Understanding Polymorphism in PHP with Examples
Overview
Table of Contents
In the topic of Object-Oriented Programming (OOP), understanding polymorphism is like unlocking a powerful tool for creating flexible and adaptable code. In this lesson, we’ll discuss the basics of PHP polymorphism. We’ll explore its types, dissect real-world examples, and uncover the benefits and limitations of this essential OOP concept. By the end of this lesson, you’ll be equipped with the knowledge and skills to harness the full potential of polymorphism in your PHP projects.
Introduction
In the world of Object-Oriented Programming (OOP), there’s a key idea called “polymorphism.” It’s like having a versatile tool in your coding toolbox that helps your code be flexible and adaptable.
Polymorphism is super important in OOP because it lets your code change its behavior to fit different situations. It’s like speaking a language that allows your code to interact with different things in a flexible way. This language helps your code grow and adjust to new needs.
In this lesson, we’ll dive into PHP polymorphism, where code can take on different forms and behaviors. We’ll look at the different types of polymorphism, explore real-life examples, and learn how to use this powerful tool to make your PHP projects more flexible and adaptable. So, let’s start our journey into the world of OOP in PHP and discover the magic of polymorphism!
Objectives of the lesson
- Achieve Proficiency in PHP Polymorphism: Develop a strong grasp of polymorphism in PHP, allowing you to confidently employ this concept in real-world programming scenarios.
- Distinguish Between Types of Polymorphism: Differentiate and apply compile-time and run-time polymorphism, as well as interface-based polymorphism, providing you with a comprehensive toolkit for code versatility.
- Apply Polymorphism with Precision: Effectively implement polymorphism through hands-on coding exercises and real-world examples, ensuring your ability to utilize this concept in practical applications.
- Evaluate the Utility of Polymorphism: Assess both the advantages and limitations of polymorphism, enabling you to make informed design decisions and optimize code flexibility in your PHP projects.
Compile-Time Polymorphism (Method Overloading):
- Definition: Compile-time polymorphism, also known as method overloading, occurs when you have multiple methods in a class with the same name but different parameters.
- Usage: It’s used when you want a single method to handle different input variations. The specific method to be executed is determined during compilation based on the number or types of arguments provided.
- Example: In PHP, you can have a Calculator class with an add method that can accept two numbers or three numbers, depending on the arguments provided.
In PHP, you cannot have multiple methods with the same name and different parameters (method overloading) as you can in some other programming languages. PHP does not support method overloading based on the number or types of parameters alone.
However, you can achieve similar functionality by defining optional parameters or using variable-length argument lists.
Example
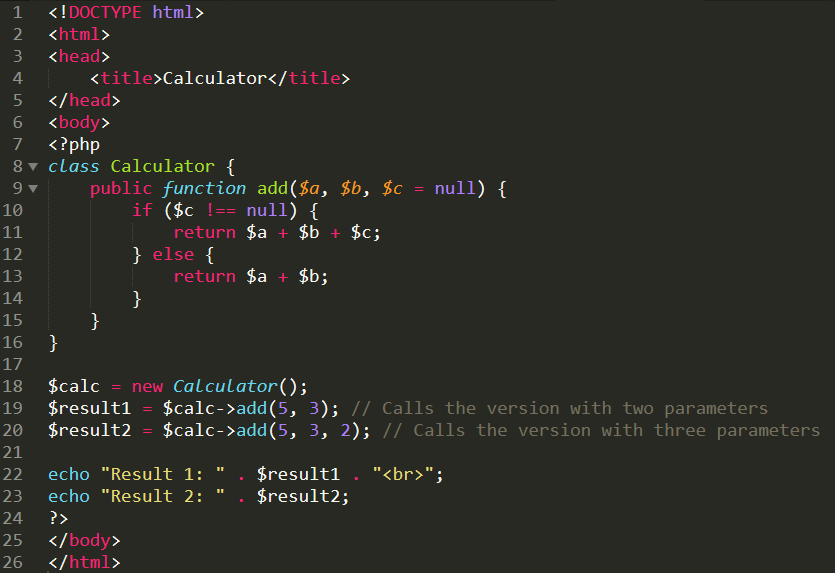
Explanation
In this example, the add method takes two mandatory parameters ($a and $b) and one optional parameter ($c), which is set to null by default. Depending on whether you provide two or three arguments, the method will return the appropriate result.
