Number Comparison in CSharp
Introduction of the lesson
Table of Contents
Welcome to our blog post or lesson on number comparison! In this tutorial, we will explore how to write a C# program that prompts the user to enter two numbers and displays the larger number using an if-else statement. This program is a fundamental exercise in programming logic and decision-making.
Whether you are a beginner learning C# or an experienced programmer looking to refresh your skills, understanding how to compare numbers is an essential concept in many programming tasks. By the end of this tutorial, you will have a solid understanding of how to use if-else statements to compare numbers and determine the larger value.
Before we dive into the code, let’s briefly discuss the importance of comparison operations in programming. Comparing numbers allows us to make decisions based on certain conditions, enabling our programs to perform different actions depending on the values provided by the user or calculated within the program.
Now, let’s get started with writing the C# program that prompts the user to enter two numbers and displays the larger number using the if-else statement. By following along with this tutorial, you will gain practical experience in implementing conditional logic in your programs.
Objectives of the lesson
In this lesson, we will explore the concept of comparing numbers and learn how to write a C# program that prompts the user to enter two numbers and displays the larger number using an if-else statement.
Objectives:
- Understand the importance of comparison operations in programming.
- Learn how to prompt the user for input in C#.
- Gain proficiency in using if-else statements to compare numbers.
- Develop problem-solving skills by implementing conditional logic in a practical scenario.
- Strengthen your understanding of basic programming principles, such as variables and data types.
By the end of this lesson, you will have a solid foundation in number comparison and be able to apply this knowledge to various programming tasks. Whether you are a beginner starting your programming journey or an experienced programmer looking to expand your skills, this lesson is designed to provide you with practical experience and understanding of how to compare numbers and make decisions based on their values.
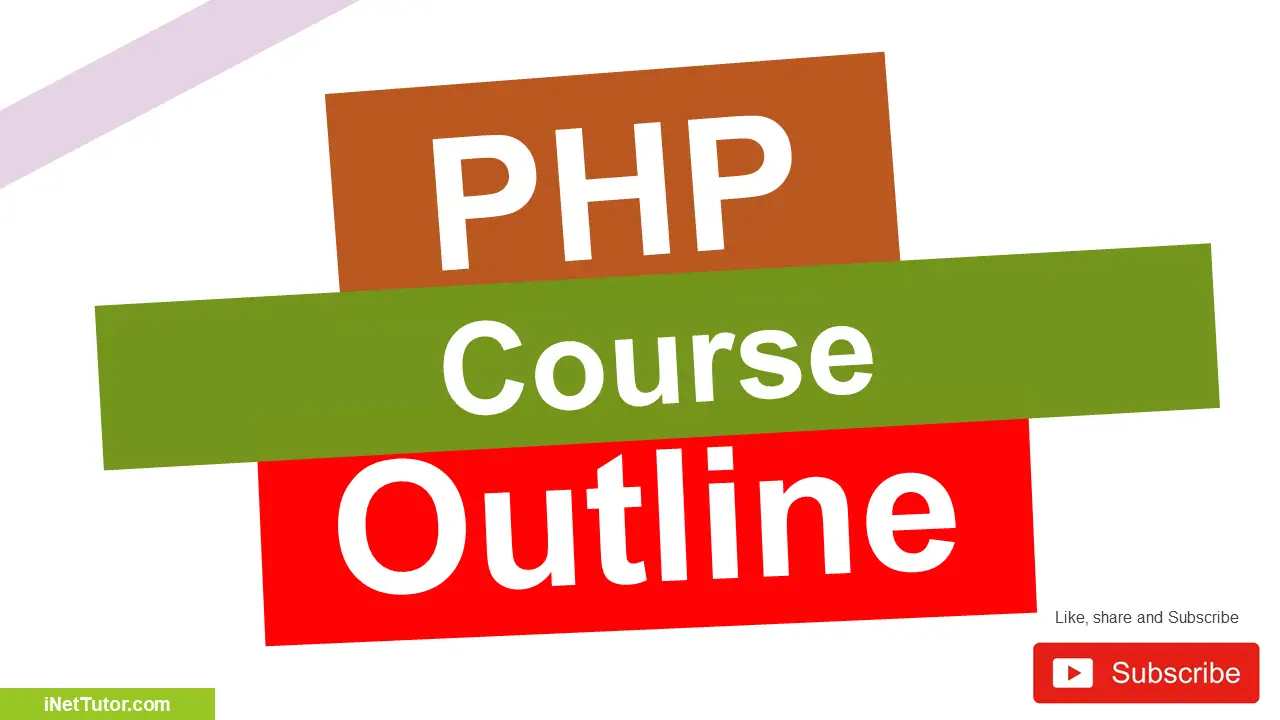
Source code example
using System; namespace NumberComparison { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - Number Comparison Program"); // Prompt the user to enter the first number Console.Write("Enter the first number: "); int firstNumber = Convert.ToInt32(Console.ReadLine()); // Prompt the user to enter the second number Console.Write("Enter the second number: "); int secondNumber = Convert.ToInt32(Console.ReadLine()); // Compare the numbers using if-else statement if (firstNumber > secondNumber) { Console.WriteLine("The larger number is: " + firstNumber); } else if (secondNumber > firstNumber) { Console.WriteLine("The larger number is: " + secondNumber); } else { Console.WriteLine("Both numbers are equal."); } Console.Write("Enter any key in the keyboard to close the console."); Console.ReadKey(); } } }
Source code explanation
- The using System; statement is used to import the System namespace, which provides access to the Console class and other essential functionalities.
