Understanding PHP Inheritance with Examples
Are you ready to unlock the true potential of your PHP applications? Welcome to the exciting world of PHP inheritance! In this blog post, we’re embarking on a journey into one of the cornerstones of Object-Oriented Programming (OOP). Understanding PHP inheritance isn’t just about grasping a concept; it’s about empowering your code to reach new heights of modularity, reusability, and efficiency.
What is Inheritance?
Table of Contents
Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that allows you to create new classes (called child classes or subclasses) based on existing classes (called parent classes or superclasses). It enables you to inherit and reuse the properties (attributes) and behaviors (methods) of a parent class in a child class, promoting code reuse and modularity. Inheritance is a core principle of OOP and is often depicted as an “is-a” relationship.
Here’s a detailed explanation and discussion of inheritance in OOP:
- Inheritance Hierarchy: Inheritance establishes a hierarchical relationship between classes. At the top of the hierarchy is the parent class, and beneath it are one or more child classes. Child classes inherit the properties and methods of the parent class.
- Reuse of Code: One of the primary benefits of inheritance is code reuse. When you have common attributes and behaviors shared among multiple classes, you can define them in a parent class and have child classes inherit those attributes and behaviors. This eliminates the need to duplicate code, making your codebase more efficient and easier to maintain.
- Extending and Specializing: Child classes can extend the functionality of the parent class by adding new attributes and methods or by overriding existing ones. This allows you to create specialized versions of a class while still benefiting from the shared features of the parent class.
- The “is-a” Relationship: Inheritance represents an “is-a” relationship between the parent and child classes. For example, if you have a Vehicle class, you can create child classes like Car and Motorcycle. A car “is-a” vehicle, and a motorcycle “is-a” vehicle, which makes inheritance a natural choice.
- Access to Parent Class Members: In most OOP languages, child classes have access to the public and protected members (properties and methods) of the parent class. This allows child classes to leverage the functionality provided by the parent class.
- Method Overriding: Child classes can override (redefine) methods inherited from the parent class to provide their own implementation. This is useful when you want a specific behavior in the child class to differ from that of the parent class.
- Constructors in Inheritance: Constructors of child classes can call the constructor of the parent class using the parent::__construct() method, ensuring that both the parent and child class constructors are executed when an object is created.
- Multiple Inheritance: Some programming languages, including PHP, support multiple inheritance, where a child class can inherit from multiple parent classes. This allows you to combine features from multiple sources, but it should be used with caution to avoid ambiguity.
- Abstract Classes and Interfaces: In addition to concrete classes, inheritance is essential for abstract classes and interfaces. Abstract classes provide a blueprint for other classes and may contain abstract methods to be implemented by child classes. Interfaces define a contract that classes must adhere to, enabling polymorphism.
Objectives of the lesson
- Understand the Concept of Inheritance
- Define the concept of inheritance in OOP and its role in code organization.
- Explain the “is-a” relationship between parent and child classes.
- Describe the types of inheritance, including single, multiple, multilevel, and hierarchical.
- Practice Implementing Inheritance
- Create a parent class (superclass) with properties and methods.
- Extend the parent class to create one or more child classes (subclasses).
- Inherit properties and methods from the parent class and add new attributes and behaviors to child classes.
- Implement method overriding to customize behavior in child classes.
- Demonstrate the use of constructors in inheritance, including invoking parent class constructors.
- Apply Inheritance in PHP Projects
- Utilize inheritance to build class hierarchies in PHP projects.
- Identify scenarios where inheritance can improve code reusability and organization.
- Evaluate and refactor existing code to leverage inheritance effectively.
- Create specialized child classes to model real-world objects or entities.
- Demonstrate the benefits of inheritance, including code reuse and specialization, in practical PHP applications.
These objectives are designed to ensure that learners not only grasp the theoretical concepts of inheritance but also gain hands-on experience in implementing and applying inheritance principles in PHP development.
