Salary Info Program in CSharp
Introduction
Table of Contents
The purpose of this program is to create a C# application that calculates an employee’s salary, taking into account various factors like the rate per day, tax deductions, and more. By collecting essential information about the employee, such as their name and rate per day, the program will provide detailed salary-related calculations. These calculations include determining the employee’s salary for different time periods, the rate per hour, and applying a tax deduction of 12%. The primary objective is to simplify the process of salary calculation, making it useful for both employees and employers.
Accurate salary calculations hold significant importance in the professional world. For employees, it ensures that they receive fair compensation for their work, allowing them to manage their finances and meet their financial goals. Employers benefit by having a structured system that calculates wages, which helps in budgeting and maintaining a motivated workforce.
In a broader sense, precise salary calculations contribute to trust and satisfaction in the workplace, which is crucial for the overall success of a business or organization. It also plays a role in ensuring legal compliance, as businesses are often required to adhere to labor laws and taxation regulations when determining employee salaries.
In addition, understanding the logic behind salary calculations can empower employees to better manage their personal finances, set savings goals, and make informed financial decisions. By creating a program that handles these calculations, we demystify the complexity of the salary determination process and make it accessible to all who seek a straightforward and reliable solution.
Problem Description: Employee Salary Calculator
You are tasked with developing a C# program to assist in calculating various aspects of an employee’s salary based on user-provided information. The program should prompt the user for the employee’s name and their rate of pay per day. Once this information is obtained, the program should perform the following calculations:
- Calculate the salary for 15 days: The program should multiply the rate per day by 15 to determine the employee’s salary for half a month.
- Calculate the salary for 30 days: Similarly, the program should multiply the rate per day by 30 to determine the employee’s salary for a full month.
- Determine the rate per hour: Assuming an 8-hour workday, the program should divide the rate per day by 8 to determine the rate per hour.
- Calculate the salary for 300 hours: The program should multiply the rate per hour by 300 to determine the potential salary for 300 hours of work.
- Calculate a 12% tax deduction: The program should calculate a 12% tax deduction based on the employee’s monthly salary (30 days’ pay).
Finally, the program should display the collected employee name, the results of the above calculations, including the salary for 15 days, salary for 30 days, rate per hour, salary for 300 hours, and the 12% tax deduction.
Ensure that the program performs input validation to handle invalid inputs for the rate per day and provides clear, formatted output for the user.
Objectives
Outcomes-Based Objectives for the Lesson:
- Learn:
- Understand the core concepts of salary calculation, including the role of rate per day and tax deductions in determining net income.
- Gain proficiency in C# programming techniques for user input, mathematical calculations, and output display.
- Learn how to implement if-else conditions to manage different scenarios, such as invalid input.
- Apply:
- Apply the acquired knowledge to create a C# program that computes an employee’s salary accurately.
- Utilize C# programming skills to receive user input, validate data, and perform mathematical calculations.
- Apply conditional statements to handle edge cases and provide informative responses.
- Create:
- Develop a functional C# console program that takes user input for the employee’s name and rate per day.
- Design and implement the logic required for calculating the salary for 15 days, 30 days, rate per hour, salary for 300 hours, and applying a 12% tax deduction.
- Create a user-friendly interface that presents the results in a clear and organized manner.
- Upgrade:
- Enhance the program by adding error handling and user prompts to guide users in case of incorrect or incomplete input.
- Extend the program’s functionality to handle additional variables, such as overtime pay or bonuses, to make it even more versatile.
- Explore ways to further improve the user experience, like providing options for currency formatting or including a summary of the calculations for better understanding.
These objectives aim to ensure that learners not only grasp the fundamentals of salary calculation and C# programming but also have the practical skills to create a fully functional program and the creativity to enhance it for better usability and versatility.
Salary Info Program in CSharp
Source code example
using System; namespace SalaryInfoProgram { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Salary Info Program"); Console.WriteLine("-------------------"); // Ask the user for the employee's name Console.Write("Enter the employee's name: "); string employeeName = Console.ReadLine(); double ratePerDay; // Ensure valid input for rate per day while (true) { Console.Write("Enter the rate per day: "); if (double.TryParse(Console.ReadLine(), out ratePerDay) && ratePerDay >= 0) { break; // Exit the loop on valid input } else { Console.WriteLine("Invalid input. Please enter a valid non-negative number for the rate per day."); } } // Calculate salary for 15 days double salaryFor15Days = ratePerDay * 15; // Calculate salary for 30 days double salaryFor30Days = ratePerDay * 30; // Calculate rate per hour (assuming 8 hours per day) double ratePerHour = ratePerDay / 8; // Calculate salary for 300 hours double salaryFor300Hours = ratePerHour * 300; // Calculate 12% tax double tax = (salaryFor30Days * 0.12); // Display the results Console.WriteLine($"Employee Name: {employeeName}"); Console.WriteLine($"Salary for 15 days: {salaryFor15Days:C}"); Console.WriteLine($"Salary for 30 days: {salaryFor30Days:C}"); Console.WriteLine($"Rate per hour: {ratePerHour:C}"); Console.WriteLine($"Salary for 300 hours: {salaryFor300Hours:C}"); Console.WriteLine($"12% Tax: {tax:C}"); Console.WriteLine("Press any key to exit."); Console.ReadKey(); } } }
Explanation
This C# program serves the purpose of calculating an employee’s salary based on the rate per day and performing related calculations. It follows these essential steps:
- Program Introduction: The program provides an introductory message to inform the user about its purpose.
