Understanding PHP Encapsulation
Introduction to Encapsulation
Table of Contents
Encapsulation, a fundamental principle of Object-Oriented Programming (OOP), serves as the cornerstone of well-structured and maintainable software systems. In the realm of PHP, a versatile and widely-used scripting language, encapsulation plays a pivotal role in shaping clean, secure, and organized code. This indispensable concept goes beyond mere coding techniques; it empowers developers to control access to data, promote data integrity, and enhance code readability.
Encapsulation is crucial in programming, including in PHP, for several compelling reasons:
- Data Protection: Encapsulation allows you to protect your data from unauthorized access and modification. By restricting direct access to an object’s properties (e.g., making them private or protected), you ensure that data remains consistent and adheres to specific rules or validations. This prevents accidental or malicious tampering with critical information.
- Abstraction: Encapsulation encourages the concept of abstraction, which means hiding complex implementation details and providing a simplified interface for interacting with an object. Users of a class don’t need to know the inner workings; they can focus on using the provided methods and properties, making code more intuitive and user-friendly.
- Code Organization: Encapsulation promotes code organization by bundling data (properties) and related behaviors (methods) into a single unit (class). This modular approach makes codebases more structured and manageable, simplifying maintenance and reducing the risk of errors.
- Flexibility and Maintenance: Encapsulation makes it easier to modify and extend code without affecting other parts of the program. When you encapsulate data and behaviors within a class, changes can be localized to that class, reducing the risk of unintended consequences in other parts of your application.
- Code Readability: Well-encapsulated code tends to be more readable and self-explanatory. With clear access modifiers and method names, developers can quickly understand how to interact with a class and its objects, which aids in collaboration and code reviews.
- Enhanced Security: In scenarios where security is paramount, encapsulation helps ensure that sensitive data or critical processes remain protected. For example, you can encrypt and securely store sensitive data within a class, making it more difficult for malicious actors to access it.
- Support for Inheritance and Polymorphism: Encapsulation is closely related to other OOP principles like inheritance and polymorphism. It enables you to define the behaviors of a class and its subclasses in a structured way, fostering code reuse and flexibility.
- Reduced Complexity: By encapsulating data and behaviors, complex operations can be abstracted into simple, high-level methods. This reduces the complexity of code, making it easier to understand and maintain.
Objectives of the lesson
- Understand the Concept of Encapsulation
- Explain the fundamental concept of encapsulation in Object-Oriented Programming (OOP).
- Define the role of access modifiers (public, private, protected) in encapsulation.
- Describe how encapsulation contributes to data protection and code organization in PHP.
- Practice Implementing Encapsulation
- Create PHP classes that encapsulate data properties with different access modifiers.
- Implement getter and setter methods to control access to class properties.
- Write code examples demonstrating the practical use of encapsulation in real-world scenarios.
- Solve coding exercises that require encapsulating properties and methods within classes.
- Apply Encapsulation in PHP Projects
- Apply the principles of encapsulation in the development of PHP applications.
- Incorporate encapsulation to protect sensitive data and control access within an application.
- Evaluate and refactor existing code to enhance encapsulation and improve code quality.
- Implement encapsulation to simplify code maintenance and promote code reusability in PHP projects.
These objectives are designed to ensure that learners not only grasp the theoretical foundations of encapsulation but also gain practical experience in implementing and applying encapsulation principles in PHP development.
