Grade Computation in CSharp
In today’s digital age, where education and evaluation have taken center stage, having a reliable tool to calculate grades efficiently is a game-changer. Whether you’re a student looking to keep track of your scores or an educator seeking a convenient grading solution, you’ve come to the right place. In this blog post, we unveil a powerful Grade Computation Program in C# that simplifies the task of calculating final grades. Plus, we’ve got a special treat for you: a free download of the complete source code and a PDF tutorial to guide you every step of the way. Say goodbye to manual grade calculations and hello to precision and speed. Let’s embark on this journey to demystify the art of grade computation with C#—your academic success is just a download away!
Introduction
Grading systems are a fundamental aspect of education, and as a budding programmer, you will find that creating a Grade Computation Program is a valuable exercise in problem-solving and practical application development. Our program will not only compute the final grade but also determine whether the student has passed or failed, enhancing its real-world relevance.
Throughout this lesson, you will gain essential skills, including:
- Variable Handling: Learn how to declare, initialize, and manipulate variables to store and process data effectively.
- Input Validation: Explore techniques for validating user inputs, ensuring that only valid data is accepted.
- Arithmetic Operations: Discover how to perform mathematical calculations, such as weighted averaging, within your C# program.
- Conditional Statements: Understand how to use conditional statements (if-else) to make decisions and control the flow of your program.
- Real-World Relevance: See how programming concepts are applied in practical scenarios, like educational grading systems.
By the end of this lesson, you will have developed a fully functional Grade Computation Program that can calculate final grades based on weighted components and provide immediate feedback on pass or fail status. This project will serve as a solid foundation for your future programming endeavors, demonstrating the power and versatility of the C# programming language in solving real-world problems.
Objectives
Objectives of the lesson on creating a Grade Computation Program in C#, presented in an outcomes-based format:
- Objective: Understand the importance of variables in programming.
- Outcome: Students will recognize the significance of variables as containers for data and essential components in solving real-world problems through programming.
- Objective: Create a C# program to calculate a student’s final grade.
- Outcome: Students will be able to design and implement a C# program that takes input for different grade components, applies weighted averaging, and calculates the final grade accurately.
- Objective: Implement input validation to ensure data integrity.
