HTML Form Controls
Introduction
HTML forms are a fundamental part of many websites, providing a way for users to interact with the website and send data to the server. In this lesson, we will cover the basics of creating and using HTML forms. We will start by looking at the <form> element, which is used to create the form container. We will then explore different form controls such as text fields, checkboxes, radio buttons, and submit buttons, which are used to gather input from the user. Additionally, we will discuss the different attributes of the form element, such as action, method and enctype, and the attributes of the form controls, such as type, name, and value. Finally, we will look at how to process the form data on the server-side using PHP. By the end of this lesson, you will have a solid understanding of how to create functional and user-friendly HTML forms, and how to handle the data submitted by the user.
Objectives
By the end of this lesson, the student should be able to create functional and user-friendly forms using HTML, understand how the form elements and attributes work, and process the form data using PHP. This will give them a solid foundation for creating web applications that require user input and interaction.
- Understand the basic structure and elements of an HTML form, including the <form> element, form controls, and attributes such as action, method, and enctype.
- Ability to create various types of form controls such as text fields, checkboxes, radio buttons, and submit buttons, and understand the attributes of each control and how they affect the form’s functionality.
- Understand how to process the form data on the server-side using PHP, including retrieving data from form controls, validating and sanitizing the data, and storing the data in a database or text file.
Lesson Proper
HTML forms are created using various form controls, which are used to gather input from the user. Some of the most common form controls include:
Text fields: Text fields, also known as text boxes, allow users to enter a single line of text. They are created using the <input> element with the type attribute set to “text”. They can also be used for other types of data, such as passwords and email addresses, by setting the type attribute to “password” or “email” respectively.
Checkboxes: Checkboxes allow users to select multiple options from a list. They are created using the <input> element with the type attribute set to “checkbox”. Each checkbox has an associated name attribute, which allows multiple checkboxes to be grouped together and processed as a single field.
Radio buttons: Radio buttons allow users to select a single option from a list. They are created using the <input> element with the type attribute set to “radio”. Like checkboxes, radio buttons also have an associated name attribute, which groups them together.
Select boxes: Select boxes, also known as drop-down lists, allow users to select a single option from a list of options. They are created using the <select> element, which contains <option> elements for each option.
Textarea: Textarea allows users to enter multiple lines of text, it’s created using the <textarea> element
Buttons: Buttons are used to create a clickable button, it can be used as a submit button or a reset button, it’s created using the <button> element
Labels: Labels are used to define a label for an input element; it’s created using the <label> element
Fieldset: Fieldset is used to group related elements in a form; it’s created using the <fieldset> element
Each form control has its own attributes and properties, such as name, value, checked, selected and more, which can be used to configure the control and determine how the form data will be processed. Understanding the different types of form controls and their attributes is essential for creating functional and user-friendly forms.
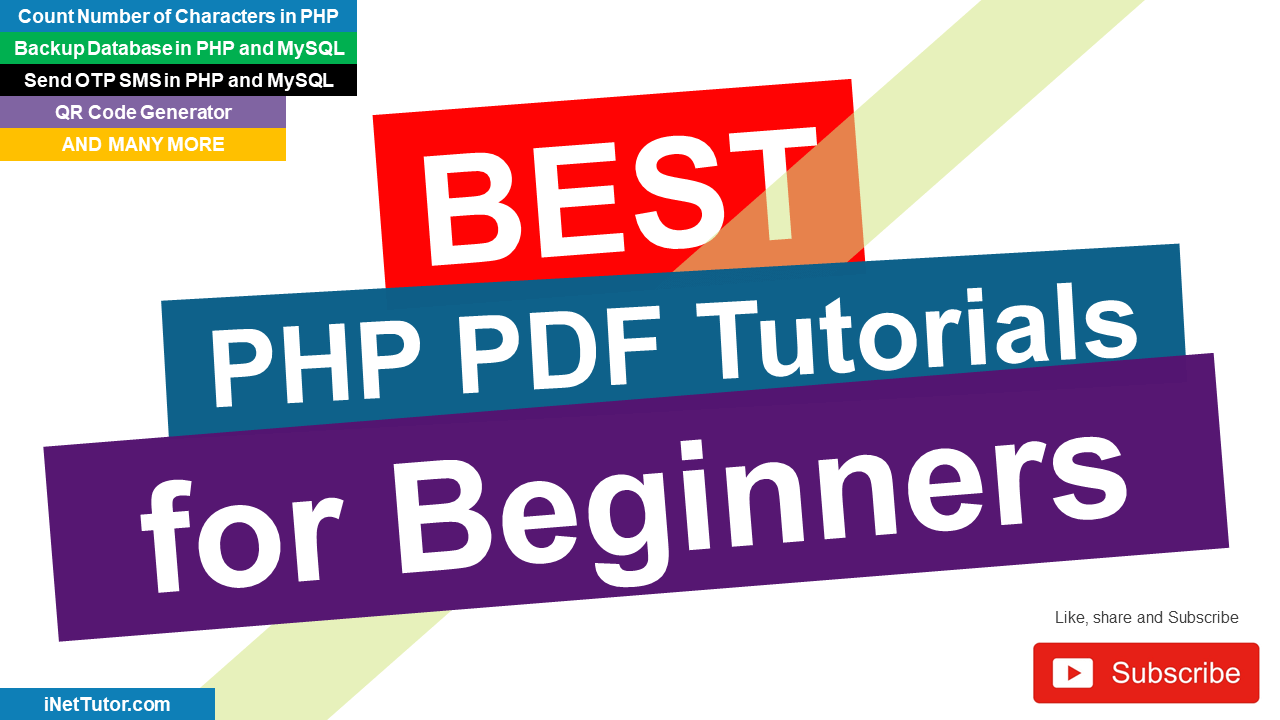
Example Source code
html_form.html