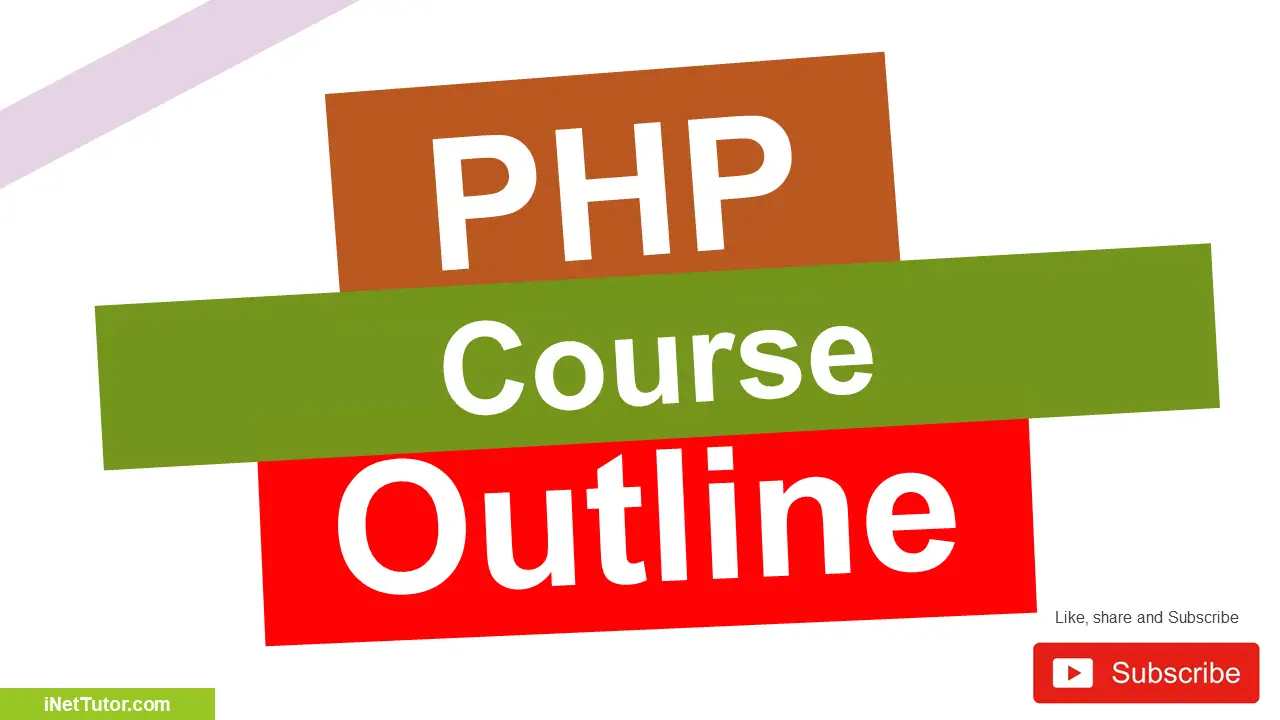
PHP Encapsulation Example (php_encapsulation.php)
<!DOCTYPE html> <html> <head> <title>Encapsulation Example</title> </head> <body> <h1>Person Information</h1> <?php class Person { // Private properties private $name; private $age; // Constructor public function __construct($name, $age) { $this->name = $name; $this->age = $age; } // Getter method for name public function getName() { return $this->name; } // Setter method for name public function setName($name) { $this->name = $name; } // Getter method for age public function getAge() { return $this->age; } // Setter method for age public function setAge($age) { if ($age >= 0) { $this->age = $age; } } // Method to display information public function displayInfo() { echo "Name: " . $this->getName() . "<br>"; echo "Age: " . $this->getAge() . "<br>"; } } // Create a Person object $person = new Person("Juan Dela Cruz", 25); // Access properties using getter methods echo "Name: " . $person->getName() . "<br>"; echo "Age: " . $person->getAge() . "<br>"; // Use setter methods to update properties $person->setName("Pedro Masipag"); $person->setAge(30); // Display updated information $person->displayInfo(); ?> </body> </html>
Explanation
- We define a Person class with private properties $name and $age. These properties are encapsulated, meaning they cannot be accessed directly from outside the class.
- We provide getter methods (getName and getAge) to retrieve the values of the private properties. These methods allow controlled access to the encapsulated data.
- We provide setter methods (setName and setAge) to update the values of the private properties. These methods allow us to apply validation or business rules before modifying the data.
- We create a Person object, set its initial values, and then use getter and setter methods to access and update the encapsulated properties.
