PHP Form Handling
Introduction
Table of Contents
In this lesson, we will continue our discussion on web development by focusing on PHP form handling. PHP is a popular server-side programming language that is well-suited for handling form data.
We will start by discussing how to access form data in PHP using the $_GET and $_POST superglobals. We will then look at how to validate and sanitize the form data to prevent security risks such as SQL injection and XSS attacks.
We will also learn how to use PHP built-in functions to handle different types of form data, such as file uploads and email submissions.
By the end of this lesson, you will have a solid understanding of how to handle form data in PHP and how to use PHP to create secure and functional forms.
Objectives
- Understand how to access and process form data in PHP using the $_GET and $_POST superglobals.
- Learn how to validate and sanitize form data to prevent security risks such as SQL injection and XSS attacks.
By the end of this lesson, students should have a good understanding of how to access and process form data in PHP, how to validate and sanitize the data to prevent security risks, and how to use built-in functions to handle different types of form data. This will give them the skills they need to create secure and functional web applications that require user input and interaction.
Lesson Proper
Form handling in PHP refers to the process of receiving, validating, and processing form data on the server-side using PHP. Forms are a common way for users to interact with a website by entering and submitting data.
When a form is submitted, the form data is sent to the server, where it can be accessed and processed using PHP. In PHP, the $_GET and $_POST superglobals are used to access form data that is sent via the GET and POST methods, respectively.
The $_GET superglobal is used to access form data that is sent via the GET method. The GET method appends the form data to the URL, making it visible in the browser address bar. GET is typically used for forms that retrieve data, such as search forms.
For example, if a form has a text field with the name “search” and the user enters “PHP” into the field and submits the form, the form data will be appended to the URL like this: example.com/search.php?search=PHP. The $_GET superglobal can be used to access the “search” parameter and its value in the PHP script.
On the other hand, the $_POST superglobal is used to access form data that is sent via the POST method. The POST method sends the form data in the body of the HTTP request, making it invisible in the browser address bar. POST is typically used for forms that submit data, such as contact forms.
For example, if a contact form has fields for name, email, and message, the form data will be sent to the server in the body of the HTTP request and can be accessed using the $_POST superglobal.
In summary, form handling in PHP is the process of receiving, validating, and processing form data on the server-side using PHP. The $_GET and $_POST superglobals are used to access form data that is sent via the GET and POST methods, respectively. GET method is typically used for forms that retrieve data, while the POST method is typically used for forms that submit data.
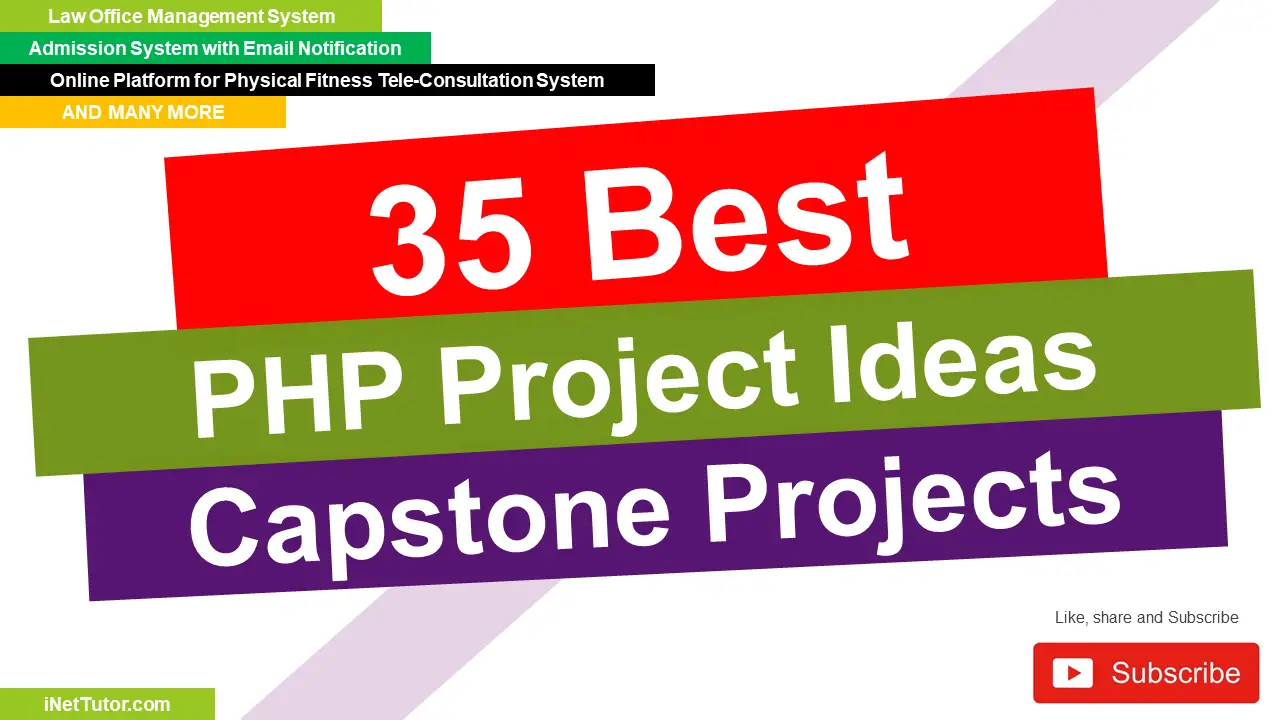
PHP Form Handling using $_POST
