Sum of Array Elements in CSharp
Introduction
Arrays play a pivotal role in programming, serving as fundamental data structures that allow for efficient organization and manipulation of data. They provide a systematic way to store and access elements of the same type, enabling programmers to manage large sets of data more effectively. Arrays facilitate streamlined data processing and retrieval through index-based access, making it easier to iterate over elements and perform various operations. This foundational structure forms the basis for more advanced data structures and algorithms, contributing significantly to the efficiency and elegance of software solutions. Understanding arrays is essential for any programmer, as they are widely used in diverse applications, from simple tasks to complex computations.
In this specific lesson, we zoom in on a common and practical task: calculating the sum of array elements using the C# programming language. The ability to compute the sum of elements is a fundamental operation with broad applications in data analysis, statistics, and algorithmic solutions. The lesson introduces beginners to the basics of working with arrays in C#, emphasizing the dynamic allocation of memory, user input for array elements, and a straightforward method for calculating the sum. By focusing on this specific task, learners gain hands-on experience with array manipulation and iteration, laying a solid foundation for more advanced programming challenges. The lesson not only addresses the technical aspects of array manipulation but also highlights the importance of input validation, ensuring robust and error-resistant code.
Objectives
Objective 1: Understand the Basics of Arrays in C# Outcome: By the end of this lesson, learners will demonstrate an understanding of the fundamental concepts related to arrays in C#. They will be able to articulate the purpose of arrays in programming, explain the syntax for creating arrays, and comprehend the significance of array-based data organization.
Objective 2: Implement Dynamic Memory Allocation for Arrays Outcome: Upon completion of the lesson, learners will be capable of dynamically allocating memory for arrays in C#. They will be able to prompt users for the size of an array, allocate memory accordingly, and input elements into the array, showcasing proficiency in managing memory dynamically.
Objective 3: Calculate the Sum of Array Elements in C# Outcome: Learners will acquire the ability to calculate the sum of array elements in C# without using additional methods or foreach loops. They will demonstrate competence in writing concise and effective code to iterate through array elements, accumulate their values, and display the resulting sum.
Objective 4: Implement Input Validation Techniques Outcome: At the conclusion of this lesson, learners will have the skills to implement input validation for both the size of the array and individual array elements. They will showcase the ability to handle invalid user inputs gracefully, enhancing the robustness and reliability of their C# programs.
These outcomes-based objectives ensure that learners not only acquire theoretical knowledge but also develop practical skills in array manipulation, memory allocation, and input validation within the context of C# programming. The objectives are structured to provide a well-rounded understanding of arrays and their applications.
Source code
using System; namespace ArraySumCalculator { class Program { static void Main() { Console.Write("Enter the number of elements in the array: "); if (int.TryParse(Console.ReadLine(), out int n) && n > 0) { int[] array = new int[n]; int sum = 0; // Input array elements and calculate the sum for (int i = 0; i < n; i++) { Console.Write($"Enter element {i + 1}: "); if (int.TryParse(Console.ReadLine(), out int element)) { array[i] = element; sum += element; } else { Console.WriteLine("Invalid input. Please enter a valid integer."); i--; // Retry the current index } } // Display the result Console.WriteLine($"Sum of array elements: {sum}"); } else { Console.WriteLine("Invalid input. Please enter a valid positive integer for the array size."); } Console.ReadKey(); // Keep the console window open } } }
Output
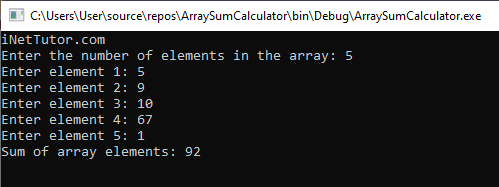
Source code Explanation
- Namespace and Class Declaration (line 3 and 5):
- The code is organized within the ArraySumCalculator namespace, providing a logical container for related code elements.
- The class is named Program, which is a conventional name for the main entry point of a C# console application.
- Main Method (line 7):
- The Main method is the entry point of the program and is automatically called when the program starts.
- User Input for Array Size (line 10 – 40):
- Prompts the user to enter the number of elements in the array.
- Uses int.TryParse to ensure that the input is a valid integer, and the entered value is greater than 0.
- Array Declaration and Sum Initialization (line 14-15):
- Declares an array of integers (array) with a size specified by the user input (n).
- Initializes a variable (sum) to store the cumulative sum of array elements.
- Input Array Elements and Calculate Sum (line 18-32):
- Uses a for loop to iterate through each element of the array.
- Prompts the user to input each element and validates if the input is a valid integer.
- If valid, assigns the input to the array and updates the sum; if invalid, prompts the user to retry the input for the current index.
- Display the Result (line35):
- Outputs the calculated sum of array elements to the console.
- Input Validation (Array Size and Element) (line 37-40):
- Handles the case when the user provides an invalid input for the array size.
- Keep Console Open (line 42):
- Uses Console.ReadKey() to keep the console window open until a key is pressed, allowing the user to view the output.
This program is designed to dynamically allocate memory for an array, input elements from the user while calculating their sum, handle invalid inputs gracefully, and display the result. It provides a practical example of array manipulation and user interaction in a C# console application.
Summary
In this insightful lesson, we explored the foundational concept of arrays in C# programming, with a particular focus on the practical task of calculating the sum of array elements. We began by understanding the pivotal role arrays play in programming, serving as efficient tools for organizing and manipulating data. Learners delved into the dynamic allocation of memory for arrays, acquiring hands-on experience in prompting users for array size, inputting elements, and implementing robust input validation. The lesson showcased an iterative approach to calculating the sum of array elements, demonstrating clear and concise code organization within a namespace. The program encouraged user interaction through meaningful prompts and engaged learners in a responsive console experience. By emphasizing best practices and user-friendly design, the lesson not only imparted specific coding skills but also empowered learners to explore further and experiment with array manipulation in the versatile landscape of C# programming.
Exercises
Recommended Exercises for Further Practice:
- Array Modification: Extend the program to allow users to modify individual elements of the array after the initial input. Implement a menu system for actions like adding, updating, or removing elements.
- Average Calculation: Modify the program to calculate and display the average of array elements. Ensure the program handles both integer and floating-point input for a more versatile application.
- Array Sorting: Implement a feature to sort the array in ascending or descending order. Explore different sorting algorithms and choose one that aligns with the lesson’s complexity level.
- Enhanced User Interaction: Enhance the user interaction by incorporating a more intuitive input process. Consider using a graphical user interface (GUI) or providing a guided menu system.
Suggestions for Expanding on the Current Topic:
- Multidimensional Arrays: Explore the world of multidimensional arrays. Modify the program to handle a 2D array and calculate the sum or average along rows and columns.
- Array Operations Library: Create a library of reusable methods for common array operations, such as finding the maximum or minimum element, checking for the presence of a specific value, or reversing the array.
- File I/O Integration: Extend the program to read array elements from an external file or write the array elements to a file. This introduces the concept of file input/output and data persistence.
- Error Handling Refinement: Refine the error-handling mechanisms to provide more detailed error messages. Implement a system that allows users to retry their input for a specific element rather than restarting the entire array input process.
These exercises and suggestions are designed to deepen understanding and provide opportunities for learners to apply and extend their knowledge of array manipulation in C#. They cater to varying levels of complexity, allowing learners to choose exercises that align with their current skill set while encouraging exploration and experimentation.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.