Email Validation in PHP Free Source code and Tutorial
Introduction
Table of Contents
An email validation script is a script that is used to verify the validity of an email address. In order to guarantee that email addresses are valid and exist, or to ensure that they follow a specific format.
In this tutorial, we will be using PHP to create a script for verifying and validating email address. You can download the source code for reference and guide and we will also provide a video presentation with explanation on how the script will work and function.
The following are the prerequisites for participating in this tutorial:
- XAMPP
- Text editor (VS Code, Sublime, Brackets), download and install a text editor of your choice
Objectives
By the end of this tutorial, you will be able to:
- Create a PHP script that will check, verify and validate whether an email address is well-formed.
- Become familiar with the PHP functions that are required in order to develop an email validation script.
- filter_var()
- FILTER_SANITIZE_EMAIL
- FILTER_VALIDATE_EMAIL
- To integrate and apply the source code in your projects
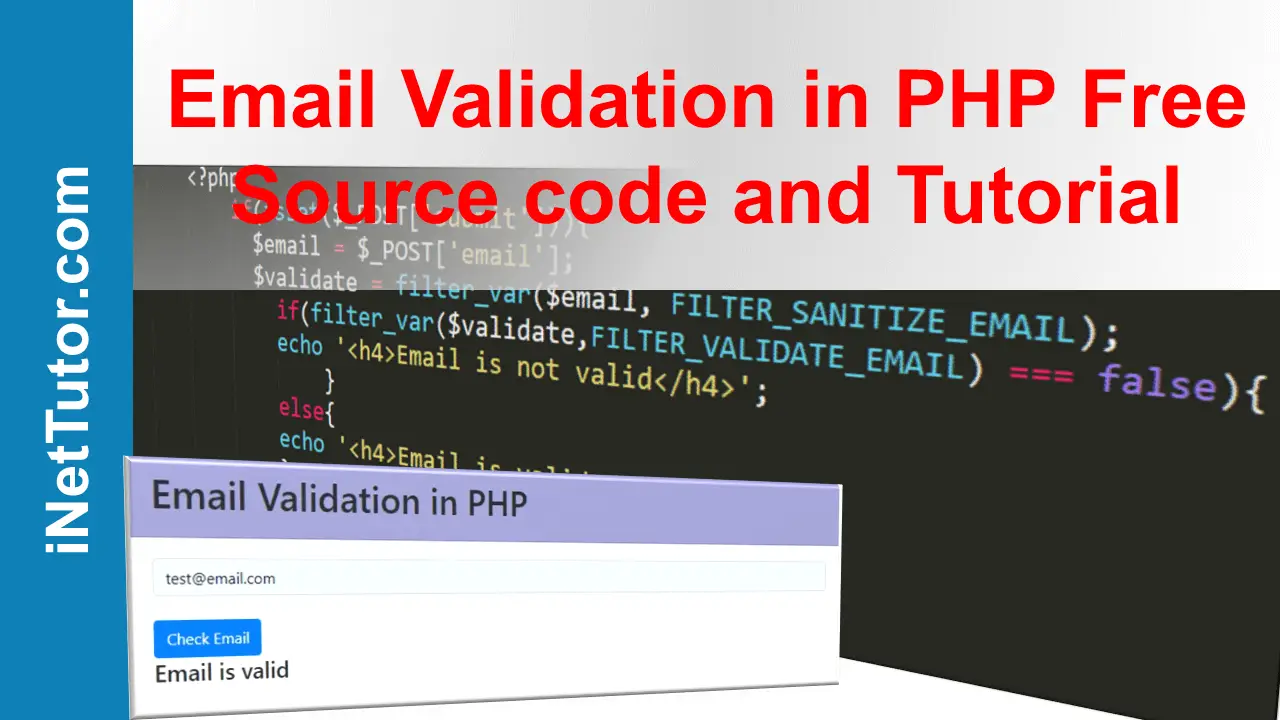
Source code
This code serves as an email validation check. It will check to see if the email address you entered is valid. If the email address is invalid, for example, if it does not contain a @ symbol, the script will display a message stating that the email address is invalid.
This is an example PHP script for email validation.
