How to Generate Random Password in PHP Free Source code and Tutorial
Introduction
Table of Contents
A password is a word or phrase used to control access to a computer system, network, or data. As part of security measure, password must be a combination of letters, number and special characters. Passwords are used a lot in web, desktop and mobile applications. In this tutorial, you will learn how to generate random passwords in PHP.
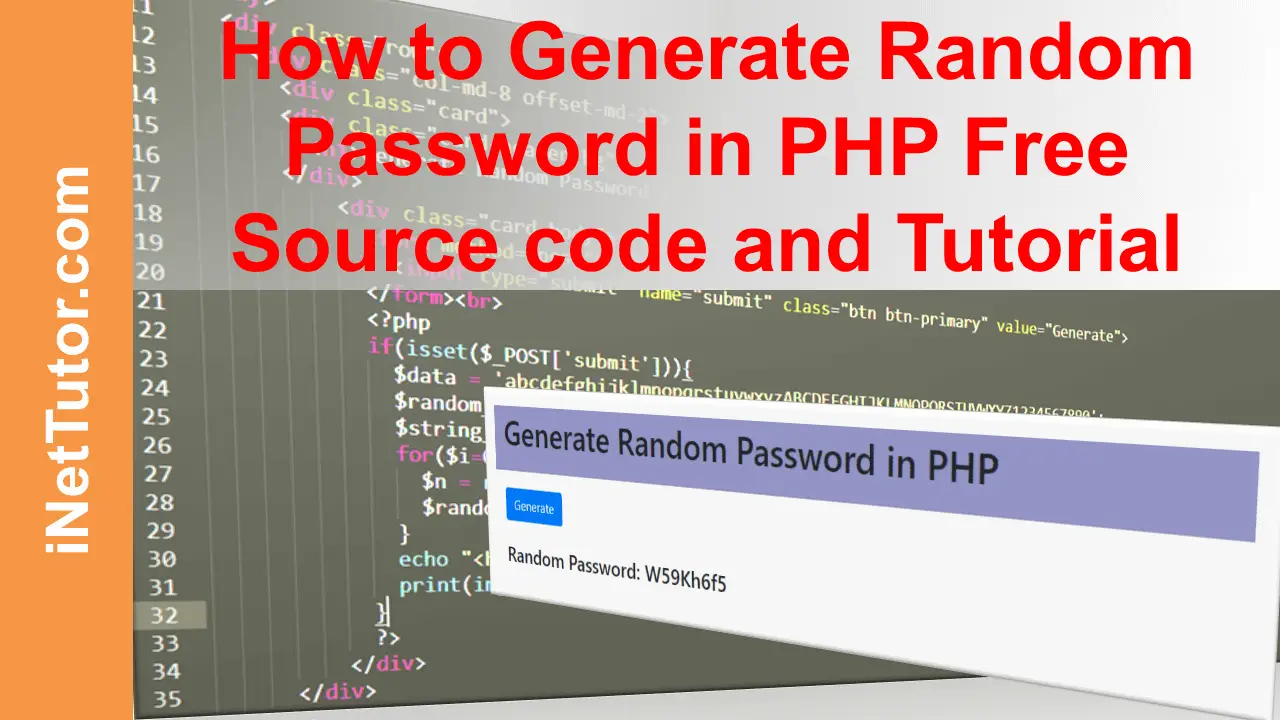
The following are the requirements for this tutorial:
- XAMPP
- Text editor (VS Code, Sublime, Brackets), download and install a text editor of your choice
Objectives
By the end of this tutorial, you will be able to:
- Create a PHP script that generates random string or password
- Understand the different functions used in order to create a random password or string in PHP
- array()
- strlen()
- rand()
- implode()
- To integrate and apply the source code in your projects
Source code
<?php if(isset($_POST['submit'])){ $data = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890'; $random_pass = array(); $string_variable = strlen($data) - 1; for($i=0; $i<8; $i++){ $n = rand(0,$string_variable); $random_pass[] = $data[$n]; } echo "<h4>Random Password: "; print(implode($random_pass)); } ?>
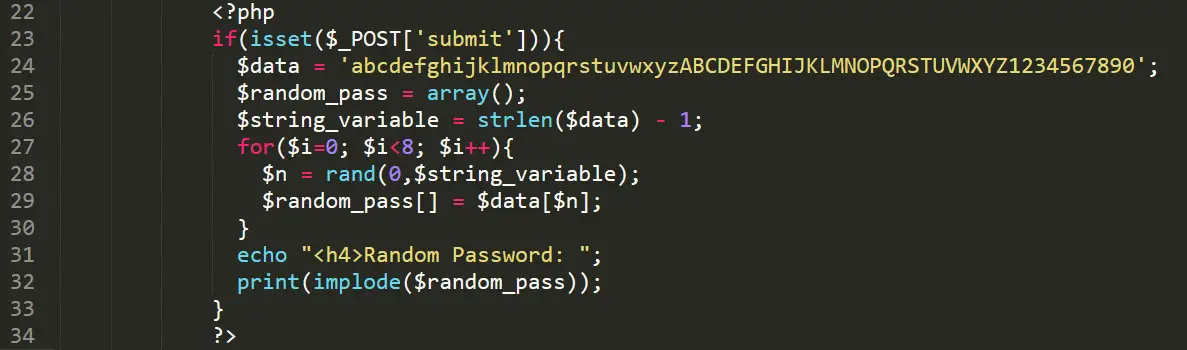
Explanation
The script will start at line 22 and end at line 34.