Here is an example of a PHP form that uses the $_POST superglobal to process form data:
<!DOCTYPE html> <html> <body> <form method="post" action="submit.php"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <br> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <br> <label for="message">Message:</label> <br> <textarea id="message" name="message" rows="5" cols="30"></textarea> <br> <input type="submit" value="Submit"> </form> </body> </html>
This form uses the <form> element with the method attribute set to “post” and the action attribute set to “submit.php”. This tells the browser to send the form data to the “submit.php” file on the server via the POST method.
The form also includes a text field for the name, an email field for the email, a textarea for the message, and a submit button. Each form control has a name attribute, which is used to identify the control when the form data is processed in PHP.
On the server-side, the “submit.php” file can access the form data using the $_POST superglobal. Here is an example of how the form data can be accessed and processed in the “submit.php” file:
<!DOCTYPE html> <html> <body> <?php if(isset($_POST["name"]) && isset($_POST["email"]) && isset($_POST["message"])) { $name = $_POST["name"]; $email = $_POST["email"]; $message = $_POST["message"]; echo "Name: " . $name . "<br>"; echo "Email: " . $email . "<br>"; echo "Message: " . $message; } ?> </body> </html>
This PHP script checks if the “name”, “email”, and “message” fields have been submitted with the form. If so, it assigns the values of the “name”, “email”, and “message” fields to variables, and then uses the echo statement to display the values on the page.
It’s important to note that this is a very basic example, in real-world scenarios you would want to validate and sanitize the data before using it, especially if you plan on storing the data in a database. You would also want to handle errors and exception, such as when the form is not submitted correctly, or when some fields are missing.
In summary, this is a basic example of how to create a PHP form using the $_POST superglobal to process form data. The form includes a text field for the name, an email field for the email, a textarea for the message, and a submit button. The form data is sent to the server via the POST method and can be accessed and processed in the “submit.php” file using the $_POST superglobal.
To execute the source code, you need to place the index.html and submit.php in one folder and name it as php_post. Next is to upload the folder in the web directory of xampp (xampp/htdocs). Lastly, type localhost/php_post in the address bar of the browser.
index.html
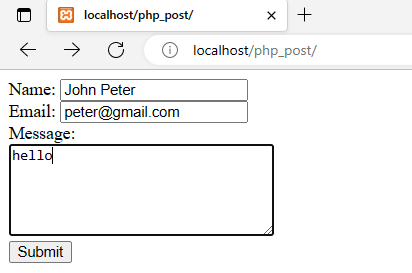
submit.php
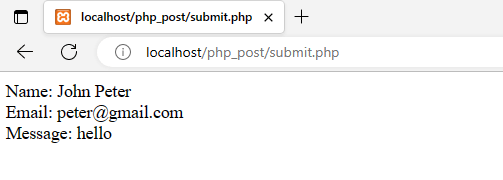
PHP Form Handling using $_GET
Here is an example of a PHP form that uses the $_GET superglobal to process form data:
<!DOCTYPE html> <html> <body> <form method="get" action="submit.php"> <label for="search">Search:</label> <input type="text" id="search" name="search" required> <input type="submit" value="Search"> </form> </body> </html>
This form uses the <form> element with the method attribute set to “get” and the action attribute set to “submit.php”. This tells the browser to append the form data to the URL and send it to the “submit.php” file on the server via the GET method.
