Introduction
Table of Contents
Welcome to an engaging journey in C# programming where we delve into the intriguing realm of counting vowels. Understanding the significance of this seemingly simple task is crucial in unraveling the broader landscape of programming. In this lesson, we’ll not only explore the mechanics of counting vowels but also dissect the intricacies of the programming process.
Importance of Counting Vowels in Programming: Counting vowels might appear as a straightforward exercise, yet its importance echoes across various programming domains. From text processing and natural language understanding to data analysis and algorithmic challenges, the ability to count vowels serves as a foundational skill. Mastering this skill not only refines your coding proficiency but also equips you with a valuable tool for handling diverse real-world scenarios.
Analyzing the Problem Statement and Defining Program Requirements: Before we embark on the coding adventure, let’s dissect the problem statement. We’ll meticulously analyze the intricacies, identifying the specific requirements of our Count Vowels program. By defining these requirements, we lay the groundwork for a systematic approach, ensuring our solution aligns seamlessly with the task at hand.
Identifying the Role of a For Loop in Efficiently Counting Vowels: Central to our exploration is the role of the for loop – a stalwart construct in programming. As we aim for efficiency in counting vowels, understanding how the for loop traverses through the given string becomes paramount. Its elegant simplicity, combined with its potential for streamlined iteration, makes the for loop an indispensable tool in our coding arsenal.
Gear up for a lesson that not only sharpens your C# programming skills but also sheds light on the broader applications of seemingly elementary tasks. Let’s unravel the intricacies of counting vowels and harness the power of programming to solve this deceptively simple challenge.
Objectives
This lesson aims to cultivate a holistic understanding of the underlying concepts, fostering an environment where learners not only acquire new knowledge but actively practice and apply it in a real-world context.
Objective-Based Education (OBE) Focus:
- Understand String Manipulation in C#:
- Outcome: Develop a profound understanding of string manipulation concepts in the context of C# programming.
- Indicator: Demonstrate comprehension through explanations and discussions on the significance of string manipulation.
- Learn the Application of For Loops:
- Outcome: Acquire a strong foundation in utilizing for loops for efficient string processing and iteration.
- Indicator: Engage in hands-on learning experiences that involve crafting and applying for loops in C#.
- Practice Problem-Solving with User Input Handling:
- Outcome: Engage in active problem-solving by handling user input, validating, and managing potential errors.
- Indicator: Successfully implement user input mechanisms, demonstrating the ability to troubleshoot and resolve issues.
- Apply Vowel Counting Concept in a Real-World Scenario:
- Outcome: Apply theoretical knowledge by building a functional Count Vowels program that addresses real-world requirements.
- Indicator: Develop a working program that accurately counts vowels in various user-provided strings, showcasing practical application skills.
This OBE-focused approach ensures a well-rounded learning experience, guiding learners to not only understand and learn essential concepts but also practice and apply their knowledge effectively. Through this journey, participants will gain valuable insights into C# programming and cultivate skills that extend beyond mere theoretical understanding. Let’s embark on this immersive exploration of C# programming and the fascinating world of counting vowels.
Source code example
using System; using System.Linq; namespace VowelCounter { class Program { static void Main() { Console.WriteLine("iNetTutor.com - Welcome to the Vowel Counter!"); // Get user input Console.Write("Enter a string: "); string userInput = Console.ReadLine(); // Initialize vowel count int vowelCount = 0; // Convert input to lowercase for case-insensitive counting userInput = userInput.ToLower(); // Iterate through each character in the string for (int i = 0; i < userInput.Length; i++) { // Check if the current character is a vowel if ("aeiou".Contains(userInput[i])) { vowelCount++; } } // Display the result Console.WriteLine($"The number of vowels in the given string is: {vowelCount}"); Console.ReadKey(); } } }
Explanation
The provided source code is a C# program that counts the number of vowels in a given string. Let’s break down the code and understand how it works.
Program Structure
The program is structured as a console application with a single class named Program. It contains a Main method, which serves as the entry point of the program.
User Input
The program prompts the user to enter a string by displaying the message “Enter a string: “. The user’s input is then stored in the userInput variable.
Vowel Counting
The program initializes a variable named vowelCount to keep track of the number of vowels in the string. It then converts the user’s input to lowercase using the ToLower method to perform case-insensitive counting.
Next, the program iterates through each character in the string using a for loop. For each character, it checks if it is a vowel by using the Contains method to check if the character is present in the string “aeiou”. If the character is a vowel, the vowelCount variable is incremented.
Displaying the Result
After counting the vowels, the program displays the result by using the Console.WriteLine method. It uses string interpolation to include the value of the vowelCount variable in the output message.
Finally, the program waits for the user to press any key before exiting, using the Console.ReadKey method.
Example Execution
Here’s an example execution of the program:
iNetTutor.com - Welcome to the Vowel Counter! Enter a string: Hello World! The number of vowels in the given string is: 3
Please note that the provided code is a simple implementation of a vowel counter and may not handle all possible edge cases or variations of vowels.
Output
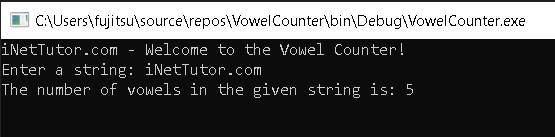
Summary
In this lesson on C# programming, we discussed string manipulation and discovered its importance through the Vowel Counter program. We learned how to efficiently and accurately count vowels in a string using for loops. This hands-on experience allowed us to see the practical application of these concepts.
As we wrap up our journey of counting vowels in C#, it’s important to recognize that the lessons learned extend beyond this specific task. String manipulation is a fundamental skill that forms the basis for solving more complex coding challenges. Armed with the knowledge gained from the Vowel Counter program, learners are now equipped to tackle a wide range of programming scenarios and navigate the intricacies of C# programming.
In conclusion, our exploration of counting vowels in C# has not only provided us with a Vowel Counter program but also equipped us with a set of skills that can be applied to various coding adventures. While our journey may end here, the knowledge and discoveries we’ve made will continue to resonate in our future coding endeavors. Happy coding!
Exercises and Assessment
Objective: Evaluate understanding and proficiency in C# programming, specifically in string manipulation and loop structures.
Assessment Tasks:
- Enhanced Vowel Counter:
- Task: Modify the existing program to count both vowels and consonants separately.
- Objective: Assess the ability to extend the program’s functionality and handle multiple counting scenarios.
- Case-Insensitive Refinement:
- Task: Enhance the program to count vowels regardless of case (both uppercase and lowercase).
- Objective: Evaluate the understanding of case-insensitive string manipulation and the effective use of string conversion.
- User-Defined String Input:
- Task: Allow users to define multiple strings for vowel counting in a single program run.
- Objective: Assess the proficiency in handling dynamic user input and managing multiple string scenarios.
- Interactive Menu System:
- Task: Implement an interactive menu system for users to choose between different counting options (vowels, consonants, both).
- Objective: Evaluate the ability to create a user-friendly interface and handle diverse counting scenarios.
These assessment tasks and coding exercises aim to challenge learners and provide opportunities for practical application, extension, and refinement of the Count Vowels program. They encourage deeper exploration of C# programming concepts and foster a more comprehensive understanding of string manipulation and loop structures.
Related Topics and Articles:
C# For Loop Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.