Line 23 – PHP $_POST is a PHP super global variable which is used to collect form data after submitting an HTML form with method=”post”. $_POST is also widely used to pass variables.
Line 24 – next, we have declared a variable $data that will handle or store the string which consists of lowercase, uppercase letters and number from 1-10. This will be the source of our random password. You may also include special characters to make our generated password more strong and secure.
Line 25 – create a variable with an array() function.
The array() function is used to create an array.
In PHP, there are three types of arrays:
- Indexed arrays – Arrays with numeric index
- Associative arrays – Arrays with named keys
- Multidimensional arrays – Arrays containing one or more arrays
Line 26 – $string_variable is a variable that will hold and store the length of the string in $data
The strlen() function in PHP returns the length of a specified string.
Line 27-30 – this is the for loop that will run or iterate for 8 times. It means that the generated password is 8 characters in length, you are free to modify it based on the number of characters you want.
We all know that the for loop has 4 components.
- Initialization – $i=0;
- Conditional statement – $i<8;
- Loop body:
$n = rand(0,$string_variable);
$random_pass[] = $data[$n];
- Update statement – $i++
It is possible to get a random integer value from the rand() function in PHP. This function can be used to get a random integer value from the range [min, max].
Line 31 – it will echo the label Random Password:
The echo() function outputs one or more strings.
Line 32 – it will print the 8 characters random generated string or password.
print() It is used to print one or more strings. It is not actually a real function, it is a language construct. So, there is no need to put parentheses around its list of arguments.
implode() function returns a string from the elements of an array $random_pass.
Output
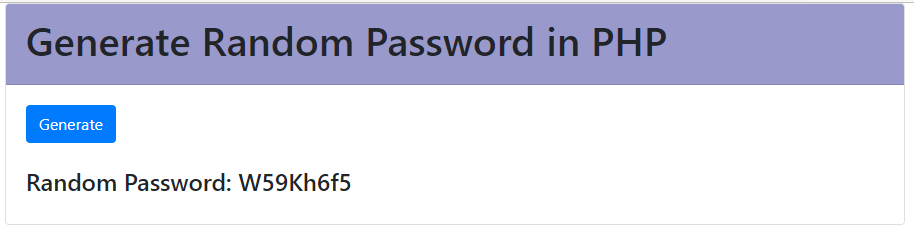
Summary
When logging into a computer system, network, or data, a password is a word or phrase that is used to control access. In order to ensure that only authorized users have access to a system or data, passwords are used. Individual users are identified through the use of passwords in conjunction with user IDs.
When creating a password, make it at least 8 characters long and include a mix of letters, numbers, and symbols. A strong password is less likely to be guess or hacked than a weak password.
With this article and tutorial, you have used different functions in PHP in order to generate random strings and password.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
Basic Ecommerce Website in Django Free Source code
Medicine Delivery Web App Free Download Template in PHP and Bootstrap
File Management System Free Template in Bootstrap and PHP
Online Job Portal using Django Web Framework Free Source code
Face Recognition Attendance System Application Free Download Bootstrap and PHP Script