Positive Negative Number Checker in CSharp
Introduction of the lesson
Table of Contents
Welcome to an engaging C# programming lesson centered on crafting a console program that discerns whether a user-entered number is positive, negative, or zero. This tutorial delves into the core principles of user input management, conditional logic, and output generation within the context of C# programming. As we embark on this learning journey, participants will gain valuable insights into soliciting and validating user input, laying a foundation for robust program behavior. The exploration of conditional statements, particularly the if-else construct, serves as a key component, offering a nuanced understanding of decision-making structures. The lesson culminates in the creation of a practical C# program, where learners will witness the seamless integration of these concepts to deliver tailored output messages based on user input. Brace yourself for a hands-on experience, honing essential skills that extend beyond this lesson into broader programming scenarios. Let’s dive in and unravel the intricacies of building responsive and intelligent C# applications!
Objectives of the lesson
In this lesson our primary goal is to empower you with fundamental skills in user input management, conditional logic, and output generation. Throughout this tutorial, you’ll embark on a journey to create a dynamic console program that evaluates user-entered numbers, determining whether they are positive, negative, or zero. The lesson is designed to provide hands-on experience, offering a blend of theoretical understanding and practical application.
- User Input Handling:
- Delve into the basics of soliciting user input, a fundamental aspect of interactive programming.
- Master the usage of double.TryParse for effectively handling numeric input, ensuring robust program behavior.
- Conditional Logic:
- Implement if-else statements, the cornerstone of decision-making in programming, to discern the nature of user-entered numbers.
- Gain a nuanced understanding of conditional structures for efficient and logical program flow.
- Output Generation:
- Explore the art of crafting meaningful and informative output messages based on the evaluation of user input.
- Develop the skills to tailor program responses to different scenarios, enhancing user interaction.
- Error Handling:
- Integrate robust error-handling mechanisms, providing a graceful approach to manage and respond to invalid inputs.
- Strengthen your programming prowess by ensuring the reliability and resilience of your C# applications.
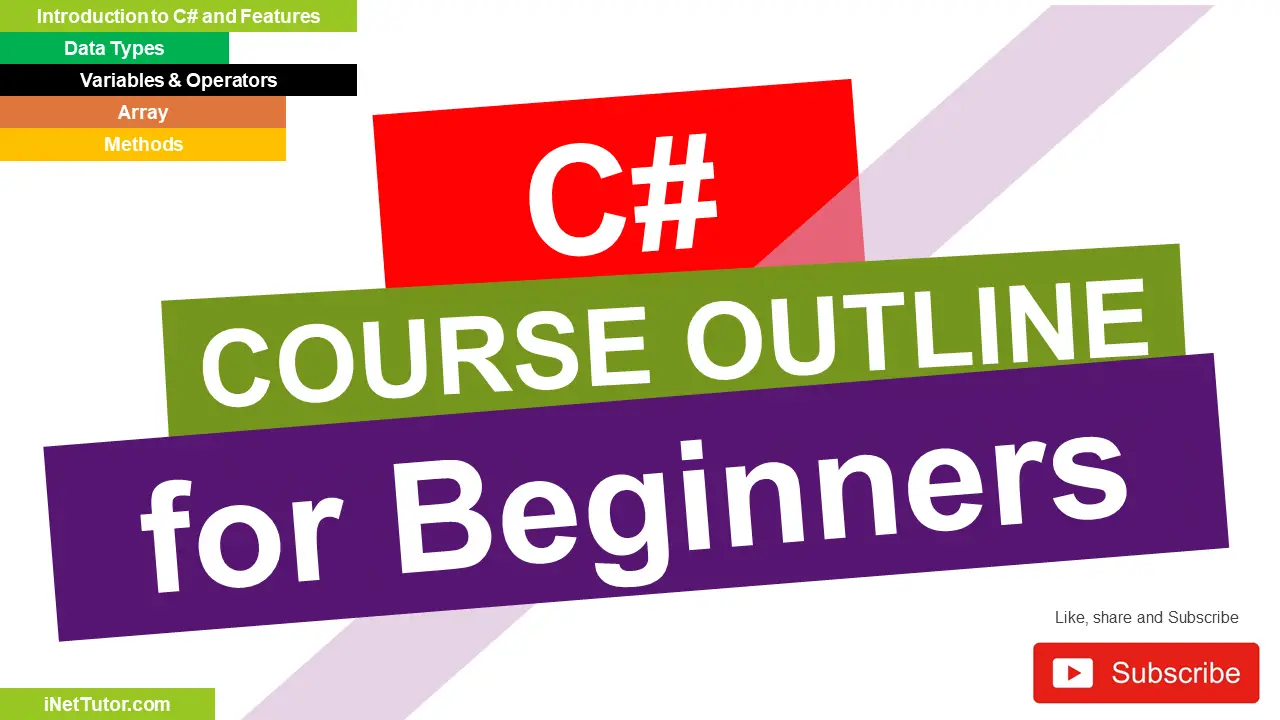
Source code example
using System; namespace PostiveNegativeChecker { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Enter a number:"); if (double.TryParse(Console.ReadLine(), out double number)) { if (number > 0) { Console.WriteLine("The number is positive."); } else if (number < 0) { Console.WriteLine("The number is negative."); } else { Console.WriteLine("The number is zero."); } } else { Console.WriteLine("Invalid input. Please enter a valid number."); } Console.WriteLine("Press any key in the keyboard to close the console."); Console.ReadKey(); } } }
Source code explanation
In our program, we’re creating something like a smart assistant. It will talk to you, and when you tell it a number, it will do some clever thinking and tell you if that number is positive (like 5), negative (like -3), or zero (like 0).