<?php if(isset($_POST['submit'])){ $email = $_POST['email']; $validate = filter_var($email, FILTER_SANITIZE_EMAIL); if(filter_var($validate,FILTER_VALIDATE_EMAIL) === false){ echo '<h4>Email is not valid</h4>'; } else{ echo '<h4>Email is valid</h4>'; } } ?>
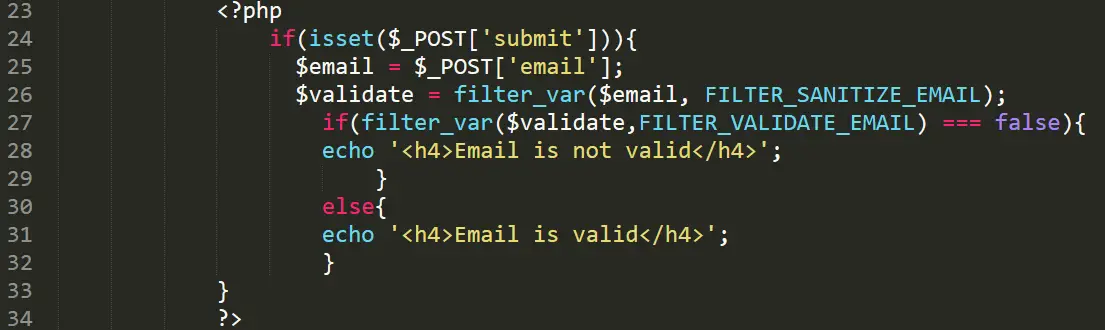
Explanation
The script will start at line 23 and end at line 34. PHP script will start at <?php and the closing tag is ?>.
Line 24 – we have declared an if statement with isset() function.
isset() function – This function tests whether a variable has been set and is not NULL. It is included in PHP and can be used to check if a variable has been set and is not NULL. If a defined variable, array, or array key has a null value, isset() returns false; otherwise, it returns true.
Line 25 – $email is the variable that will hold the data of email field when the user clicks the submit button.
Line 26 – we have a variable $validate that will hold the sanitize and validated value of $email.
filter_var() – The filter var() function filters a variable using the filter that has been specified. This function is used to validate and sanitize the data at the same time.
filter_var(var, filtername, options) – it has 3 parameters, var is a required field or parameter for it will be the variable that will sanitized. In our case it is the $email. The filtername parameter is used to specify the ID or name of the filter to use.
Filter name ID used in our tutorial is FILTER_SANITIZE_EMAIL – Remove all illegal characters from an email address
Line 27-32 – we have set an if else statement and used filter_var() with the filtername of FILTER_SANITIZE_EMAIL which is used to check and validate if the email address entered by the user is a valid email address. If the input is valid then it will have a message of Email is valid if not, a message of Email is not valid will appear.
Output
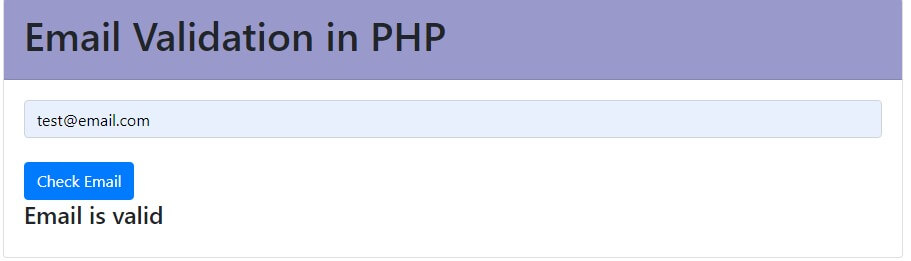
Summary
The purpose of email address validation is to ensure that the email address is valid and exists. Email address sanitization is the process of removing potentially harmful content from an email address, such as embedded links or scripts.
It is important to validate and sanitize email addresses because invalid or unsafe email addresses can lead to spam, malware, and phishing attacks. Invalid email addresses can also cause problems when sending and receiving emails, such as not being able to send or receive messages because the recipient’s email address is invalid.
Email address validation and sanitization can be done using a variety of methods, including:
- validating an email address using a validated third-party service
- checking an email address against a list of known bad or invalid addresses
- removing potentially harmful content from an email address, such as embedded links or scripts
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
How to Generate Random Password in PHP Free Source code and Tutorial
Image Gallery Web Application in Bootstrap Free Source code
Law Office Management Information System in Bootstrap and PHP Script
Cloud-Based Farm Management Assistant System Free Bootstrap Source code
Online Birth Certificate Processing System with SMS Notification Free Bootstrap Template