- The code is wrapped inside the NumberComparison namespace. Namespaces are used to organize code and prevent naming conflicts.
- The Program class is defined within the NumberComparison namespace. This class contains the Main method, which is the entry point of the program.
- Inside the Main method, the line Console.WriteLine(“iNetTutor.com”); displays the text “iNetTutor.com” on the console. This can be considered as a header or introduction to the program.
- The program prompts the user to enter the first number using Console.Write(“Enter the first number: “);. The user’s input is then read as a string using Console.ReadLine() and converted to an integer using Convert.ToInt32() to store it in the firstNumber variable.
- Similarly, the program prompts the user to enter the second number, reads the input, and converts it to an integer, storing it in the secondNumber variable.
- The program uses an if-else statement to compare the two numbers. If the firstNumber is greater than the secondNumber, it displays “The larger number is: ” followed by the value of firstNumber. If the secondNumber is greater, it displays “The larger number is: ” followed by the value of secondNumber. If both numbers are equal, it displays “Both numbers are equal.”
- Finally, the program waits for the user to press any key to close the console window using Console.ReadKey().
This program demonstrates the basic usage of if-else statements and user input in C#. It allows users to compare two numbers and determine the larger value.
Summary and Conclusion
Number comparison is a fundamental concept in programming, and mastering it is crucial for various tasks. By understanding how to compare numbers in C#, we can make informed decisions and perform different actions based on the values provided by the user or calculated within our programs.
In this lesson, we successfully wrote a C# program that prompts the user to enter two numbers and determines the larger value using an if-else statement. We explored the source code step by step, discussing the purpose and functionality of each segment.
Remember, the skills and knowledge gained from this lesson can be applied to numerous programming scenarios. Whether you’re a beginner or an experienced programmer, understanding number comparison in C# is essential for building robust and efficient applications.
Now that you have a solid understanding of number comparison in C#, continue practicing and applying this concept to more complex programming tasks. As you progress in your programming journey, you will encounter various scenarios where number comparison plays a crucial role.
Congratulations on completing this lesson on number comparison in C#! You are now equipped with the skills to compare numbers and make informed decisions in your future programming endeavors. Keep exploring, learning, and coding!
Exercises
To further improve the number comparison program, you can consider the following exercises:
- Input Validation: Add input validation to ensure that the user enters valid numbers. You can check if the input is a valid integer and handle any exceptions that may occur if the user enters invalid input.
- Handling Negative Numbers: Modify the program to handle negative numbers as well. Currently, the program only compares positive numbers. You can add logic to handle negative numbers and display the appropriate message.
- Float or Decimal Numbers: Extend the program to handle floating-point or decimal numbers instead of just integers. You can modify the data type of the variables to double or decimal and update the input and comparison logic accordingly.
- Error Handling: Implement error handling to gracefully handle any exceptions that may occur during the execution of the program. This can include handling scenarios such as division by zero or other arithmetic errors.
- User-Friendly Interface: Enhance the user interface by providing clear instructions and error messages. You can add prompts for the user to retry input if invalid values are entered or display informative messages when an error occurs.
- Looping and Multiple Comparisons: Extend the program to allow the user to compare more than two numbers. You can use loops to repeatedly prompt the user for input and compare the numbers until they choose to exit the program.
Remember, these exercises are meant to challenge you and help you further develop your programming skills. Feel free to explore additional enhancements and modifications to the program based on your learning goals and interests.
Related Topics and Articles:
C# IF ELSE IF Statement Video Tutorial and Source code
Positive Negative Number Checker in CSharp
C# IF ELSE Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.