Here’s how it works:
- Asking for a Number:
- Imagine you’re talking to a friend, and you want them to tell you a number. The program does the same – it politely asks you to give it a number.
- Understanding Your Number:
- Now, our program is smart but careful. It wants to make sure you give it a proper number, not something strange. It uses a trick called double.TryParse to check if the number you said is a real number (like 7.5) or not.
- Smart Decision Time:
- Once it knows you’ve given a real number, it thinks for a moment. It uses a special trick called ‘if-else’ to decide whether your number is positive, negative, or zero. It’s like your friend saying, “Oh, that’s a big number!” or “Oh, that’s a small number!”
- Talking Back to You:
- After doing its thinking, our program talks back to you. It says something nice, like “Your number is positive!” or “Your number is zero.” But, if you try to trick it and say something weird, it’ll tell you, “Hey, that’s not a proper number! Tell me a real one.”
So, it’s like having a little helper that understands numbers and can chat with you about them!
Summary and Conclusion
Summary:
In this lesson, we embarked on a journey to create a clever program in C# that can determine whether a number is positive, negative, or zero. We started by understanding the basics of user input – it’s like asking a friend for a number. The program then showed its smart side by checking if the number you gave was a proper one using a trick called double.TryParse. After that, it thought for a moment using ‘if-else’ statements to decide if your number is big, small, or zero. Finally, it talked back to you, saying something nice about your number.
Key Objectives Achieved:
- User Input Magic:
- Explored the art of asking for a number and ensuring it’s a real, proper number.
- Smart Decision-Making:
- Unveiled the secrets of ‘if-else’ statements, making our program clever enough to decide the nature of your number.
- Friendly Conversations:
- Showed how the program can talk back to you, giving friendly messages about your number.
Conclusion:
In wrapping up our adventure, we’ve learned that programming is a bit like having a helpful friend who understands numbers and can chat with us about them. Understanding how to handle what people tell our program and making smart decisions based on that information is like teaching our friend to be even more helpful. So, keep practicing, keep learning, and soon you’ll be creating programs that are not just smart but also great at chatting with you! Happy coding!
Exercises
Now that you’ve successfully crafted a program that can cleverly analyze whether a number is positive, negative, or zero, it’s time to elevate your skills through hands-on exercises. These exercises are designed to provide you with practical challenges, further solidifying your understanding of user input handling, conditional statements, and output generation in C#. Each exercise presents a unique scenario, encouraging you to explore additional functionalities, handle custom inputs, and refine your error-handling mechanisms. Embrace these exercises as opportunities to apply your newfound knowledge in diverse programming situations, reinforcing your ability to create responsive and intelligent C# applications.
- Decimal Numbers:
- Modify the program to handle decimal numbers (e.g., 5.7). Ensure it correctly identifies positive, negative, or zero decimals.
- User-defined Ranges:
- Allow users to define their own ranges (e.g., positive numbers between 10 and 20). Update the program to accommodate these custom ranges.
- Extended Outputs:
- Enhance the program to provide more descriptive outputs. Instead of just saying “positive,” specify if the number is “small positive” or “large positive.”
- Interactive Conversations:
- Extend the conversation with the user. After evaluating the number, ask if they’d like to input another number and continue the conversation.
- Multi-Number Analysis:
- Allow users to input multiple numbers at once, and analyze each one. Display a summary of how many were positive, negative, or zero.
- Enhanced Error Handling:
- Improve error handling by providing specific feedback on what went wrong. For example, if the user enters a word instead of a number, suggest they enter a valid number.
Related Topics and Articles:
C# IF ELSE IF Statement Video Tutorial and Source code
C# IF ELSE Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.