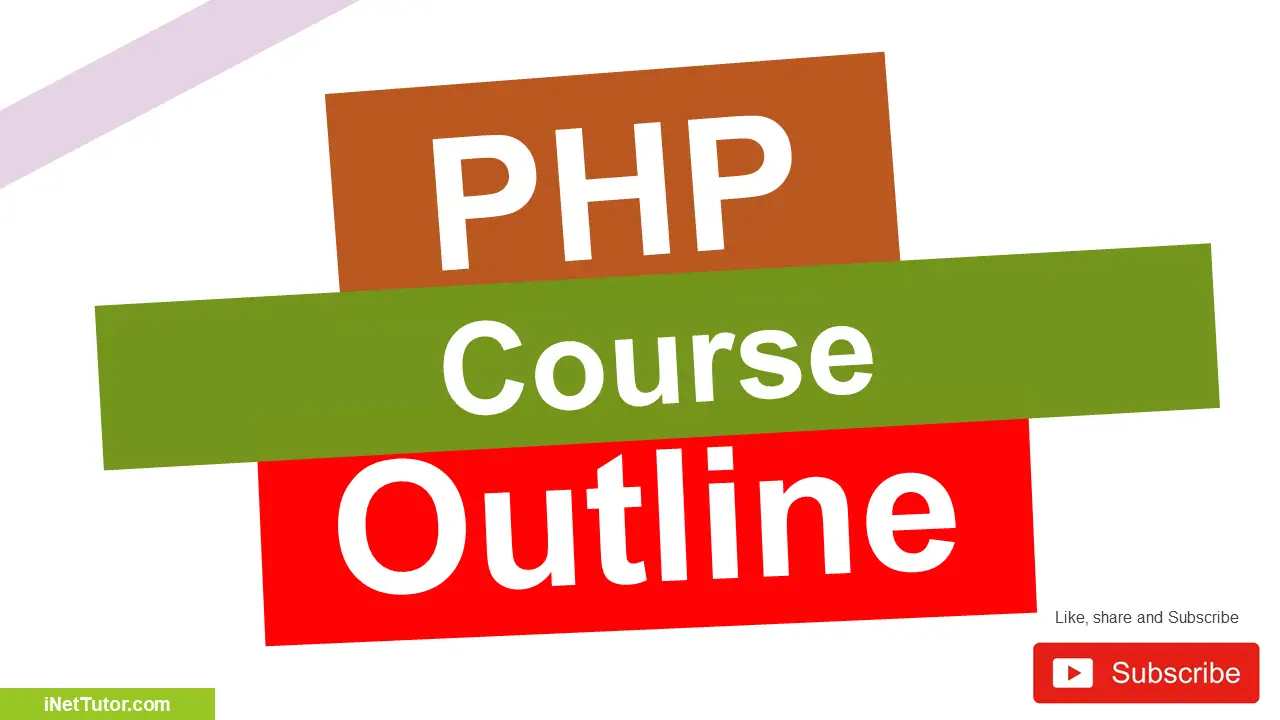
Benefits and Best Practices
Advantages of Inheritance in PHP:
Inheritance offers several notable advantages in PHP and Object-Oriented Programming (OOP) in general. First and foremost, it promotes code reusability by allowing developers to define a common set of properties and methods in a parent class, known as the superclass. Child classes, referred to as subclasses, can then inherit these members, eliminating the need for redundant code. This not only makes codebases more efficient but also simplifies maintenance.
Furthermore, inheritance supports modularity, facilitating organized code structures. Developers can extend parent classes by adding new functionality to child classes without altering the parent’s core implementation. This separation of concerns enhances code maintainability, as changes to one part of the codebase don’t ripple through the entire system. Additionally, inheritance enables specialization. Child classes can build upon the parent class’s foundation while customizing and enhancing specific behaviors. For instance, a generic “Shape” class might have child classes like “Circle” and “Rectangle,” each specializing in its respective shape.
Inheritance also enforces consistency by ensuring that related classes share method names and signatures. This consistency makes it easier for developers to work with classes intuitively, fosters predictability, and simplifies understanding and usage. Lastly, inheritance supports efficient updates. When changes are required, modifications made to the parent class automatically propagate to all child classes, ensuring that updates are applied consistently and efficiently.
Best Practices for Using Inheritance in PHP:
When applying inheritance in PHP, certain best practices should be followed to ensure effective and maintainable code. First, it’s crucial to adhere to the “is-a” relationship principle. This means that a child class should represent a more specialized version of the parent class, reflecting a clear hierarchy of objects.
Careful use of access modifiers (public, protected, private) is vital. Public members should provide a well-defined and stable interface for external code, while protected and private members should be used for internal implementation details.
Deep inheritance hierarchies should be avoided, as they can lead to complex and challenging-to-maintain code. Strive for reasonably shallow hierarchies to maintain clarity and simplicity.
It’s also essential to consider alternatives to inheritance. In some cases, composition (object containment) may be a more appropriate solution, especially when you want to avoid tight coupling between classes.