Output
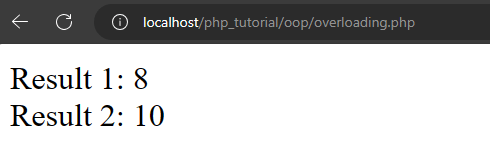
Run-Time Polymorphism (Method Overriding):
- Explanation: Run-time polymorphism, also known as method overriding, occurs when a subclass provides a specific implementation for a method that is already defined in its parent class.
- Significance: It allows you to customize the behavior of a method in a child class while maintaining a common interface with the parent class. This is crucial for achieving flexibility and adaptability in your code.
Example
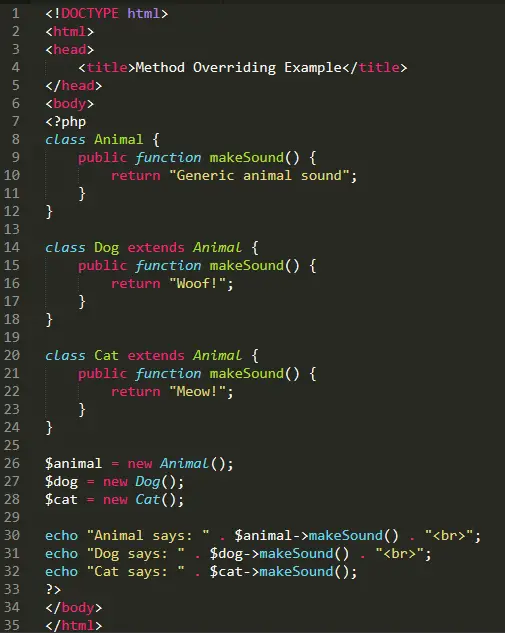
Output
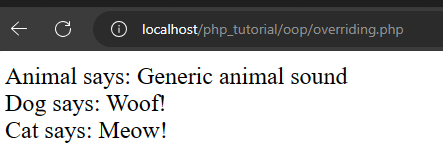
Explanation
This PHP code demonstrates the concept of method overriding in an object-oriented programming (OOP) context. Here’s a brief explanation:
- Three Classes:
- There are three classes defined: Animal, Dog, and Cat.
- Animal is the base class, and Dog and Cat are derived classes that inherit from Animal.
- Method Overriding:
- Each class defines a method named makeSound(). These methods have the same name and exist in both the base class (Animal) and its derived classes (Dog and Cat).
- Method overriding occurs when a derived class provides its own implementation of a method that is already defined in the base class. In this case, both Dog and Cat override the makeSound() method inherited from Animal.
- Object Creation:
- Three objects are created: $animal (an instance of Animal), $dog (an instance of Dog), and $cat (an instance of Cat).
- Method Invocation:
- The makeSound() method is called on each object:
- $animal->makeSound() calls the method from the base class Animal.
- $dog->makeSound() and $cat->makeSound() call the overridden methods in the Dog and Cat classes, respectively.
- The makeSound() method is called on each object:
- Output:
- The code prints out the sound produced by each object:
- “Animal says: Generic animal sound” (from the base class)
- “Dog says: Woof!” (from the Dog class)
- “Cat says: Meow!” (from the Cat class)
- The code prints out the sound produced by each object:
This example demonstrates how method overriding allows derived classes to provide their own specific implementations for methods inherited from a base class, resulting in polymorphic behavior based on the type of object being used.
Benefits and Limitations of Polymorphism
Benefits of Polymorphism:
- Flexibility and Extensibility: Polymorphism enhances code flexibility by allowing you to create generic classes and then extend them with more specialized subclasses. This makes it easier to adapt your code to changing requirements.
- Code Reusability: Polymorphism promotes the reuse of code. You can use a common interface or base class to define behavior, and multiple classes can implement or extend that interface or class, reducing the need for redundant code.
- Ease of Maintenance: When you need to modify the behavior of objects, you can do so in the derived classes without affecting the base class or other parts of the code. This isolation simplifies maintenance.
