PDF Viewer in VB.NET Source code and Tutorial
Problem
Create a Windows Form Application program in Visual Basic.Net that will allow users to view pdf.
Description
This tutorial will allow the user to open and view a pdf file within the form of visual basic.
Before the tutorial the following are required to start:
- Microsoft Visual Studio 2008 – Above
- Edraw Office Viewer Component
The tutorial starts here:
- Download Edraw Office Viewer Component from the internet and save it to your preferred directory.
- Open Microsoft Visual Studio 2012
- Select a New Project on the File menu.
- Select Visual Basic, Windows Form Application then click OK.
- On the solution Panel, right click the “name of your project” then click add reference
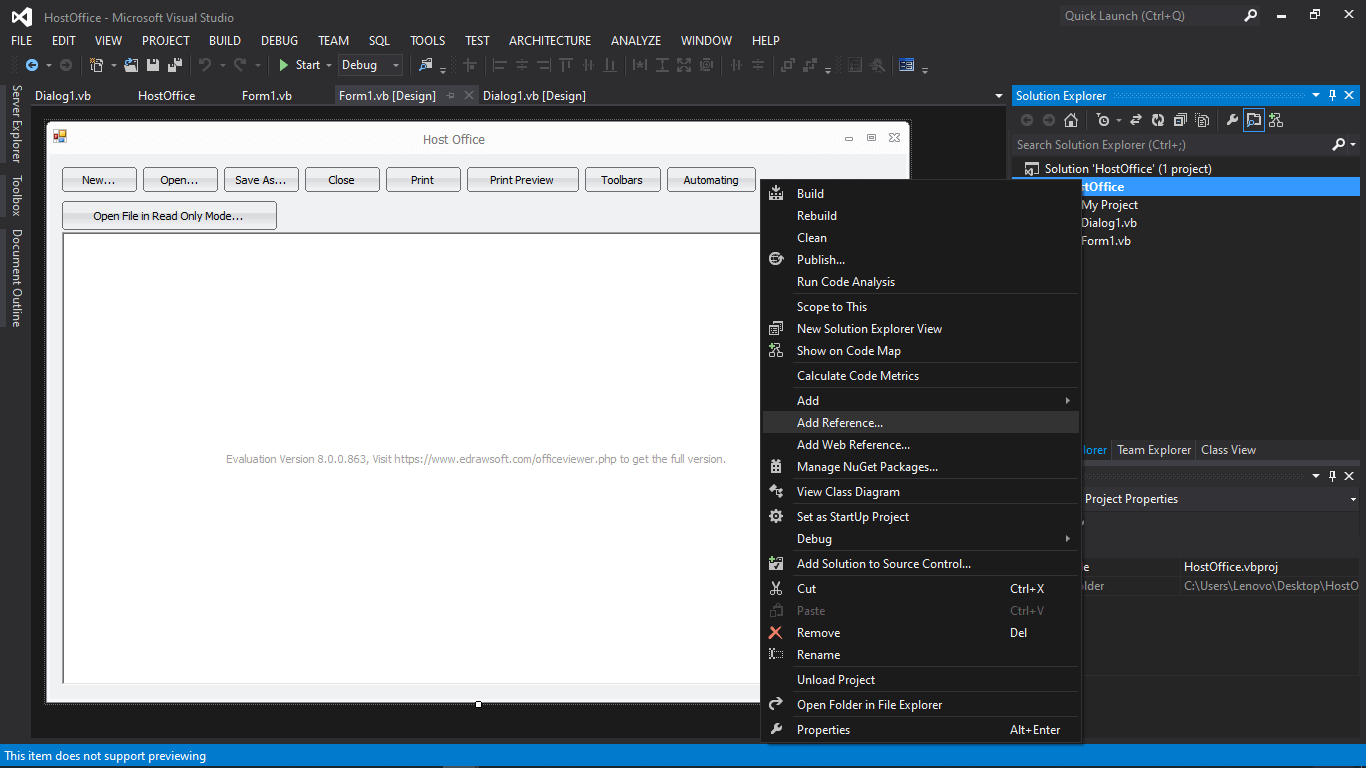
- On the reference manager, Click Browse on the left panel and check the Edraw Office Viewer Component, if it doesn’t appear, then click the Browse button on the bottom. Search and select for Edraw Office Viewer Component then click “Add”
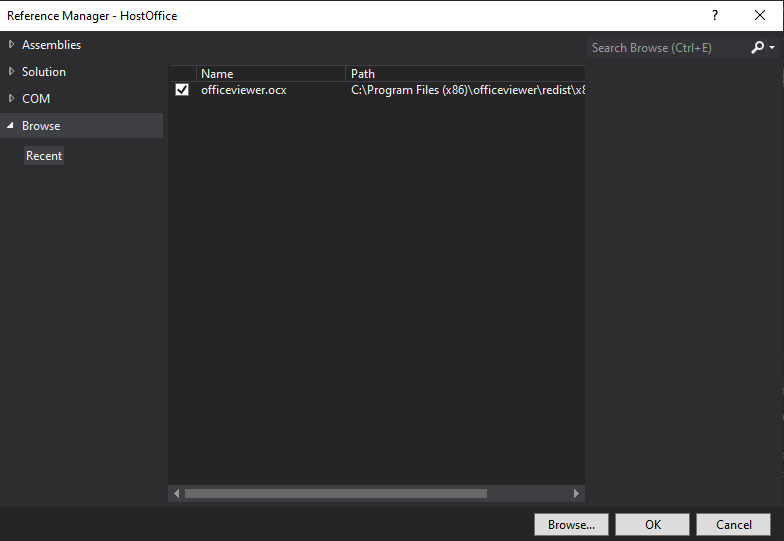
- Go to COM panel, look and check for Edraw Office Viewer Component then hit “Ok” and we are ready now to design our form
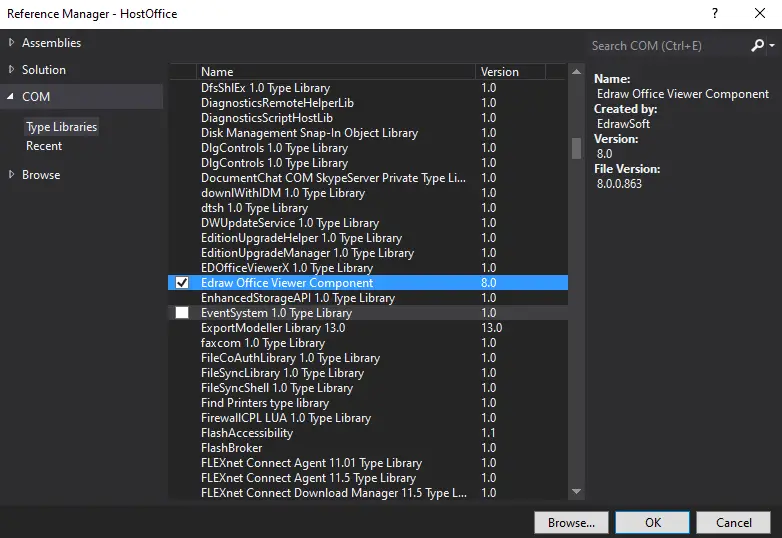
- We need the following for our forms:
For the first form:
- 9 Command Buttons – 9 buttons for New, Open, Save As, Close, Print, Print Preview, Toolbars, Automating, and Open file in read-only mode.
- 1 Office Viewer Component– picture box where the barcode is displayed.
For the second form:
- 5 Radio Buttons – 9 buttons for MS word, MS Excel, MS Power Point, MS Visio, and MS Project.
- 2 Command Buttons – 2 Command Buttons for OK and cancel
- We will also name our form controls in this way:
For the first form(form1):
- btnNew is the name of the command button for New.
- btnOpen is the name of the command button for Open.
- OpenReadOnly is the name of the command button for Open File Read-only mode.
- btnSaveAs is the name of the command button for save as.
- btnClose is the name of the command button for Close.
- btnPrint is the name of the command button for Print.
- btnPreview is the name of the command button for Print Preview.
- btnToolbars is the name of the command button for Toolbars.
- Automating is the name of the command button for Automating.
For the second form (dailog1):
- OK_Button is the name of the command button for OK.
- Cancel_Button is the name of the command button for Cancel.
- RadioButtonWord is the name of the radio button for MS word.
- RadioButtonExcel is the name of the radio button for MS Excel.
- RadioButtonPpt is the name of the radio button for MS Power point.
- RadioButtonVisio is the name of the radio button for MS Visio.
- RadioButtonProject is the name of the radio button for MS Project.