<!DOCTYPE html> <html> <body> <form> <!-- Text Field --> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <br> <!-- Text Field --> <!-- Checkbox Field --> <label for="fruit">Select your favorite fruits:</label> <br> <input type="checkbox" id="apple" name="fruit" value="apple"> <label for="apple">Apple</label> <br> <input type="checkbox" id="orange" name="fruit" value="orange"> <label for="orange">Orange</label> <br> <input type="checkbox" id="banana" name="fruit" value="banana"> <label for="banana">Banana</label> <br> <!-- Checkbox Field --> <!-- Radio Button --> <label for="gender">Select your gender:</label> <br> <input type="radio" id="male" name="gender" value="male"> <label for="male">Male</label> <br> <input type="radio" id="female" name="gender" value="female"> <label for="female">Female</label> <br> <!-- Radio Button --> <!-- Select box --> <label for="car">Select your car:</label> <br> <select id="car" name="car"> <option value="volvo">Volvo</option> <option value="saab">Saab</option> <option value="mercedes">Mercedes</option> <option value="audi">Audi</option> </select> <br> <!-- Select box --> <!-- Textarea --> <label for="message">Enter your message:</label> <br> <textarea id="message" name="message" rows="5" cols="30"></textarea> <br> <!-- Textarea --> <!-- Fieldset --> <fieldset> <legend> Personal information: </legend> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <br> <label for="email">Email:</label> <input type="email" id="email" name="email" required> </fieldset> <!-- Fieldset --> <!-- Button --> <button type="submit">Submit</button> <button type="reset">Reset</button> <!-- Button --> </form> </body> </html>
These are basic examples of the common form controls in HTML, they can be customized with CSS and JavaScript to enhance their appearance and functionality. Keep in mind that form validation and sanitization is important when handling the data submitted by the user, it should be handled on the server-side using PHP or other server-side languages.
Summary
In summary, this lesson provided an overview of how to create and use HTML forms, including understanding the different form controls, the <form> element and its attributes, form control attributes, and the importance of form validation and sanitization. By the end of this lesson, students should have a good understanding of how to create functional and user-friendly forms using HTML, as well as how to handle the data submitted by the user.
Exercise
An exercise or activity that can be done to reinforce the concepts covered in this lesson on HTML form controls could be creating a simple survey form. The exercise could be structured as follows:
- Students should create a form that includes a variety of form controls such as text fields, checkboxes, radio buttons, and a select box. The form should ask questions related to a topic of their choice.
- Students should use the <label> element to properly label each form control, and use the <fieldset> element to group related controls together.
- Students should use the <button> element to create a submit button and a reset button, and use the action and method attributes of the <form> element to specify the URL of the file that will process the form data and the HTTP method used to submit the form data.
- Students should use CSS to style the form and make it visually appealing.
- After the form is complete, students should test the form, validate the form data on the client-side using JavaScript, and process the data on the server-side using PHP.
This exercise will give students hands-on experience creating and styling HTML forms, and understanding how to use the different form controls and their attributes. Additionally, it will help students understand how to validate and process form data on the client-side and server-side, which are essential for creating web applications that require user input.
Readers are also interested in:
English Tagalog Online Dictionary using PHP
How to Display Date and Time in PHP
Best PHP PDF Tutorials for Beginners
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.