Password Indicator in PHP Free Source code and Tutorial
Introduction
This tutorial will teach you how to use PHP to develop a password indicator system. This system will tell you how strong your password is and make advice on how to make it even stronger. This is a great approach to make your website more secure. You will have a better grasp of how to establish a password indicator system after completing this lesson, and you will be able to create one for your own website.
Objectives
By the end of this tutorial, you will be able to:
- To create a PHP script that will validate and check how strong is your password.
- Use preg_match() function in PHP.
- To integrate and apply the concept of this tutorial in your projects.
Relevant Source code
The following are the requirements for this tutorial:
- XAMPP
- Text editor (VS Code, Sublime, Brackets), download and install a text editor of your choice
Front-end

Line 20-23 – this is the form used to capture and submit the password for testing how strong and secure it is.
<form method="post"> <input type="text" name="pwd" class="form-control" placeholder="Enter password"><br> <input type="submit" name="submit" class="btn btn-primary" value="Check Password"> </form>
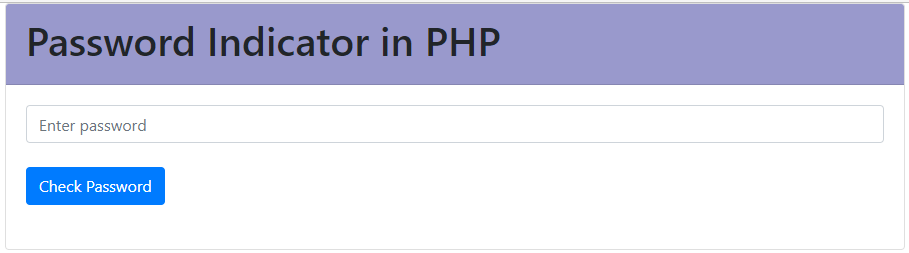
Back-end
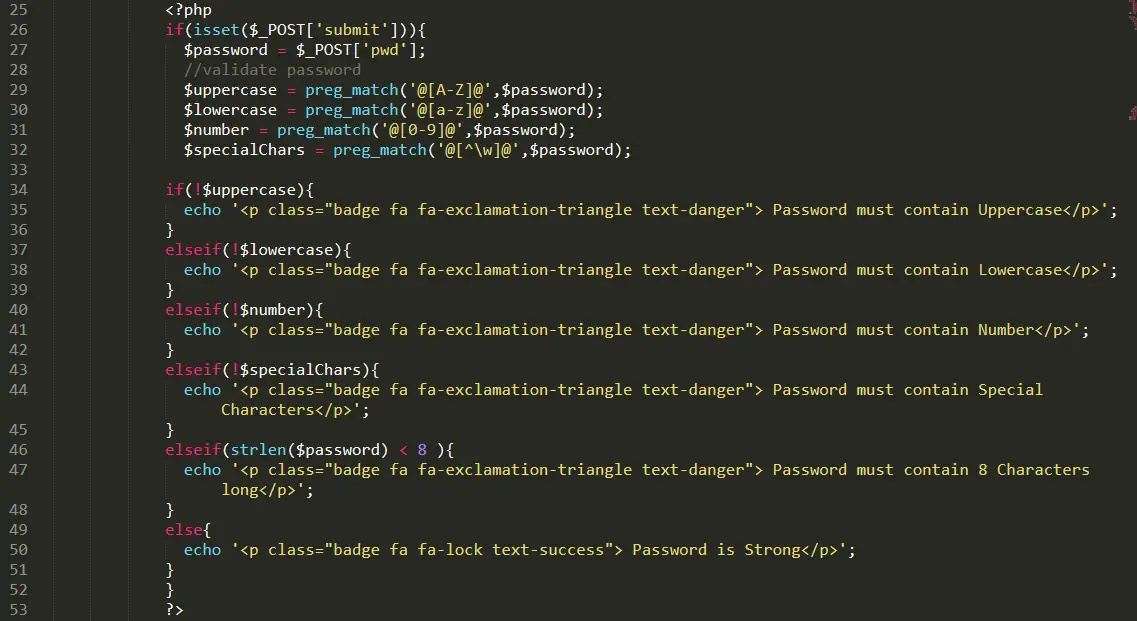
Line 25-53 – this PHP script that will check how strong your password is. The script will be executed when the user inputs the password and click Check Password button.
