Count 1-5 in CSharp
Imagine writing a set of instructions in a computer language. Loops are like a magical spell that makes the computer repeat those instructions. In this lesson, we’ll focus on a special kind of loop called the do-while loop. It’s a bit different because it guarantees to do the instructions at least once. It’s like a friendly dance where the computer follows our lead.
So, let’s take a simple stroll into the loop world. We’ll talk about why loops are cool and then get to know this special do-while loop, understanding what makes it stand out. It’s all about making the computer do things in a friendly and reliable way. Ready to join the loop dance? Let’s take the first step together!
Introduction
Table of Contents
In this module, we will be exploring the do-while loop in C#. The do-while loop is a type of loop in programming that allows a block of code to be executed repeatedly until a certain condition becomes false. Unlike other loops, the do-while loop guarantees that the code block will be executed at least once, regardless of the condition.
Loops are an essential concept in programming as they allow us to perform repetitive tasks efficiently. In C#, there are different types of loops, including the while loop, for loop, and the do-while loop. Each loop has its own distinctive features and use cases.
The do-while loop in C# is similar to the while loop, but with one key difference. In a do-while loop, the condition is checked at the end of the loop, after the code block has been executed. This means that even if the condition is initially false, the code block will still be executed at least once. If the condition is true, the loop will continue to execute the code block until the condition becomes false.
Now that we have a brief overview of loops in programming and an introduction to the do-while loop, let’s dive deeper into its syntax and usage.
Syntax of Do-While Loop in C#
The syntax of the do-while loop in C# is as follows:
do { // code block to be executed } while (condition);
In this syntax, the code block is enclosed within curly braces {} and is followed by the while keyword and a condition in parentheses. The condition is evaluated after the code block has been executed. If the condition is true, the loop will continue to execute the code block. If the condition is false, the loop will terminate, and the program will proceed to the next statement after the loop.
Distinctive Features of the Do-While Loop
The do-while loop has some distinctive features that set it apart from other types of loops:
- Guaranteed Execution: The do-while loop guarantees that the code block will be executed at least once, regardless of the condition. This can be useful in situations where you want to ensure that a certain block of code is executed before checking the condition.
- Exit-Controlled Loop: The do-while loop is an exit-controlled loop, meaning that the condition is checked at the end of the loop. This allows the loop to execute the code block first and then evaluate the condition. If the condition is true, the loop will continue; otherwise, it will terminate.
- Flexible Usage: The do-while loop can be used in various scenarios where you need to repeat a certain task until a specific condition is met. It is particularly useful when you want to perform an action at least once and then repeat it based on a condition.
Objectives
In computer programming, loops are essential tools that allow us to repeat a set of instructions multiple times. One type of loop that we can utilize in C# is the do-while loop. Unlike other loop structures, the do-while loop guarantees that the instructions will be executed at least once before checking the loop condition. In this lesson, we will explore the concept and implementation of a do-while loop in C#, specifically focusing on using it to count from 1 to 5. By the end of this lesson, you will have a solid understanding of how to implement a do-while loop, recognize its syntax, and modify it to count in different patterns or increments. Let’s dive in and discover the power of the do-while loop in C#!
- Objective: Understand the concept and purpose of a do-while loop in C#.
- Outcome: Explain the purpose of a do-while loop and its difference from other loop structures.
- Assessment: Provide a written explanation of the concept and purpose of a do-while loop.
- Objective: Implement a do-while loop to count from 1 to 5 in C#.
- Outcome: Write a C# program that utilizes a do-while loop to count from 1 to 5.
- Assessment: Provide the code snippet of the C# program that uses a do-while loop to count from 1 to 5.
- Objective: Recognize the syntax and structure of a do-while loop in C#.
- Outcome: Identify the correct syntax and structure of a do-while loop in C#.
- Assessment: Identify and correct any errors in a given code snippet that uses a do-while loop.
- Objective: Explain the purpose and usage of loop control variables in a do-while loop.
- Outcome: Describe the purpose and usage of loop control variables in a do-while loop.
- Assessment: Provide a written explanation of how loop control variables are used in a do-while loop in C#.
