(C#) CSharp Variables
Introduction to Variables in C#
Table of Contents
In the world of programming, variables are like the building blocks that allow us to store, manage, and manipulate data within our code. They act as placeholders for various types of information, making it possible for us to work with dynamic and changing data. Whether you’re a beginner stepping into the realm of programming or an experienced developer, understanding the concept of variables is fundamental to writing effective and efficient code in any language.
In this blog post, we’ll dive into the core concept of variables and explore their significance in the realm of programming. We’ll specifically focus on C#, a versatile and widely used programming language developed by Microsoft. C# empowers developers to create a wide range of applications, from desktop software to web applications and even games. Central to this language are variables, which allow us to store and manipulate data in a flexible and organized manner.
Explanation of the Concept of Variables
Imagine variables as containers that hold different types of information. Just as you might use labeled boxes to store different items in your home, variables hold data within a program. They can store various types of information, such as numbers, text, dates, and more. The beauty of variables lies in their dynamic nature—they can be updated and changed as your program runs, enabling you to build interactive and responsive applications.
Importance of Variables in Programming
Variables play a crucial role in programming for several reasons. They allow us to:
- Store and Manipulate Data: Variables give us the ability to store data temporarily in memory, which we can then use, manipulate, or process as needed. This is essential for performing calculations, making decisions, and displaying dynamic content.
- Enhance Readability: By assigning meaningful names to variables, we enhance the readability of our code. Descriptive variable names provide insights into the purpose of the stored data, making the code more understandable and maintainable.
- Enable Reusability: Variables allow us to reuse and reference data throughout our code. Instead of hardcoding values, we can use variables to hold those values and refer to them multiple times, promoting code efficiency.
- Facilitate Debugging: Debugging becomes easier when variables are used appropriately. By inspecting the values stored in variables during runtime, developers can identify issues and correct them efficiently.
Overview of Variable Declaration and Initialization in C#
In C#, declaring a variable involves specifying its data type and giving it a name. The process of assigning an initial value to a variable is known as initialization. C# supports a variety of data types, such as integers, floating-point numbers, characters, strings, and more. Properly declaring and initializing variables ensures that they’re ready to hold data and participate in your program’s logic.
Throughout this blog post, we’ll explore various aspects of working with variables in C#. We’ll learn how to declare them, initialize them with values, and use them in different contexts to build powerful and dynamic applications.
As we delve deeper into the world of C# variables, you’ll discover how they lay the foundation for creating efficient, organized, and interactive code. So, whether you’re just beginning your programming journey or seeking to enhance your skills, understanding the concept of variables is an essential step towards becoming a proficient C# developer. Let’s embark on this journey to harness the power of variables in C# programming!
Objectives
- Objective: Understand the Concept of Variables
- Outcome: Develop a clear comprehension of what variables are in programming, recognizing their role as placeholders to store and manage different types of data, enabling dynamic data manipulation.
- Objective: Demonstrate Proper Variable Declaration and Initialization
- Outcome: Learn how to declare variables by specifying data types and providing meaningful names. Gain proficiency in initializing variables with initial values, ensuring they are ready to hold and interact with data.
- Objective: Utilize Variables for Data Manipulation
- Outcome: Acquire the ability to perform arithmetic operations, string concatenation, and other data manipulations using variables. Understand how to update variable values and reuse them within a program’s logic.
- Objective: Enhance Code Readability and Maintainability
- Outcome: Discover the importance of using descriptive variable names to improve code readability. Learn how well-named variables contribute to better code organization and make the logic of a program more understandable to fellow developers.
Variable Declaration and Initialization
In C# programming, variables are essential components that allow us to store and manage data within our programs. They act as named placeholders for various types of information, enabling dynamic data manipulation and processing. In this section, we will discuss the syntax for declaring variables, initializing them with values, understanding default values for different data types, and exploring the concept of variable scope.
Syntax for Declaring Variables in C#
In C#, variables are declared using a specific syntax that includes the data type, variable name, and optional initialization with a value. The basic syntax for declaring a variable is as follows:
data_type variable_name;
For example, to declare an integer variable named “age,” the syntax would be:
int age;
Initializing Variables with Values
Variables can be initialized with values at the time of declaration. This involves assigning an initial value to the variable, allowing it to hold data from the start. Initialization is done using the following syntax:
data_type variable_name = initial_value;
For instance, to declare and initialize an integer variable “score” with an initial value of 100, the syntax would be:
int score = 100;
Default Values for Different Data Types In C#, variables have default values assigned to them if they are not explicitly initialized. The default values depend on the data type of the variable. Some common default values include:
- 0 for numeric data types (int, double, float, etc.)
- false for boolean data type
- null for reference types (string, object, etc.)
