default.
d) There’s no need to worry about whitespace in user input.
Related Topics and Articles:
Count Vowels in a String using CSharp
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.