- Objective: Modify a do-while loop to count in a different pattern or increment.
- Outcome: Modify a given code snippet that uses a do-while loop to count in a different pattern or increment.
- Assessment: Provide the modified code snippet that uses a do-while loop to count in a different pattern or increment.
By achieving these objectives, you will gain a thorough understanding of the concept and implementation of a do-while loop in C#, allowing you to effectively count from 1 to 5 using this loop structure.
Source code example
using System; namespace CountingExample { class Program { static void Main(string[] args) { Console.WriteLine("iNetTutor.com - Count 1-5 using do while"); int count = 1; // Initialize the count variable to 1 do { Console.WriteLine(count); // Print the current count value count++; // Increment the count by 1 } while (count <= 5); // Continue the loop as long as count is less than or equal to 5 Console.WriteLine("Press any key in the keyboard to close the console."); Console.ReadKey(); } } }
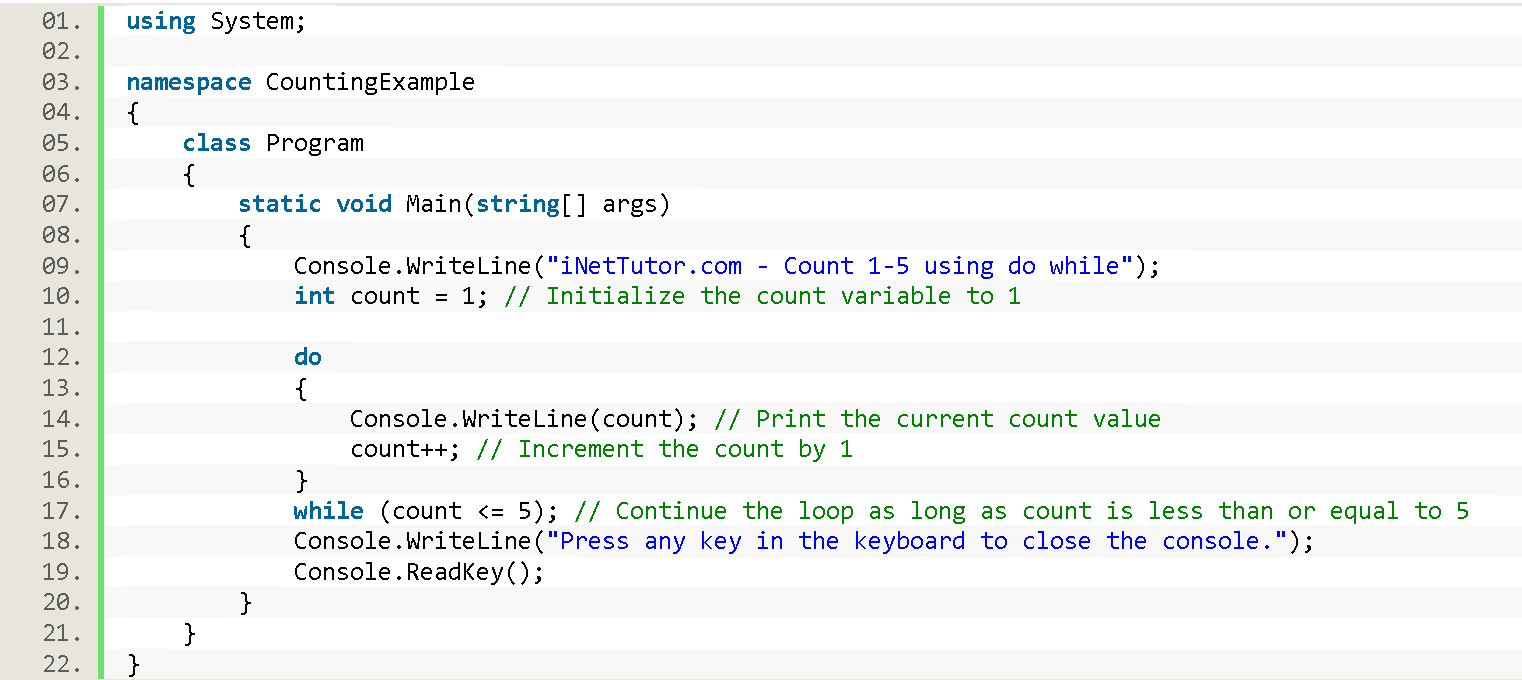
Explanation
The Main method serves as the entry point of the program. Inside the method, we declare and initialize a variable called count to 1. We then start the do-while loop, which will execute the code block enclosed in the curly braces at least once before checking the loop condition. Inside the loop, we use Console.WriteLine() to print the current value of count, and then increment count by 1 using the ++ operator. The loop continues as long as count is less than or equal to 5. Once count exceeds 5, the loop terminates, and the program execution ends.
