Respondents Calculator in CSharp
Introduction
Table of Contents
The purpose of this program is to calculate the number of respondents using Sloven’s formula. Sloven’s formula is a vital tool in statistics and research, particularly when conducting surveys and experiments. It helps in determining the sample size required to make statistically meaningful inferences about a larger population. In essence, this program will assist users in making informed decisions about how many individuals should be included in a survey or study, ensuring that their research results are representative and reliable.
Determining the appropriate sample size is a fundamental aspect of research and survey methodologies. It directly influences the validity and accuracy of research findings. Without a correctly sized sample, results may not be representative of the population and can lead to biased or inconclusive outcomes. In fields ranging from social sciences to market research, having a precise understanding of the number of respondents required is crucial for making informed decisions and drawing reliable conclusions. Sloven’s formula provides a structured approach to this problem, allowing researchers to strike a balance between the costs and benefits of data collection, ultimately enhancing the quality of their research.
Understanding the significance of respondents calculation is not limited to research professionals alone; it’s a valuable skill for anyone who wishes to make data-driven decisions, whether in business, academia, or public policy. This program empowers individuals with the ability to calculate the ideal sample size, making it an indispensable tool in the realm of data analysis and research.
Objectives
- Learn Sloven’s Formula: Understand the concept of Sloven’s formula and how it is used to calculate the number of respondents in a given population. Gain insight into the formula’s components, including population size, margin of error, and the desired sample size.
- Apply Respondents Calculation: Apply the knowledge of Sloven’s formula to real-world scenarios where determining an appropriate sample size is crucial. Learn how to use the formula to optimize data collection for surveys, research, and experiments.
- Create a User-Friendly Program: Create a C# program that enables users to input a population size and automates the calculation of the required sample size using Sloven’s formula. Develop user-friendly interfaces and interactive features for a seamless user experience.
By the end of this lesson, learners will not only grasp the theoretical foundation of Sloven’s formula but also be able to apply it practically through the development of a user-friendly C# program. These objectives empower individuals to make well-informed decisions and conduct reliable research across various domains.
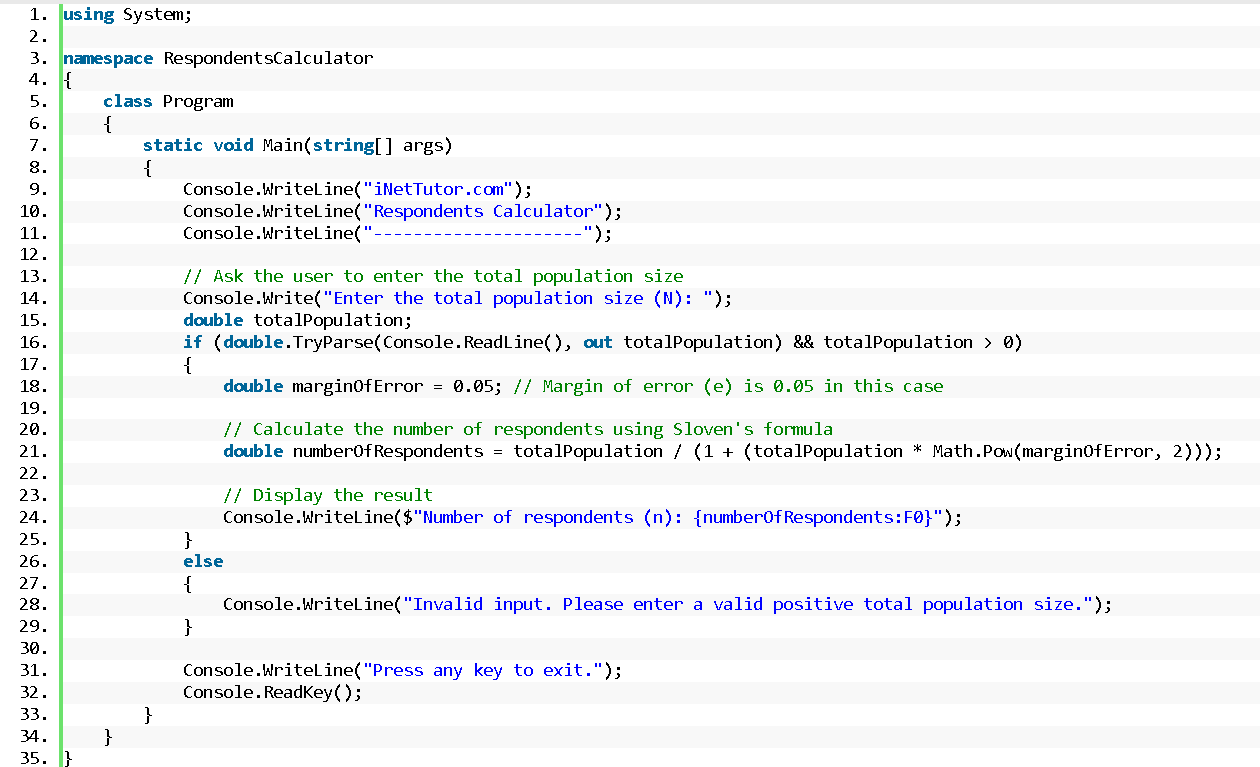
Source code example
using System; namespace RespondentsCalculator { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Respondents Calculator"); Console.WriteLine("---------------------"); // Ask the user to enter the total population size Console.Write("Enter the total population size (N): "); double totalPopulation; if (double.TryParse(Console.ReadLine(), out totalPopulation) && totalPopulation > 0) { double marginOfError = 0.05; // Margin of error (e) is 0.05 in this case // Calculate the number of respondents using Sloven's formula double numberOfRespondents = totalPopulation / (1 + (totalPopulation * Math.Pow(marginOfError, 2))); // Display the result Console.WriteLine($"Number of respondents (n): {numberOfRespondents:F0}"); } else { Console.WriteLine("Invalid input. Please enter a valid positive total population size."); } Console.WriteLine("Press any key to exit."); Console.ReadKey(); } } }
Explanation
The provided C# source code is for a program called “Respondents Calculator” that calculates the number of respondents needed using Sloven’s formula based on user input. Here’s an explanation of each part of the code:
- Namespaces and Class:
- The code begins by declaring the necessary namespaces and defining a class named Program. The System namespace is included for input and output operations.