- This is how we design the form. (Feel free to layout your own)
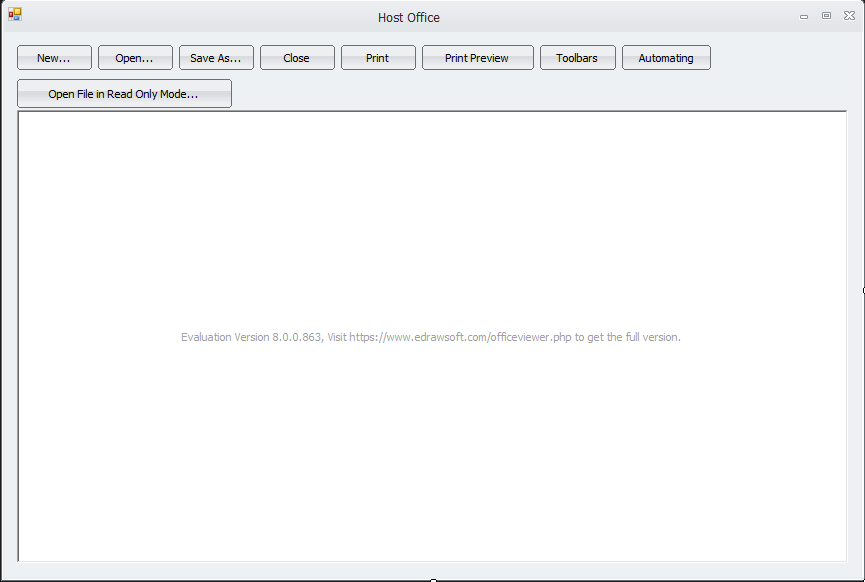
Figure 1. Design of the Form1
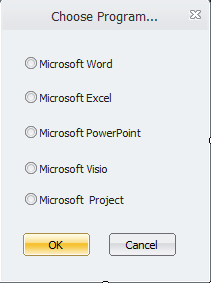
Figure 2. Design of the Dialog1
- Paste the following code to import the statement.
Code here
Imports Microsoft.Office.Interop.Word
End Code
Code Explanation:
The code imported above is to support Microsoft office word automation in the form.
- Paste the following codes for all the functions in each button at the first form.
Code here
Private Sub btnNew_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnNew.Click If Dialog1.ShowDialog() Then If Dialog1.GetChooseType() = 1 Then AxEDOffice1.CreateNew("Word.Application") ElseIf Dialog1.GetChooseType() = 2 Then AxEDOffice1.CreateNew("Excel.Application") ElseIf Dialog1.GetChooseType() = 3 Then AxEDOffice1.CreateNew("PowerPoint.Application") ElseIf Dialog1.GetChooseType() = 4 Then AxEDOffice1.CreateNew("Visio.Application") ElseIf Dialog1.GetChooseType() = 5 Then AxEDOffice1.CreateNew("MSProject.Application") End If End If End Sub Private Sub btnOpen_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOpen.Click AxEDOffice1.OpenFileDialog() End Sub Private Sub OpenReadOnly_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles OpenReadOnly.Click AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableNew, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableOpen, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableOfficeButton, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableSave, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableSaveAs, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableClose, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisablePrint, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisablePrintQuick, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisablePrintPreview, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableSaveAsMenu, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableCopyButton, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableCutButton, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableUpgradeDocument, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisablePermissionRestrictMenu, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisablePrepareMenu, True) AxEDOffice1.DisableFileCommand(EDOfficeLib.WdUIType.wdUIDisableServerTasksMenu, True) AxEDOffice1.OpenFileDialog() If AxEDOffice1.GetCurrentProgID = "Word.Application" Then AxEDOffice1.WordDisableCopyHotKey(True) AxEDOffice1.WordDisableDragAndDrop(True) AxEDOffice1.WordDisablePrintHotKey(True) AxEDOffice1.WordDisableSaveHotKey(True) AxEDOffice1.DisableViewRightClickMenu(True) AxEDOffice1.ProtectDoc(EDOfficeLib.WdProtectType.wdAllowOnlyFormFields) ElseIf AxEDOffice1.GetCurrentProgID = "Excel.Application" Then AxEDOffice1.WordDisableCopyHotKey(True) AxEDOffice1.WordDisablePrintHotKey(True) AxEDOffice1.WordDisableSaveHotKey(True) AxEDOffice1.DisableViewRightClickMenu(True) AxEDOffice1.ProtectDoc(EDOfficeLib.XlProtectType.XlProtectTypeNormal) End If End Sub Private Sub btnSaveAs_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSaveAs.Click AxEDOffice1.SaveFileDialog() End Sub Private Sub btnClose_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnClose.Click AxEDOffice1.CloseDoc() End Sub Private Sub btnPrint_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPrint.Click AxEDOffice1.PrintDialog() End Sub Private Sub btnPreview_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnPreview.Click AxEDOffice1.PrintPreview() End Sub Private Sub btnToolbars_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnToolbars.Click If AxEDOffice1.Toolbars = True Then AxEDOffice1.Toolbars = False Else AxEDOffice1.Toolbars = True End If End Sub Private Sub Automating_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Automating.Click If AxEDOffice1.GetCurrentProgID() = "Word.Application" Then Dim word = AxEDOffice1.GetApplication() word.Selection.TypeText("This the First text") ElseIf AxEDOffice1.GetCurrentProgID() = "Excel.Application" Then Dim excel = AxEDOffice1.ActiveDocument() Dim oSheet As Object = excel.Worksheets(1) 'Dim cellValue As String = oSheet.Range("A2").Value oSheet.Range("A2").Value = "This is the First Text" End If End Sub End Class
End Code
Code Explanation:
The code will give functions to each button in the form 1 that will enable the user to close/open or save/create a new document, print or show a print preview, open a file in read-only mode, and automate other MS Office Applications.
