Rectangle Perimeter Calculator in CSharp
Introduction
Table of Contents
Perimeter is the total length of the boundary of a two-dimensional shape. It is a measure of the distance around the outside of a shape. For a rectangle, the perimeter is calculated by adding the lengths of all its sides. In the case of a rectangle, the formula for calculating the perimeter is 2 times the sum of the length and width of the rectangle.
In this lesson, we delve into the fundamental concept of computing the perimeter of a rectangle using C#. The program allows us to understand the essential elements involved in calculating the perimeter of a geometric shape, emphasizing the significance of this process in various mathematical and practical contexts. By exploring the details of the code and its application, we aim to develop a comprehensive understanding of how to implement this geometric operation.
Objectives
- Understand the concept of perimeter in geometry and its significance in real-world scenarios. Apply:
- Apply the knowledge of perimeter calculation to solve problems and perform geometric operations in practical applications. Create:
- Develop a functional C# program to compute the perimeter of a rectangle, demonstrating proficiency in coding and algorithmic thinking. Upgrade:
- Explore and implement advanced features or modifications to the program, showcasing the ability to enhance the program’s functionality and performance.
Source code example
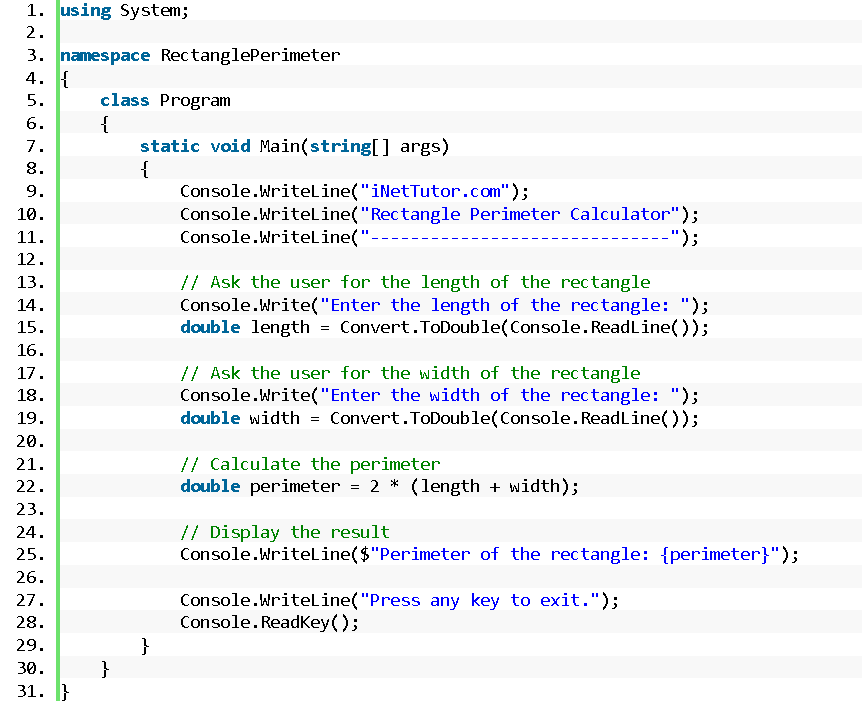
using System; namespace RectanglePerimeter { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com"); Console.WriteLine("Rectangle Perimeter Calculator"); Console.WriteLine("------------------------------"); // Ask the user for the length of the rectangle Console.Write("Enter the length of the rectangle: "); double length = Convert.ToDouble(Console.ReadLine()); // Ask the user for the width of the rectangle Console.Write("Enter the width of the rectangle: "); double width = Convert.ToDouble(Console.ReadLine()); // Calculate the perimeter double perimeter = 2 * (length + width); // Display the result Console.WriteLine($"Perimeter of the rectangle: {perimeter}"); Console.WriteLine("Press any key to exit."); Console.ReadKey(); } } }
Explanation
General explanation
This C# program calculates the perimeter of a rectangle based on user input for the length and width of the rectangle. It prompts the user to provide the length and width of the rectangle, converts the input to double data type, and then computes the perimeter using the formula: 2 * (length + width). The program then displays the computed perimeter, allowing the user to view the result. Finally, it prompts the user to press any key to exit the program.
Line by line explanation
- The first line of the code is an import statement that allows the program to use classes and methods from the “System” namespace, which contains fundamental classes and base classes.
- The “RectanglePerimeter” namespace groups related code together, helping to organize and manage the application.
- The “Program” class serves as the entry point of the program, containing the Main method, which is where the program execution begins.
- “Console.WriteLine” is used to print out the string “iNetTutor.com” as a header.
- The next line prints “Rectangle Perimeter Calculator” as a header.
- The comment “Ask the user for the length of the rectangle” is a descriptive note for the user’s understanding.
- “Console.Write” prompts the user to enter the length of the rectangle, and “Convert.ToDouble(Console.ReadLine())” takes the user input and converts it into a double data type, storing it in the “length” variable.
- Similarly, the program asks the user to input the width of the rectangle and stores the value in the “width” variable.
- The “perimeter” is calculated using the formula 2 * (length + width) and is stored in the “perimeter” variable.
- The Console.WriteLine statement displays the calculated perimeter to the user.
- Finally, the program waits for any key press to exit.
Output
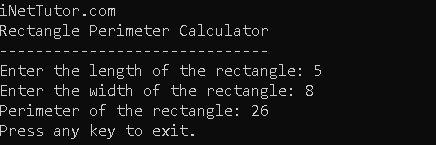
Summary
This lesson introduced the creation of a C# program to calculate the perimeter of a rectangle. Key concepts included user input collection, data type conversion, and performing calculations based on the provided inputs. The program demonstrated the utilization of the formula 2 * (length + width) to compute the perimeter. By exploring this program, learners can gain a better understanding of handling user input and performing basic calculations in C#. Experimenting with variations of the code can deepen their comprehension of these fundamental concepts.
Exercises and Assessment
Here are some suggested exercises and challenges for the lesson:
- Advanced Challenges:
- Implement error handling for cases where negative values are input for length or width.
- Enhance the program to calculate the area of the rectangle in addition to the perimeter.
- Enable the program to handle decimal values for length and width, ensuring accurate calculations.
- Further Enhancements:
- Develop a graphical user interface for the rectangle perimeter calculator.
- Create a version of the program that can calculate the perimeters of various shapes, including squares and triangles.
- Enable the program to calculate the diagonal length of the rectangle in addition to the perimeter.
Related Topics and Articles:
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.