The form also includes a text field for the search term, and a submit button. Each form control has a name attribute, which is used to identify the control when the form data is processed in PHP.
On the server-side, the “submit.php” file can access the form data using the $_GET superglobal. Here is an example of how the form data can be accessed and processed in the “submit.php” file:
<!DOCTYPE html> <html> <body> <?php if(isset($_GET["search"])) { $search_term = $_GET["search"]; echo "Search term: " . $search_term; } ?> </body> </html>
This PHP script checks if the “search” field has been submitted with the form. If so, it assigns the value of the “search” field to a variable, and then uses the echo statement to display the value on the page.
As with the previous example, in real-world scenarios, you would want to validate and sanitize the data before using it, especially if you plan on storing the data in a database. You would also want to handle errors and exceptions, such as when the form is not submitted correctly, or when some fields are missing.
In summary, this is an example of how to create a PHP form using the $_GET superglobal to process form data. The form includes a text field for the search term and a submit button. The form data is appended to the URL and sent to the server via the GET method and can be accessed and processed in the “submit.php” file using the $_GET superglobal.
To execute the source code, you need to place the index.html and submit.php in one folder and name it as php_get. Next is to upload the folder in the web directory of xampp (xampp/htdocs). Lastly, type localhost/php_get in the address bar of the browser.
index.html
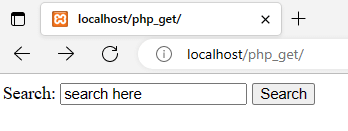
submit.php
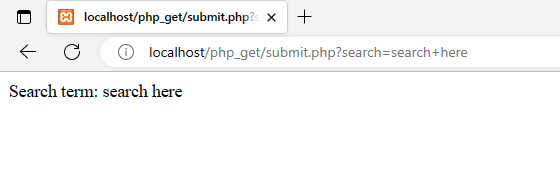
$_GET vs $_POST
The $_GET and $_POST superglobals are used to access form data that is sent to the server via the GET and POST methods, respectively.
The GET method appends the form data to the URL and sends it to the server as part of the request. The form data is visible in the browser address bar and can be cached by the browser. The GET method is typically used for forms that retrieve data, such as search forms. The $_GET superglobal can be used to access the form data in PHP.
On the other hand, the POST method sends the form data in the body of the HTTP request, making it invisible in the browser address bar. The POST method is typically used for forms that submit data, such as contact forms. The $_POST superglobal can be used to access the form data in PHP.
It’s important to note that the GET method has a limitation on the amount of data that can be sent and also the data is visible on the URL, that’s why it’s not recommended to use GET method for sensitive data, for example, passwords or credit card numbers.
Additionally, the GET method should be used only for retrieving data and it should not change the data in the server, while the POST method is used to submit data and it may change the data in the server.
In summary, the main difference between the $_GET and $_POST superglobals is the way they are used to access form data sent to the server via the GET and POST methods. The GET method is used to retrieve data and it appends the form data to the URL, while the POST method is used to submit data and sends it in the body of the HTTP request.
Summary
In this lesson, we discussed how to handle forms in PHP. We started by explaining what form handling in PHP is and the difference between the $_GET and $_POST superglobals. We explained that the $_GET superglobal is used to access form data that is sent via the GET method, which is typically used for forms that retrieve data, while the $_POST superglobal is used to access form data that is sent via the POST method, which is typically used for forms that submit data. We also provided examples of how to create a PHP form using both the $_GET and $_POST superglobals, and how to access and process form data in PHP. In general, it’s important to validate and sanitize the data before using it, especially if you plan on storing the data in a database.
Readers are also interested in:
PHP Course Outline for Beginners
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.