Methods in C#
Introduction
Table of Contents
This lesson is all about the methods in C#. The lesson includes sample program to better understand how a method works in C#.
A method is a function that is associated with a class. Methods are used to conduct operations on objects of the class. For example, the function print() can be used to print the contents of an object to the console. Methods can also be used to return values. For example, the method getName() can be used to return the name of an object. Methods are normally declared with the keyword method.
Methods can be used to conduct a range of operations on objects. For example, methods can be used to retrieve the attributes and methods of an object. Methods can also be used to create new items from existing objects. Methods are often employed to conduct specific tasks that need to be performed repeatedly. Methods can be used to build reusable code. Methods are also often employed to give a greater level of abstraction than the basic operations that can be performed on objects.
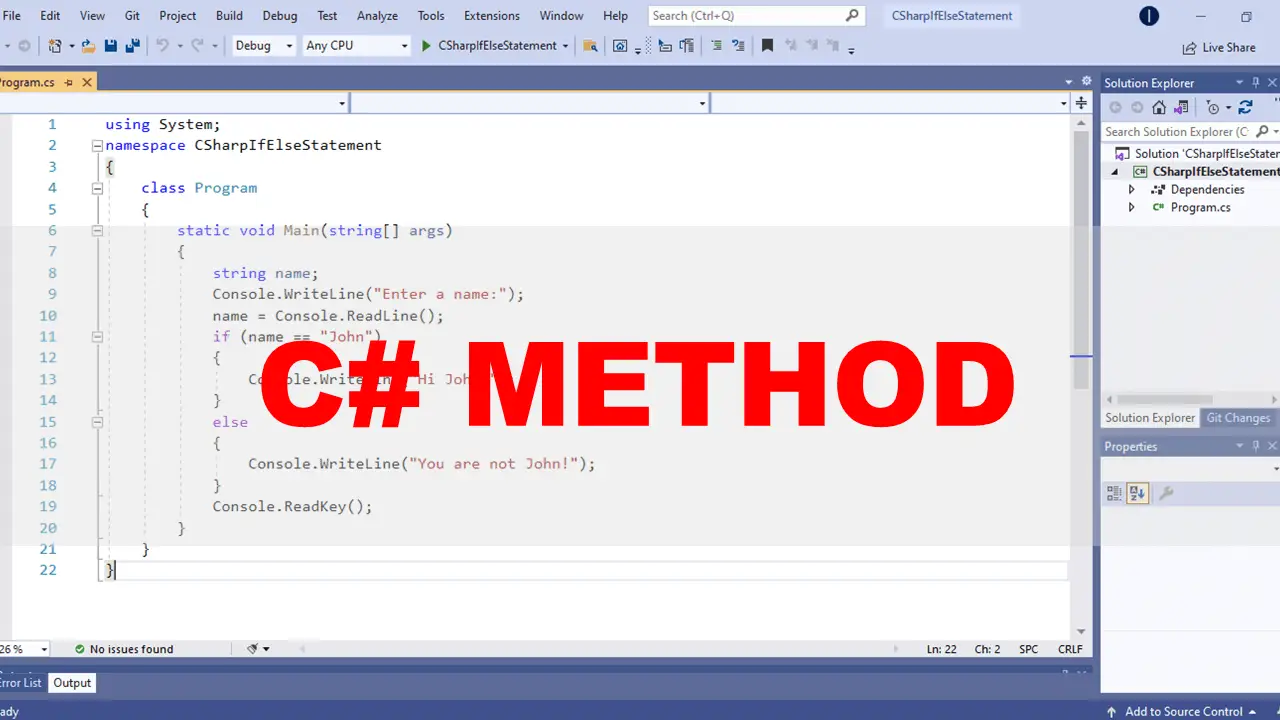
Objectives
By the end of this lesson, you should
- Explain how method works
- Explain the components of methods
- Design and Create a program that implements the use of methods
Lesson Proper
- A method is a block of code that only executes when it is invoked by the user.
- You can pass data into a method, which is referred to as passing parameters.
- Methods are used to conduct specific actions, and they are also referred to as functions.
- What is the point of using methods?
- To reuse code, first define the code once and then utilize it numerous times throughout the program.
Method Declaration
A method declaration in C# consists of the following components, which are listed below:
Modifier: It describes the access type of the method and the location in your application from which it can be accessed. There are three types of access modifiers in C#: Public, Protected, and Private.
The Method Name is: It provides the name of the user-defined method, as well as the way by which the user invokes or refers to it. Eg. GetName()
Return type: This parameter specifies the data type that will be returned by the method. It is dependent on the user because it may also return a void value, which means it will return nothing.