Output
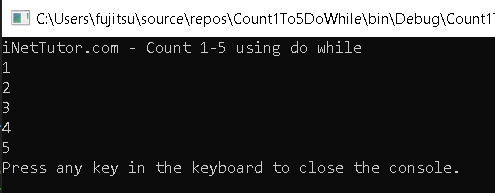
Summary
In this lesson, we explored the concept and implementation of a do-while loop in C#, specifically focusing on using it to count from 1 to 5. We learned that a do-while loop is a loop structure that guarantees the execution of a set of instructions at least once before checking the loop condition. We also examined the syntax and structure of a do-while loop, recognizing the importance of initializing loop control variables, defining the loop condition, and updating the loop control variables within the loop block. Through a hands-on example, we saw how to use a do-while loop to count from 1 to 5, printing each number along the way.
Conclusion:
By completing this lesson, you have gained a solid understanding of how to count from 1 to 5 using a do-while loop in C#. You have learned the purpose and difference of a do-while loop compared to other loop structures, recognized its syntax and structure, and successfully implemented a do-while loop to achieve the desired counting pattern. Additionally, you have explored the flexibility of the do-while loop by modifying it to count in different patterns or increments. This knowledge will serve as a foundation for utilizing do-while loops in various scenarios, allowing you to efficiently repeat a set of instructions until a specific condition is met. Keep practicing and applying this loop structure to enhance your programming skills and expand your problem-solving capabilities.
Exercises and Assessment
To improve the source code for counting from 1 to 5 using a do-while loop, you can consider the following exercises and activities:
- Reverse Counting: Modify the code to count down from 5 to 1 using a do-while loop. This exercise will help you practice changing the loop condition and decrementing the count variable.
- Custom Increment: Experiment with different increments when counting. For example, modify the code to count from 1 to 5 in increments of 2 or 3. This exercise will help you understand how to customize the loop increment and observe the resulting output.
- User Input: Enhance the code to allow user input for the count limit. Prompt the user to enter a number, and then use a do-while loop to count from 1 to the entered number. This exercise will help you practice accepting user input and incorporating it into your code logic.
- Enhanced Output: Modify the code to provide more meaningful output. Instead of simply printing the count number, display a message that includes the count. For example, print “Count: 1” instead of just “1”. This exercise will help you practice formatting output and making it more user-friendly.
- Nested Loop: Explore nesting a do-while loop within another loop. For instance, create a nested loop to print the count numbers in a pattern, such as a pyramid or a square. This exercise will help you understand how to structure and control nested loops effectively.
- Error Handling: Implement error handling for invalid user input. If the user enters a negative number or a non-numeric value, display an error message and prompt them to enter a valid input. This exercise will help you practice handling exceptions and ensuring the robustness of your code.
- Testing and Debugging: Perform thorough testing of your code and identify any potential issues or bugs. Use debugging techniques to step through the code and observe the program flow. This exercise will improve your ability to identify and resolve errors in your code.
By engaging in these exercises and activities, you can deepen your understanding of do-while loops, improve your code quality, and enhance your problem-solving skills. Remember to approach each exercise with curiosity and creativity, as it will help you grow as a programmer.
Related Topics and Articles:
C# Do While Loop Statement Video Tutorial and Source code
You may visit our Facebook page for more information, inquiries, and comments. Please subscribe also to our YouTube Channel to receive free capstone projects resources and computer programming tutorials.
Hire our team to do the project.