- Main Method:
- The Main method is the entry point of the program, where execution starts. It’s the primary function that interacts with the user.
- User Interface:
- The program starts by displaying a header with the text “iNetTutor.com” and “Respondents Calculator” to inform the user of the program’s purpose.
- The line of hyphens creates a visual separator.
- User Input:
- The program prompts the user to enter the total population size (N) with the message: “Enter the total population size (N): “.
- The user’s input is read as a string and then parsed into a double variable named totalPopulation.
- Input Validation:
- The program checks whether the user’s input is a valid positive number. It uses a combination of double.TryParse to convert the user’s input to a numeric value and ensures that the total population size is greater than zero.
- Sloven’s Formula:
- The program defines a constant marginOfError with a value of 0.05, representing the margin of error (e) specified in the Sloven’s formula.
- It then calculates the number of respondents (n) using Sloven’s formula:
Where:
-
-
- n is the number of respondents.
- N is the total population size.
- e is the margin of error.
-
7. Display Result:
-
- The program displays the calculated number of respondents (n) using Console.WriteLine. The :F0 format specifier is used to display the number as a whole (no decimal places).
8. Input Error Handling:
-
- If the user enters invalid input (e.g., a non-positive number or non-numeric input), the program informs the user with the message: “Invalid input. Please enter a valid positive total population size.”
9. Exit Prompt:
-
- The program displays the message “Press any key to exit.” and waits for the user to press any key to close the console window.
Output
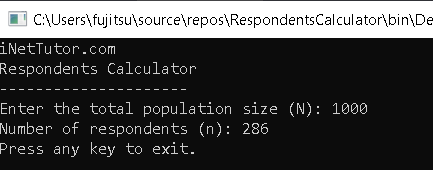
Summary
In this “Respondents Calculator” lesson, you’ve crafted a C# program that efficiently computes the number of respondents using Sloven’s formula, a vital tool for survey and research planning. The core concepts you’ve encountered include collecting user input for the total population size, validating that input to ensure it’s a positive numeric value, and applying the mathematical algorithm of Sloven’s formula. The program calculates the number of respondents accurately and presents the result clearly. It’s essential for comprehending sample size determination in research and surveys, and you’re encouraged to experiment further with this program. Explore ways to improve the user interface, handle different input formats, enhance error handling, or extend its applicability to various scenarios. By doing so, you’ll not only solidify your programming skills but also gain insights into the practical applications of this mathematical concept.
Exercises and Assessment
Here are some challenges to enhance the “Respondents Calculator” program:
- Graphical User Interface (GUI): Transform the program into a Windows Forms Application or a WPF application, providing a user-friendly interface with input fields, labels, and a button to trigger the calculation.
- Custom Error Handling: Implement custom error handling to provide more informative error messages, guiding users on how to correct invalid inputs.
- Variable Margin of Error: Allow users to input a custom margin of error (e) instead of hardcoding it. This enhancement will make the program more versatile for different survey scenarios.
- File I/O: Enable the program to read the total population size from a text file and write the results to another file, making it easier to process large datasets.
- Conversion Calculator: Expand the program’s functionality by including the option to convert the number of respondents into a percentage of the total population, providing additional insights for researchers.
These challenges will not only broaden your C# programming skills but also make the “Respondents Calculator” more robust and adaptable to various real-world research needs.
Quiz
Here’s a quiz to test your understanding of the “Respondents Calculator” source code and concepts:
- What is the primary purpose of the “Respondents Calculator” program?
a. Calculate the margin of error
b. Determine the number of survey questions
c. Calculate the number of respondents
d. Analyze population demographics - Which formula is used in the program to calculate the number of respondents?
a. Euler’s formula
b. Archimedes’ formula
c. Pythagoras’ theorem
d. Sloven’s formula - What is the margin of error (e) set to in the program?
a. 0.01
b. 0.05
c. 0.10
d. 0.20 - What is the main input required from the user in the program?
a. Margin of error
b. Total survey questions
c. Total population size (N)
d. Number of survey respondents - If a user enters a non-positive total population size, what message will be displayed?
a. “Invalid input. Please enter a valid positive total population size.”
b. “Calculations are not possible with the provided inputs.”
c. “Please input the margin of error first.”
d. “The population size must be a whole number.”
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.