Counting Positive and Negative Numbers Array
Introduction
Table of Contents
In the world of programming, arrays are a fundamental data structure that allows us to store and organize a collection of elements of the same data type. Arrays provide a convenient way to work with multiple values efficiently, enabling us to solve a wide range of problems.
Working with arrays is crucial in various applications. Whether it’s analyzing large datasets, managing inventories, or performing mathematical calculations, arrays play a vital role in organizing and manipulating data. By understanding how to work with arrays, we gain the ability to tackle complex tasks more effectively and write efficient, scalable code.
In this lesson, we will focus on a specific task: counting positive and negative numbers in an array. This task is a common requirement in many applications. It allows us to determine the number of positive and negative values within a given set of integers, providing valuable insights into the data’s characteristics.
By mastering the technique of counting positive and negative numbers in an array, you will gain a deeper understanding of array traversal, conditional statements, and basic arithmetic operations. This knowledge will empower you to solve a wide range of problems that involve data analysis, filtering, and statistics.
Throughout this lesson, we will guide you through the process of writing a C# program that counts the number of positive and negative numbers in a given array of integers. We will explain the necessary concepts, provide code examples, and encourage you to experiment with different inputs to solidify your understanding.
Objectives
In this lesson, our main objective is to learn how to count positive and negative numbers in an array using C#. By the end of this lesson, you will have a solid understanding of array traversal, conditional statements, and basic arithmetic operations. You will be able to confidently write a C# program that counts the number of positive and negative numbers in a given array of integers. Throughout the lesson, we will focus on four key objectives: understanding the concept, learning the necessary techniques, practicing through coding examples, and applying the knowledge to real-world scenarios.
Objective 1: Understand the Concept We will start by gaining a thorough understanding of the concept of counting positive and negative numbers in an array. This involves understanding how arrays work, the significance of positive and negative numbers, and the importance of counting them in various applications. By understanding the concept, you will be able to identify the problem and its requirements more effectively.
Objective 2: Learn the Necessary Techniques Next, we will delve into the techniques required to count positive and negative numbers in an array. We will cover topics such as array traversal, conditional statements, and basic arithmetic operations. By learning these techniques, you will gain the necessary skills to implement the counting logic efficiently.
Objective 3: Practice through Coding Examples To reinforce your understanding, we will provide you with several coding examples that demonstrate how to count positive and negative numbers in an array using C#. These examples will cover different scenarios and edge cases, allowing you to practice your skills and enhance your problem-solving abilities.
Objective 4: Apply the Knowledge to Real-World Scenarios Lastly, we will explore real-world scenarios where counting positive and negative numbers in an array is crucial. We will discuss applications such as data analysis, financial modeling, and performance evaluation, where understanding the distribution of positive and negative numbers can provide valuable insights. By applying the knowledge gained in this lesson, you will be prepared to tackle similar problems in practical situations.
Throughout this lesson, we encourage you to actively engage with the material, ask questions, and experiment with different inputs. By doing so, you will enhance your learning experience and gain confidence in your ability to count positive and negative numbers in an array using C#.
Source code example
using System;
class CountNumbersInArray
{
static void Main()
{
Console.WriteLine(“iNetTutor.com – Counting Positive and Negative Numbers”);
// Declare and initialize an array
int[] numbers = { 5, -2, 10, -8, 3, 0, -6 };
// Initialize counters
int positiveCount = 0;
int negativeCount = 0;
// Loop through each element in the array
foreach (int number in numbers)
{
// Check if the number is positive or negative
if (number > 0)
{
positiveCount++;
}
else if (number < 0)
{
negativeCount++;
}
}
// Display the results
Console.WriteLine(“Array: [” + string.Join(“, “, numbers) + “]”);
Console.WriteLine(“Positive Numbers: ” + positiveCount);
Console.WriteLine(“Negative Numbers: ” + negativeCount);
Console.ReadLine();
}
}
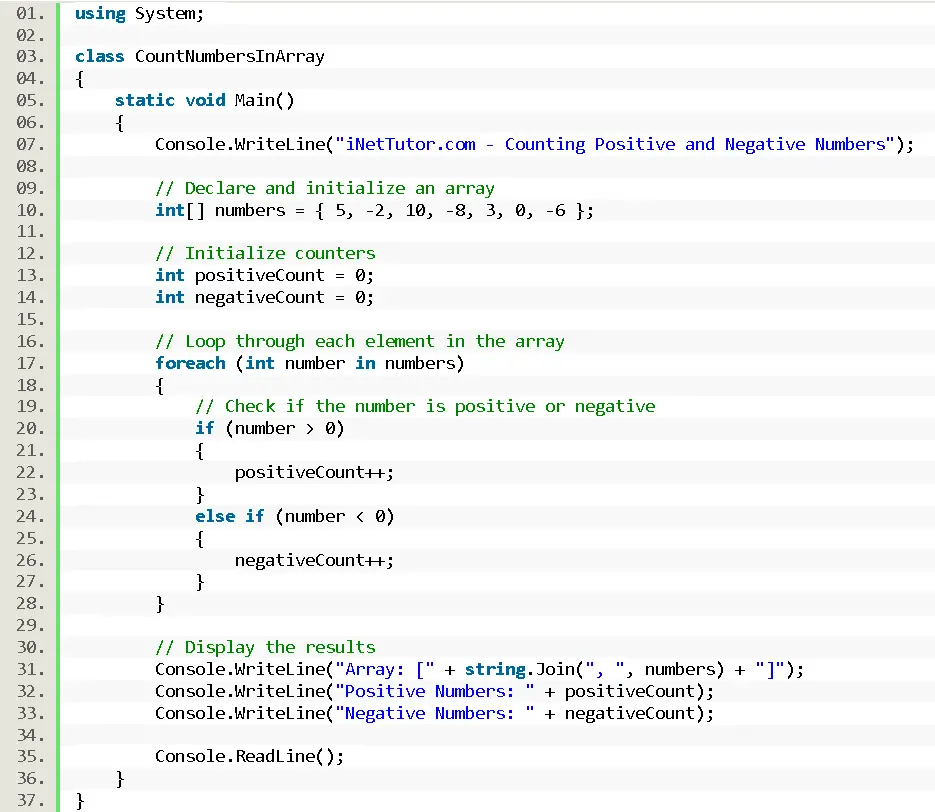
Explanation
This C# code effectively counts positive and negative numbers within an array and presents the results in a user-friendly manner. Here’s a breakdown of how it works:
- Introduction and Array Initialization:
- Prints a welcome message to the user.