The Method’s Body: It refers to the line of code that contains the tasks that the method will complete while it is being executed. It is contained within the braces.
List of parameters: Within the enclosing parenthesis, a comma-separated list of the input parameters is defined, each of which is preceded by the data type for which it is intended. Empty parenthesis () must be used if there are no arguments to pass in the code.
Method Signature
A method signature is a way for the C# compiler to identify a method by its unique identifier.
Method Naming: The name of a method or a function in any programming language, whether in C++, Java, or C#, is extremely important and is mostly utilized in order to call that method for its execution, among other things.
For example, findSum, computeMax, setX and getX etc. There are certain pre-defined rules for naming methods which a user should follow :
- The method name must be some kind of Noun or a verb.
- It’s naming should be done in such a way that it must describe the purpose of that method.
- The first letter of the method name can be either a small letter or a Capital letter, however, it is recommended to use the capital one.
These rules are not mandatory, but recommendable. Generally, a method has a unique name within the class in which it is defined but sometime a method might have the same name as other method names within the same class as method overloading is allowed in C#.
Method Calling
Method Invocation or Method Calling is done when the user wants to execute the method. The method needs to be called for using its functionality. To call (execute) a method, write the method’s name followed by two parentheses () and a semicolon;
How it works
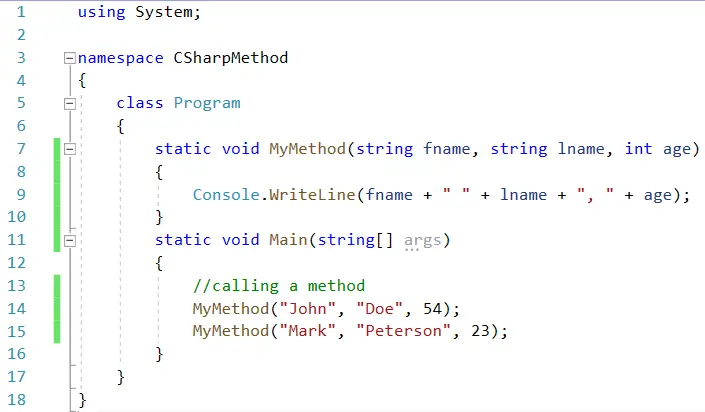
Line 1 – To add namespaces and class files, we commonly use the using keyword. It then makes all of the classes, interfaces, and abstract classes, as well as their methods and properties, available on the current page. Console is a member function or method in the System namespace. Because we need the console function to show and read user inputs, we must include it on the first line of our program.
Line 3 – The line specifies the name of the program, which is CSharpMethod in this case.
In this example source code, our class name is Program which consists of two methods, the MyMethod() and the Main().
Line 7-10 – this is the scope of our first method which is the MyMethod().
Line 7 – this is the start of our method declaration.
MyMethod is the name of the method. It has 3 parameters; fname and lname are of string type and age of integer type.
Line 9 – is the body of our method. The function of this method is to print the first name, last name and age.
Line 11-16 – this is the scope of the second method which is the Main() method. In C#, the entry point is the main method.
Line 14 – this line is a method call with the values for our 3 parameters. Take note that the number of values in the method call should match the number of parameters of the method. If the method as three parameters, the values in the method call should also be three.
Line 15 – another method call with different values.
using System; namespace CSharpMethod { class Program { static void MyMethod(string fname, string lname, int age) { Console.WriteLine(fname + " " + lname + ", " + age); } static void Main(string[] args) { //calling a method MyMethod("John", "Doe", 54); MyMethod("Mark", "Peterson", 23); } } }
Video Tutorial
Summary
Method is crucial in computer programming since it is what helps you to structure your code and makes it more legible. It also makes your code more reusable, which might save you time in the long run. Additionally, well-defined methods might help to avoid problems in your code. Finally, using methods might help to make your code more efficient. Overall, methods are necessary for producing quality code. If you want to be successful in programming, you need to learn how to use methods efficiently. Fortunately, there are many tools accessible online that may help you gain a firm understanding of this vital part of coding. Keep in mind that it is crucial to use the proper method for the right task – if you don’t know what method to use, visit a credible resource. Finally, always be careful to test your code before you release it into production. This will enable you to avoid any potential mistakes and guarantee that your software is working as planned.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
For the step by step tutorial on how to create a sample program on method in C#, please watch the video uploaded on our YouTube Channel.
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
C# For Loop Statement Video Tutorial and Source code
Array in C# Video Tutorial and Source code
C# While Loop Statement Video Tutorial and Source code
C# NESTED IF Statement Video Tutorial and Source code
C# IF ELSE IF Statement Video Tutorial and Source code