- Enhanced Readability: Polymorphic code tends to be more readable because it allows you to work with objects in a consistent and abstract manner. You don’t need to know the specific class; you only need to know the interface or base class.
- Polymorphism in PHP: In PHP, polymorphism allows you to create reusable code through interfaces and inheritance. For example, PHP interfaces enable you to define a contract that multiple classes can implement, ensuring consistent behavior across different objects.
Limitations of Polymorphism:
- Complexity: Polymorphism can introduce complexity, especially when you have many classes and inheritance hierarchies. Overusing polymorphism can lead to code that’s difficult to understand and maintain.
- Performance Overhead: While polymorphism enhances code flexibility, it can introduce some performance overhead, especially when dealing with a large number of objects and method calls. This overhead is generally minimal in most applications.
- Design Challenges: Implementing polymorphism correctly requires careful design. It’s essential to strike the right balance between flexibility and maintainability, and it can be challenging to get this balance right, especially in large projects.
- Debugging Complexity: Polymorphic code can be harder to debug because the actual method that gets executed may not be evident from the code that calls it. This can make it more challenging to trace and fix issues.
- PHP-specific Considerations: In PHP, method overloading (compile-time polymorphism) is not directly supported. Achieving similar behavior can be more challenging, as shown earlier. PHP’s dynamic typing can also lead to unexpected behavior if not used carefully.
In conclusion, polymorphism is a powerful concept in OOP that offers numerous benefits, such as flexibility, code reusability, and ease of maintenance. However, it should be used judiciously to avoid complexity and performance issues. In PHP, polymorphism is facilitated through inheritance and interfaces, but it’s essential to be aware of the language-specific considerations.
Summary
Polymorphism is a cornerstone of OOP, offering the ability to create code that adapts to different situations, promotes code reusability, and enhances maintainability. Armed with this knowledge, you’re equipped to create more versatile and efficient PHP applications that can accommodate evolving requirements and maximize code efficiency.
Through practical examples, we observed how polymorphism enhances code readability, reusability, and maintenance while allowing for the creation of versatile and adaptable applications in PHP. Understanding polymorphism is a crucial step in becoming a proficient PHP developer, enabling you to write more elegant and flexible code.
Quiz
Question 1: What is polymorphism in Object-Oriented Programming (OOP)?
- A programming language
B. A design pattern
C. The ability of objects to take on different forms
D. A specific type of variable
Question 2: Which type of polymorphism involves having multiple methods with the same name but different parameters?
- Compile-Time Polymorphism
B. Run-Time Polymorphism
C. Interface-Based Polymorphism
D. Static Polymorphism
Question 3: In PHP, method overriding is associated with which type of polymorphism?
- Compile-Time Polymorphism
B. Run-Time Polymorphism
C. Interface-Based Polymorphism
D. Static Polymorphism
Question 4: What is the primary benefit of method overriding in PHP?
- Improved code efficiency
B. Code reusability
C. Enhanced code security
D. Faster execution
Question 5: Which of the following is NOT a type of polymorphism in PHP?
- Compile-Time Polymorphism
B. Run-Time Polymorphism
C. Inheritance-Based Polymorphism
D. Interface-Based Polymorphism
Question 6: What is the purpose of an interface in PHP?
- To create abstract classes
B. To implement multiple inheritance
C. To define a set of methods that implementing classes must adhere to
D. To hide the implementation details of a class
Question 7: In PHP, which keyword is used to declare a method in a class as “abstract”?
- func
B. abs
C. abstract
D. method
Question 8: What is the main advantage of using polymorphism in PHP?
- Improved code execution speed
B. Simplified debugging
C. Enhanced code reusability and flexibility
D. Stronger data type enforcement
Question 9: Which type of polymorphism allows you to work with objects in a consistent and abstract manner?
- Compile-Time Polymorphism
B. Run-Time Polymorphism
C. Static Polymorphism
D. Interface-Based Polymorphism
Question 10: Which type of polymorphism is also known as “method overloading”?
- Compile-Time Polymorphism
B. Run-Time Polymorphism
C. Static Polymorphism
D. Dynamic Polymorphism
Readers are also interested in:
Understanding PHP Inheritance with Examples
Understanding PHP Encapsulation
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.