The preg_match() function returns whether a match was found in a string.
Line 29 – this line is responsible for checking if your password contains upper case letter.
Line 30 – this line is responsible for checking if your password contains lower case letter.
Line 31 – this line is responsible for checking if your password contains a number.
Line 32 – this line is responsible for checking if your password contains special characters.
<?php if(isset($_POST['submit'])){ $password = $_POST['pwd']; //validate password $uppercase = preg_match('@[A-Z]@',$password); $lowercase = preg_match('@[a-z]@',$password); $number = preg_match('@[0-9]@',$password); $specialChars = preg_match('@[^\w]@',$password); if(!$uppercase){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Uppercase</p>'; } elseif(!$lowercase){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Lowercase</p>'; } elseif(!$number){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Number</p>'; } elseif(!$specialChars){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Special Characters</p>'; } elseif(strlen($password) < 8 ){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain 8 Characters long</p>'; } else{ echo '<p class="badge fa fa-lock text-success"> Password is Strong</p>'; } } ?>
In general, the script will provide you a message indicating that you should include the following in your password to make it stronger and difficult to guess.
- At least 8 characters
- Contains upper and lower case
- Contains special characters
Complete Source code
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0, shrink-to-fit=no"> <title>Password Indicator in PHP</title> <link rel="stylesheet" href="assets/bootstrap/css/bootstrap.min.css"> <link rel="stylesheet" href="assets/fontawesome/css/all.min.css"> </head> <body> <div class="row"> <div class="col-md-8 offset-md-2"> <div class="card"> <div class="card-header bg"> <h1>Password Indicator in PHP</h1> </div> <div class="card-body"> <form method="post"> <input type="text" name="pwd" class="form-control" placeholder="Enter password"><br> <input type="submit" name="submit" class="btn btn-primary" value="Check Password"> </form> <br> <?php if(isset($_POST['submit'])){ $password = $_POST['pwd']; //validate password $uppercase = preg_match('@[A-Z]@',$password); $lowercase = preg_match('@[a-z]@',$password); $number = preg_match('@[0-9]@',$password); $specialChars = preg_match('@[^\w]@',$password); if(!$uppercase){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Uppercase</p>'; } elseif(!$lowercase){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Lowercase</p>'; } elseif(!$number){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Number</p>'; } elseif(!$specialChars){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain Special Characters</p>'; } elseif(strlen($password) < 8 ){ echo '<p class="badge fa fa-exclamation-triangle text-danger"> Password must contain 8 Characters long</p>'; } else{ echo '<p class="badge fa fa-lock text-success"> Password is Strong</p>'; } } ?> </div> </div> </div> </div> <script src="assets/js/jquery.min.js"></script> <script src="assets/bootstrap/js/bootstrap.min.js"></script> </body> </html>
Video Demo
Summary
A strong password is important because it helps to keep your account safe from hackers. A strong password is also important because it helps to prevent other people from accessing your account. A strong password should be at least eight characters long and should include a mix of letters, numbers, and symbols. A strong password should also be changed regularly. Finally, it is important to remember to never use the same password on more than one website. If you do, you could be opening yourself up to potential online security risks. The article is a simple guide that you can use and enhance to create a password checker and suggests how to create a strong password.
We hope you found this tutorial to be helpful! Wishing you the best of luck with your projects! Happy Coding!
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.
Related Topics and Articles:
How to Generate Random Password in PHP Free Source code and Tutorial
Email Validation in PHP Free Source code and Tutorial
Merge Two Strings in PHP Free Source code and Tutorial
Get File size and File Extension in PHP Free Source code and Tutorial