- Outcome: Students will develop the skill to validate user input effectively, preventing erroneous data from compromising program functionality.
- Objective: Utilize arithmetic operations for weighted averaging.
- Outcome: Students will demonstrate proficiency in performing arithmetic operations to calculate final grades based on given weightings.
- Objective: Incorporate conditional statements to determine pass or fail status.
- Outcome: Students will be able to use conditional statements to evaluate whether a student has passed or failed based on a defined passing threshold.
- Objective: Enhance problem-solving skills through practical application.
- Outcome: Students will gain experience in problem-solving by applying programming concepts to a practical scenario, enhancing their critical thinking and analytical abilities.
- Objective: Develop a fully functional Grade Computation Program.
- Outcome: Students will successfully create a Grade Computation Program that computes final grades and provides clear pass/fail feedback, demonstrating the application of programming skills to real-world situations.
- Objective: Foster a foundation for further programming exploration.
- Outcome: Students will establish a strong foundation in C# programming, which will empower them to tackle more complex programming challenges and pursue further learning and projects.
Source code example
using System; namespace GradeComputation { class Program { static void Main(string[] args) { // Initialize variables to store grades and weights double prelimGrade, midtermGrade, endTermGrade; double prelimWeight = 0.30, midtermWeight = 0.30, endTermWeight = 0.40; Console.WriteLine("Grade Computation Program"); // Prompt and validate Prelim Grade prelimGrade = ValidateGrade("Prelim Grade"); // Prompt and validate Midterm Grade midtermGrade = ValidateGrade("Midterm Grade"); // Prompt and validate End Term Grade endTermGrade = ValidateGrade("End Term Grade"); // Calculate the final grade double finalGrade = (prelimGrade * prelimWeight) + (midtermGrade * midtermWeight) + (endTermGrade * endTermWeight); // Display the final grade Console.WriteLine($"Your Final Grade is: {finalGrade:F2}"); // Check if the final grade is a pass or fail if (finalGrade >= 75) { Console.WriteLine("Congratulations! You passed."); } else { Console.WriteLine("Sorry, you failed."); } Console.ReadLine(); // Keep the console window open } // method to check (input validation) if the user input is numeric static double ValidateGrade(string gradeType) { double grade; bool validInput = false; do { Console.Write($"Enter {gradeType}: "); if (double.TryParse(Console.ReadLine(), out grade)) { validInput = true; } else { Console.WriteLine($"Invalid input for {gradeType}. Please enter a valid number."); } } while (!validInput); return grade; } } }
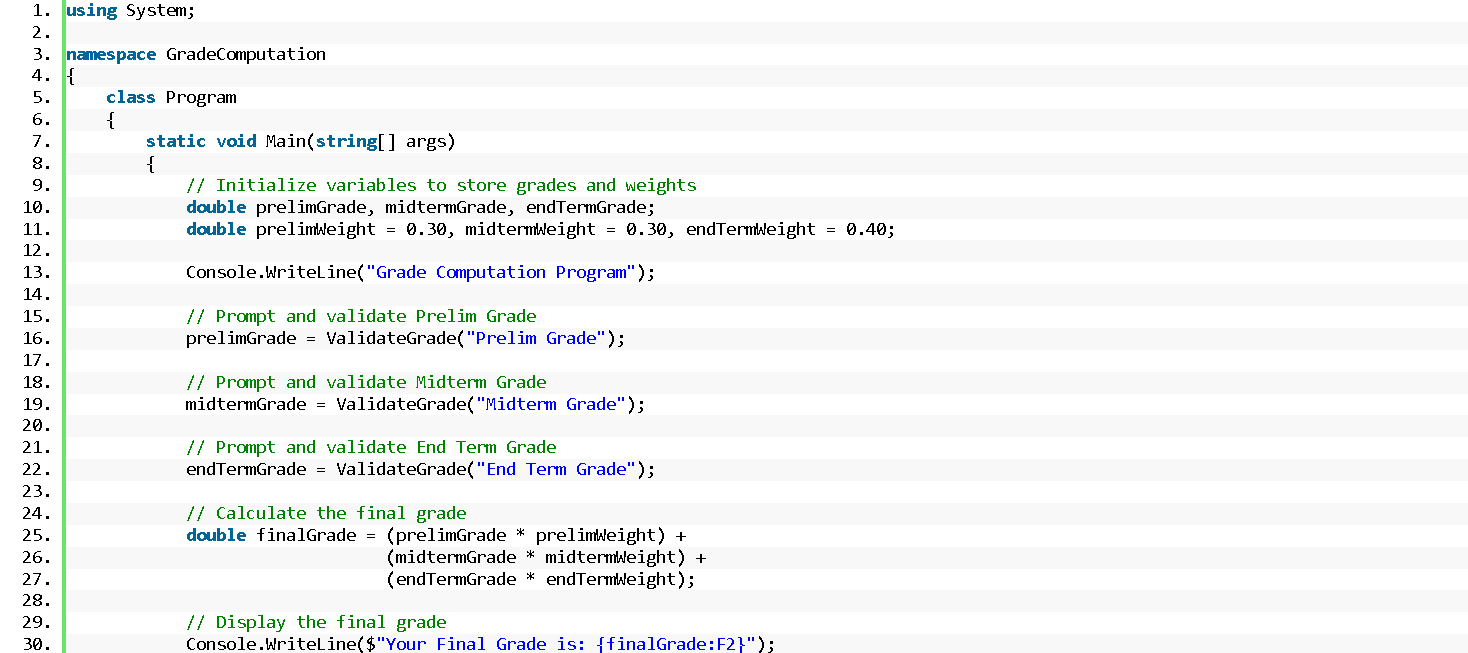
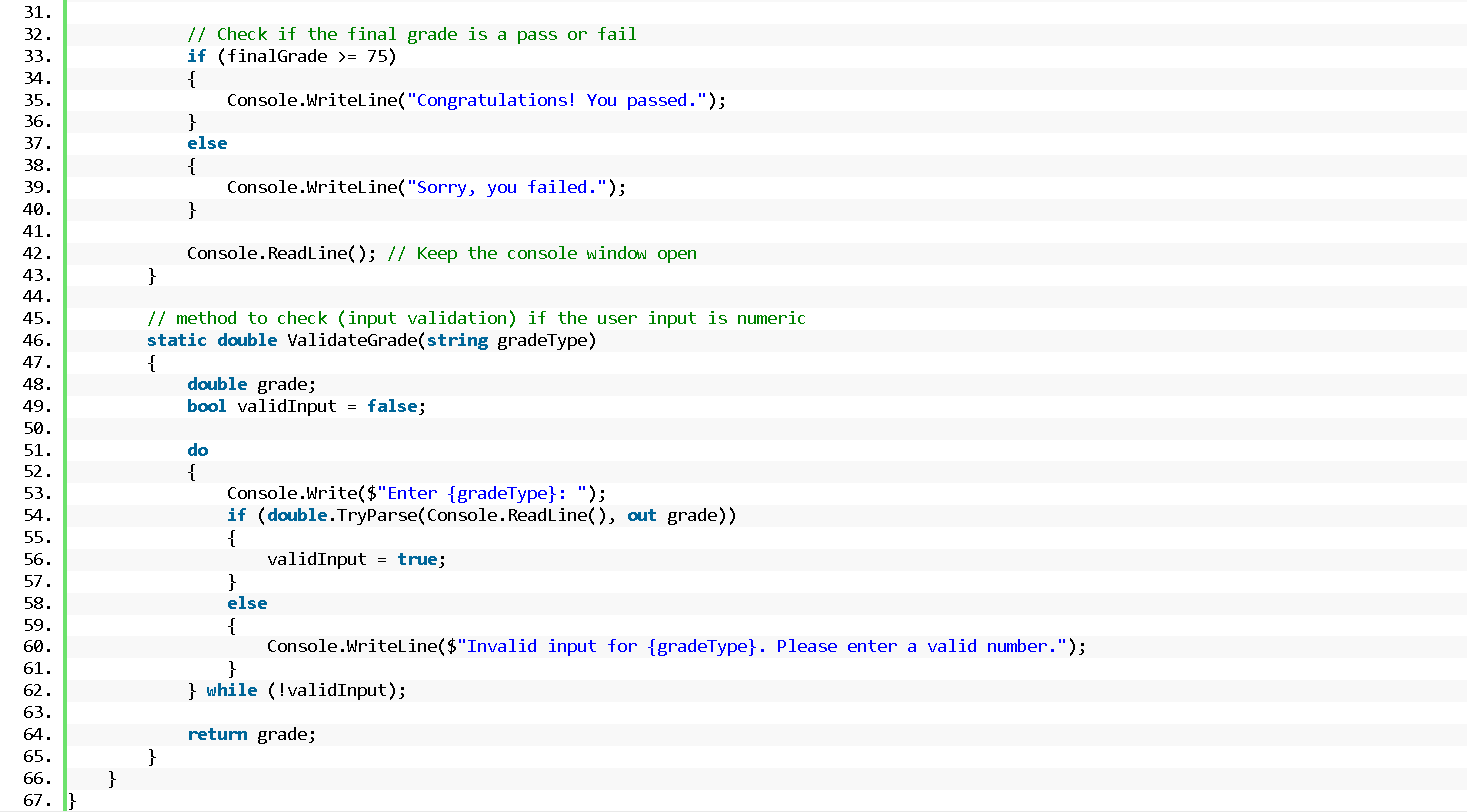
Grade Computation in CSharp – source code
Explanation
- We begin by defining a C# program within the GradeComputation namespace.
