Swap the value of two variables in C#
Introduction
Table of Contents
In programming, variable swapping refers to the act of exchanging the values stored in two variables. It allows for the efficient reassignment of values, leading to flexible and dynamic code execution. Variable swapping is a fundamental concept in many programming languages, including C#, and is widely used in various scenarios.
Explanation of Variable Swapping and its Importance in Programming
Variable swapping is essential in programming as it enables the manipulation and reorganization of data. By swapping the values of two variables, programmers can achieve tasks such as sorting, reordering, and reassigning values efficiently. It allows for flexibility in data manipulation and can streamline code execution in numerous scenarios.
Overview of Scenarios where Variable Swapping is Useful
- Sorting Algorithms: Variable swapping plays a vital role in sorting algorithms like bubble sort, where elements are compared and swapped to arrange them in the desired order.
- Swapping Values in Algorithms: Many algorithms require swapping values to perform calculations or solve specific problems efficiently. For example, in graph algorithms, swapping is often used to update distances or rearrange nodes.
- Data Structure Operations: Variable swapping assists in various data structure operations such as swapping pointers, rearranging linked lists, or swapping values in binary trees.
- User Input and Data Validation: Swapping variables can be useful when validating user input or performing data validation tasks. It allows for comparing and rearranging values to ensure data integrity and application robustness.
- Parallel Assignments: Variable swapping simplifies parallel assignments, where multiple variables need to exchange values simultaneously, improving code readability and reducing the number of temporary variables required.
- Efficient Memory Management: In certain scenarios, swapping variables can help optimize memory usage by reassigning values between variables rather than creating new memory locations.
Overall, variable swapping is a powerful tool in programming that enables developers to manipulate and rearrange data efficiently. Its significance extends to various domains, including sorting algorithms, data structures, user input validation, and memory management. Understanding and utilizing variable swapping techniques is crucial for writing efficient and robust code in C# and other programming languages.
Objectives
The objectives of this lesson on variable swapping in C# are designed to provide a comprehensive understanding of the concept and its applications. By the end of this lesson, learners will have gained the necessary knowledge and skills to effectively swap the values of two variables in their C# programs. The focus will be on understanding the concept, learning different techniques, practicing through hands-on exercises, and applying variable swapping in real-world scenarios.
Objective 1: Understand Variable Swapping
- Gain a clear understanding of the concept of variable swapping.
- Comprehend the importance of variable swapping in programming.
- Recognize the benefits and limitations of variable swapping in C#.
Objective 2: Learn Different Techniques
- Learn the technique of using a temporary variable for swapping.
- Understand alternative methods of swapping without a temporary variable, such as bitwise XOR and arithmetic operations.
- Explore built-in functions available in C# for swapping variables.
Objective 3: Practice through Hands-on Exercises
- Engage in coding exercises to practice implementing variable swapping techniques.
- Apply the learned techniques to swap the values of two variables in different scenarios.
- Strengthen problem-solving skills by solving exercises that require variable swapping.
Objective 4: Apply Variable Swapping in Real-world Scenarios
- Understand the practical applications of variable swapping in programming.
- Apply variable swapping techniques in real-world scenarios such as sorting algorithms, data structure operations, and user input validation.
- Evaluate and select the most suitable variable swapping technique based on specific requirements and constraints.
By achieving these objectives, learners will not only have a solid theoretical understanding of variable swapping but also gain practical experience in applying the techniques to solve real-world programming challenges. This comprehensive approach ensures that learners can effectively understand, learn, practice, and apply variable swapping in their C# programs.