Documentation plays a crucial role in maintaining code clarity. Document the role and usage of each class within the inheritance hierarchy, and use PHPDoc comments to describe methods and properties effectively.
Constructor chaining (calling parent class constructors) should be done judiciously to prevent creating overly complex initialization processes. Prioritize readability by using meaningful class and method names that enhance code understanding.
Extensive testing is essential to ensure that inheritance hierarchies function as intended. Implement unit tests to verify that child classes correctly inherit and override methods.
Lastly, evaluate whether inheritance is the best solution for a specific problem. In some cases, alternative design patterns, such as composition or interfaces, may be more suitable and lead to a cleaner and more maintainable codebase.
By adhering to these best practices, developers can harness the power of inheritance while maintaining a clean, maintainable, and efficient PHP codebase. Inheritance is a powerful tool when used judiciously and aligned with sound design principles.
PHP Inheritance Syntax
Inheritance in PHP is achieved through the extends keyword. Here’s the syntax for creating child classes that inherit from a parent class:

Now, let’s break down the syntax and explain it in detail:
- class ParentClass { … }: This is the definition of the parent class, also known as the superclass. Inside this class, you define properties and methods that are common to all child classes that will inherit from it. These properties and methods are available for inheritance.
- class ChildClass extends ParentClass { … }: This is the definition of the child class, also known as the subclass. By using the extends keyword, you specify that ChildClass inherits all the properties and methods of ParentClass. In other words, ChildClass is extending the functionality of ParentClass.
- Additional Properties and Methods: Inside the child class, you can define additional properties and methods that are specific to this child class. These properties and methods are in addition to what the child class inherits from the parent class. This allows you to customize and specialize the behavior of the child class.
Example (php_inheritance.php)
<!DOCTYPE html> <html> <head> <title>PHP Inheritance Example</title> </head> <body> <?php class Animal { public $name; public function eat() { echo $this->name . " is eating.<br>"; } } class Dog extends Animal { public function bark() { echo $this->name . " is barking.<br>"; } } $dog = new Dog(); $dog->name = "Brownie"; ?> <h1>Animal Actions</h1> <p><?php $dog->eat(); ?></p> <p><?php $dog->bark(); ?></p> </body> </html>
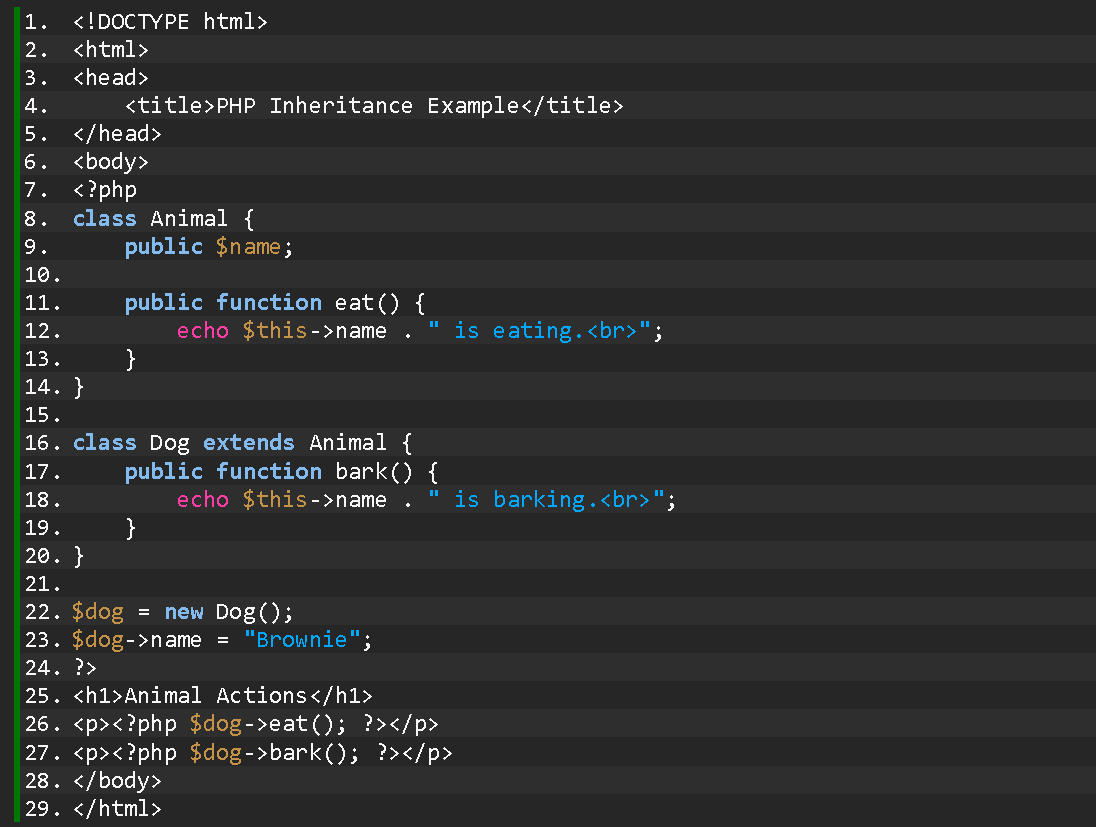
Output
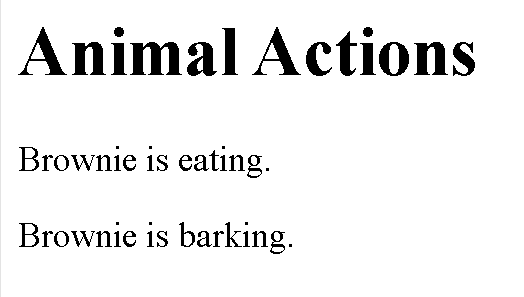
Explanation
In this example, we have a Animal class with a property $name and a method eat(). Then, we create a Dog class that extends Animal. The Dog class inherits the $name property and the eat() method from the Animal class, and it also has its own method bark(). When we create a Dog object and call its methods, it demonstrates how inheritance allows you to reuse and extend functionality in PHP classes.
Summary