- Finally, we use the displayInfo method to display the person’s information, demonstrating that encapsulated data can be accessed and modified through controlled methods.
This example illustrates how encapsulation in PHP helps protect data integrity and provides controlled access to properties and methods within a class.
Output
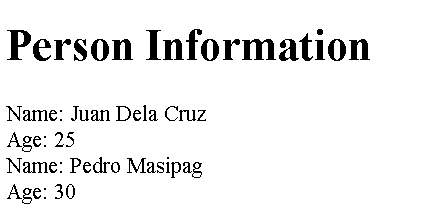
How encapsulation enhances code security and maintainability
Encapsulation in PHP significantly enhances code security and maintainability. By encapsulating data with private or protected access modifiers, it restricts direct access to critical properties, preventing unauthorized modification and ensuring data integrity. Moreover, encapsulation promotes standardized getter and setter methods, simplifying code maintenance, enhancing readability, and enabling flexibility when modifying internal class implementations. This abstraction layer minimizes complexity, supports efficient testing, and facilitates collaboration among developers, ultimately contributing to secure, clean, and maintainable PHP codebases.
Benefits of getter and setter methods
Getter and setter methods, often referred to as accessors and mutators, play a crucial role in Object-Oriented Programming (OOP) and encapsulation. Getter and setter methods are essential tools for achieving encapsulation, promoting code consistency, ensuring data integrity, and enhancing the flexibility and maintainability of PHP and OOP codebases. They are a fundamental component of object-oriented design and development.
Here are the benefits of using getter and setter methods in PHP and other programming languages:
- Controlled Access:
- Getter Methods: They provide controlled access to the private or protected properties of a class, allowing you to retrieve their values. This control ensures that data is accessed according to your predefined rules and validations.
- Setter Methods: Setter methods enable controlled modification of property values. You can apply checks and validations before updating a property, ensuring data consistency and preventing invalid or unauthorized changes.
- Encapsulation and Abstraction:
- Getter and setter methods are a fundamental part of encapsulation. They abstract the internal representation of data and provide a simplified interface to interact with class properties, promoting the concept of abstraction.
- Data Validation:
- Setter methods allow you to implement data validation and constraints. For example, you can ensure that a numeric property remains within a specific range or that a string property adheres to a certain format.
- Flexibility and Future-Proofing:
- If the internal representation of a property needs to change in the future (e.g., from a simple variable to a calculated value), using setter and getter methods ensures that external code relying on the class’s interface remains unaffected. This flexibility is critical for maintaining and evolving codebases.
- Code Consistency:
- By providing a standardized way to access and modify properties, getter and setter methods contribute to code consistency. Developers working with the class can rely on a well-defined interface, reducing the chances of introducing errors.
- Improved Debugging and Logging:
- Getter and setter methods can be enhanced with logging or debugging statements to track property changes, making it easier to diagnose issues and monitor application behavior.
- Security and Access Control:
- Getter and setter methods are instrumental in enforcing access control. You can implement access control logic within these methods to restrict certain actions based on user roles or permissions, contributing to application security.
- Documentation and Self-Documenting Code:
- Well-named getter and setter methods serve as self-documenting code. They convey the purpose and usage of properties, making code more understandable for developers who work with the class.
- Testing:
- Getter and setter methods facilitate unit testing. You can write test cases to verify that these methods behave correctly, ensuring that property access and modification meet the desired expectations.
Best practices for method encapsulation
Encapsulation is a fundamental concept in Object-Oriented Programming (OOP) that promotes secure and organized code. Here are best practices for method encapsulation in PHP:
- Use Access Modifiers:
- Choose the appropriate access modifier (public, private, or protected) for each method based on its intended visibility and accessibility.
- Public methods should provide the main interface for interacting with the class, while private and protected methods should be used for internal processes.
- Keep Methods Focused:
- Each method should have a single, well-defined responsibility. Avoid creating methods that do too many things.
- Follow the Single Responsibility Principle (SRP) to ensure that each method has one reason to change.
- Descriptive Method Names:
- Use descriptive and meaningful names for methods that convey their purpose and functionality.