For example, if you declare an integer variable without initializing it, it will have a default value of 0:
int count; // Default value of count is 0
Scope of Variables
The scope of a variable refers to the portion of code where the variable can be accessed and used. Variables can have different levels of scope, which determine their visibility and availability within different parts of a program. In C#, variables can have local scope, meaning they are accessible only within the block of code where they are declared.
For example:
void SomeMethod()
{
int localVar = 5; // localVar is accessible only within this method
// … code …
}
Variable declaration and initialization are fundamental concepts in C# programming. Understanding how to declare variables with proper syntax, initialize them with values, grasp default values, and manage their scope is crucial for effective and organized coding. By mastering these concepts, programmers can efficiently handle and manipulate data throughout their programs, contributing to the creation of robust and functional software.
Variable Naming Conventions and Best Practices
When working with variables in C# programming, adopting consistent and meaningful naming conventions is essential. Proper naming enhances code readability, maintainability, and collaboration among developers. In this section, we will discuss guidelines for choosing variable names, explore different naming conventions, emphasize avoiding reserved keywords, and emphasize the importance of clarity and readability in variable naming.
Guidelines for Choosing Variable Names
- Descriptive and Meaningful: Choose variable names that reflect the purpose and content of the data they store. Aim for names that provide a clear understanding of the variable’s role within the context of the program.
- Avoid Abbreviations: While abbreviations might save space, they can make code harder to understand. Opt for full words that convey the variable’s meaning.
- Be Concise: While names should be descriptive, they should also be concise. Long variable names can make code harder to read. Strike a balance between descriptive and concise names.
Camel Case vs. Pascal Case Camel case and Pascal case are two common naming conventions for variables in C#.
- Camel Case: In camel case, the first word is in lowercase, and the first letter of subsequent words is capitalized. Example: studentName, totalAmount.
- Pascal Case: In Pascal case, the first letter of each word is capitalized. This convention is often used for class names and method names. Example: StudentName, TotalAmount.
Choose the appropriate convention based on the context. For local variables and parameters, camel case is commonly used. For class-level variables and properties, Pascal case is more prevalent.
- Avoiding Reserved Keywords C# has a set of reserved keywords that have special meanings in the language. Avoid using these keywords as variable names to prevent conflicts and confusion. For example, you cannot use int as a variable name because it’s a reserved keyword for the integer data type.
- Clarity and Readability in Variable Naming Variable names should convey their purpose clearly to anyone reading the code. Consider the following tips:
- Use nouns for variables that represent data.
- Use verbs for variables that represent actions or behaviors.
- Add context if necessary to distinguish variables with similar names.
Discussion
Proper variable naming conventions and practices contribute to code quality and maintainability. Here’s how each aspect discussed above benefits your programming efforts:
- Descriptive and Meaningful Names: Clear names make it easier to understand the purpose of variables. This is particularly important when you revisit your code after some time or when collaborating with others.
- Abbreviations: While abbreviations might make sense in specific domains, avoid overly cryptic or domain-specific abbreviations that might not be understood by others.
- Concise Names: Strive for concise names that convey the variable’s role without excessive length. Concise names improve code readability and comprehension.
- Camel Case vs. Pascal Case: Consistency in naming conventions improves code consistency and predictability. Choose the convention that aligns with the variable’s usage.
- Avoiding Reserved Keywords: Avoiding reserved keywords ensures that your variable names won’t cause conflicts with the language itself.
- Clarity and Readability: Clear and readable variable names reduce the chances of misinterpretation and errors. They contribute to a smoother coding experience.
Variable Assignment and Manipulation
In C# programming, working with variables involves not only declaring them but also assigning values to them and manipulating those values as needed. This section will cover the fundamental concepts of assigning values to variables and updating those values through manipulation.
Assigning Values to Variables
Assigning a value to a variable means storing a specific piece of data in that variable. In C#, the assignment operator (=) is used to assign a value to a variable. Here’s the basic syntax:
dataType variableName = value;
- dataType: The data type of the variable, such as int, double, string, etc.
- variableName: The name of the variable you’re assigning the value to.
- value: The actual value you’re assigning to the variable.
For example:
int age = 25; double price = 9.99; string name = “John”;
Updating Variable Values
Once a value is assigned to a variable, you can update or manipulate that value based on your program’s requirements. This is particularly useful when you need to perform calculations or modify the stored data. You can use arithmetic operators and assignment operators to update variable values.