- Declares and initializes an integer array named numbers containing sample values.
- Counter Initialization:
- Declares and initializes two integer variables: positiveCount and negativeCount to keep track of the respective counts.
- Looping and Counting:
- Employs a foreach loop to iterate through each element (number) in the numbers array.
- Inside the loop:
- Uses an if statement to check if the current number is positive (number > 0). If true, increments positiveCount.
- Employs an else if statement to check if the number is negative (number < 0). If true, increments negativeCount.
- Displaying Results:
- Prints the original array in a clear format using string concatenation and string.Join to display the elements separated by commas within square brackets.
- Prints the count of positive and negative numbers using string interpolation for formatting.
- Calls Console.ReadLine() to wait for the user to press any key before exiting.
Key Points:
- The code utilizes a clear and concise approach with well-named variables and comments to enhance readability.
- The foreach loop provides a convenient way to iterate through the array elements without explicitly managing indices.
- The conditional statements effectively categorize the numbers and increment the corresponding counters.
- String formatting techniques are used to present the array and results in a user-friendly manner.
This code effectively demonstrates the core principles of counting positive and negative numbers in arrays within C#, making it suitable for beginners to grasp the concepts and practice their coding skills.
Output
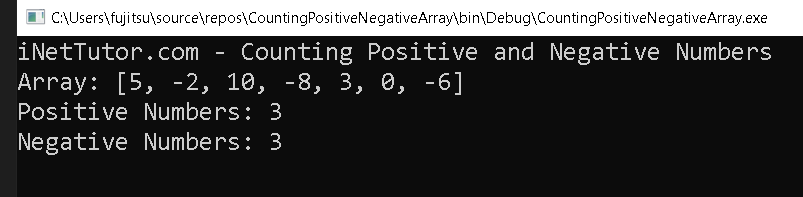
Summary
This lesson empowered you to transform from a passive observer of data to an active analyst! You explored the essential skill of counting positive and negative numbers within arrays in C#.
Here’s what you accomplished:
- Understanding: Grasped the concept of arrays, their importance in data storage, and the value of analyzing positive/negative number distribution.
- Learning: Mastered techniques for iterating through arrays to identify and count positive and negative values using conditional statements.
- Practicing: Honed your skills through code examples and exercises, solidifying your understanding of counting within various array scenarios.
Now you can:
- Apply: Integrate this skillset into real-world scenarios like analyzing user feedback, financial data, or scientific measurements.
- Explore further: Leverage this foundation to delve deeper into advanced array manipulation techniques and data analysis methods.
Remember: This is just the beginning! As you progress in your programming journey, continue to explore and experiment with arrays to unlock their full potential in extracting valuable insights from data.
Exercises and Assessment
To further improve your understanding and skills in working with arrays and counting positive and negative numbers, here are some suggested exercises, an assessment, and a lab exam:
Exercises:
- Array Expansion: Modify the code to handle arrays of varying sizes. Prompt the user to enter the size of the array and its elements dynamically.
- Additional Counters: Extend the program to count zero and even numbers in the array. Update the display to show counts for positive, negative, zero, and even numbers.
- Input Validation: Implement input validation to ensure that the user enters only numeric values when initializing the array, and provide appropriate error messages.
Assessment:
Project Task: Ask students to enhance the program to find the average of positive and negative numbers separately in the array.
Quiz Questions:
- What is the significance of arrays in programming?
- How would you modify the code to handle an array of size determined by user input?
- Explain the importance of input validation in this context.
Lab Exam:
Task 1: Array Manipulation Students should write a program that performs multiple array manipulations, including inserting an element, updating an element, and reversing the array.
Task 2: Error Handling Require students to modify the original code to include comprehensive error handling, such as handling non-numeric inputs and ensuring the array size is within a reasonable range.
Quiz
- What loop construct is used to iterate through each element of an array in C#?
a) for loop
b) while loop
c) foreach loop
d) do-while loop - Which statement checks if a number is positive in the code?
a) if (number > 0)
b) if (number != 0)
c) if (number >= 0)
d) All of the above - How does the code display the elements of the array?
a) By directly printing the array object
b) Using a for loop and accessing elements by index
c) Using string concatenation and joining the elements
d) Using the ToString() method of the array
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.