Source code example
using System; namespace VariableSwapping { class Program { static void Main() { Console.WriteLine("iNetTutor.com - Variable Swapping Example"); // Accept user input for two numbers Console.Write("Enter the first number: "); int firstNumber = int.Parse(Console.ReadLine()); Console.Write("Enter the second number: "); int secondNumber = int.Parse(Console.ReadLine()); Console.WriteLine($"Before swapping: First Number = {firstNumber}, Second Number = {secondNumber}"); // Swap the values using a temporary variable int temp = firstNumber; firstNumber = secondNumber; secondNumber = temp; Console.WriteLine($"After swapping: First Number = {firstNumber}, Second Number = {secondNumber}"); Console.ReadLine(); } } }
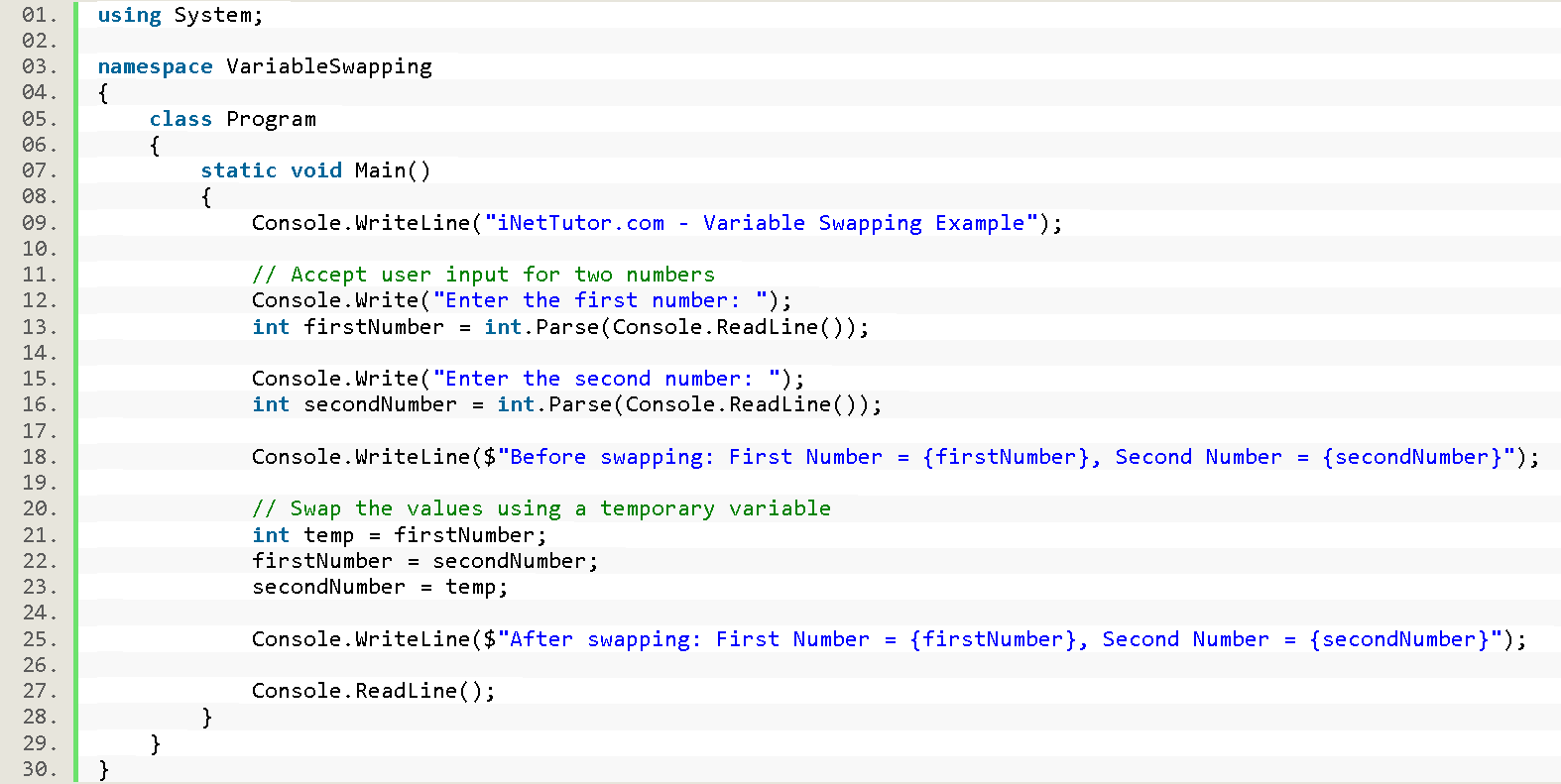
Explanation
The provided source code is a beginner-friendly example that demonstrates variable swapping in C#. Let’s go through the code step by step:
- Namespaces and Class:
- The code belongs to the namespace “VariableSwapping,” which helps organize code elements.
- Within this namespace, a class named “Program” contains the code’s logic.
- Main Method:
- The Main method serves as the program’s entry point.
- User Input:
- Console.Write(“Enter the first number: “) prompts the user to enter the first number.
- int.Parse(Console.ReadLine()) reads the user’s input, converts it to an integer using int.Parse, and stores it in the variable firstNumber.
- Similar steps are repeated to get the second number and store it in secondNumber.
- Displaying Initial Values:
- Console.WriteLine($”Before swapping: First Number = {firstNumber}, Second Number = {secondNumber}”) displays the user-entered values before swapping.