Arithmetic operators:
- +: Addition
- –: Subtraction
- *: Multiplication
- /: Division
- %: Modulus (remainder)
Assignment operators:
- =: Assigns a value
- +=: Adds a value and assigns the result
- -=: Subtracts a value and assigns the result
- *=: Multiplies by a value and assigns the result
- /=: Divides by a value and assigns the result
- %=: Performs modulus and assigns the result
Here are some examples:
int count = 5; count = count + 2; // count is now 7 double total = 100.0; total -= 20.0; // total is now 80.0 int x = 10; x *= 3; // x is now 30 int y = 15; y %= 7; // y is now 1
Discussion
Assigning values to variables and updating those values are fundamental operations in programming. These concepts play a crucial role in creating dynamic and interactive applications. Here’s how these concepts are used in practical scenarios:
- Assigning Initial Values: Assigning values to variables at the beginning of a program allows you to store and manage data that your program will work with.
- User Input: When you prompt users to input data, you assign their input to variables. This allows your program to process and manipulate the user’s data.
- Calculations: Variables are commonly used to store and manipulate data for calculations. You can update variables to hold intermediate results or the final output of a computation.
- Dynamic Behavior: By updating variables, you can create dynamic behavior in your programs. For example, you can create counters, trackers, or animations that change over time.
- Data Transformation: Variables enable data transformation by allowing you to change data from one form to another based on specified rules or algorithms.
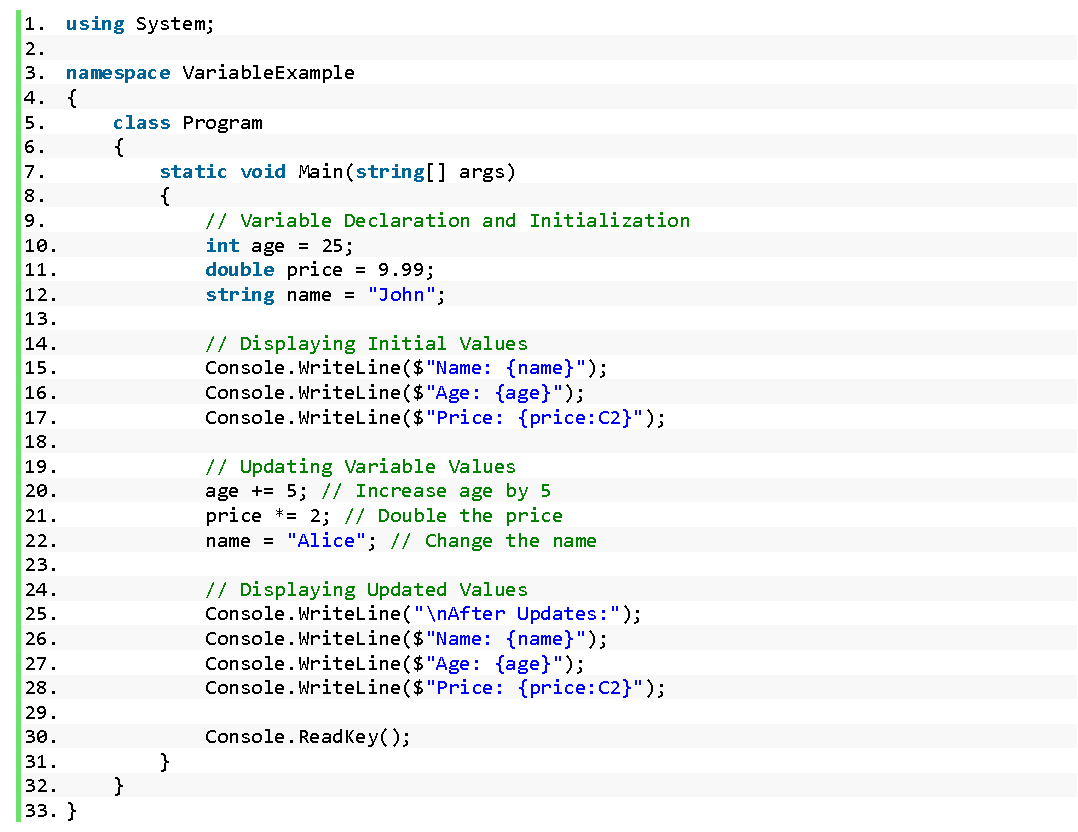
Source code Example
using System; namespace VariableExample { class Program { static void Main(string[] args) { // Variable Declaration and Initialization int age = 25; double price = 9.99; string name = "John"; // Displaying Initial Values Console.WriteLine($"Name: {name}"); Console.WriteLine($"Age: {age}"); Console.WriteLine($"Price: {price:C2}"); // Updating Variable Values age += 5; // Increase age by 5 price *= 2; // Double the price name = "Alice"; // Change the name // Displaying Updated Values Console.WriteLine("\nAfter Updates:"); Console.WriteLine($"Name: {name}"); Console.WriteLine($"Age: {age}"); Console.WriteLine($"Price: {price:C2}"); Console.ReadKey(); } } }
Code Explanation
- Declare and initialize variables for age, price, and name.
- Display the initial values of these variables using Console.WriteLine.