- Method names should be verbs or verb phrases that describe the action performed.
- Limit Method Length:
- Keep methods concise and focused on their specific tasks. Long methods can be difficult to understand and maintain.
- Consider breaking down complex methods into smaller, reusable ones.
- Minimize Method Parameters:
- Avoid methods with an excessive number of parameters. Too many parameters can make method calls and testing cumbersome.
- Use parameter objects or associative arrays when passing multiple values.
- Avoid Global State:
- Methods should not rely on global variables or modify global state. Instead, they should operate on their own encapsulated data.
- Limit side effects to improve predictability and testability.
- Favor Composition Over Inheritance:
- Encapsulate behavior through composition rather than relying solely on inheritance. This promotes flexibility and reduces class coupling.
- Prefer using methods to delegate tasks to other objects (composition) rather than inheriting behavior (inheritance).
- Validate Inputs:
- Implement input validation and error handling within methods, especially for public methods that accept external data.
- Validate inputs to ensure they conform to expected formats or constraints.
- Document Methods:
- Provide clear and concise documentation for each method, including descriptions of their purpose, parameters, return values, and any exceptions they may throw.
- Use PHPDoc comments to document methods in a standardized format.
- Test Methods Thoroughly:
- Write unit tests for methods to verify their behavior and ensure they work as expected.
- Test edge cases and boundary conditions to cover various scenarios.
- Review and Refactor:
- Regularly review your class’s methods and refactor them as needed to maintain clean, efficient, and maintainable code.
- Apply design principles like SOLID to improve the overall structure of your code.
- Follow Coding Standards:
- Adhere to coding standards and conventions to ensure consistency and readability in your codebase.
By following these best practices, you can create well-encapsulated methods that contribute to the overall quality and maintainability of your PHP code. Encapsulation helps manage complexity, reduce errors, and promote reusability in your object-oriented designs.
Summary
In the blog post, “Understanding PHP Encapsulation,” we embarked on a journey into the heart of Object-Oriented Programming, unraveling the essential concept of encapsulation and its profound implications in PHP development. We explored how encapsulation empowers developers to safeguard data, enforce validation rules, and maintain code integrity through the strategic use of access modifiers, getter and setter methods, and the principle of abstraction. This comprehensive exploration shed light on how encapsulation serves as a cornerstone for secure, maintainable, and efficient PHP applications, where code remains protected, organized, and adaptable in the face of evolving requirements, reinforcing the idea that encapsulation is not just a programming technique but a paradigm that leads to robust and resilient software systems.
Quiz
1. What is encapsulation in PHP?
- A programming language
- A design pattern
- A fundamental OOP concept
- A database management system
2. Which access modifier restricts access to class members to within the same class only?
- public
- protected
- private
- static
3. What is the primary purpose of getter and setter methods in encapsulation?
- To make properties public
- To hide properties from the class
- To control access to properties
- To define properties
4. How can encapsulation enhance code security?
- By making all properties public
- By using global variables
- By restricting direct access to data
- By avoiding the use of methods
5. True or False: Encapsulation encourages the principle of abstraction by hiding complex implementation details.
6. Which principle suggests that each method should have a single, well-defined responsibility?
- Inheritance
- Polymorphism
- Encapsulation
- Single Responsibility Principle (SRP)
7. What should method names in encapsulation be?
- Short and cryptic
- Irrelevant to their purpose
- Descriptive and meaningful
- Numerical
8. Which practice helps ensure method maintainability?
- Creating long, complex methods
- Avoiding documentation
- Keeping methods concise and focused
- Ignoring unit testing
9. What is the benefit of using composition over inheritance in encapsulation?
- It simplifies code.
- It promotes reusability.
- It avoids encapsulation.
- It introduces complexity.
10. How can you enforce access control in encapsulated classes?
- By using only public methods
- By avoiding encapsulation
- By implementing access checks in getter and setter methods
- By exposing all properties
Readers are also interested in:
Introduction to Object Oriented Programming
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.