- In another form named dialog1 and paste the code to import a statement.
Code here
Imports System.Windows.Forms
End Code
Code Explanation:
The code imported above is a name space that contains classes for creating Windows-based applications.
- paste the following code for the command and radio buttons.
Code here
Dim nType As Integer = 1 Public Function GetChooseType() As Integer Return nType End Function Private Sub OK_Button_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles OK_Button.Click Me.DialogResult = System.Windows.Forms.DialogResult.OK Me.Close() End Sub Private Sub Cancel_Button_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Cancel_Button.Click Me.DialogResult = System.Windows.Forms.DialogResult.Cancel Me.Close() End Sub Private Sub RadioButtonWord_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButtonWord.CheckedChanged nType = 1 End Sub Private Sub RadioButtonExcel_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButtonExcel.CheckedChanged nType = 2 End Sub Private Sub RadioButtonPpt_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButtonPpt.CheckedChanged nType = 3 End Sub Private Sub RadioButtonVisio_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButtonVisio.CheckedChanged nType = 4 End Sub Private Sub RadioButtonProject_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButtonProject.CheckedChanged nType = 5 End Sub End Code
Code Explanation:
The ntype is an integer that will serve as a request from 1 (default) which is MS Word to 5 which is MS Project. When the OK command button is clicked, it will return the request to the form which application is going to be automated according the value of the ntype.
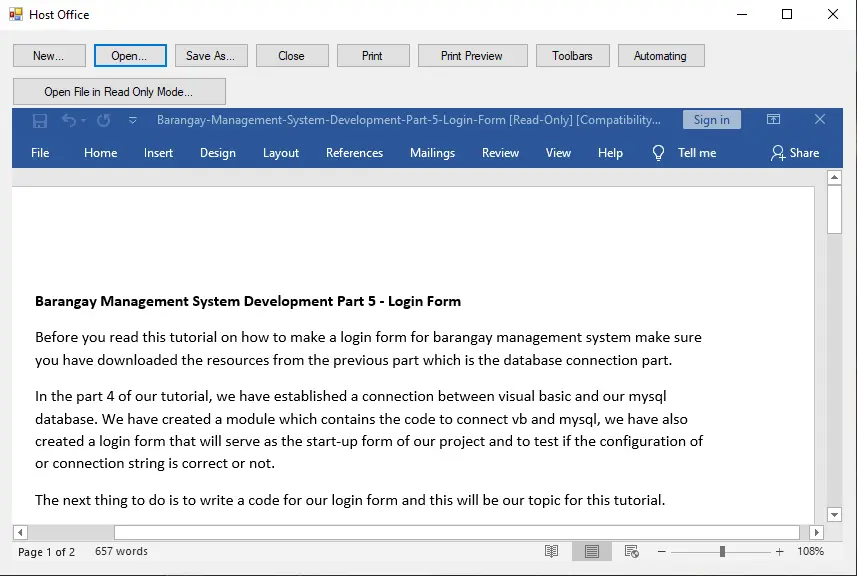
Author:
Name: Charlie Devera
Email Address: charliedevera000@gmail.com
Free Download Source code (PDF Viewer in VB.NET)
You may visit our facebook page for more information, inquiries and comments.
Hire our team to do the project.