Password Generator in C# Console
Introduction
Table of Contents
In today’s digital world, passwords are our frontline defense against unauthorized access to our personal information. From bank accounts to social media profiles, strong passwords act as the gatekeepers, safeguarding our valuable data. But let’s face it, relying on memory alone to create and manage diverse, complex passwords for every platform is a recipe for disaster. Thankfully, the world of coding offers a powerful solution: password generators.
Forget the days of predictable patterns and recycled birthdays! By harnessing the magic of C# and loops, we can write our own password generator software, crafting unique, random passwords tailored to our specific needs. No more struggling to remember intricate combinations, no more compromising security with easily guessable words.
But why is this so important?
- The Peril of Weak Passwords:
Imagine your favorite online haven, protected by a flimsy lock that anyone can pick. That’s essentially what happens when we use weak passwords. Hackers are constantly employing sophisticated techniques to crack simple passwords, potentially exposing our sensitive information to theft and misuse. Weak passwords are the chink in our digital armor, leaving us vulnerable to identity theft, financial loss, and even reputational damage.
- Beyond Human Limitations:
Let’s be honest, creating truly strong passwords that are both complex and memorable is a challenge. We resort to predictable patterns, reuse old passwords, or rely on personal details that compromise security. Password generators address these limitations by leveraging the power of randomness and algorithms. They can generate long, complex passwords incorporating a variety of characters, making them virtually impossible to guess, even for the most determined attackers.
- Your Personal Security Ally:
This C# lesson equips you with the tools to build your own password generator, empowering you to take control of your online security. You’ll learn how to:
- Define the desired password length and complexity.
- Utilize loops and string manipulation techniques in C# to generate random passwords.
- Craft passwords that meet specific criteria, incorporating uppercase letters, lowercase letters, numbers, and symbols.
Objectives
This lesson aims to provide learners with a comprehensive understanding of the importance of strong passwords in the realm of online security. Participants will learn the limitations associated with manual password creation and the potential risks involved. Through hands-on practice and application, students will gain proficiency in developing a Password Generator in C# Console. The objectives encompass not only understanding the significance of secure passwords but also acquiring the practical skills needed to implement an efficient password generator, enhancing both knowledge and application capabilities.
Source code example
using System; using System.Text; class PasswordGenerator { static void Main() { Console.WriteLine("iNetTutor.com - Welcome to the Password Generator!"); Console.Write("Enter the desired password length: "); int length = int.Parse(Console.ReadLine()); Console.WriteLine("Select the character types to include:"); Console.WriteLine("1. Uppercase letters"); Console.WriteLine("2. Lowercase letters"); Console.WriteLine("3. Digits"); Console.WriteLine("4. Special characters"); Console.WriteLine("Enter the options (e.g., 1234): "); string options = Console.ReadLine(); string uppercaseLetters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; string lowercaseLetters = "abcdefghijklmnopqrstuvwxyz"; string digits = "0123456789"; string specialCharacters = "!@#$%^&*()_+-="; StringBuilder password = new StringBuilder(); Random random = new Random(); while (password.Length < length) { if (options.Contains("1")) password.Append(uppercaseLetters[random.Next(uppercaseLetters.Length)]); if (options.Contains("2")) password.Append(lowercaseLetters[random.Next(lowercaseLetters.Length)]); if (options.Contains("3")) password.Append(digits[random.Next(digits.Length)]); if (options.Contains("4")) password.Append(specialCharacters[random.Next(specialCharacters.Length)]); } Console.WriteLine("Generated Password: " + password); Console.ReadKey(); } }
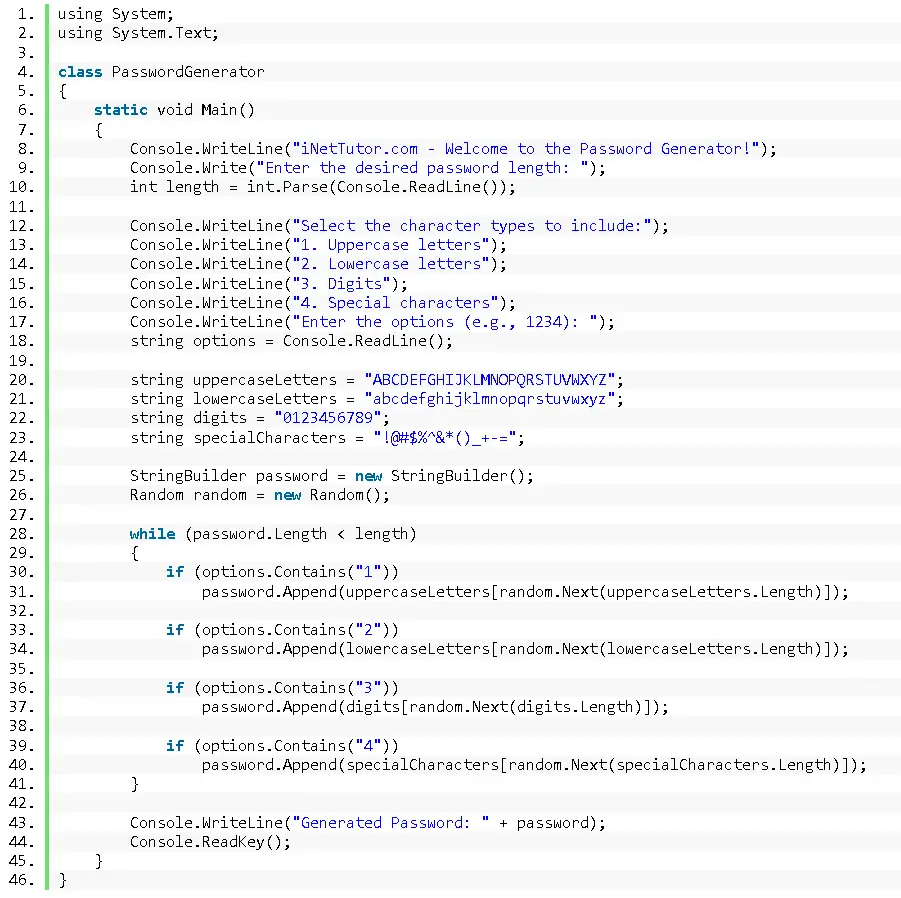
Explanation
This source code is for a password generator in C# Console. Let’s go through the code step by step:
- The code begins with the necessary using directives using System; and using System.Text; to include the required namespaces for Console input/output and StringBuilder.