- User Input: Employee’s Name and Rate Per Day: It prompts the user to enter the employee’s name and rate per day, which are required for calculating the salary. The program stores the employee’s name in a variable and declares a variable to hold the rate per day.
- Rate Per Day Input Validation: To ensure the input’s validity, the program employs a loop that continues until the user enters a valid, non-negative number for the rate per day. This is achieved by using the double.TryParse method to validate the input. If an invalid input is provided, an error message is displayed, prompting the user to re-enter the rate per day.
- Salary and Tax Calculations: Once valid input is obtained, the program performs various calculations. These include calculating the salary for 15 and 30 days based on the rate per day, determining the rate per hour (assuming an 8-hour workday), calculating the salary for 300 hours, and computing a 12% tax on the salary for 30 days.
- Display Results: After these calculations, the program displays the results to the user. This includes presenting the employee’s name, salaries, rate per hour, and the calculated tax.
- Program Exit: Finally, the program allows the user to review the results by waiting for any key press before exiting.
In summary, this C# program is designed to take user input for an employee’s name and the rate per day, perform a series of calculations based on this input, and present the employee’s name and various salary-related information, including the tax amount. It ensures that the user provides valid input for the rate per day, making the program robust and user-friendly.Top of Form
Output
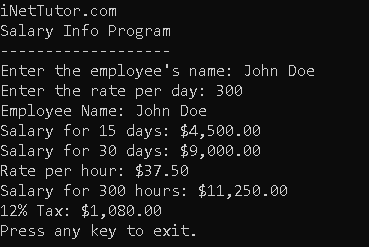
Recap
This program serves as an excellent introduction to key programming concepts, including:
- User Input: Understanding how to collect user input is fundamental in many programming tasks. In this program, the user provides the employee’s name and the rate per day, illustrating the importance of receiving and handling data from users.
- Input Validation: The program demonstrates the significance of validating user input. The loop used for validating the rate per day showcases how to create user-friendly programs that ensure data accuracy.
- Mathematical Calculations: The program involves various mathematical calculations, such as computing salaries for different time periods, determining rates per hour, and calculating taxes. These calculations are essential in various applications beyond this program.
- Output Display: The program teaches the importance of presenting calculated results to the user in a clear and understandable format. This skill is valuable for creating user-friendly applications.
Learners are encouraged to experiment with this program. They can:
- Modify Input Validation: Try altering the input validation process to accept different types of data or apply specific constraints.
- Extend Calculations: Experiment with additional calculations or different formulas to calculate salaries or taxes.
- Enhance User Interaction: Improve the program’s user interface or provide more detailed explanations and prompts to enhance the user experience.
- Incorporate Conditions: Add more conditions and remarks for different salary and tax brackets to make the program more comprehensive.
The logic and concepts applied in this salary calculation program have real-world applications in payroll systems and financial software. Payroll systems in organizations commonly use similar calculations to determine employee salaries, deductions, and taxes. The principles of user input, validation, and data processing are vital for developing efficient and accurate payroll software. This program is a small-scale representation of the calculations and processes that occur in larger payroll and financial systems, making it a valuable introduction to real-world applications.
By understanding and experimenting with this program, learners can lay the foundation for more complex financial and payroll software development, making it a valuable learning experience.
Exercises and Assessment
Here are some advanced challenges and exercises to enhance the Salary Info program:
- Multiple Employees: Modify the program to handle multiple employees’ information. Ask the user how many employees they want to calculate salaries for, and then take input for each employee’s name and rate per day. Calculate and display the salaries for all employees.
- Dynamic Tax Rates: Instead of a fixed 12% tax rate, allow the user to input the tax rate. This feature will make the program more versatile for different taxation systems or scenarios.
- Hourly Wage Variations: Modify the program to accept variations in the number of hours worked per day, allowing for more accurate hourly wage calculations.
- Additional Deductions: Implement a feature that allows the user to enter additional deductions (e.g., health insurance, retirement contributions) and factor these into the salary calculation.
These challenges will not only enhance the functionality of the program but also provide opportunities to learn more advanced programming concepts and techniques. Experimenting with these exercises will further solidify your C# programming skills and make the program more versatile and practical.
Quiz
1. How is invalid input handled when the user enters the rate per day?
a. The program closes immediately
b. An error message is displayed, and the program continues to run
c. The user is asked to input another value
d. An exception is thrown
2. What does the variable tax represent in the program?
a. The employee’s name
b. The rate per day
c. The calculated salary for 15 days
d. The 12% tax on the salary for 30 days
3. In the program, what happens when the user enters a negative rate per day?
a. The program calculates the salary normally
b. The program displays an error message and continues to run
c. The program crashes
d. The program prompts the user to enter a positive rate per day
4. How would you enhance the program to display salaries in a currency format, e.g., with a “$” symbol?
a. By implementing a complex currency conversion algorithm
b. By modifying the system’s regional settings
c. By using a built-in formatting feature to display currency
d. By writing a separate currency conversion program
5. What does the line Console.ReadKey(); do at the end of the program?
a. Closes the console window
b. Prompts the user to press a key
c. Saves the output to a file
d. Repeats the program
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.