Age Category Checker in C#
Introduction
Table of Contents
Welcome to the next lesson on C#! In this lesson, we will be learning about the Age Category Checker in C#. This program will allow us to determine the age category of a person based on their age.
Before we dive into the details of the Age Category Checker, let’s briefly discuss C# and its importance in the world of programming.
C# is a general-purpose programming language that was developed by Microsoft in the early 2000s. It is widely used for developing a variety of applications, including desktop, web, and mobile applications. C# is known for its simplicity, readability, and object-oriented programming capabilities.
Now that we have a basic understanding of C#, let’s move on to the Age Category Checker program. This program will take a person’s age as input and determine which age category they fall into. The age categories can be defined based on specific criteria, such as child, teenager, adult, or senior citizen.
Throughout this lesson, we will explore the concepts of conditional statements, variables, and user input in C#. By the end of this lesson, you will have a solid understanding of how to create a program that categorizes individuals based on their age.
Objectives
In this lesson on the Age Category Checker in C#, we will focus on the following objectives:
- Understand the concept of conditional statements in C# and their role in decision-making within a program.
- Learn how to declare and initialize variables in C# to store and manipulate data.
- Practice writing conditional statements using if-else statements and switch-case statements in C# to categorize individuals based on their age.
- Apply the knowledge gained to create a complete Age Category Checker program that takes user input, determines the age category, and displays the result.
By the end of this lesson, you will have a solid understanding of conditional statements, variables, and user input in C#. You will be able to write a program that categorizes individuals based on their age and apply this knowledge to solve similar problems in your future projects.
Source code example
using System; namespace AgeCategoryChecker { class Program { static void Main() { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Welcome to the Age Category Checker!"); Console.Write("Enter your age: "); int age = int.Parse(Console.ReadLine()); if (age >= 0 && age <= 12) { Console.WriteLine("You are a child! Enjoy your youth!"); } else if (age >= 13 && age <= 19) { Console.WriteLine("Welcome to the exciting world of being a teenager!"); } else if (age >= 20 && age <= 64) { Console.WriteLine("Congratulations! You are an adult now."); } else if (age >= 65) { Console.WriteLine("Welcome to the wonderful stage of being a senior citizen!"); } else { Console.WriteLine("Invalid age entered. Please enter a valid age."); } Console.ReadLine(); } } }
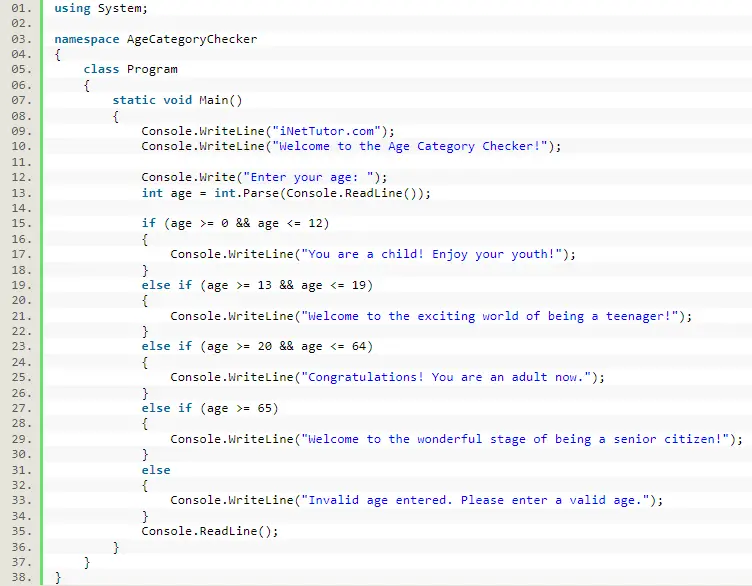
Explanation
This C# code implements a program that categorizes users based on their age, displaying personalized messages for each category.
Breakdown:
- Namespace and Class:
- using System;: Includes the System namespace, providing essential functionalities like input/output.
- namespace AgeCategoryChecker: Organizes the code within a specific namespace.
- class Program: Defines the main program class.
- Main Method:
- static void Main(): The entry point of the program.
- Welcome Message:
- Console.WriteLine(“iNetTutor.com”);: Displays a personalized message, but you can remove it if desired.
- Console.WriteLine(“Welcome to the Age Category Checker!”);: Prints the program’s purpose.
- User Input:
- Console.Write(“Enter your age: “);: Prompts the user to enter their age.
- int age = int.Parse(Console.ReadLine());: Reads the user’s input, parses it into an integer, and stores it in the age variable.
- Age Category Check:
- if-else if chain: A series of conditional statements used to evaluate the user’s age and assign them to a category:
- age >= 0 && age <= 12: Checks if the age is between 0 and 12 (inclusive), categorizing them as a child.
- Similar conditions exist for teenagers (13-19), adults (20-64), and senior citizens (65+).
- Each if or else if block prints a corresponding message if the condition is met.
- else: If the age doesn’t fit any category, an error message is displayed.
- if-else if chain: A series of conditional statements used to evaluate the user’s age and assign them to a category:
- Closing:
- Console.ReadLine();: Pauses the program before closing, allowing the user to see the output.
Additional Notes:
- This code only uses basic if-else statements. You could explore using a switch statement for a more concise version depending on your preference.
- You can modify the age ranges and category messages to suit specific needs.
- Remember to handle potential errors, such as invalid input types or ages outside the expected range.
By understanding this code and its explanations, you gain valuable insights into C# programming fundamentals like:
- User input and output using Console class.
- Conditional statements (if-else if) for decision-making.
- Variable declaration and usage.
- Basic program structure and execution.
Output
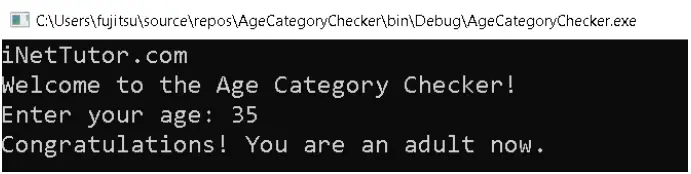
Summary
In this lesson, we covered the Age Category Checker in C#. We learned how to prompt the user for their age and categorize them into different age groups: child, teenager, adult, or senior citizen. By using if-else statements, we were able to check the age entered by the user and display the appropriate message for each category.
By completing the lesson, you should now have a solid understanding of how to use if-else statements in C# to categorize individuals based on their age. You have also gained experience in handling user input and displaying appropriate messages based on the entered age.
Remember to practice what you have learned by experimenting with different inputs and modifying the program to suit your needs. Building upon this foundation, you can continue exploring more complex programming concepts and expand your skills in C#.
Exercises and Assessment
Remember: Utilize the provided code as a starting point, but don’t be afraid to rewrite or restructure it as needed. Experiment with different approaches, test your code thoroughly, and seek help if you get stuck. By tackling these exercises and the lab exam, you’ll not only refine your age category checker skills but also gain valuable experience in user input, date manipulation, conditional statements, and building practical applications in C#.
These exercises and lab exam elements encourage students to not only grasp the existing concepts but also apply critical thinking to improve and expand the functionality of the Age Category Checker program.
Tasks:
- Dynamic age ranges: Instead of fixed ranges, allow users to input the minimum and maximum ages for each category, making the program more adaptable.
- Multiple categories: Add more age categories like “young adult” or “middle-aged” to provide a more nuanced classification.
- Error handling: Implement robust error handling to gracefully handle invalid input (e.g., non-numeric input, ages outside expected range).
Related Topics and Articles:
Rock, Paper, Scissors Game in C#
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.