- Next, a class named PasswordGenerator is defined.
- Inside the class, the Main method is declared as static void Main(). This is the entry point of the program.
- The program starts by displaying a welcome message and prompts the user to enter the desired password length using Console.Write and Console.ReadLine. The input is stored in the length variable as an integer.
- Then, the program displays a menu of character types to include in the password, such as uppercase letters, lowercase letters, digits, and special characters. The user is asked to enter the options (e.g., “1234”) to select the desired character types. The input is stored in the options variable as a string.
- Next, four string variables uppercaseLetters, lowercaseLetters, digits, and specialCharacters are defined. These variables hold the characters from which the password will be generated.
- The program creates a StringBuilder object named password to build the generated password.
- It also creates a Random object named random to generate random indices for selecting characters from the character type strings.
- Inside the while loop, the program checks if the length of the password is less than the desired length. If it is, it randomly selects characters from the character type strings based on the selected options using random.Next() and StringBuilder.Append().
- Finally, the generated password is displayed using Console.WriteLine along with the welcome message. Console.ReadKey() is used to wait for a key press before the program exits.
This code generates a random password by combining characters from the selected character types and ensures that the password length matches the desired length specified by the user.
Output
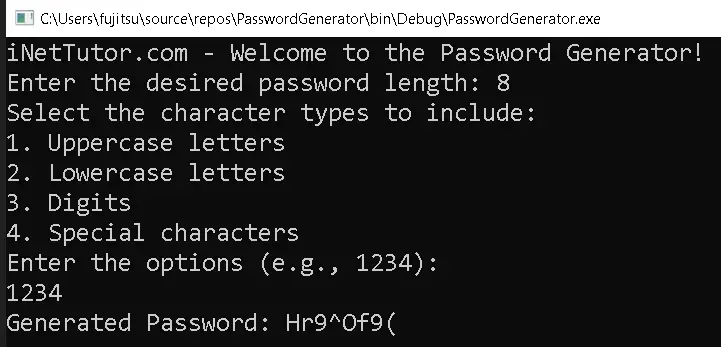
Summary
In this lesson, we explored the concept of password generation in C# Console. We began by understanding the importance of strong passwords in online security and the limitations of manually creating them. To overcome these challenges, we introduced the concept of password generators, which can automate the process of generating secure passwords with specified lengths and character types.
Throughout the lesson, we learned how to implement loops and string manipulation techniques to create a password generator in C# Console. By utilizing the capabilities of the StringBuilder class and the Random class, we were able to generate random passwords that meet the user’s requirements.
By prompting the user to enter the desired password length and select the character types to include, we provided a customizable experience that allows users to generate passwords tailored to their specific needs. The generated passwords can include uppercase and lowercase letters, digits, and special characters.
Through this lesson, we emphasized the significance of strong passwords in enhancing online security. Weak and easily guessable passwords can leave our sensitive information vulnerable to cyber threats. Therefore, the ability to generate strong and unique passwords using password generators is a valuable skill for protecting our online accounts and personal data.
By gaining a comprehensive understanding of password generation in C# Console, you are now equipped with the knowledge and skills to create your own password generator. This lesson has empowered you to automate the process of generating secure passwords, enabling you to bolster your digital security and safeguard your online presence.
Exercises and Assessment
Lab Exam: Password Generator Enhancement To further enhance the password generator project, you can consider the following lab exam:
- Task: Implement a password strength checker
- Extend the existing password generator code to include a password strength checker.
- The password strength checker should evaluate the generated password and provide a rating or score based on its strength.
- Consider factors such as password length, character complexity, and the presence of uppercase letters, lowercase letters, digits, and special characters.
- Display the password strength rating to the user along with the generated password.
- Task: Implement user preferences
- Modify the password generator code to allow users to set their preferences for password length and character types.
- Prompt the user to enter their desired password length and select the character types they want to include.
- Validate the user input to ensure it meets the required criteria.
- Generate a password based on the user’s preferences and display it to the user.
- Task: Add password history and uniqueness check
- Enhance the password generator to keep track of generated passwords in a history log.
- Implement a check to ensure that each generated password is unique and has not been previously generated.
- Display the password history to the user, allowing them to view their previously generated passwords.
These lab exam tasks will challenge you to extend the functionality of the password generator, improve user experience, and reinforce your understanding of C# Console programming concepts.
Related Topics and Articles:
Password Validation with Retry Limit in C#
Encrypt and Decrypt Password Using C#
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.