- Update the values of the age, price, and name variables using arithmetic and assignment operators.
- Display the updated values of the variables.
- Use Console.ReadKey() to pause the program and wait for a key press before exiting.
When you run this program, you’ll see the initial values displayed, then the updated values after the manipulations. This demonstrates the basic concepts of variable assignment and manipulation in C#.
Output
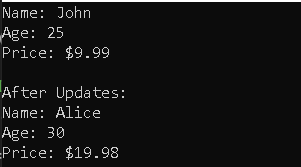
Summary
In the journey through the topic of C# variables, we delved into essential concepts that serve as the foundation of programming in C#. We explored variable declaration, initialization, assignment, and manipulation, gaining a comprehensive understanding of how variables function as crucial building blocks in creating dynamic and functional programs.
Recap of Key Concepts: We began by understanding the syntax of variable declaration in C# and learned how to assign initial values to variables. We explored various data types and their default values, recognizing that each data type plays a distinct role in storing different types of information. We also discussed variable scope and its impact on where variables can be accessed and modified within a program.
Variable Naming Conventions and Best Practices: A crucial aspect of variables is their naming. We delved into the guidelines for choosing meaningful and descriptive variable names, promoting clarity and readability in our code. We explored the difference between camel case and Pascal case, and the importance of avoiding reserved keywords as variable names.
Variable Assignment and Manipulation: The ability to assign values to variables and manipulate those values is at the heart of programming. We learned how to use assignment operators to update variables and observed how different operations impact variable values. Through examples, we demonstrated the process of incrementing, decrementing, and performing arithmetic operations on variables.
Importance of Understanding Variables in C# Programming: The significance of understanding variables cannot be overstated. Variables provide a means to store and manage data dynamically, allowing programs to perform calculations, make decisions, and respond to user input. A solid grasp of variables enables programmers to create adaptable and interactive applications.
Encouragement to Practice and Experiment with Variables: As with any programming concept, practice is key to mastery. We encourage you to experiment with variables, test different data types, and explore how variable values change through manipulations. By applying variables in various scenarios, you’ll gain confidence and proficiency in their use.
Resources for Further Learning and Practice: To deepen your understanding of variables and expand your programming skills, consider exploring the following resources:
- Official Microsoft C# Documentation: The official documentation provides comprehensive information on variables, data types, and programming concepts. Visit the Microsoft Docs website for in-depth C# documentation.
- Online Coding Platforms: Websites like LeetCode, HackerRank, and CodeSignal offer coding challenges and exercises that allow you to practice using variables in different contexts.
- C# Programming Books: Numerous books cover C# programming topics, including variables and data types. Choose a book that aligns with your learning level and goals.
- Programming Communities: Engage with fellow programmers on platforms like Stack Overflow and Reddit. Participating in discussions and asking questions can provide insights and solutions to challenges you encounter.
As you continue your programming journey, remember that variables are the foundation upon which you’ll build complex applications. By mastering variables, you’re equipping yourself with the tools to create dynamic, responsive, and powerful software solutions. So, dive into practice, experiment fearlessly, and embrace the journey of becoming a proficient C# programmer. Happy coding!
Quiz
- What is a variable in programming?
a) A fixed value that cannot be changed
b) A named storage location for data that can hold different values during program execution
c) A reserved keyword in C#
d) A type of loop construct - Which of the following is NOT a valid variable name in C#?
a) user_name
b) 123variable
c) totalAmount
d) First Name\ - What is the default value of an uninitialized integer variable in C#?
a) 0
b) -1
c) null
d) It depends on the context - What does the term “variable scope” refer to?
a) The size of memory allocated for a variable
b) The time it takes to assign a value to a variable
c) The range within which a variable can be accessed and modified
d) The data type of a variable - Which of the following is an example of a proper camel case variable name?
a) TotalAmount
b) total-amount
c) totalAmount
d) Total_Amount - What is the purpose of variable initialization?
a) To create a new variable
b) To allocate memory for a variable
c) To assign an initial value to a variable when it is declared
d) To convert a variable’s data type - How can you update the value of a variable in C#?
a) By redeclaring the variable
b) By using the “set” keyword
c) By using assignment operators like += or -=
d) Variables cannot be updated once assigned - Which data type is used to store whole numbers in C#?
a) double
b) string
c) int
d) char - What does the operator “++” do when applied to a variable?
a) It adds 1 to the variable’s value
b) It subtracts 1 from the variable’s value
c) It multiplies the variable’s value by 2
d) It divides the variable’s value by 2 - What is the purpose of using variables in programming?
a) To confuse other programmers
b) To store data and manipulate it during program execution
c) To slow down program execution
d) To replace functions in a program
Related Topics and Articles:
C# Program Structure and Basic Syntax
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.