- Inside the Main method (the program’s entry point), we declare variables to store the preliminary grade (prelimGrade), midterm grade (midtermGrade), and end-term grade (endTermGrade). We also define constants (prelimWeight, midtermWeight, and endTermWeight) to represent the weight of each grade.
- We print a welcome message to the console to indicate that this is the “Grade Computation Program.”
- We use the ValidateGrade method to prompt the user for their preliminary, midterm, and end-term grades while validating the input. The ValidateGrade method is defined later in the code.
- After obtaining the valid grades, we calculate the final grade by applying the respective weights to each grade component and summing them up.
- We display the final grade using Console.WriteLine with formatting to show two decimal places.
- Next, we check if the final grade is greater than or equal to 75 (the passing grade). If it is, we display “Congratulations! You passed.” Otherwise, we display “Sorry, you failed.”
- Finally, we use Console.ReadLine() to keep the console window open until the user presses Enter, allowing them to view the results.
The ValidateGrade method is defined as a separate function to handle the input validation. It repeatedly prompts the user for a grade input until a valid numeric value is provided.
This program takes user inputs for grades, calculates a final grade based on predefined weights, and determines if the student has passed or failed based on a passing grade of 75. It’s a simple example of input validation and conditional statements in C#.
Output
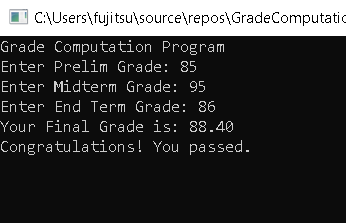
Summary
In our recent blog post, we took a step into creating a Grade Computation Program in C#. We simplified variables, made naming conventions easier to understand, and broke down arithmetic operations for precise grade calculations. We ensured data accuracy through input validation and used conditional statements to determine pass/fail outcomes. By the end, readers became ready to use code to solve practical problems. We encouraged ongoing practice and exploration, emphasizing that this program is not just about code—it’s a tool for academic success and programming expertise.
Assessment
Assessment 1 (50 points):
Enhancing Input Validation Assignment: Modify the program to improve input validation. Currently, it validates that the input is a number, but it doesn’t check if the entered grades are within the valid range (0-100). Enhance the program to ensure that the entered grades are valid, and if not, display an error message and prompt the user to re-enter the grade.
Assessment 2 (50 points):
Implementing Letter Grades Assignment: Expand the program to include letter grades based on the final numeric grade. Define a grading scale (e.g., A for 90-100, B for 80-89, etc.), and calculate and display both the numeric and letter grades for the user.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.