In the lesson “Understanding PHP Inheritance with Examples,” we have explained concept of inheritance and its practical implications within PHP. We learned that inheritance serves as a fundamental building block that enables the creation of hierarchical relationships between classes, empowering code reusability, modularity, and specialization. By extending parent classes to create child classes, developers can seamlessly inherit properties and methods, fostering efficient code organization and maintenance.
Throughout the lesson, we explored the syntax of inheritance in PHP and witnessed its power through hands-on examples. We witnessed how child classes inherit the attributes and behaviors of parent classes, enabling the customization and enhancement of functionality to suit specific needs. We practiced method overriding, gained insights into constructor chaining, and grasped the essence of the “is-a” relationship that underpins inheritance. From the foundations of parent and child classes to the intricacies of polymorphism and practical implementations, we delved into the heart of inheritance and its profound role in shaping structured and maintainable PHP applications.
Quiz
1. What is inheritance in PHP?
a. A database management system
b. A code editor
c. A way to create child classes based on existing classes
d. A version control system
2. Which keyword is used to create a child class that inherits from a parent class in PHP?
a. implements
b. extends
c. inherits
d. inheritsFrom
3. What is the primary advantage of inheritance in PHP?
a. It reduces the need for code documentation.
b. It allows multiple inheritance.
c. It promotes code reusability and modularity.
d. It eliminates the need for classes.
4. Which relationship is represented by inheritance in OOP?
a. “has-a”
b. “uses-a”
c. “is-a”
d. “does-a”
5. In a child class, which keyword is used to call a method from the parent class that has been overridden?
a. override
b. call_parent
c. parent::method()
d. $this->method()
6. What does method overriding mean in PHP inheritance?
a. It creates new methods in the child class.
b. It allows multiple methods with the same name in the same class.
c. It replaces a method in the child class with a new one.
d. It redefines a method in the child class with a specialized implementation.
7. What is the purpose of constructor chaining in inheritance?
a. To create a constructor in the child class
b. To call the constructor of the parent class from the child class constructor
c. To create multiple constructors in the same class
d. To break the inheritance chain
8. Which of the following statements is true regarding deep inheritance hierarchies?
a. They are recommended for complex projects.
b. They simplify code maintenance.
c. They should be avoided as they can lead to code complexity.
d. They promote code reusability.
9. What should you do when implementing inheritance in PHP to maintain code clarity?
a. Avoid documenting your classes.
b. Use cryptic class and method names.
c. Prioritize readability with meaningful class and method names.
d. Create deep inheritance hierarchies.
10. When might you consider using composition over inheritance?
a. When you want to reuse code efficiently.
b. When you want to create a hierarchy of classes.
c. When you want to avoid tight coupling between classes.
d. When you want to eliminate classes entirely.
Readers are also interested in:
Introduction to Object Oriented Programming
Understanding PHP Encapsulation
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.