- Swapping with Temporary Variable:
- Temporary Storage: An integer variable temp is declared to hold the value of firstNumber temporarily.
- Swapping Steps:
- firstNumber = secondNumber;: Assigns the value of secondNumber to firstNumber.
- secondNumber = temp;: Assigns the previously stored value of firstNumber (now in temp) to secondNumber.
- This effectively swaps the values between firstNumber and secondNumber.
- Displaying Swapped Values:
- Console.WriteLine($”After swapping: First Number = {firstNumber}, Second Number = {secondNumber}”) displays the final values after the swap has been performed.
- Pausing the Program:
- Console.ReadLine() pauses the program execution before closing the console window, allowing the user to see the output.
Key Points:
- This code demonstrates a user-friendly approach to variable swapping, incorporating user input for the numbers.
- It utilizes a clear and well-commented temporary variable method for swapping.
Additional Notes:
- Error handling for user input (e.g., invalid characters) can be further implemented to ensure robustness.
- More advanced swapping techniques without a temporary variable are not included in this example.
Output
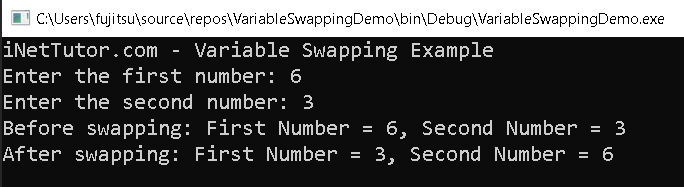
Summary
This lesson equipped you with the fundamental skills to swap variables effectively in C#, a crucial concept for data manipulation in programming. Here’s a recap of the key takeaways:
Importance of Variable Swapping:
- Highlighted the significance of swapping variables in various programming scenarios, such as sorting algorithms, game logic, and data processing.
Understanding the Process:
- Explained the limitations of direct assignment and introduced the concept of using a temporary variable to facilitate successful swapping.
Code Implementation:
- Provided a clear and well-commented code example using a temporary variable to swap two user-entered integer values.
- Demonstrated the step-by-step process of storing a variable’s value temporarily, assigning the other variable’s value, and finally transferring the stored value to complete the swap.
User Interaction:
- Showcased how to incorporate user input to make the swapping process interactive, allowing users to provide the numbers they want to swap.
Key Points:
- Grasp the concept: Understand why swapping variables is necessary and how it impacts data manipulation.
- Temporary variable method: Master the traditional approach using a temporary variable to hold the value of one variable while assigning the other’s value.
- Clear and concise code: Focus on writing well-structured and commented code for better readability and maintainability.
- User-friendly input: Consider incorporating user input to make your programs more interactive.
Remember:
- This lesson focused on a fundamental technique for swapping variables.
- More advanced scenarios might involve swapping elements within arrays or data structures using different approaches.
- Choosing the appropriate swapping method depends on the specific programming task and desired efficiency.
By effectively applying these concepts, you’ll be well on your way to becoming a proficient C# programmer capable of manipulating data dynamically and tackling various programming challenges that require variable swapping.
Exercises and Assessment
Exercises:
- Multiple Swaps:
- Extend the program to perform multiple swaps, allowing the user to swap the values of more than two variables in a single run.
- No Temporary Variable:
- Challenge learners to swap two variables without using a temporary variable. Encourage them to explore alternative methods and discuss the pros and cons of each approach.
- Variable Swapping Function:
- Modify the program to encapsulate the swapping logic within a function. Users should then call this function to perform the swap, enhancing code organization.
- Explore alternative swapping techniques without a temporary variable (e.g., using the XOR operator). Research the efficiency implications of each method.
Quiz
- What is the primary purpose of variable swapping in programming?
- A. Enhancing code readability
- B. Improving program efficiency
- C. Reordering variable declarations
- D. Facilitating user input
- In the context of swapping two variables, why might a temporary variable be used?
- A. To save memory
- B. To simplify the code
- C. To avoid errors
- D. To store an intermediate value
- How can the program be enhanced to accept user input for variable values?
- A. By using constants
- B. By incorporating a loop
- C. By avoiding user input
- D. By using complex data types
- What would be a potential challenge when swapping variables without a temporary variable?
- A. Increased code complexity
- B. Improved performance
- C. Simpler implementation
- D. Unchanged code structure
- Why is encapsulating the swapping logic in a function beneficial?
- A. It simplifies the code.
- B. It avoids the need for comments.
- C. It increases code redundancy.
- D. It decreases program modularity.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.